
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
SerialBuf.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef SERIALBUF_H 00025 #define SERIALBUF_H 00026 00027 #include "mbed.h" 00028 #include "netCfg.h" 00029 #if NET_USB_SERIAL 00030 #include "drv/serial/usb/UsbSerial.h" 00031 #endif 00032 00033 class SerialBuf 00034 { 00035 public: 00036 SerialBuf(int len); //Buffer length 00037 ~SerialBuf(); 00038 00039 void attach(Serial* pSerial); 00040 void detach(); 00041 00042 #if NET_USB_SERIAL 00043 void attach(UsbSerial* pUsbSerial); 00044 #endif 00045 00046 //Really useful for debugging 00047 char getc(); 00048 void putc(char c); 00049 bool readable(); 00050 bool writeable(); 00051 /*protected:*/ 00052 void setReadMode(bool readMode); //If true, keeps chars in buf when read, false by default 00053 void flushRead(); //Delete chars that have been read & return chars len (only useful with readMode = true) 00054 void resetRead(); //Go back to initial read position & return chars len (only useful with readMode = true) 00055 protected: 00056 virtual bool onRead();// = 0; //Called when new bytes are received : WARN: executed in an interrupt context >> only fast & non-blocking code (eg no printf;)) 00057 //return true if handled, false otherwise 00058 private: 00059 void onSerialInterrupt(); //Callback from m_pSerial 00060 00061 volatile bool m_trmt; //For debugging (Was transmitting?) 00062 00063 Serial* m_pSerial; //Not owned 00064 00065 char get(); //Get a char from buf 00066 void put(char c); //Put a char in buf 00067 int room(); //Return room available in buf 00068 int len(); //Return chars len in buf 00069 00070 char* m_buf; 00071 int m_bufLen; 00072 volatile char* m_pReadStart; 00073 volatile char* m_pRead; 00074 00075 volatile char* m_pReadByInt; //Chars read during interrupt 00076 volatile bool m_intCanReadData; 00077 00078 volatile char* m_pWrite; 00079 volatile bool m_readMode; 00080 00081 #if NET_USB_SERIAL 00082 //USB Serial Impl 00083 UsbSerial* m_pUsbSerial; //Not owned 00084 Ticker m_usbTick; 00085 #endif 00086 }; 00087 00088 00089 #endif
Generated on Tue Jul 12 2022 18:38:38 by
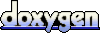