
Skeleton program for Federico's 4YP project.
Dependencies: WebSocketClient WiflyInterface mbed messages
Fork of IoT_Ex by
pwm.h
00001 // ************ 00002 // * iQ_PWM.h * 00003 // ************ 00004 // 00005 // Created: 2015/03/19 00006 // By: Damien Frost 00007 // 00008 // Description: 00009 // This file provides all of the functions used to configure the 00010 // high speed PWM module of the STM32F401RE microcontroller. 00011 00012 #ifndef PWWM_H 00013 #define PWWM_H 00014 00015 #define SETUDIS TIM1->CR1 |= TIM_CR1_UDIS 00016 #define CLEARUDIS TIM1->CR1 &= ~TIM_CR1_UDIS 00017 #define PWMHIGHPRIORITY 0 00018 #define PWMLOWPRIORITY 1 00019 00020 #define PS_RESET 0 00021 #define PS_SHIFT 1 00022 00023 #define PWMST_SETUPCONV 0 00024 #define PWMST_STARTCONV 1 00025 #define PWMST_RUNSCSKDC 2 00026 #define PWMST_CALCNEWPH 3 00027 #define PWMST_SETNEWPH 4 00028 #define PWMST_SHIFT 5 00029 #define PWMST_SAMPLECHTROUGH 6 00030 #define PWMST_WAITSAMPLETROUGH 7 00031 #define PWMST_WAIT 8 00032 #define PWMST_SAMPLECHHILL 9 00033 #define PWMST_WAITSAMPLEHILL 10 00034 #define PWMST_SKIPONECYCLE 11 00035 #define PWMST_MAXST 12 00036 00037 #define PWMSTEP_US (10.0f/839.0f) 00038 #define TS (PWMPER_US/1000000) 00039 #define PWMARRMAX (65535) 00040 00041 00042 00043 // Global variables: 00044 extern float CurrentPhaseShift; 00045 extern unsigned int OneCycleSkipExitState; 00046 extern void (*const pwmsm_StateFunction [PWMST_MAXST]) (void); 00047 00048 // Function prototypes: 00049 00050 // Configures the PWM for use, using an initial duty cycle of 'duty' 00051 void ConfigurePWM(float duty_us, float period_us); 00052 00053 // PWM ISR, used fo rdebugging purposes: 00054 void TIM1_CC_IRQHandler(void); 00055 00056 // Configures the Dead time of the PWM, using an initial dead time of 'dt': 00057 void ConfigureDeadTime(float dt_ns); 00058 00059 // Set duty by specifying the duty cycle in us: 00060 float SetDuty_us(float duty_us); 00061 00062 // Set a new duty cycle by specifying it as a number between 0 and 1: 00063 float SetDuty(float duty); 00064 00065 // This function checks to make sure the duty cycle is within the min and max constraints, it is called by the SetDuty functions. 00066 float CheckMinMaxDuty(float duty); 00067 00068 // Turn on the the PWM signal: 00069 void TurnOnPWM(bool trueForOn); 00070 00071 // Apply a phase shift: 00072 int PhaseShift(unsigned int cmd, float phase); 00073 00074 // Set a new PWM Period: 00075 void SetPWMPeriodAndDuty(int pwmper); 00076 void SetPWMPeriodAndDuty_us(float period); 00077 00078 // Set the time at which the PWM isr fires: 00079 void SetISRTime(unsigned int isrtime); 00080 00081 // PWM State Machine functions: 00082 void pwmsm_SetupConv(void); // PWMST_SETUPCONV 0 00083 void pwmsm_StartConv(void); // PWMST_STARTCONV 1 00084 void pwmsm_RunScskdc(void); // PWMST_RUNSCSKDC 2 00085 void pwmsm_CalcNewPh(void); // PWMST_CALCNEWPH 3 00086 void pwmsm_SetNewPh(void); // PWMST_SETNEWPH 4 00087 void pwmsm_Shift(void); // PWMST_SHIFT 5 00088 void pwmsm_SampleChTrough(void); // PWMST_SAMPLECHTROUGH 6 00089 void pwmsm_WaitSampleTrough(void); // PWMST_WAITSAMPLETROUGH 7 00090 void pwmsm_Wait(void); // PWMST_WAIT 8 00091 void pwmsm_SampleChHill(void); // PWMST_SAMPLECHHILL 9 00092 void pwmsm_WaitSampleHill(void); // PWMST_WAITSAMPLEHILL 10 00093 void pwmsm_SkipOneCycle(void); // PWMST_SKIPONECYCLE 11 00094 void SetupOneCycleSkip(unsigned int nextState); 00095 00096 #endif /* PWWM_H */
Generated on Tue Jul 12 2022 21:32:12 by
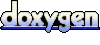