
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
PPPNetIf.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "PPPNetIf.h" 00025 #include "mbed.h" 00026 #include "ppp/ppp.h" 00027 #include "lwip/init.h" 00028 #include "lwip/sio.h" 00029 00030 #define __DEBUG 00031 #include "dbg/dbg.h" 00032 00033 #include "netCfg.h" 00034 #if NET_PPP 00035 00036 #define PPP_TIMEOUT 60000 00037 00038 #define BUF_SIZE 128 00039 00040 PPPNetIf::PPPNetIf(GPRSModem* pIf) : LwipNetIf(), m_pIf(pIf),/* m_open(false),*/ m_connected(false), m_status(PPP_DISCONNECTED), m_fd(0) //, m_id(0) 00041 { 00042 //FIXME: Check static refcount 00043 pppInit(); 00044 m_buf = new uint8_t[BUF_SIZE]; 00045 } 00046 00047 PPPNetIf::~PPPNetIf() 00048 { 00049 delete[] m_buf; 00050 } 00051 00052 #if 0 00053 PPPErr PPPNetIf::open(Serial* pSerial) 00054 { 00055 GPRSErr err = m_pIf->open(pSerial); 00056 if(err) 00057 return PPP_MODEM; 00058 m_open = true; 00059 #if 0 00060 m_id = sioMgr::registerSerialIf(this); 00061 if(!m_id) 00062 { 00063 close(); 00064 return PPP_CLOSED; 00065 } 00066 #endif 00067 return PPP_OK; 00068 } 00069 #endif 00070 00071 00072 PPPErr PPPNetIf::GPRSConnect(const char* apn, const char* userId, const char* password) //Connect using GPRS 00073 { 00074 LwipNetIf::init(); 00075 //TODO: Tell ATIf that we get ownership of the serial port 00076 00077 GPRSErr gprsErr; 00078 gprsErr = m_pIf->connect(apn); 00079 if(gprsErr) 00080 return PPP_NETWORK; 00081 00082 DBG("\r\nPPPNetIf: If Connected.\r\n"); 00083 00084 if( userId == NULL ) 00085 pppSetAuth(PPPAUTHTYPE_NONE, NULL, NULL); 00086 else 00087 pppSetAuth(PPPAUTHTYPE_PAP, userId, password); //TODO: Allow CHAP as well 00088 00089 DBG("\r\nPPPNetIf: Set Auth.\r\n"); 00090 00091 m_pIf->flushBuffer(); //Flush buffer before passing serial port to PPP 00092 00093 m_status = PPP_CONNECTING; 00094 int res = pppOverSerialOpen((void*)m_pIf, sPppCallback, (void*)this); 00095 if(res<0) 00096 { 00097 disconnect(); 00098 return PPP_PROTOCOL; 00099 } 00100 00101 DBG("\r\nPPPNetIf: PPP Started with res = %d.\r\n", res); 00102 00103 m_fd = res; 00104 m_connected = true; 00105 Timer t; 00106 t.start(); 00107 while( m_status == PPP_CONNECTING ) //Wait for callback 00108 { 00109 poll(); 00110 if(t.read_ms()>PPP_TIMEOUT) 00111 { 00112 DBG("\r\nPPPNetIf: Timeout.\r\n"); 00113 disconnect(); 00114 return PPP_PROTOCOL; 00115 } 00116 } 00117 00118 DBG("\r\nPPPNetIf: Callback returned.\r\n"); 00119 00120 if( m_status == PPP_DISCONNECTED ) 00121 { 00122 disconnect(); 00123 return PPP_PROTOCOL; 00124 } 00125 00126 return PPP_OK; 00127 00128 } 00129 00130 PPPErr PPPNetIf::ATConnect(const char* number) //Connect using a "classic" voice modem or GSM 00131 { 00132 //TODO: IMPL 00133 return PPP_MODEM; 00134 } 00135 00136 PPPErr PPPNetIf::disconnect() 00137 { 00138 pppClose(m_fd); //0 if ok, else should gen a WARN 00139 m_connected = false; 00140 00141 m_pIf->flushBuffer(); 00142 m_pIf->printf("+++"); 00143 wait(.1); 00144 m_pIf->flushBuffer(); 00145 00146 GPRSErr gprsErr; 00147 gprsErr = m_pIf->disconnect(); 00148 if(gprsErr) 00149 return PPP_NETWORK; 00150 00151 return PPP_OK; 00152 } 00153 00154 #if 0 00155 PPPErr PPPNetIf::close() 00156 { 00157 GPRSErr err = m_pIf->close(); 00158 if(err) 00159 return PPP_MODEM; 00160 m_open = false; 00161 return PPP_OK; 00162 } 00163 #endif 00164 00165 00166 #if 0 00167 //We have to use : 00168 00169 /** Pass received raw characters to PPPoS to be decoded. This function is 00170 * thread-safe and can be called from a dedicated RX-thread or from a main-loop. 00171 * 00172 * @param pd PPP descriptor index, returned by pppOpen() 00173 * @param data received data 00174 * @param len length of received data 00175 */ 00176 void 00177 pppos_input(int pd, u_char* data, int len) 00178 { 00179 pppInProc(&pppControl[pd].rx, data, len); 00180 } 00181 #endif 00182 00183 void PPPNetIf::poll() 00184 { 00185 if(!m_connected) 00186 return; 00187 LwipNetIf::poll(); 00188 //static u8_t buf[128]; 00189 int len = sio_tryread((sio_fd_t) m_pIf, m_buf, BUF_SIZE); 00190 if(len > 0) 00191 pppos_input(m_fd, m_buf, len); 00192 } 00193 00194 //Link Callback 00195 void PPPNetIf::pppCallback(int errCode, void *arg) 00196 { 00197 //DBG("\r\nPPPNetIf: Callback errCode = %d.\r\n", errCode); 00198 switch ( errCode ) 00199 { 00200 //No error 00201 case PPPERR_NONE: 00202 m_status = PPP_CONNECTED; 00203 break; 00204 default: 00205 //Disconnected 00206 m_status = PPP_DISCONNECTED; 00207 break; 00208 } 00209 } 00210 00211 #endif
Generated on Tue Jul 12 2022 18:38:37 by
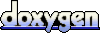