
Please run it on your NUCLEO-L152
Embed:
(wiki syntax)
Show/hide line numbers
MCUFRIEND_kbv.cpp
00001 //#define SUPPORT_0139 //S6D0139 +280 bytes 00002 #define SUPPORT_0154 //S6D0154 +320 bytes 00003 //#define SUPPORT_1289 //SSD1289,SSD1297 (ID=0x9797) +626 bytes, 0.03s 00004 //#define SUPPORT_1580 //R61580 Untested 00005 #define SUPPORT_1963 //only works with 16BIT bus anyway 00006 //#define SUPPORT_4532 //LGDP4532 +120 bytes. thanks Leodino 00007 #define SUPPORT_4535 //LGDP4535 +180 bytes 00008 #define SUPPORT_68140 //RM68140 +52 bytes defaults to PIXFMT=0x55 00009 //#define SUPPORT_7735 00010 #define SUPPORT_7781 //ST7781 +172 bytes 00011 //#define SUPPORT_8230 //UC8230 +118 bytes 00012 //#define SUPPORT_8347D //HX8347-D, HX8347-G, HX8347-I, HX8367-A +520 bytes, 0.27s 00013 //#define SUPPORT_8347A //HX8347-A +500 bytes, 0.27s 00014 //#define SUPPORT_8352A //HX8352A +486 bytes, 0.27s 00015 //#define SUPPORT_8352B //HX8352B 00016 //#define SUPPORT_8357D_GAMMA //monster 34 byte 00017 //#define SUPPORT_9163 // 00018 //#define SUPPORT_9225 //ILI9225-B, ILI9225-G ID=0x9225, ID=0x9226, ID=0x6813 +380 bytes 00019 //#define SUPPORT_9326_5420 //ILI9326, SPFD5420 +246 bytes 00020 //#define SUPPORT_9342 //costs +114 bytes 00021 //#define SUPPORT_9806 //UNTESTED 00022 #define SUPPORT_9488_555 //costs +230 bytes, 0.03s / 0.19s 00023 #define SUPPORT_B509_7793 //R61509, ST7793 +244 bytes 00024 #define OFFSET_9327 32 //costs about 103 bytes, 0.08s 00025 00026 #include "MCUFRIEND_kbv.h" 00027 #if defined(USE_SERIAL) 00028 #include "utility/mcufriend_serial.h" 00029 //uint8_t running; 00030 #elif defined(__MBED__) 00031 #include "utility/mcufriend_mbed.h" 00032 #elif defined(__CC_ARM) || defined(__CROSSWORKS_ARM) 00033 #include "utility/mcufriend_keil.h" 00034 #else 00035 #include "utility/mcufriend_shield.h" 00036 #endif 00037 00038 #define MIPI_DCS_REV1 (1<<0) 00039 #define AUTO_READINC (1<<1) 00040 #define READ_BGR (1<<2) 00041 #define READ_LOWHIGH (1<<3) 00042 #define READ_24BITS (1<<4) 00043 #define XSA_XEA_16BIT (1<<5) 00044 #define READ_NODUMMY (1<<6) 00045 #define INVERT_GS (1<<8) 00046 #define INVERT_SS (1<<9) 00047 #define MV_AXIS (1<<10) 00048 #define INVERT_RGB (1<<11) 00049 #define REV_SCREEN (1<<12) 00050 #define FLIP_VERT (1<<13) 00051 #define FLIP_HORIZ (1<<14) 00052 00053 #if (defined(USES_16BIT_BUS)) //only comes from SPECIALs 00054 #define USING_16BIT_BUS 1 00055 #else 00056 #define USING_16BIT_BUS 0 00057 #endif 00058 00059 MCUFRIEND_kbv::MCUFRIEND_kbv(int CS, int RS, int WR, int RD, int _RST):Adafruit_GFX(240, 320) 00060 { 00061 // we can not access GPIO pins until AHB has been enabled. 00062 } 00063 00064 static uint8_t done_reset, is8347, is555, is9797; 00065 static uint16_t color565_to_555(uint16_t color) { 00066 return (color & 0xFFC0) | ((color & 0x1F) << 1) | ((color & 0x01)); //lose Green LSB, extend Blue LSB 00067 } 00068 static uint16_t color555_to_565(uint16_t color) { 00069 return (color & 0xFFC0) | ((color & 0x0400) >> 5) | ((color & 0x3F) >> 1); //extend Green LSB 00070 } 00071 static uint8_t color565_to_r(uint16_t color) { 00072 return ((color & 0xF800) >> 8); // transform to rrrrrxxx 00073 } 00074 static uint8_t color565_to_g(uint16_t color) { 00075 return ((color & 0x07E0) >> 3); // transform to ggggggxx 00076 } 00077 static uint8_t color565_to_b(uint16_t color) { 00078 return ((color & 0x001F) << 3); // transform to bbbbbxxx 00079 } 00080 static void write24(uint16_t color) { 00081 uint8_t r = color565_to_r(color); 00082 uint8_t g = color565_to_g(color); 00083 uint8_t b = color565_to_b(color); 00084 write8(r); 00085 write8(g); 00086 write8(b); 00087 } 00088 00089 void MCUFRIEND_kbv::reset(void) 00090 { 00091 done_reset = 1; 00092 setWriteDir(); 00093 CTL_INIT(); 00094 CS_IDLE; 00095 RD_IDLE; 00096 WR_IDLE; 00097 RESET_IDLE; 00098 delay(50); 00099 RESET_ACTIVE; 00100 delay(100); 00101 RESET_IDLE; 00102 delay(100); 00103 WriteCmdData(0xB0, 0x0000); //R61520 needs this to read ID 00104 } 00105 00106 static void writecmddata(uint16_t cmd, uint16_t dat) 00107 { 00108 CS_ACTIVE; 00109 WriteCmd(cmd); 00110 WriteData(dat); 00111 CS_IDLE; 00112 } 00113 00114 