
Detects any kind of suspicious activity
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ESP8266.h" 00003 00004 InterruptIn motion(D2); 00005 00006 Serial pc(USBTX,USBRX); //UART/Serial Communication 00007 00008 ESP8266 wifi(PTE0, PTE1, 115200); // Setting up Baud Rate for WIFI ESP Module 00009 char snd[255],rcv[1000]; 00010 00011 #define IP "184.106.153.149" // Defining Thingspeak IP Address 00012 00013 00014 int dm = 0; //dm = Detected Motion 00015 00016 void motiondetection(void) 00017 { 00018 dm = 1; 00019 } 00020 00021 //Defining the necessary Functions to be used// 00022 00023 void wifi_send(void); 00024 00025 int cnt; 00026 00027 00028 //Execution of Main program 00029 00030 int main(void) 00031 { 00032 cnt = 0; //Setting counter value to zero 00033 motion.rise(&motiondetection); 00034 00035 pc.baud(9600); //Setting up Baud Rate for UART 00036 00037 // WIFI Initialization Part// 00038 00039 pc.printf("SET mode to AP\r\n"); 00040 wifi.SetMode(1); // Setting ESP to mode 1 00041 wifi.RcvReply(rcv, 1000); //In order to receive response from ESP 00042 pc.printf("%s",rcv); //Display obtained response on TeraTerm 00043 pc.printf("Conneting to AP\r\n"); 00044 00045 //wifi.Join("BHNTG1682G0382", "a2c77382"); 00046 wifi.Join("hello", "hellohello"); // Add Username and Password of the Network to which WIFI ESP module is connected 00047 wifi.RcvReply(rcv, 1000); //In order to receive response from ESP 00048 pc.printf("%s", rcv); //Display obtained response on TeraTerm 00049 wait(8); //Wait for 8 seconds 00050 00051 pc.printf("Getting IP\r\n"); // Gets IP address 00052 wifi.GetIP(rcv); //To receive IP 00053 pc.printf("%s", rcv);//Display obtained response on TeraTerm 00054 wait(5); // Delay 5 sec to give the pir time to get snapshut of the surrounding 00055 pc.printf("Initializing WiFi\r\n"); 00056 00057 while(1) { 00058 if(dm) { 00059 cnt++; 00060 dm = 0; 00061 pc.printf("ALERT!! Intrusion Detected!! I've detected %d times since reset\n", cnt); 00062 pc.printf("Now uploading status to Cloud\n\r"); 00063 wifi_send(); 00064 } 00065 } 00066 } 00067 // Main program ends// 00068 00069 00070 //Routing the inofrmation to Cloud// 00071 00072 void wifi_send(void) 00073 { 00074 pc.printf("*************Uploading WIFI Data*************\r\n"); 00075 00076 pc.printf("\r\nTo set WIFI into Single Channel mode\r\n"); 00077 00078 strcpy(snd,"AT+CIPMUX=0");//To Set WIFI into Single Channel mode 00079 wifi.SendCMD(snd); 00080 pc.printf(snd); 00081 00082 wifi.RcvReply(rcv, 1000); 00083 pc.printf("\r\nMode Status: %s", rcv); 00084 00085 00086 00087 //Connecting to THINGSPEAK server// 00088 pc.printf("\r\nConnecting to THINGSPEAK server\r\n"); 00089 strcpy(snd,"AT+CIPSTART=\"TCP\",\"api.thingspeak.com\",80"); 00090 pc.printf("\r\nSending: %s",snd); 00091 wifi.SendCMD(snd); 00092 wifi.RcvReply(rcv, 1000); 00093 pc.printf("\r\nSever Status: %s", rcv); 00094 00095 //Deliver Data/Characters// 00096 pc.printf("\r\nDelivering Data.."); 00097 strcpy(snd,"AT+CIPSEND=84"); 00098 wifi.SendCMD(snd); 00099 pc.printf("\r\nSending: %s",snd); 00100 wifi.RcvReply(rcv, 1000); 00101 pc.printf("\r\nSent Status: %s", rcv); 00102 00103 //Upload values to Cloud based website i.e. ThingSpeak in order to obtain Graphical Display// 00104 00105 pc.printf("Uploading values to Thingspeak\r\n"); 00106 sprintf(snd,"GET https://api.thingspeak.com/update?key=2DNGOOKD3OZBMVV5&field1=%d HTTP/1.0\r\n\r\n",cnt); //67,76,84 00107 //sprintf(snd,"GET https://api.thingspeak.com/update?key=2DNGOOKD3OZBMVV5&field1=%d HTTP/1.0\r\n\r\n",cnt); //67,76,84 00108 pc.printf("\r\nSending: %s",snd); 00109 wifi.SendCMD(snd); 00110 wait(1); 00111 wifi.RcvReply(rcv, 1000); 00112 pc.printf("\r\nSent Status: %s", rcv); 00113 wait(1); 00114 00115 //Closing the connection with Server// 00116 00117 pc.printf("\r\nClose the Connection\r\n"); 00118 strcpy(snd,"AT+CIPCLOSE"); //Command used to close the connection with server 00119 wifi.SendCMD(snd); 00120 pc.printf("\r\nSending: %s",snd); 00121 wait(1); 00122 wifi.RcvReply(rcv, 1000); 00123 pc.printf("Close Connection Status: %s", rcv); 00124 00125 pc.printf("\r\nClose connection\r\n"); 00126 strcpy(snd,"AT+CIPCLOSE"); 00127 wifi.SendCMD(snd); 00128 pc.printf("\r\nSending: %s",snd); 00129 wifi.RcvReply(rcv, 1000); 00130 pc.printf("Close Connection Status: %s", rcv); 00131 pc.printf("\r\nEnd of Closed Connection Status Response\r\n\r\n"); 00132 00133 }
Generated on Tue Jul 26 2022 00:59:18 by
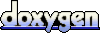