Snake game for a 5x5 LED matrix
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * 5x5 LED Snake Game 00003 * Author: Daniel Hamilton 00004 * ECE 4180 Lab 3 00005 * 00006 */ 00007 00008 #include "mbed.h" 00009 #include "ledCube.h" 00010 #include "snake.h" 00011 #include "main.h" 00012 00013 snake mySnake(snakeStartRow,snakeStartCol); // Snake represents the coordinates making up the snake 00014 food myFood(foodStartRow, foodStartCol); // food pellet the snake is trying to eat 00015 ledCube cube; // Currently a square, but represents and controls the physical LEDs 00016 AnalogIn joyVer(p19); // vertical analog joystick input pin 00017 AnalogIn joyHor(p18); // Horizontal analog joystick input pin 00018 DigitalIn select(p17); // Pushbutton on the joystick (currently unused) 00019 00020 int main() 00021 { 00022 printf("Start\n"); 00023 int snakeUpdateCounter = 0; // keeps track of when we should move Snake 00024 cube.turnOnLed(snakeStartRow, snakeStartCol, 0); // Starts the snake at Row 0 Col 0 00025 updateFoodLed(); // Lights up the food LED 00026 printf("Setup Complete\n"); 00027 bool gameover = false; // Is set to true when the game is over and keeps the LEDs blinking 00028 00029 while(1) { 00030 // Update snake position if we are greater than the set movement speed 00031 if(snakeUpdateCounter++ >= mySnake.movementSpeed) { 00032 snakeUpdateCounter = 0; 00033 if(mySnake.moveSnake(&cube) || gameover) { 00034 gameover = true; 00035 cube.blink(); 00036 } 00037 // See if the snake is on the Food's LED 00038 if(checkForSnakeEating()) { 00039 myFood.moveFood(rand() % 5, rand() % 5); 00040 updateFoodLed(); 00041 } 00042 } 00043 updateDirectionInput(); 00044 wait(.1); 00045 } 00046 00047 } 00048 00049 // Return true if the snake is on a food, false otherwise 00050 bool checkForSnakeEating() 00051 { 00052 return mySnake.isEating(myFood.currRow, myFood.currCol); 00053 } 00054 00055 // Update the food's loction 00056 void updateFoodLed() 00057 { 00058 cube.turnOnLed(myFood.currRow, myFood.currCol, 0); 00059 printf("FOOD: Row: %d Col: %d\n", myFood.currRow, myFood.currCol); 00060 if(mySnake.movementSpeed > 0){ 00061 mySnake.movementSpeed--; 00062 } 00063 } 00064 00065 // Updates the direction the snake is traveling based on the analog controller's input 00066 void updateDirectionInput(){ 00067 float verValue, horValue; 00068 int pushed; 00069 select.mode(PullUp); 00070 verValue = joyVer; 00071 horValue = joyHor; 00072 pushed = select; 00073 if(horValue < .4){ 00074 mySnake.movementDirection = Left; 00075 //printf("Left\n"); 00076 } 00077 else if(horValue > .6){ 00078 mySnake.movementDirection = Right; 00079 //printf("Right\n"); 00080 } 00081 if(verValue < .4){ 00082 mySnake.movementDirection = Down; 00083 //printf("Down\n"); 00084 } 00085 else if(verValue > .6){ 00086 mySnake.movementDirection = Up; 00087 //printf("Up\n"); 00088 } 00089 }
Generated on Wed Jul 13 2022 02:48:01 by
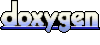