void MCUFRIEND_kbv::WriteCmdData(uint16_t cmd, uint16_t dat) { writecmddata(cmd, dat); } 00115 00116 static void WriteCmdParamN(uint16_t cmd, int8_t N, uint8_t * block) 00117 { 00118 CS_ACTIVE; 00119 WriteCmd(cmd); 00120 while (N-- > 0) { 00121 uint8_t u8 = *block++; 00122 write8(u8); 00123 if (N && is8347) { 00124 cmd++; 00125 WriteCmd(cmd); 00126 } 00127 } 00128 CS_IDLE; 00129 } 00130 00131 static inline void WriteCmdParam4(uint8_t cmd, uint8_t d1, uint8_t d2, uint8_t d3, uint8_t d4) 00132 { 00133 uint8_t d[4]; 00134 d[0] = d1, d[1] = d2, d[2] = d3, d[3] = d4; 00135 WriteCmdParamN(cmd, 4, d); 00136 } 00137 00138 //#define WriteCmdParam4(cmd, d1, d2, d3, d4) {uint8_t d[4];d[0] = d1, d[1] = d2, d[2] = d3, d[3] = d4;WriteCmdParamN(cmd, 4, d);} 00139 void MCUFRIEND_kbv::pushCommand(uint16_t cmd, uint8_t * block, int8_t N) { WriteCmdParamN(cmd, N, block); } 00140 00141 static uint16_t read16bits(void) 00142 { 00143 uint16_t ret; 00144 uint8_t lo; 00145 #if USING_16BIT_BUS 00146 READ_16(ret); //single strobe to read whole bus 00147 if (ret > 255) //ID might say 0x00D3 00148 return ret; 00149 #else 00150 READ_8(ret); 00151 #endif 00152 //all MIPI_DCS_REV1 style params are 8-bit 00153 READ_8(lo); 00154 return (ret << 8) | lo; 00155 } 00156 00157 uint16_t MCUFRIEND_kbv::readReg(uint16_t reg, int8_t index) 00158 { 00159 uint16_t ret; 00160 uint8_t lo; 00161 if (!done_reset) 00162 reset(); 00163 CS_ACTIVE; 00164 WriteCmd(reg); 00165 setReadDir(); 00166 delay(1); //1us should be adequate 00167 // READ_16(ret); 00168 do { ret = read16bits(); }while (--index >= 0); //need to test with SSD1963 00169 RD_IDLE; 00170 CS_IDLE; 00171 setWriteDir(); 00172 return ret; 00173 } 00174 00175 uint32_t MCUFRIEND_kbv::readReg32(uint16_t reg) 00176 { 00177 uint16_t h = readReg(reg, 0); 00178 uint16_t l = readReg(reg, 1); 00179 return ((uint32_t) h << 16) | (l); 00180 } 00181 00182 uint32_t MCUFRIEND_kbv::readReg40(uint16_t reg) 00183 { 00184 uint16_t h = readReg(reg, 0); 00185 uint16_t m = readReg(reg, 1); 00186 uint16_t l = readReg(reg, 2); 00187 return ((uint32_t) h << 24) | (m << 8) | (l >> 8); 00188 } 00189 00190 uint16_t MCUFRIEND_kbv::readID(void) 00191 { 00192 uint16_t ret, ret2; 00193 uint8_t msb; 00194 ret = readReg(0); //forces a reset() if called before begin() 00195 if (ret == 0x5408) //the SPFD5408 fails the 0xD3D3 test. 00196 return 0x5408; 00197 if (ret == 0x5420) //the SPFD5420 fails the 0xD3D3 test. 00198 return 0x5420; 00199 if (ret == 0x8989) //SSD1289 is always 8989 00200 return 0x1289; 00201 ret = readReg(0x67); //HX8347-A 00202 if (ret == 0x4747) 00203 return 0x8347; 00204 //#if defined(SUPPORT_1963) && USING_16BIT_BUS 00205 ret = readReg32(0xA1); //SSD1963: [01 57 61 01] 00206 if (ret == 0x6101) 00207 return 0x1963; 00208 if (ret == 0xFFFF) //R61526: [xx FF FF FF] 00209 return 0x1526; //subsequent begin() enables Command Access 00210 // if (ret == 0xFF00) //R61520: [xx FF FF 00] 00211 // return 0x1520; //subsequent begin() enables Command Access 00212 //#endif 00213 ret = readReg40(0xBF); 00214 if (ret == 0x8357) //HX8357B: [xx 01 62 83 57 FF] 00215 return 0x8357; 00216 if (ret == 0x9481) //ILI9481: [xx 02 04 94 81 FF] 00217 return 0x9481; 00218 if (ret == 0x1511) //?R61511: [xx 02 04 15 11] not tested yet 00219 return 0x1511; 00220 if (ret == 0x1520) //?R61520: [xx 01 22 15 20] 00221 return 0x1520; 00222 if (ret == 0x1526) //?R61526: [xx 01 22 15 26] 00223 return 0x1526; 00224 if (ret == 0x1581) //R61581: [xx 01 22 15 81] 00225 return 0x1581; 00226 if (ret == 0x1400) //?RM68140:[xx FF 68 14 00] not tested yet 00227 return 0x6814; 00228 ret = readReg32(0xD4); 00229 if (ret == 0x5310) //NT35310: [xx 01 53 10] 00230 return 0x5310; 00231 ret = readReg32(0xD7); 00232 if (ret == 0x8031) //weird unknown from BangGood [xx 20 80 31] PrinceCharles 00233 return 0x8031; 00234 ret = readReg40(0xEF); //ILI9327: [xx 02 04 93 27 FF] 00235 if (ret == 0x9327) 00236 return 0x9327; 00237 ret = readReg32(0xFE) >> 8; //weird unknown from BangGood [04 20 53] 00238 if (ret == 0x2053) 00239 return 0x2053; 00240 uint32_t ret32 = readReg32(0x04); 00241 msb = ret32 >> 16; 00242 ret = ret32; 00243 // if (msb = 0x38 && ret == 0x8000) //unknown [xx 38 80 00] with D3 = 0x1602 00244 if (msb == 0x00 && ret == 0x8000) { //HX8357-D [xx 00 80 00] 00245 #if 1 00246 uint8_t cmds[] = {0xFF, 0x83, 0x57}; 00247 pushCommand(0xB9, cmds, 3); 00248 msb = readReg(0xD0); 00249 if (msb == 0x99) return 0x0099; //HX8357-D from datasheet 00250 if (msb == 0x90) //HX8357-C undocumented 00251 #endif 00252 return 0x9090; //BIG CHANGE: HX8357-D was 0x8357 00253 } 00254 // if (msb == 0xFF && ret == 0xFFFF) //R61526 [xx FF FF FF] 00255 // return 0x1526; //subsequent begin() enables Command Access 00256 if (ret == 0x1526) //R61526 [xx 06 15 26] if I have written NVM 00257 return 0x1526; //subsequent begin() enables Command Access 00258 if (ret == 0x89F0) //ST7735S: [xx 7C 89 F0] 00259 return 0x7735; 00260 if (ret == 0x8552) //ST7789V: [xx 85 85 52] 00261 return 0x7789; 00262 if (ret == 0xAC11) //?unknown [xx 61 AC 11] 00263 return 0xAC11; 00264 ret32 = readReg32(0xD3); //[xx 91 63 00] 00265 ret = ret32 >> 8; 00266 if (ret == 0x9163) return ret; 00267 ret = readReg32(0xD3); //for ILI9488, 9486, 9340, 9341 00268 msb = ret >> 8; 00269 if (msb == 0x93 || msb == 0x94 || msb == 0x98 || msb == 0x77 || msb == 0x16) 00270 return ret; //0x9488, 9486, 9340, 9341, 7796 00271 if (ret == 0x00D3 || ret == 0xD3D3) 00272 return ret; //16-bit write-only bus 00273 /* 00274 msb = 0x12; //read 3rd,4th byte. does not work in parallel 00275 pushCommand(0xD9, &msb, 1); 00276 ret2 = readReg(0xD3); 00277 msb = 0x13; 00278 pushCommand(0xD9, &msb, 1); 00279 ret = (ret2 << 8) | readReg(0xD3); 00280 // if (ret2 == 0x93) 00281 return ret2; 00282 */ 00283 return readReg(0); //0154, 7783, 9320, 9325, 9335, B505, B509 00284 } 00285 00286 // independent cursor and window registers. S6D0154, ST7781 increments. ILI92320/5 do not. 00287 int16_t MCUFRIEND_kbv::readGRAM(int16_t x, int16_t y, uint16_t * block, int16_t w, int16_t h) 00288 { 00289 uint16_t ret, dummy, _MR = _MW; 00290 int16_t n = w * h, row = 0, col = 0; 00291 uint8_t r, g, b, tmp; 00292 if (!is8347 && _lcd_capable & MIPI_DCS_REV1) // HX8347 uses same register 00293 _MR = 0x2E; 00294 if (_lcd_ID == 0x1602) _MR = 0x2E; 00295 setAddrWindow(x, y, x + w - 1, y + h - 1); 00296 while (n > 0) { 00297 if (!(_lcd_capable & MIPI_DCS_REV1)) { 00298 WriteCmdData(_MC, x + col); 00299 WriteCmdData(_MP, y + row); 00300 } 00301 CS_ACTIVE; 00302 WriteCmd(_MR); 00303 setReadDir(); 00304 if (_lcd_capable & READ_NODUMMY) { 00305 ; 00306 } else if ((_lcd_capable & MIPI_DCS_REV1) || _lcd_ID == 0x1289) { 00307 READ_8(r); 00308 } else { 00309 READ_16(dummy); 00310 } 00311 if (_lcd_ID == 0x1511) READ_8(r); //extra dummy for R61511 00312 while (n) { 00313 if (_lcd_capable & READ_24BITS) { 00314 READ_8(r); 00315 READ_8(g); 00316 READ_8(b); 00317 if (_lcd_capable & READ_BGR) 00318 ret = color565(b, g, r); 00319 else 00320 ret = color565(r, g, b); 00321 } else { 00322 READ_16(ret); 00323 if (_lcd_capable & READ_LOWHIGH) 00324 ret = (ret >> 8) | (ret << 8); 00325 if (_lcd_capable & READ_BGR) 00326 ret = (ret & 0x07E0) | (ret >> 11) | (ret << 11); 00327 } 00328 #if defined(SUPPORT_9488_555) 00329 if (is555) ret = color555_to_565(ret); 00330 #endif 00331 *block++ = ret; 00332 n--; 00333 if (!(_lcd_capable & AUTO_READINC)) 00334 break; 00335 } 00336 if (++col >= w) { 00337 col = 0; 00338 if (++row >= h) 00339 row = 0; 00340 } 00341 RD_IDLE; 00342 CS_IDLE; 00343 setWriteDir(); 00344 } 00345 if (!(_lcd_capable & MIPI_DCS_REV1)) 00346 setAddrWindow(0, 0, width() - 1, height() - 1); 00347 return 0; 00348 } 00349 00350 void MCUFRIEND_kbv::setRotation(uint8_t r) 00351 { 00352 uint16_t GS, SS_v, ORG, REV = _lcd_rev; 00353 uint8_t val, d[3]; 00354 rotation = r & 3; // just perform the operation ourselves on the protected variables 00355 _width = (rotation & 1) ? HEIGHT : WIDTH; 00356 _height = (rotation & 1) ? WIDTH : HEIGHT; 00357 switch (rotation) { 00358 case 0: //PORTRAIT: 00359 val = 0x48; //MY=0, MX=1, MV=0, ML=0, BGR=1 00360 break; 00361 case 1: //LANDSCAPE: 90 degrees 00362 val = 0x28; //MY=0, MX=0, MV=1, ML=0, BGR=1 00363 break; 00364 case 2: //PORTRAIT_REV: 180 degrees 00365 val = 0x98; //MY=1, MX=0, MV=0, ML=1, BGR=1 