
Skeleton program for Federico's 4YP project.
Dependencies: WebSocketClient WiflyInterface mbed messages
Fork of IoT_Ex by
main.cpp
00001 /** 00002 * @author Damien Frost 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2016 Damien Frost 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @file "main.cpp" 00027 * 00028 * @section DESCRIPTION 00029 * Simple Internet of Things main program. The device sends data every 3 00030 * seconds, and can receive data from a server. 00031 * 00032 */ 00033 00034 #include "mbed.h" 00035 #include "globals.h " 00036 #include "WiflyInterface.h" 00037 #include "Commands.h " 00038 #include "Websocket.h" 00039 #include "ADC.h " 00040 #include "pwm.h" 00041 00042 //#define DEBUG 00043 #define INFOMESSAGES 00044 #define WARNMESSAGES 00045 #define ERRMESSAGES 00046 #define FUNCNAME "IoT" 00047 #include "messages.h" 00048 00049 00050 00051 // Main Loop! 00052 int main() { 00053 unsigned int wifi_cmd = NO_WIFI_CMD; 00054 float wifi_data = 0.0f; 00055 unsigned int ADCRaw; 00056 char msg[128]; 00057 00058 // Set the IoT ID: 00059 IoT_ID = 5; 00060 00061 // Set the Auto reconnect flag: 00062 IotStatus.SetFlag(SF_AUTOCONNECT); 00063 00064 // Send a startup message to serial port: 00065 INFO(""); 00066 INFO(""); 00067 INFO("Starting up..."); 00068 INFO("CPU SystemCoreClock is %d Hz", SystemCoreClock); 00069 00070 // Configure the ADC to sample the internal temperature sensor. You cannot 00071 // use AnalogIn() unfortunately... 00072 ConfigureADC(); 00073 00074 // Connect to the wifi network. It will basically get stuck here until it 00075 // connects to the network. 00076 SetupNetwork(5000); 00077 00078 // Configure the baud rate of the wifi shield: 00079 // This will make our wireless transmissions much faster. 00080 ws.setBaud(115200); 00081 wait(0.5f); 00082 00083 // Configure the PWM module: 00084 ConfigurePWM(Duty_us, PwmPeriod_us); 00085 // Turn on the PWM: 00086 TurnOnPWM(true); 00087 00088 // Check to see we are connected to the network: 00089 if(IotStatus.CheckFlag(SF_WIRELESSCONNECTED)){ 00090 // Try to connect to the WebSocket server: 00091 sprintf(msg, "ws://%s:%d/ws", SERVER_IP, WS_PORT); 00092 ws.Initialize(msg); 00093 INFO("Connecting to Websocket Server on %s...", msg); 00094 if(ws.connect()){ 00095 // Set a status flag: 00096 INFO("Connected."); 00097 IotStatus.SetFlag(SF_SERVERCONNECTED); 00098 }else{ 00099 // We could not connect right now.. 00100 IotStatus.ClearFlag(SF_SERVERCONNECTED); 00101 INFO("Could not connect to server, will try again later."); 00102 ReconnectAttempts++; 00103 } 00104 } 00105 00106 // Start the display timer which will send data to the server every 00107 // 3 seconds. 00108 DisplayTimer.start(); 00109 00110 // Inifinite main loop: 00111 while(1) { 00112 00113 // Process the wifi command: 00114 if(wifi_cmd > NO_WIFI_CMD){ 00115 // Modify the desired variable: 00116 ModifyVariable(wifi_cmd, wifi_data); 00117 // Reset the command: 00118 wifi_cmd = NO_WIFI_CMD; 00119 } 00120 00121 // Check for new wifi data: 00122 if((wifi_cmd == NO_WIFI_CMD)){ 00123 ReceiveNetworkData(&wifi_cmd, &wifi_data); 00124 } 00125 00126 // Send the network data every 3 seconds: 00127 if(DisplayTimer.read()>(3.0f)){ 00128 // Sample the internal temperature sensor: 00129 STARTADCCONVERSION; 00130 // Wait for the conversion to complete: 00131 while(!ADCCONVERSIONCOMPLETE); 00132 // Save the raw value from the ADC: 00133 ADCRaw = ADC1->DR; 00134 // Calculate the temperature using information from the datasheet: 00135 TempSensor = ((((float)ADCRaw)/ADC_MAX)*IT_VMAX - IT_V25)/IT_AVG_SLOPE + 25.0f; 00136 // Output the result: 00137 DBG("TempSensor = %.5f", TempSensor); 00138 DBG("ADC1->DR = %d", ADCRaw); 00139 00140 // Send data over network: 00141 SendNetworkData(); 00142 00143 // Increment a counter: 00144 SendCounter++; 00145 00146 // Reset the timer: 00147 DisplayTimer.reset(); 00148 00149 } 00150 } // while(1) 00151 } // main()
Generated on Tue Jul 12 2022 21:32:12 by
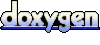