
Code for LHS 12_12_2013
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //=======================================| 00002 //%|==================================|%%| 00003 //%| |%%| 00004 //%| PLTW PROGRAM |%%| 00005 //%| ---------------------------- |%%| 00006 //%| Controls a PWM output using |%%| 00007 //%| digital inputs |%%| 00008 //%| |%%| 00009 //%| MOTOR SPEED: |%%| 00010 //%| 0.00 - 0.49 reverse |%%| 00011 //%| 0.49 - 0.51 zero |%%| 00012 //%| 0.51 - 1.00 forward |%%| 00013 //%| |%%| 00014 //%|==================================|%%| 00015 //=======================================| 00016 00017 //================================== 00018 // Add any libraries that you use: 00019 //================================== 00020 00021 #include "mbed.h" 00022 #include "Servo.h" 00023 00024 00025 //================================== 00026 // Add variables: 00027 //================================== 00028 00029 //add a motor to PWM output on pin 25 00030 Servo motor1(p25); 00031 00032 //add a variable for motor speed 00033 float speed = 0.5; 00034 00035 //add variables for the onboard LEDS, which display for each button 00036 DigitalOut led1(LED1), led2(LED2), led3(LED3), led4(LED4); 00037 00038 //add variables for button inputs on pins 15, 16, 17, and 18. 00039 InterruptIn a(p18), b(p17), c(p16), d(p15); 00040 00041 //add variable for button press //=========================================== NEW 00042 int apress = 0; //=========================================== NEW 00043 00044 //================================== 00045 // Functions for each button press 00046 //================================== 00047 00048 00049 // Functions for button 1 00050 void a_hit_interrupt (void) { 00051 00052 if(apress == 0){ // PRESSING A WILL DO NOTHING AFTER THE FIRST PRESS 00053 // UNLESS THE B BUTTON HAS BEEN PRESSED 00054 //set the apress variable to 1 // SET APRESS VARIABLE TO 1 00055 apress = 1; // SET APRESS VARIABLE TO 1 00056 00057 //move VEX motor (1 is full CCW, 0 is full CW) 00058 speed = 1.0; 00059 motor1 = speed; 00060 //set the motor on duration in seconds: 00061 wait(1); 00062 //stop motor 00063 speed = 0.5; 00064 motor1 = speed; 00065 00066 //Set LED 1 00067 led1 = 1, led2 = 0, led3 = 0, led4 = 0; 00068 } 00069 } 00070 00071 00072 00073 // Functions for button 2 00074 void b_hit_interrupt (void) { 00075 if(apress == 1){ // PRESSING B WILL DO NOTHING IF A HAS NOT PRESSED 00076 00077 //set the apress variable to 1 // RESET APRESS VARIABLE TO 0 00078 apress = 0; // RESET APRESS VARIABLE TO 0 00079 00080 //move VEX motor (1 is full CCW, 0 is full CW) 00081 speed = 0.0; 00082 motor1 = speed; 00083 //set the motor on duration in seconds: 00084 wait(1); 00085 //stop motor 00086 speed = 0.5; 00087 motor1 = speed; 00088 00089 //Set LED 2 00090 led1 = 0, led2 = 1, led3 = 0, led4 = 0; 00091 } 00092 } 00093 00094 00095 00096 // Functions for button 3 00097 void c_hit_interrupt (void) { 00098 00099 //Set LED 3 00100 led1 = 0, led2 = 0, led3 = 1, led4 = 0; 00101 } 00102 00103 00104 00105 // Functions for button 4 00106 void d_hit_interrupt (void) { 00107 00108 //Set LED 4 00109 led1 = 0, led2 = 0, led3 = 0, led4 = 1; 00110 } 00111 00112 00113 00114 //========================================================== 00115 // MAIN FUNCTION 00116 // waits for button press, then uses the button code above 00117 //========================================================== 00118 00119 int main() { 00120 00121 // Use internal pullup resistors to limit the signal current 00122 a.mode(PullUp); 00123 b.mode(PullUp); 00124 c.mode(PullUp); 00125 d.mode(PullUp); 00126 00127 // Delay for initial pullup switching to take effect 00128 wait(.01); 00129 00130 // tells the code what function should be called for each switch press 00131 a.fall(&a_hit_interrupt); 00132 b.fall(&b_hit_interrupt); 00133 c.fall(&c_hit_interrupt); 00134 d.fall(&d_hit_interrupt); 00135 00136 00137 while(1) { 00138 //reset LEDs 00139 led1 = 0, led2 = 0, led3 = 0, led4 = 0; 00140 00141 } 00142 }
Generated on Mon Aug 8 2022 07:04:55 by
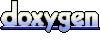