00366 break; 00367 case 3: //LANDSCAPE_REV: 270 degrees 00368 val = 0xF8; //MY=1, MX=1, MV=1, ML=1, BGR=1 00369 break; 00370 } 00371 if (_lcd_capable & INVERT_GS) 00372 val ^= 0x80; 00373 if (_lcd_capable & INVERT_SS) 00374 val ^= 0x40; 00375 if (_lcd_capable & INVERT_RGB) 00376 val ^= 0x08; 00377 if (_lcd_capable & MIPI_DCS_REV1) { 00378 if (_lcd_ID == 0x6814) { //.kbv my weird 0x9486 might be 68140 00379 GS = (val & 0x80) ? (1 << 6) : 0; //MY 00380 SS_v = (val & 0x40) ? (1 << 5) : 0; //MX 00381 val &= 0x28; //keep MV, BGR, MY=0, MX=0, ML=0 00382 d[0] = 0; 00383 d[1] = GS | SS_v | 0x02; //MY, MX 00384 d[2] = 0x3B; 00385 WriteCmdParamN(0xB6, 3, d); 00386 goto common_MC; 00387 } else if (_lcd_ID == 0x1963 || _lcd_ID == 0x9481 || _lcd_ID == 0x1511) { 00388 if (val & 0x80) 00389 val |= 0x01; //GS 00390 if ((val & 0x40)) 00391 val |= 0x02; //SS 00392 if (_lcd_ID == 0x1963) val &= ~0xC0; 00393 if (_lcd_ID == 0x9481) val &= ~0xD0; 00394 if (_lcd_ID == 0x1511) { 00395 val &= ~0x10; //remove ML 00396 val |= 0xC0; //force penguin 180 rotation 00397 } 00398 // val &= (_lcd_ID == 0x1963) ? ~0xC0 : ~0xD0; //MY=0, MX=0 with ML=0 for ILI9481 00399 goto common_MC; 00400 } else if (is8347) { 00401 _MC = 0x02, _MP = 0x06, _MW = 0x22, _SC = 0x02, _EC = 0x04, _SP = 0x06, _EP = 0x08; 00402 if (_lcd_ID == 0x0065) { //HX8352-B 00403 val |= 0x01; //GS=1 00404 if ((val & 0x10)) val ^= 0xD3; //(ML) flip MY, MX, ML, SS, GS 00405 if (r & 1) _MC = 0x82, _MP = 0x80; 00406 else _MC = 0x80, _MP = 0x82; 00407 } 00408 if (_lcd_ID == 0x5252) { //HX8352-A 00409 val |= 0x02; //VERT_SCROLLON 00410 if ((val & 0x10)) val ^= 0xD4; //(ML) flip MY, MX, SS. GS=1 00411 } 00412 goto common_BGR; 00413 } 00414 common_MC: 00415 _MC = 0x2A, _MP = 0x2B, _MW = 0x2C, _SC = 0x2A, _EC = 0x2A, _SP = 0x2B, _EP = 0x2B; 00416 common_BGR: 00417 WriteCmdParamN(is8347 ? 0x16 : 0x36, 1, &val); 00418 _lcd_madctl = val; 00419 // if (_lcd_ID == 0x1963) WriteCmdParamN(0x13, 0, NULL); //NORMAL mode 00420 } 00421 // cope with 9320 variants 00422 else { 00423 switch (_lcd_ID) { 00424 #if defined(SUPPORT_9225) 00425 case 0x9225: 00426 _SC = 0x37, _EC = 0x36, _SP = 0x39, _EP = 0x38; 00427 _MC = 0x20, _MP = 0x21, _MW = 0x22; 00428 GS = (val & 0x80) ? (1 << 9) : 0; 00429 SS_v = (val & 0x40) ? (1 << 8) : 0; 00430 WriteCmdData(0x01, GS | SS_v | 0x001C); // set Driver Output Control 00431 goto common_ORG; 00432 #endif 00433 #if defined(SUPPORT_0139) || defined(SUPPORT_0154) 00434 #ifdef SUPPORT_0139 00435 case 0x0139: 00436 _SC = 0x46, _EC = 0x46, _SP = 0x48, _EP = 0x47; 00437 goto common_S6D; 00438 #endif 00439 #ifdef SUPPORT_0154 00440 case 0x0154: 00441 _SC = 0x37, _EC = 0x36, _SP = 0x39, _EP = 0x38; 00442 goto common_S6D; 00443 #endif 00444 common_S6D: 00445 _MC = 0x20, _MP = 0x21, _MW = 0x22; 00446 GS = (val & 0x80) ? (1 << 9) : 0; 00447 SS_v = (val & 0x40) ? (1 << 8) : 0; 00448 // S6D0139 requires NL = 0x27, S6D0154 NL = 0x28 00449 WriteCmdData(0x01, GS | SS_v | ((_lcd_ID == 0x0139) ? 0x27 : 0x28)); 00450 goto common_ORG; 00451 #endif 00452 case 0x5420: 00453 case 0x7793: 00454 case 0x9326: 00455 case 0xB509: 00456 _MC = 0x200, _MP = 0x201, _MW = 0x202, _SC = 0x210, _EC = 0x211, _SP = 0x212, _EP = 0x213; 00457 GS = (val & 0x80) ? (1 << 15) : 0; 00458 uint16_t NL; 00459 NL = ((432 / 8) - 1) << 9; 00460 if (_lcd_ID == 0x9326 || _lcd_ID == 0x5420) NL >>= 1; 00461 WriteCmdData(0x400, GS | NL); 00462 goto common_SS; 00463 default: 00464 _MC = 0x20, _MP = 0x21, _MW = 0x22, _SC = 0x50, _EC = 0x51, _SP = 0x52, _EP = 0x53; 00465 GS = (val & 0x80) ? (1 << 15) : 0; 00466 WriteCmdData(0x60, GS | 0x2700); // Gate Scan Line (0xA700) 00467 common_SS: 00468 SS_v = (val & 0x40) ? (1 << 8) : 0; 00469 WriteCmdData(0x01, SS_v); // set Driver Output Control 00470 common_ORG: 00471 ORG = (val & 0x20) ? (1 << 3) : 0; 00472 #ifdef SUPPORT_8230 00473 if (_lcd_ID == 0x8230) { // UC8230 has strange BGR and READ_BGR behaviour 00474 if (rotation == 1 || rotation == 2) { 00475 val ^= 0x08; // change BGR bit for LANDSCAPE and PORTRAIT_REV 00476 } 00477 } 00478 #endif 00479 if (val & 0x08) 00480 ORG |= 0x1000; //BGR 00481 _lcd_madctl = ORG | 0x0030; 00482 WriteCmdData(0x03, _lcd_madctl); // set GRAM write direction and BGR=1. 