
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
MySQLClient.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef MYSQL_CLIENT_H 00025 #define MYSQL_CLIENT_H 00026 00027 #include "if/net/net.h" 00028 #include "api/TCPSocket.h" 00029 #include "api/DNSRequest.h" 00030 #include "mbed.h" 00031 00032 #include <string> 00033 using std::string; 00034 00035 #include <map> 00036 using std::map; 00037 00038 typedef unsigned char byte; 00039 00040 enum MySQLResult 00041 { 00042 MYSQL_OK, 00043 MYSQL_PROCESSING, 00044 MYSQL_PRTCL, 00045 MYSQL_SETUP, //Not properly configured 00046 MYSQL_DNS, //Could not resolve name 00047 MYSQL_AUTHFAILED, //Auth failure 00048 MYSQL_READY, //Ready to send commands 00049 MYSQL_SQL, //SQL Error 00050 MYSQL_TIMEOUT, //Connection timeout 00051 MYSQL_CONN //Connection error 00052 }; 00053 00054 class MySQLClient : protected NetService 00055 { 00056 public: 00057 MySQLClient(); 00058 virtual ~MySQLClient(); 00059 00060 //High Level setup functions 00061 MySQLResult open(Host& host, const string& user, const string& password, const string& db, void (*pMethod)(MySQLResult)); //Non blocking 00062 template<class T> 00063 MySQLResult open(Host& host, const string& user, const string& password, const string& db, T* pItem, void (T::*pMethod)(MySQLResult)) //Non blocking 00064 { 00065 setOnResult(pItem, pMethod); 00066 setup(host, user, password, db); 00067 return MYSQL_PROCESSING; 00068 } 00069 00070 MySQLResult sql(string& sqlCommand); 00071 00072 MySQLResult exit(); 00073 00074 void setOnResult( void (*pMethod)(MySQLResult) ); 00075 class CDummy; 00076 template<class T> 00077 void setOnResult( T* pItem, void (T::*pMethod)(MySQLResult) ) 00078 { 00079 m_pCb = NULL; 00080 m_pCbItem = (CDummy*) pItem; 00081 m_pCbMeth = (void (CDummy::*)(MySQLResult)) pMethod; 00082 } 00083 00084 void setTimeout(int ms); 00085 00086 virtual void poll(); //Called by NetServices 00087 00088 protected: 00089 void resetTimeout(); 00090 00091 void init(); 00092 void close(); 00093 00094 void setup(Host& host, const string& user, const string& password, const string& db); //Setup connection, make DNS Req if necessary 00095 void connect(); //Start Connection 00096 00097 void handleHandshake(); 00098 void sendAuth(); 00099 00100 void handleAuthResult(); 00101 void sendAuth323(); 00102 00103 void sendCommand(byte command, byte* arg, int len); 00104 void handleCommandResult(); 00105 00106 void readData(); //Copy to buf 00107 void writeData(); //Copy from buf 00108 00109 void onTCPSocketEvent(TCPSocketEvent e); 00110 void onDNSReply(DNSReply r); 00111 void onResult(MySQLResult r); //Called when exchange completed or on failure 00112 void onTimeout(); //Connection has timed out 00113 00114 private: 00115 CDummy* m_pCbItem; 00116 void (CDummy::*m_pCbMeth)(MySQLResult); 00117 00118 void (*m_pCb)(MySQLResult); 00119 00120 TCPSocket* m_pTCPSocket; 00121 00122 Timer m_watchdog; 00123 int m_timeout; 00124 00125 DNSRequest* m_pDnsReq; 00126 00127 bool m_closed; 00128 00129 enum MySQLStep 00130 { 00131 // MYSQL_INIT, 00132 MYSQL_HANDSHAKE, 00133 MYSQL_AUTH, 00134 MYSQL_COMMANDS, 00135 MYSQL_CLOSED 00136 }; 00137 00138 //Parameters 00139 Host m_host; 00140 00141 string m_user; 00142 string m_password; 00143 string m_db; 00144 00145 //Low-level buffers & state-machine 00146 MySQLStep m_state; 00147 00148 byte* m_buf; 00149 byte* m_pPos; 00150 int m_len; 00151 int m_size; 00152 00153 int m_packetId; 00154 00155 }; 00156 00157 #endif
Generated on Tue Jul 12 2022 18:38:37 by
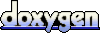