
Demo project to demonstrate that ILI9340 display driver and graphics library. very simple but a good starting point for any project using such a display. Please use this to thoroughly enjoy yourself and make your projects cool!
Dependencies: ILI9340_Driver_Lib mbed
Demo.cpp
00001 /*************************************************************** 00002 ILI9340_Driver v1.1 26.05.14 Ian Weston 00003 00004 Demo project to demonstrate the ILI9340 display driver and graphics 00005 library in action. very simple, good base for any project. 00006 00007 About the Library: 00008 00009 Driver and integrated graphics library for displays that use the 00010 ILI9340 controller in SPI mode. 00011 00012 The code was prted from several sources, the driver section 00013 was completely ported from the Adafruits Arduino source code, and 00014 the graphics functions were ported from the Adafruits GFX library 00015 and some elements were ported from code by Elmicros seeduio port. 00016 00017 Future revisions will include more advanced graphics functions. 00018 00019 Rough and ready Demo code to for showing the driver and some 00020 functions in action. 00021 00022 ***************************************************************/ 00023 00024 00025 #include "mbed.h" 00026 #include "ILI9340_Driver.h" 00027 00028 00029 int main() { 00030 00031 // create the display object 00032 ILI9340_Display tft = ILI9340_Display(p5, p6, p7, p24, p25, p26); 00033 00034 // initialise the display 00035 tft.DispInit(); 00036 00037 // clears the screen to remove all noise data 00038 tft.FillScreen(ILI9340_WHITE); 00039 00040 00041 00042 // set up variables for graphics functions 00043 uint16_t c1, c2, c3, c4, c5, c6; 00044 uint8_t r = 0, g = 0, b = 0; 00045 char elapsed[] = "1111"; 00046 int counter = 0; 00047 00048 // variables for the 'waiting..' squares 00049 int red[] = {0,30,60,90,120}; 00050 int green[] = {0,30,60,90,120}; 00051 int blue[] = {0,30,60,90,120}; 00052 00053 00054 while(true) { 00055 // draws a black window 00056 tft.DrawRect(20, 20, 200, 280, ILI9340_BLACK); 00057 00058 // Small amount of text to the display. 00059 tft.DrawString("Hello ILI9340 Lib!", 50, 120, 1, ILI9340_BLACK); 00060 tft.DrawString("Frame Count:", 70, 135, 1, ILI9340_BLACK); 00061 tft.DrawString("Go Create!", 45, 210, 2, ILI9340_BLUE); 00062 00063 // convert the RGB values into values that can be writen to the screen 00064 c1 = tft.Colour565(r, g, b); 00065 c2 = tft.Colour565(red[0], green[0], blue[0]); 00066 c3 = tft.Colour565(red[1], green[1], blue[1]); 00067 c4 = tft.Colour565(red[2], green[2], blue[2]); 00068 c5 = tft.Colour565(red[3], green[3], blue[3]); 00069 c6 = tft.Colour565(red[4], green[4], blue[4]); 00070 00071 // Print a 'waiting..' animation to the screen. 00072 tft.FillRect( 30, 60, 20, 20, c6); 00073 tft.FillRect( 70, 60, 20, 20, c5); 00074 tft.FillRect( 110, 60, 20, 20, c4); 00075 tft.FillRect( 150, 60, 20, 20, c3); 00076 tft.FillRect( 190, 60, 20, 20, c2); 00077 00078 // change the RGB vlaues for circle effect 00079 r += 4; g += 6; b += 8; 00080 00081 // change RGB values for the 'waiting' animation effect 00082 for (int i = 0; i < 5; i++) { 00083 red[i] += 5; 00084 green[i] += 5; 00085 blue[i] += 5; 00086 } 00087 00088 00089 //Write the frame count to screen, first overwriting the previos value in the background colour 00090 tft.IntToChars(elapsed, counter, 4, 10, 70, 160, 3, ILI9340_WHITE); 00091 if (counter++ > 9999) {counter = 0;} 00092 tft.IntToChars(elapsed, counter, 4, 10, 70, 160, 3, ILI9340_RED); 00093 00094 // Draw the circle ripples to the screen 00095 tft.DrawCircle(120, 265, r, c1); 00096 00097 00098 // Do the waiting thang... 00099 wait(0.025); 00100 00101 } 00102 00103 } 00104
Generated on Thu Jul 28 2022 18:50:02 by
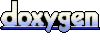