
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
HTTPFile.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "HTTPFile.h" 00025 00026 HTTPFile::HTTPFile(const char* path) : HTTPData(), m_fp(NULL), m_path(path), m_len(0), m_chunked(false) 00027 { 00028 00029 } 00030 00031 HTTPFile::~HTTPFile() 00032 { 00033 closeFile(); 00034 } 00035 00036 void HTTPFile::clear() 00037 { 00038 closeFile(); 00039 //Force reopening 00040 } 00041 00042 int HTTPFile::read(char* buf, int len) 00043 { 00044 if(!openFile("r")) //File does not exist, or I/O error... 00045 return 0; 00046 len = fread(buf, 1, len, m_fp); 00047 if( feof(m_fp) ) 00048 { 00049 //File read completely, we can close it 00050 closeFile(); 00051 } 00052 return len; 00053 } 00054 00055 int HTTPFile::write(const char* buf, int len) 00056 { 00057 if(!openFile("w")) //File does not exist, or I/O error... 00058 return 0; 00059 len = fwrite(buf, 1, len, m_fp); 00060 if( (!m_chunked && (ftell(m_fp) >= m_len)) || 00061 (m_chunked && !len) ) 00062 { 00063 //File received completely, we can close it 00064 closeFile(); 00065 } 00066 return len; 00067 } 00068 00069 string HTTPFile::getDataType() //Internet media type for Content-Type header 00070 { 00071 return ""; //Unknown 00072 } 00073 00074 void HTTPFile::setDataType(const string& type) //Internet media type from Content-Type header 00075 { 00076 //Do not really care here 00077 } 00078 00079 bool HTTPFile::getIsChunked() //For Transfer-Encoding header 00080 { 00081 return false; 00082 } 00083 00084 void HTTPFile::setIsChunked(bool chunked) //From Transfer-Encoding header 00085 { 00086 m_chunked = chunked; 00087 } 00088 00089 int HTTPFile::getDataLen() //For Content-Length header 00090 { 00091 return m_len; 00092 } 00093 00094 void HTTPFile::setDataLen(int len) //From Content-Length header, or if the transfer is chunked, next chunk length 00095 { 00096 if(!m_chunked) 00097 m_len = len; //Useful so that we can close file when last byte is written 00098 } 00099 00100 bool HTTPFile::openFile(const char* mode) //true on success, false otherwise 00101 { 00102 if(m_fp) 00103 return true; 00104 00105 m_fp = fopen(m_path.c_str(), mode); 00106 if(m_fp && mode[0]=='r') 00107 { 00108 //Seek EOF to get length 00109 fseek(m_fp, 0, SEEK_END); 00110 m_len = ftell(m_fp); 00111 fseek(m_fp, 0, SEEK_SET); //Goto SOF 00112 } 00113 00114 if(!m_fp) 00115 return false; 00116 00117 return true; 00118 } 00119 00120 void HTTPFile::closeFile() 00121 { 00122 if(m_fp) 00123 fclose(m_fp); 00124 }
Generated on Tue Jul 12 2022 18:38:36 by
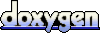