
Please run it on your NUCLEO-L152
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "Arduino.h" 00002 00003 #include <MCUFRIEND_kbv.h> 00004 MCUFRIEND_kbv tft; 00005 00006 Serial pc(SERIAL_TX, SERIAL_RX); 00007 00008 // Assign human-readable names to some common 16-bit color values: 00009 #define BLACK 0x0000 00010 #define BLUE 0x001F 00011 #define RED 0xF800 00012 #define GREEN 0x07E0 00013 #define CYAN 0x07FF 00014 #define MAGENTA 0xF81F 00015 #define YELLOW 0xFFE0 00016 #define WHITE 0xFFFF 00017 #define GRAY 0x8410 00018 00019 uint16_t version = MCUFRIEND_KBV_H_; 00020 00021 void setup() 00022 { 00023 uint16_t ID = tft.readID(); // 00024 pc.printf("\nI have run this on a NUCLEO-F072\n"); 00025 pc.printf("Please run it on your NUCLEO-L152\n"); 00026 pc.printf("Found ID = 0x%04X\n", ID); 00027 tft.begin(ID); 00028 } 00029 00030 void loop() 00031 { 00032 static uint8_t aspect = 0; 00033 const char *aspectname[] = { 00034 "PORTRAIT", "LANDSCAPE", "PORTRAIT_REV", "LANDSCAPE_REV" 00035 }; 00036 const char *colorname[] = { "BLUE", "GREEN", "RED", "GRAY" }; 00037 uint16_t colormask[] = { BLUE, GREEN, RED, GRAY }; 00038 uint16_t ID = tft.readID(); // 00039 tft.setRotation(aspect); 00040 int width = tft.width(); 00041 int height = tft.height(); 00042 tft.fillScreen(colormask[aspect]); 00043 tft.drawRect(0, 0, width, height, WHITE); 00044 tft.drawRect(32, 32, width - 64, height - 64, WHITE); 00045 tft.setTextSize(2); 00046 tft.setTextColor(BLACK); 00047 tft.setCursor(40, 40); 00048 tft.print("ID=0x"); 00049 tft.println(ID, HEX); 00050 if (ID == 0xD3D3) tft.print(" w/o"); 00051 tft.setCursor(40, 70); 00052 tft.print(aspectname[aspect]); 00053 tft.setCursor(40, 100); 00054 tft.print(width); 00055 tft.print(" x "); 00056 tft.print(height); 00057 tft.setTextColor(WHITE); 00058 tft.setCursor(40, 130); 00059 tft.print(colorname[aspect]); 00060 tft.setCursor(40, 160); 00061 tft.setTextSize(1); 00062 tft.print("MCUFRIEND_KBV_H_ = "); 00063 tft.print(version); 00064 if (++aspect > 3) aspect = 0; 00065 delay(5000); 00066 } 00067 00068 uint32_t millis(void) 00069 { 00070 static Timer t; 00071 static int first = 1; 00072 if (first) first = 0, t.start(); 00073 return t.read_ms(); 00074 } 00075 00076 uint32_t micros(void) 00077 { 00078 static Timer t; 00079 static int first = 1; 00080 if (first) first = 0, t.start(); 00081 return t.read_us(); 00082 } 00083 00084 void main(void) 00085 { 00086 setup(); 00087 while (1) { 00088 loop(); 00089 } 00090 }
Generated on Sat Jul 23 2022 01:16:42 by
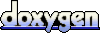