
Example program to create IoT devices for a local network, which connect to a local server.
Dependencies: WebSocketClient WiflyInterface mbed messages
Commands.h
00001 /** 00002 * @author Damien Frost 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2016 Damien Frost 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @file "Commands.h" 00027 * 00028 * @section DESCRIPTION 00029 * Commands used in the IoT example 00030 * 00031 */ 00032 00033 #ifndef IQ_COMMANDS_H 00034 #define IQ_COMMANDS_H 00035 00036 #include "StatusReg.h" 00037 #include "globals.h " 00038 00039 #define BIT0 0x1 00040 #define BIT1 0x2 00041 #define BIT2 0x4 00042 #define BIT3 0x8 00043 #define BIT4 0x10 00044 #define BIT5 0x20 00045 #define BIT6 0x40 00046 #define BIT7 0x80 00047 #define BIT8 0x100 00048 #define BIT9 0x200 00049 #define BIT10 0x400 00050 #define BIT11 0x800 00051 #define BIT12 0x1000 00052 #define BIT13 0x2000 00053 #define BIT14 0x4000 00054 #define BIT15 0x8000 00055 00056 #define BIT16 0x10000 00057 #define BIT17 0x20000 00058 #define BIT18 0x40000 00059 #define BIT19 0x80000 00060 #define BIT20 0x100000 00061 #define BIT21 0x200000 00062 #define BIT22 0x400000 00063 #define BIT23 0x800000 00064 #define BIT24 0x1000000 00065 #define BIT25 0x2000000 00066 #define BIT26 0x4000000 00067 #define BIT27 0x8000000 00068 #define BIT28 0x10000000 00069 #define BIT29 0x20000000 00070 #define BIT30 0x40000000 00071 #define BIT31 0x80000000 00072 00073 // Status regsiter flags: 00074 #define SF_SERVERCONNECTED BIT0 00075 #define SF_AUTOCONNECT BIT1 // Set this flag to automatically start connecting to the websocket server 00076 #define SF_WIRELESSCONNECTED BIT2 // Flag to indicate whether or not the wireless network is connected 00077 00078 // Wifi Commands 00079 #define NO_WIFI_CMD 0 00080 #define CV_LED_WIFI_CMD 1 00081 00082 // Change variable commands: 00083 #define CV_LED 1 00084 00085 extern StatusReg IotStatus; 00086 00087 #endif /* IQ_COMMANDS_H */
Generated on Wed Jul 13 2022 16:56:12 by
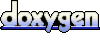