
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
NTPClient.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef NTP_CLIENT_H 00025 #define NTP_CLIENT_H 00026 00027 #include "if/net/net.h" 00028 #include "api/UDPSocket.h" 00029 #include "api/DNSRequest.h" 00030 #include "mbed.h" 00031 00032 enum NTPResult 00033 { 00034 NTP_OK, 00035 NTP_PROCESSING, 00036 NTP_PRTCL, //Protocol error 00037 NTP_TIMEOUT, //Connection timeout 00038 NTP_DNS //Could not resolve DNS Addr 00039 }; 00040 00041 class NTPClient : protected NetService 00042 { 00043 public: 00044 NTPClient(); 00045 virtual ~NTPClient(); 00046 00047 //High level setup functions 00048 NTPResult setTime(const Host& host); //Blocking 00049 NTPResult setTime(const Host& host, void (*pMethod)(NTPResult)); //Non blocking 00050 template<class T> 00051 NTPResult setTime(const Host& host, T* pItem, void (T::*pMethod)(NTPResult)) //Non blocking 00052 { 00053 setOnResult(pItem, pMethod); 00054 doSetTime(host); 00055 return NTP_PROCESSING; 00056 } 00057 00058 void doSetTime(const Host& host); 00059 00060 void setOnResult( void (*pMethod)(NTPResult) ); 00061 class CDummy; 00062 template<class T> 00063 void setOnResult( T* pItem, void (T::*pMethod)(NTPResult) ) 00064 { 00065 m_pCbItem = (CDummy*) pItem; 00066 m_pCbMeth = (void (CDummy::*)(NTPResult)) pMethod; 00067 } 00068 00069 void close(); 00070 00071 protected: 00072 virtual void poll(); //Called by NetServices 00073 00074 private: 00075 void init(); 00076 void open(); 00077 00078 __packed struct NTPPacket //See RFC 4330 for Simple NTP 00079 { 00080 //WARN: We are in LE! Network is BE! 00081 //LSb first 00082 unsigned mode : 3; 00083 unsigned vn : 3; 00084 unsigned li : 2; 00085 00086 uint8_t stratum; 00087 uint8_t poll; 00088 uint8_t precision; 00089 //32 bits header 00090 00091 uint32_t rootDelay; 00092 uint32_t rootDispersion; 00093 uint32_t refId; 00094 00095 uint32_t refTm_s; 00096 uint32_t refTm_f; 00097 uint32_t origTm_s; 00098 uint32_t origTm_f; 00099 uint32_t rxTm_s; 00100 uint32_t rxTm_f; 00101 uint32_t txTm_s; 00102 uint32_t txTm_f; 00103 }; 00104 00105 void process(); //Main state-machine 00106 00107 void setTimeout(int ms); 00108 void resetTimeout(); 00109 00110 void onTimeout(); //Connection has timed out 00111 void onDNSReply(DNSReply r); 00112 void onUDPSocketEvent(UDPSocketEvent e); 00113 void onResult(NTPResult r); //Called when exchange completed or on failure 00114 00115 NTPResult blockingProcess(); //Called in blocking mode, calls Net::poll() until return code is available 00116 00117 UDPSocket* m_pUDPSocket; 00118 00119 enum NTPStep 00120 { 00121 NTP_PING, 00122 NTP_PONG 00123 }; 00124 00125 NTPStep m_state; 00126 00127 NTPPacket m_pkt; 00128 00129 CDummy* m_pCbItem; 00130 void (CDummy::*m_pCbMeth)(NTPResult); 00131 00132 void (*m_pCb)(NTPResult); 00133 00134 Timer m_watchdog; 00135 int m_timeout; 00136 00137 bool m_closed; 00138 00139 Host m_host; 00140 00141 DNSRequest* m_pDnsReq; 00142 00143 NTPResult m_blockingResult; //Result if blocking mode 00144 00145 }; 00146 00147 #endif
Generated on Tue Jul 12 2022 18:38:37 by
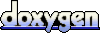