00483 break; 00484 #ifdef SUPPORT_1289 00485 case 0x1289: 00486 _MC = 0x4E, _MP = 0x4F, _MW = 0x22, _SC = 0x44, _EC = 0x44, _SP = 0x45, _EP = 0x46; 00487 if (rotation & 1) 00488 val ^= 0xD0; // exchange Landscape modes 00489 GS = (val & 0x80) ? (1 << 14) : 0; //called TB (top-bottom), CAD=0 00490 SS_v = (val & 0x40) ? (1 << 9) : 0; //called RL (right-left) 00491 ORG = (val & 0x20) ? (1 << 3) : 0; //called AM 00492 _lcd_drivOut = GS | SS_v | (REV << 13) | 0x013F; //REV=0, BGR=0, MUX=319 00493 if (val & 0x08) 00494 _lcd_drivOut |= 0x0800; //BGR 00495 WriteCmdData(0x01, _lcd_drivOut); // set Driver Output Control 00496 if (is9797) WriteCmdData(0x11, ORG | 0x4C30); else // DFM=2, DEN=1, WM=1, TY=0 00497 WriteCmdData(0x11, ORG | 0x6070); // DFM=3, EN=0, TY=1 00498 break; 00499 #endif 00500 } 00501 } 00502 if ((rotation & 1) && ((_lcd_capable & MV_AXIS) == 0)) { 00503 uint16_t x; 00504 x = _MC, _MC = _MP, _MP = x; 00505 x = _SC, _SC = _SP, _SP = x; //.kbv check 0139 00506 x = _EC, _EC = _EP, _EP = x; //.kbv check 0139 00507 } 00508 setAddrWindow(0, 0, width() - 1, height() - 1); 00509 vertScroll(0, HEIGHT, 0); //reset scrolling after a rotation 00510 } 00511 00512 void MCUFRIEND_kbv::drawPixel(int16_t x, int16_t y, uint16_t color) 00513 { 00514 // MCUFRIEND just plots at edge if you try to write outside of the box: 00515 if (x < 0 || y < 0 || x >= width() || y >= height()) 00516 return; 00517 #if defined(SUPPORT_9488_555) 00518 if (is555) color = color565_to_555(color); 00519 #endif 00520 setAddrWindow(x, y, x, y); 00521 // CS_ACTIVE; WriteCmd(_MW); write16(color); CS_IDLE; //-0.01s +98B 00522 if (is9797) { CS_ACTIVE; WriteCmd(_MW); write24(color); CS_IDLE;} else 00523 WriteCmdData(_MW, color); 00524 } 00525 00526 void MCUFRIEND_kbv::setAddrWindow(int16_t x, int16_t y, int16_t x1, int16_t y1) 00527 { 00528 #if defined(OFFSET_9327) 00529 if (_lcd_ID == 0x9327) { 00530 if (rotation == 2) y += OFFSET_9327, y1 += OFFSET_9327; 00531 if (rotation == 3) x += OFFSET_9327, x1 += OFFSET_9327; 00532 } 00533 #endif 00534 #if 1 00535 if (_lcd_ID == 0x1526 && (rotation & 1)) { 00536 int16_t dx = x1 - x, dy = y1 - y; 00537 if (dy == 0) { y1++; } 00538 else if (dx == 0) { x1 += dy; y1 -= dy; } 00539 } 00540 #endif 00541 if (_lcd_capable & MIPI_DCS_REV1) { 00542 WriteCmdParam4(_SC, x >> 8, x, x1 >> 8, x1); //Start column instead of _MC 00543 WriteCmdParam4(_SP, y >> 8, y, y1 >> 8, y1); // 00544 if (is8347 && _lcd_ID == 0x0065) { //HX8352-B has separate _MC, _SC 00545 uint8_t d[2]; 00546 d[0] = x >> 8; d[1] = x; 00547 WriteCmdParamN(_MC, 2, d); //allows !MV_AXIS to work 00548 d[0] = y >> 8; d[1] = y; 00549 WriteCmdParamN(_MP, 2, d); 00550 } 00551 } else { 00552 WriteCmdData(_MC, x); 00553 WriteCmdData(_MP, y); 00554 if (!(x == x1 && y == y1)) { //only need MC,MP for drawPixel 00555 if (_lcd_capable & XSA_XEA_16BIT) { 00556 if (rotation & 1) 00557 y1 = y = (y1 << 8) | y; 00558 else 00559 x1 = x = (x1 << 8) | x; 00560 } 00561 WriteCmdData(_SC, x); 00562 WriteCmdData(_SP, y); 00563 WriteCmdData(_EC, x1); 00564 WriteCmdData(_EP, y1); 00565 } 00566 } 00567 } 00568 00569 void MCUFRIEND_kbv::fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color) 00570 { 00571 int16_t end; 00572 #if defined(SUPPORT_9488_555) 00573 if (is555) color = color565_to_555(color); 00574 #endif 00575 if (w < 0) { 00576 w = -w; 00577 x -= w; 00578 } //+ve w 00579 end = x + w; 00580 if (x < 0) 00581 x = 0; 00582 if (end > width()) 00583 end = width(); 00584 w = end - x; 00585 if (h < 0) { 00586 h = -h; 00587 y -= h; 00588 } //+ve h 00589 end = y + h; 00590 if (y < 0) 00591 y = 0; 00592 if (end > height()) 00593 end = height(); 00594 h = end - y; 00595 setAddrWindow(x, y, x + w - 1, y + h - 1); 00596 CS_ACTIVE; 00597 WriteCmd(_MW); 00598 if (h > w) { 00599 end = h; 00600 h = w; 00601 w = end; 00602 } 00603 uint8_t hi = color >> 8, lo = color & 0xFF; 00604 while (h-- > 0) { 00605 end = w; 00606 #if USING_16BIT_BUS 00607 #if defined(__MK66FX1M0__) //180MHz M4 00608 #define STROBE_16BIT {WR_ACTIVE4;WR_ACTIVE;WR_IDLE4;WR_IDLE;} //56ns 00609 #elif defined(__SAM3X8E__) //84MHz