
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
UsbEndpoint.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef USB_ENDPOINT_H 00025 #define USB_ENDPOINT_H 00026 00027 #include "mbed.h" 00028 #include "USBHostLite/usbhost_inc.h" 00029 00030 typedef int32_t RC; 00031 00032 class EdPool 00033 { 00034 public: 00035 EdPool(int size); 00036 ~EdPool(); 00037 HCED* get(); 00038 private: 00039 uint8_t* m_pool; 00040 int m_pos; 00041 00042 }; 00043 00044 class TdPool 00045 { 00046 public: 00047 TdPool(int size); 00048 ~TdPool(); 00049 HCTD* get(); 00050 private: 00051 uint8_t* m_pool; 00052 int m_pos; 00053 00054 }; 00055 00056 class UsbEndpoint 00057 { 00058 public: 00059 UsbEndpoint( /* UsbDevice*, */ uint8_t ep, bool dir, uint16_t size ); 00060 ~UsbEndpoint(); 00061 00062 RC transfer(volatile uint8_t* buf, uint32_t len); 00063 00064 RC status(); 00065 00066 //static void completed(){} 00067 00068 private: 00069 EdPool m_edPool; 00070 TdPool m_tdPool; 00071 00072 bool m_dir; 00073 00074 bool m_done; 00075 00076 uint32_t m_len; 00077 00078 volatile uint8_t* m_pBufStartPtr; 00079 00080 volatile HCED* m_pEd; //Ep descriptor 00081 volatile HCTD* m_pTdHead; //Head trf descriptor 00082 volatile HCTD* m_pTdTail; //Tail trf descriptor 00083 00084 //static volatile HCED* m_pNextEd; 00085 00086 }; 00087 00088 #endif
Generated on Tue Jul 12 2022 18:38:38 by
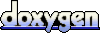