
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
HTTPClient.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef HTTP_CLIENT_H 00025 #define HTTP_CLIENT_H 00026 00027 class HTTPData; 00028 00029 #include "if/net/net.h" 00030 #include "api/TCPSocket.h" 00031 #include "api/DNSRequest.h" 00032 #include "HTTPData.h" 00033 #include "mbed.h" 00034 00035 #include <string> 00036 using std::string; 00037 00038 #include <map> 00039 using std::map; 00040 00041 enum HTTPResult 00042 { 00043 HTTP_OK, 00044 HTTP_PROCESSING, 00045 HTTP_PARSE, //URI Parse error 00046 HTTP_DNS, //Could not resolve name 00047 HTTP_PRTCL, //Protocol error 00048 HTTP_NOTFOUND, //404 Error 00049 HTTP_REFUSED, //403 Error 00050 HTTP_ERROR, //xxx error 00051 HTTP_TIMEOUT, //Connection timeout 00052 HTTP_CONN //Connection error 00053 }; 00054 00055 00056 00057 class HTTPClient : protected NetService 00058 { 00059 public: 00060 HTTPClient(); 00061 virtual ~HTTPClient(); 00062 00063 void basicAuth(const char* user, const char* password); //Basic Authentification 00064 00065 //High Level setup functions 00066 HTTPResult get(const char* uri, HTTPData* pDataIn); //Blocking 00067 HTTPResult get(const char* uri, HTTPData* pDataIn, void (*pMethod)(HTTPResult)); //Non blocking 00068 template<class T> 00069 HTTPResult get(const char* uri, HTTPData* pDataIn, T* pItem, void (T::*pMethod)(HTTPResult)) //Non blocking 00070 { 00071 setOnResult(pItem, pMethod); 00072 doGet(uri, pDataIn); 00073 return HTTP_PROCESSING; 00074 } 00075 00076 HTTPResult post(const char* uri, const HTTPData& dataOut, HTTPData* pDataIn); //Blocking 00077 HTTPResult post(const char* uri, const HTTPData& dataOut, HTTPData* pDataIn, void (*pMethod)(HTTPResult)); //Non blocking 00078 template<class T> 00079 HTTPResult post(const char* uri, const HTTPData& dataOut, HTTPData* pDataIn, T* pItem, void (T::*pMethod)(HTTPResult)) //Non blocking 00080 { 00081 setOnResult(pItem, pMethod); 00082 doPost(uri, dataOut, pDataIn); 00083 return HTTP_PROCESSING; 00084 } 00085 00086 void doGet(const char* uri, HTTPData* pDataIn); 00087 void doPost(const char* uri, const HTTPData& dataOut, HTTPData* pDataIn); 00088 00089 void setOnResult( void (*pMethod)(HTTPResult) ); 00090 class CDummy; 00091 template<class T> 00092 //Linker bug : Must be defined here :( 00093 void setOnResult( T* pItem, void (T::*pMethod)(HTTPResult) ) 00094 { 00095 m_pCb = NULL; 00096 m_pCbItem = (CDummy*) pItem; 00097 m_pCbMeth = (void (CDummy::*)(HTTPResult)) pMethod; 00098 } 00099 00100 void setTimeout(int ms); 00101 00102 virtual void poll(); //Called by NetServices 00103 00104 int getHTTPResponseCode(); 00105 void setRequestHeader(const string& header, const string& value); 00106 string& getResponseHeader(const string& header); 00107 void resetRequestHeaders(); 00108 00109 protected: 00110 void resetTimeout(); 00111 00112 void init(); 00113 void close(); 00114 00115 void setup(const char* uri, HTTPData* pDataOut, HTTPData* pDataIn); //Setup request, make DNS Req if necessary 00116 void connect(); //Start Connection 00117 00118 int tryRead(); //Read data and try to feed output 00119 void readData(); //Data has been read 00120 void writeData(); //Data has been written & buf is free 00121 00122 void onTCPSocketEvent(TCPSocketEvent e); 00123 void onDNSReply(DNSReply r); 00124 void onResult(HTTPResult r); //Called when exchange completed or on failure 00125 void onTimeout(); //Connection has timed out 00126 00127 private: 00128 HTTPResult blockingProcess(); //Called in blocking mode, calls Net::poll() until return code is available 00129 00130 bool readHeaders(); //Called first when receiving data 00131 bool writeHeaders(); //Called to create req 00132 int readLine(char* str, int maxLen, bool* pIncomplete = NULL); 00133 00134 enum HTTP_METH 00135 { 00136 HTTP_GET, 00137 HTTP_POST, 00138 HTTP_HEAD 00139 }; 00140 00141 HTTP_METH m_meth; 00142 00143 CDummy* m_pCbItem; 00144 void (CDummy::*m_pCbMeth)(HTTPResult); 00145 00146 void (*m_pCb)(HTTPResult); 00147 00148 TCPSocket* m_pTCPSocket; 00149 map<string, string> m_reqHeaders; 00150 map<string, string> m_respHeaders; 00151 00152 Timer m_watchdog; 00153 int m_timeout; 00154 00155 DNSRequest* m_pDnsReq; 00156 00157 Host m_server; 00158 string m_path; 00159 00160 bool m_closed; 00161 00162 enum HTTPStep 00163 { 00164 // HTTP_INIT, 00165 HTTP_WRITE_HEADERS, 00166 HTTP_WRITE_DATA, 00167 HTTP_READ_HEADERS, 00168 HTTP_READ_DATA, 00169 HTTP_READ_DATA_INCOMPLETE, 00170 HTTP_DONE, 00171 HTTP_CLOSED 00172 }; 00173 00174 HTTPStep m_state; 00175 00176 HTTPData* m_pDataOut; 00177 HTTPData* m_pDataIn; 00178 00179 bool m_dataChunked; //Data is encoded as chunks 00180 int m_dataPos; //Position in data 00181 int m_dataLen; //Data length 00182 char* m_buf; 00183 char* m_pBufRemaining; //Remaining 00184 int m_bufRemainingLen; //Data length in m_pBufRemaining 00185 00186 int m_httpResponseCode; 00187 00188 HTTPResult m_blockingResult; //Result if blocking mode 00189 00190 }; 00191 00192 //Including data containers here for more convenience 00193 #include "data/HTTPFile.h" 00194 #include "data/HTTPStream.h" 00195 #include "data/HTTPText.h" 00196 #include "data/HTTPMap.h" 00197 00198 #endif
Generated on Tue Jul 12 2022 18:38:36 by
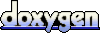