M3 00610 #define STROBE_16BIT {WR_ACTIVE4;WR_ACTIVE2;WR_IDLE4;WR_IDLE2;} //286ns ?ILI9486 00611 //#define STROBE_16BIT {WR_ACTIVE4;WR_ACTIVE;WR_IDLE4;WR_IDLE;} //238ns SSD1289 00612 //#define STROBE_16BIT {WR_ACTIVE2;WR_ACTIVE;WR_IDLE2;} //119ns RM68140 00613 #else //16MHz AVR 00614 #define STROBE_16BIT {WR_ACTIVE;WR_ACTIVE;WR_IDLE; } //375ns ?ILI9486 00615 #endif 00616 write_16(color); //we could just do the strobe 00617 lo = end & 7; 00618 hi = end >> 3; 00619 if (hi) 00620 do { 00621 STROBE_16BIT; 00622 STROBE_16BIT; 00623 STROBE_16BIT; 00624 STROBE_16BIT; 00625 STROBE_16BIT; 00626 STROBE_16BIT; 00627 STROBE_16BIT; 00628 STROBE_16BIT; 00629 } while (--hi > 0); 00630 while (lo-- > 0) { 00631 STROBE_16BIT; 00632 } 00633 #else 00634 #if defined(SUPPORT_1289) 00635 if (is9797) { 00636 uint8_t r = color565_to_r(color); 00637 uint8_t g = color565_to_g(color); 00638 uint8_t b = color565_to_b(color); 00639 do { 00640 write8(r); 00641 write8(g); 00642 write8(b); 00643 } while (--end != 0); 00644 } else 00645 #endif 00646 do { 00647 write8(hi); 00648 write8(lo); 00649 } while (--end != 0); 00650 #endif 00651 } 00652 CS_IDLE; 00653 if (!(_lcd_capable & MIPI_DCS_REV1) || ((_lcd_ID == 0x1526) && (rotation & 1))) 00654 setAddrWindow(0, 0, width() - 1, height() - 1); 00655 } 00656 00657 static void pushColors_any(uint16_t cmd, uint8_t * block, int16_t n, bool first, uint8_t flags) 00658 { 00659 uint16_t color; 00660 uint8_t h, l; 00661 bool isconst = flags & 1; 00662 bool isbigend = (flags & 2) != 0; 00663 CS_ACTIVE; 00664 if (first) { 00665 WriteCmd(cmd); 00666 } 00667 00668 if (!isconst && !isbigend) { 00669 uint16_t *block16 = (uint16_t*)block; 00670 while (n-- > 0) { 00671 color = *block16++; 00672 write16(color); 00673 } 00674 } else 00675 00676 while (n-- > 0) { 00677 if (isconst) { 00678 h = pgm_read_byte(block++); 00679 l = pgm_read_byte(block++); 00680 } else { 00681 h = (*block++); 00682 l = (*block++); 00683 } 00684 color = (isbigend) ? (h << 8 | l) : (l << 8 | h); 00685 #if defined(SUPPORT_9488_555) 00686 if (is555) color = color565_to_555(color); 00687 #endif 00688 if (is9797) write24(color); else 00689 write16(color); 00690 } 00691 CS_IDLE; 00692 } 00693 00694 void MCUFRIEND_kbv::pushColors(uint16_t * block, int16_t n, bool first) 00695 { 00696 pushColors_any(_MW, (uint8_t *)block, n, first, 0); 00697 } 00698 void MCUFRIEND_kbv::pushColors(uint8_t * block, int16_t n, bool first) 00699 { 00700 pushColors_any(_MW, (uint8_t *)block, n, first, 2); //regular bigend 00701 } 00702 void MCUFRIEND_kbv::pushColors(const uint8_t * block, int16_t n, bool first, bool bigend) 00703 { 00704 pushColors_any(_MW, (uint8_t *)block, n, first, bigend ? 3 : 1); 00705 } 00706 00707 void MCUFRIEND_kbv::vertScroll(int16_t top, int16_t scrollines, int16_t offset) 00708 { 00709 #if defined(OFFSET_9327) 00710 if (_lcd_ID == 0x9327) { 00711 if (rotation == 2 || rotation == 3) top += OFFSET_9327; 00712 } 00713 #endif 00714 int16_t bfa = HEIGHT - top - scrollines; // bottom fixed area 00715 int16_t vsp; 00716 int16_t sea = top; 00717 if (_lcd_ID == 0x9327) bfa += 32; 00718 if (offset <= -scrollines || offset >= scrollines) offset = 0; //valid scroll 00719 vsp = top + offset; // vertical start position 00720 if (offset < 0) 00721 vsp += scrollines; //keep in unsigned range 00722 sea = top + scrollines - 1; 00723 if (_lcd_capable & MIPI_DCS_REV1) { 00724 uint8_t d[6]; // for multi-byte parameters 00725 /* 00726 if (_lcd_ID == 0x9327) { //panel is wired for 240x432 00727 if (rotation == 2 || rotation == 3) { //180 or 270 degrees 00728 if (scrollines == HEIGHT) { 00729 scrollines = 432; // we get a glitch but hey-ho 00730 vsp -= 432 - HEIGHT; 00731 } 00732 if (vsp < 0) 00733 vsp += 432; 00734 } 00735 bfa = 432 - top - scrollines; 00736 } 00737 */ 00738 d[0] = top >> 8; //TFA 00739 d[1] = top; 00740 d[2] = scrollines >> 8; //VSA 00741 d[3] = scrollines; 00742 d[4] = bfa >> 8; //BFA 00743 d[5] = bfa; 00744 WriteCmdParamN(is8347 ? 0x0E : 0x33, 6, d); 00745 // if (offset == 0 && rotation > 1) vsp = top + scrollines; //make non-valid 00746 d[0] = vsp >> 8; //VSP 00747 d[1] = vsp; 00748 WriteCmdParamN(is8347 ? 