
Code for LHS 12_12_2013
Embed:
(wiki syntax)
Show/hide line numbers
code_help.cpp
00001 //%%=================================%% 00002 //%% FLOWCHART TO CODE CONVERSION %% 00003 //%%=================================%% 00004 // 00005 // DECISION BOX 00006 // -------------- 00007 // 00008 // 00009 // ____ 00010 // / A=B \__TRUE____> 00011 // \_____/ 00012 // |______FALSE 00013 // 00014 // CODE 00015 // -------- 00016 // 00017 // a equals b 00018 // 00019 // if(a == b){ 00020 // ; 00021 // ; 00022 // } 00023 // 00024 // a less than or = to b 00025 // 00026 // if(a <= b){ 00027 // ; 00028 // ; 00029 // } 00030 // 00031 //======================================= 00032 // 00033 // INPUTS 00034 // -------- 00035 // ____ 00036 // |____| 00037 // | 00038 // V 00039 // CODE 00040 // -------- 00041 // type name(pin); 00042 // 00043 // TYPES: 00044 // DigitalIn 00045 // AnalogIn 00046 // 00047 // EXAMPLES: 00048 // DigitalIn enable(p30); 00049 // AnalogIn ain(p19); 00050 // 00051 // 00052 // 00053 //========================================== 00054 // 00055 // VARIABLES 00056 // ----------- 00057 // ____ 00058 // |____| 00059 // | 00060 // V 00061 // CODE 00062 // -------- 00063 // 00064 // type variable = value; 00065 // types: 00066 // int.........integer (whole numbers, 1, 4, 128, etc) 00067 // float.......floating point decimal (0.1, 3.62783, etc) 00068 // 00069 // NOTE: value has to match data type. 00070 // see website http://mbed.org/handbook/C-Data-Types for more information 00071 // 00072 // 00073 //=========================================== 00074 // 00075 // 00076 // COUNTER LOOP EXAMPLE 00077 // ---------------------- 00078 // ____________ 00079 // | | 00080 // | MOTOR | 00081 // | SPEED = A | 00082 // |____________| 00083 // | ___________________________________ 00084 // ______V____________ | | 00085 // | | | SOME INCREMENTED ACTION, EXAMPLE: | 00086 // | IF COUNT = X THEN |<_______| MOTOR SPEED IS MOTOR SPEED + A | 00087 // | BREAK THE LOOP | |___________________________________| 00088 // |___________________| ^ 00089 // | | ___________ | 00090 // | | | | | 00091 // | |_________>| COUNT + 1 |_____________| 00092 // V |___________| 00093 // 00094 // CODE 00095 // ------ 00096 // 00097 // for(int count = 0; count <10; count = count + 1){ 00098 // ; 00099 // ; 00100 // } 00101 // 00102 // 00103 // 00104 //============================================ 00105 // 00106 // 00107 // CONTINUOUS LOOP EXAMPLE 00108 // ------------------------- 00109 // 00110 // _______________ 00111 // | | 00112 // | START OF LOOP | 00113 // |_______________| 00114 // | 00115 // |<--------------- 00116 // | | 00117 // ________V________ | 00118 // | | | 00119 // | LOGIC STATEMENT | | 00120 // |_________________| | 00121 // | | 00122 // ________V________ | 00123 // | | | 00124 // | LOGIC STATEMENT |_______| 00125 // |_________________| 00126 // 00127 // CODE 00128 // ------ 00129 // 00130 // while(1) { 00131 // ; 00132 // ; 00133 // } 00134 //
Generated on Mon Aug 8 2022 07:04:55 by
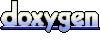