
Example program to create IoT devices for a local network, which connect to a local server.
Dependencies: WebSocketClient WiflyInterface mbed messages
globals.cpp
00001 /** 00002 * @author Damien Frost 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2016 Damien Frost 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @file "globals.cpp" 00027 * 00028 * @section DESCRIPTION 00029 * Global definitions for the IoT example program. 00030 * 00031 */ 00032 00033 #include "mbed.h" 00034 #include "globals.h " 00035 00036 //#define DEBUG 00037 #define INFOMESSAGES 00038 #define WARNMESSAGES 00039 #define ERRMESSAGES 00040 #define FUNCNAME "GBL" 00041 #include "messages.h" 00042 00043 // Hardware declarations: 00044 Serial pc(USBTX, USBRX); 00045 InterruptIn UIBut1(USER_BUTTON); 00046 Timer DisplayTimer; 00047 DigitalOut Led(D5); 00048 00049 // Global variable declarations: 00050 int ReconnectAttempts = 0; 00051 int SendCounter = 0; 00052 extern int IoT_ID = 0; 00053 float TempSensor = 0.0f; 00054 char* wifissid = "SC"; 00055 char* wifipassword = "smartcellshield"; 00056 00057 // Wifily interface declaration: 00058 WiflyInterface eth(D8, D2, D6, LED1, wifissid, wifipassword, WPA2); 00059 // WebSocket declaration: 00060 Websocket ws; 00061 00062 00063 int SetupNetwork(int Tries){ 00064 // Initialize the interface. 00065 // If no param is passed to init() then DHCP will be used on connect() 00066 int s = eth.init(); 00067 int attempts = 1; 00068 00069 wait(1); 00070 if (s != NULL) { 00071 ERR("Could not initialise. Halting!"); 00072 exit(0); 00073 } 00074 00075 INFO("Connecting to: %s", wifissid); 00076 DBG("Getting IP address..."); 00077 00078 while (1) { 00079 // Connect to network: 00080 s = eth.connect(); 00081 // If connection fails, retry for 5 attempts: 00082 if (s == false || s < 0) { 00083 INFO("Could not connect to network. Retrying!"); 00084 attempts++; 00085 wait(1); 00086 } else { 00087 00088 break; 00089 } 00090 if(attempts > Tries){ 00091 ERR("Network connection failed after %d attempts", Tries); 00092 return 0; 00093 } 00094 } 00095 INFO("Connected to: %s", wifissid); 00096 INFO("Got IP address: %s", eth.getIPAddress()); 00097 IotStatus.SetFlag(SF_WIRELESSCONNECTED); 00098 return 1; 00099 00100 } 00101 00102 void SendNetworkData(void){ 00103 char msg_buffer[CHARMSGBUFF]; 00104 int intresult; 00105 00106 if(IotStatus.CheckFlag(SF_SERVERCONNECTED)){ 00107 sprintf(msg_buffer, "%d,%d,%.5f", IoT_ID, SendCounter,TempSensor); 00108 INFO("Sending: %s", msg_buffer); // When this line is commented out, the mbed never tries to reconnect to the server after one try. SUPER. Keeping this here also uses precious CPU time 00109 intresult = ws.send(msg_buffer); 00110 }else{ 00111 intresult = -1; 00112 } 00113 DBG("intresult: %d", intresult); 00114 00115 if(intresult < 0){ 00116 // Clear a status flag: 00117 IotStatus.ClearFlag(SF_SERVERCONNECTED); 00118 // Check to see if the wireless is still connected: 00119 DBG("Checking network status..."); 00120 if(eth.checkNetworkStatus() != 3){ 00121 IotStatus.ClearFlag(SF_WIRELESSCONNECTED); 00122 // Connect to the wireless network: 00123 if(IotStatus.CheckFlag(SF_AUTOCONNECT)){ 00124 INFO("Reconnecting to Network..."); 00125 if(SetupNetwork(1)>0){ 00126 IotStatus.SetFlag(SF_WIRELESSCONNECTED); 00127 INFO("Connected to Network."); 00128 }else{ 00129 WARN("Could not re-connect to the wireless network."); 00130 } 00131 } 00132 }else{ 00133 DBG("Network connected."); 00134 } 00135 00136 if(IotStatus.CheckFlag(SF_AUTOCONNECT) && IotStatus.CheckFlag(SF_WIRELESSCONNECTED)){ 00137 // Server connection was closed, try to reconnect: 00138 INFO("Reconnecting to Websocket Server on ws://%s:%d/ws...", SERVER_IP, WS_PORT); 00139 if(!ws.connect()){ 00140 WARN("Could not connect to the server again..."); 00141 IotStatus.ClearFlag(SF_SERVERCONNECTED); 00142 ReconnectAttempts++; 00143 if(ReconnectAttempts > 4){ 00144 INFO("Failed after %d reconnect attempts. Resetting the Wifi Shield...", ReconnectAttempts); 00145 SetupNetwork(1); 00146 ReconnectAttempts = 0; 00147 } 00148 }else{ 00149 INFO("Connected to ws://%s:%d/ws", SERVER_IP, WS_PORT); 00150 // Set a status flag: 00151 IotStatus.SetFlag(SF_SERVERCONNECTED); 00152 } 00153 } 00154 } 00155 00156 return; 00157 } 00158 00159 void ReceiveNetworkData(unsigned int * wifi_cmd, float * value){ 00160 char msg_buffer[CHARMSGBUFF]; 00161 char msg_buffer2[CHARMSGBUFF]; 00162 int resp; 00163 if(IotStatus.CheckFlag(SF_SERVERCONNECTED)){ 00164 // Check for data on the websocket: 00165 resp = ws.readmsg(msg_buffer); 00166 if(resp == 1){ 00167 INFO("Received: %s", msg_buffer); 00168 sscanf(msg_buffer, "%d,%s", wifi_cmd, msg_buffer2); 00169 if(*wifi_cmd == CV_LED_WIFI_CMD){ 00170 // Get one more value: 00171 sscanf(msg_buffer2, "%f", value); 00172 } 00173 }else if(resp == -1){ 00174 // Connection to the server is lost: 00175 IotStatus.ClearFlag(SF_SERVERCONNECTED); 00176 }else{ 00177 //DBG("Did not receive anything :(\n\r"); 00178 *wifi_cmd = NO_WIFI_CMD; 00179 *value = 0.0f; 00180 } 00181 } 00182 return; 00183 } 00184 00185 void ModifyVariable(unsigned int wifi_var, float wifi_data){ 00186 // modifies something in the SCS Controller: 00187 switch(wifi_var){ 00188 case CV_LED: 00189 if(wifi_data > 0){ 00190 Led = 1; 00191 }else{ 00192 Led = 0; 00193 } 00194 break; 00195 00196 default: 00197 break; 00198 } 00199 return; 00200 } 00201 00202 00203
Generated on Wed Jul 13 2022 16:56:12 by
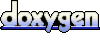