0x14 : 0x37, 2, d); 00749 if (is8347) { 00750 d[0] = (offset != 0) ? (_lcd_ID == 0x8347 ? 0x02 : 0x08) : 0; 00751 WriteCmdParamN(_lcd_ID == 0x8347 ? 0x18 : 0x01, 1, d); //HX8347-D 00752 } else if (offset == 0 && (_lcd_capable & MIPI_DCS_REV1)) { 00753 WriteCmdParamN(0x13, 0, NULL); //NORMAL i.e. disable scroll 00754 } 00755 return; 00756 } 00757 // cope with 9320 style variants: 00758 switch (_lcd_ID) { 00759 case 0x7783: 00760 WriteCmdData(0x61, _lcd_rev); //!NDL, !VLE, REV 00761 WriteCmdData(0x6A, vsp); //VL# 00762 break; 00763 #ifdef SUPPORT_0139 00764 case 0x0139: 00765 WriteCmdData(0x07, 0x0213 | (_lcd_rev << 2)); //VLE1=1, GON=1, REV=x, D=3 00766 WriteCmdData(0x41, vsp); //VL# check vsp 00767 break; 00768 #endif 00769 #if defined(SUPPORT_0154) || defined(SUPPORT_9225) //thanks tongbajiel 00770 case 0x9225: 00771 case 0x0154: 00772 WriteCmdData(0x31, sea); //SEA 00773 WriteCmdData(0x32, top); //SSA 00774 WriteCmdData(0x33, vsp - top); //SST 00775 break; 00776 #endif 00777 #ifdef SUPPORT_1289 00778 case 0x1289: 00779 WriteCmdData(0x41, vsp); //VL# 00780 break; 00781 #endif 00782 case 0x5420: 00783 case 0x7793: 00784 case 0x9326: 00785 case 0xB509: 00786 WriteCmdData(0x401, (1 << 1) | _lcd_rev); //VLE, REV 00787 WriteCmdData(0x404, vsp); //VL# 00788 break; 00789 default: 00790 // 0x6809, 0x9320, 0x9325, 0x9335, 0xB505 can only scroll whole screen 00791 WriteCmdData(0x61, (1 << 1) | _lcd_rev); //!NDL, VLE, REV 00792 WriteCmdData(0x6A, vsp); //VL# 00793 break; 00794 } 00795 } 00796 00797 void MCUFRIEND_kbv::invertDisplay(boolean i) 00798 { 00799 uint8_t val; 00800 _lcd_rev = ((_lcd_capable & REV_SCREEN) != 0) ^ i; 00801 if (_lcd_capable & MIPI_DCS_REV1) { 00802 if (is8347) { 00803 // HX8347D: 0x36 Panel Characteristic. REV_Panel 00804 // HX8347A: 0x36 is Display Control 10 00805 if (_lcd_ID == 0x8347 || _lcd_ID == 0x5252) // HX8347-A, HX5352-A 00806 val = _lcd_rev ? 6 : 2; //INVON id bit#2, NORON=bit#1 00807 else val = _lcd_rev ? 8 : 10; //HX8347-D, G, I: SCROLLON=bit3, INVON=bit1 00808 // HX8347: 0x01 Display Mode has diff bit mapping for A, D 00809 WriteCmdParamN(0x01, 1, &val); 00810 } else 00811 WriteCmdParamN(_lcd_rev ? 0x21 : 0x20, 0, NULL); 00812 return; 00813 } 00814 // cope with 9320 style variants: 00815 switch (_lcd_ID) { 00816 #ifdef SUPPORT_0139 00817 case 0x0139: 00818 #endif 00819 case 0x9225: //REV is in reg(0x07) like Samsung 00820 case 0x0154: 00821 WriteCmdData(0x07, 0x13 | (_lcd_rev << 2)); //.kbv kludge 00822 break; 00823 #ifdef SUPPORT_1289 00824 case 0x1289: 00825 _lcd_drivOut &= ~(1 << 13); 00826 if (_lcd_rev) 00827 _lcd_drivOut |= (1 << 13); 00828 WriteCmdData(0x01, _lcd_drivOut); 00829 break; 00830 #endif 00831 case 0x5420: 00832 case 0x7793: 00833 case 0x9326: 00834 case 0xB509: 00835 WriteCmdData(0x401, (1 << 1) | _lcd_rev); //.kbv kludge VLE 00836 break; 00837 default: 00838 WriteCmdData(0x61, _lcd_rev); 00839 break; 00840 } 00841 } 00842 00843 #define TFTLCD_DELAY 0xFFFF 00844 #define TFTLCD_DELAY8 0x7F 00845 static void init_table(const void *table, int16_t size) 00846 { 00847 #ifdef SUPPORT_8357D_GAMMA 00848 uint8_t *p = (uint8_t *) table, dat[36]; //HX8357_99 has GAMMA[34] 00849 #else 00850 uint8_t *p = (uint8_t *) table, dat[24]; //R61526 has GAMMA[22] 00851 #endif 00852 while (size > 0) { 00853 uint8_t cmd = pgm_read_byte(p++); 00854 uint8_t len = pgm_read_byte(p++); 00855 if (cmd == TFTLCD_DELAY8) { 00856 delay(len); 00857 len = 0; 00858 } else { 00859 for (uint8_t i = 0; i < len; i++) 00860 dat[i] = pgm_read_byte(p++); 00861 WriteCmdParamN(cmd, len, dat); 00862 } 00863 size -= len + 2; 00864 } 00865 } 00866 00867 static void init_table16(const void *table, int16_t size) 00868 { 00869 uint16_t *p = (uint16_t *) table; 00870 while (size > 0) { 00871 uint16_t cmd = pgm_read_word(p++); 00872 uint16_t d = pgm_read_word(p++); 00873 if (cmd == TFTLCD_DELAY) 00874 delay(d); 00875 else { 00876 writecmddata(cmd, d); //static function 00877 } 00878 size -= 2 * sizeof(int16_t); 00879 } 00880 } 00881 00882 void MCUFRIEND_kbv::begin(uint16_t ID) 00883 { 00884 int16_t *p16; //so we can "write" to a const protected variable. 00885 const uint8_t *table8_ads = NULL; 00886 int16_t table_size; 00887 reset(); 00888 _lcd_xor = 0; 00889 switch (_lcd_ID = ID) { 00890 /* 00891 static const uint16_t _regValues[] PROGMEM = { 00892 0x0000, 0x0001, // start oscillation 00893 0x0007, 0x0000, // source output control 0 D0 00894 0x0013, 0x0000, // power control 3 off 00895 0x0011, 0x2604, // 00896 0x0014, 0x0015, // 00897 0x0010, 0x3C00, // 00898 // 0x0013, 0x0040, // 00899 // 0x0013, 0x0060, // 00900 // 0x0013, 0x0070, // 00901 0x0013, 0x0070, // power control 3 PON PON1 AON 00902 00903 0x0001, 0x0127, // driver output control 00904 // 0x0002, 0x0700, // field 0 b/c waveform xor waveform 00905 0x0003, 0x1030, // 00906 0x0007, 0x0000, // 00907 0x0008, 0x0404, // 00908 0x000B, 0x0200, // 00909 0x000C, 0x0000, // 00910 0x00015,0x0000, // 00911 00912 //gamma setting 00913 0x0030, 0x0000, 00914 0x0031, 0x0606, 00915 0x0032, 0x0006, 00916 0x0033, 0x0403, 00917 0x0034, 0x0107, 00918 0x0035, 0x0101, 00919 0x0036, 0x0707, 00920 0x0037, 0x0304, 00921 0x0038, 0x0A00, 00922 0x0039, 0x0706, 00923 00924 0x0040, 0x0000, 00925 0x0041, 0x0000, 00926 0x0042, 0x013F, 00927 0x0043, 0x0000, 00928 0x0044, 0x0000, 00929 0x0045, 0x0000, 00930 0x0046, 0xEF00, 00931 0x0047, 0x013F, 00932 0x0048, 0x0000, 00933 0x0007, 0x0011, 00934 0x0007, 0x0017, 00935 }; 00936 */ 00937 #ifdef SUPPORT_0139 00938 case 0x0139: 00939 _lcd_capable = REV_SCREEN | XSA_XEA_16BIT; //remove AUTO_READINC 00940 static const uint16_t S6D0139_regValues[] PROGMEM = { 00941 0x0000, 0x0001, //Start oscillator 00942 0x0011, 0x1a00, //Power Control 2 00943 0x0014, 0x2020, //Power Control 4 00944 0x0010, 0x0900, //Power Control 1 00945 0x0013, 0x0040, //Power Control 3 00946 0x0013, 0x0060, //Power Control 3 00947 0x0013, 0x0070, //Power Control 3 00948 0x0011, 0x1a04, //Power Control 2 00949 0x0010, 0x2f00, //Power Control 1 00950 0x0001, 0x0127, //Driver Control: SM=0, GS=0, SS=1, 240x320 00951 0x0002, 0x0100, //LCD Control: (.kbv was 0700) FLD=0, BC= 0, EOR=1 00952 0x0003, 0x1030, //Entry Mode: TR1=0, DFM=0, BGR=1, I_D=3 00953 0x0007, 0x0000, //Display Control: everything off 00954 0x0008, 0x0303, //Blank Period: FP=3, BP=3 00955 0x0009, 0x0000, //f.k. 00956 0x000b, 0x0000, //Frame Control: 00957 0x000c, 0x0000, //Interface Control: system i/f 00958 0x0040, 0x0000, //Scan Line 00959 0x0041, 0x0000, //Vertical Scroll Control 00960 0x0007, 0x0014, //Display Control: VLE1=0, SPT=0, GON=1, REV=1, D=0 (halt) 00961 0x0007, 0x0016, //Display Control: VLE1=0, SPT=0, GON=1, REV=1, D=2 (blank) 00962 0x0007, 0x0017, //Display Control: VLE1=0, SPT=0, GON=1, REV=1, D=3 (normal) 00963 // 0x0007, 0x0217, //Display Control: VLE1=1, SPT=0, GON=1, REV=1, D=3 00964 }; 00965 init_table16(S6D0139_regValues, sizeof(S6D0139_regValues)); 00966 break; 00967 #endif 00968 00969 #ifdef SUPPORT_0154 00970 case 0x0154: 00971 _lcd_capable = AUTO_READINC | REV_SCREEN; 00972 static const uint16_t S6D0154_regValues[] PROGMEM = { 00973 0x0011, 0x001A, 00974 0x0012, 0x3121, //BT=3, DC1=1, DC2=2, DC3=1 00975 0x0013, 0x006C, //GVD=108 00976 0x0014, 0x4249, //VCM=66, VML=73 00977 00978 0x0010, 0x0800, //SAP=8 00979 TFTLCD_DELAY, 10, 00980 0x0011, 0x011A, //APON=0, PON=1, AON=0, VCI1_EN=1, VC=10 00981 TFTLCD_DELAY, 10, 00982 0x0011, 0x031A, //APON=0, PON=3, AON=0, VCI1_EN=1, VC=10 00983 TFTLCD_DELAY, 10, 00984 0x0011, 0x071A, //APON=0, PON=7, AON=0, VCI1_EN=1, VC=10 00985 TFTLCD_DELAY, 10, 00986 0x0011, 0x0F1A, //APON=0, PON=15, AON=0, VCI1_EN=1, VC=10 00987 TFTLCD_DELAY, 10, 00988 0x0011, 0x0F3A, //APON=0, PON=15, AON=1, VCI1_EN=1, VC=10 00989 TFTLCD_DELAY, 30, 00990 00991 0x0001, 0x0128, 00992 0x0002, 0x0100, 00993 0x0003, 0x1030, 00994 0x0007, 0x1012, 00995 0x0008, 0x0303, 00996 0x000B, 0x1100, 00997 0x000C, 0x0000, 00998 0x000F, 0x1801, 00999 0x0015, 0x0020, 01000 01001 0x0050,0x0101, 01002 0x0051,0x0603, 01003 0x0052,0x0408, 01004 0x0053,0x0000, 01005 0x0054,0x0605, 01006 0x0055,0x0406, 01007 0x0056,0x0303, 01008 0x0057,0x0303, 01009 0x0058,0x0010, 01010 0x0059,0x1000, 01011 01012 0x0007, 0x0012, //GON=1, REV=0, D=2 01013 TFTLCD_DELAY, 40, 01014 0x0007, 0x0013, //GON=1, REV=0, D=3 01015 0x0007, 0x0017, //GON=1, REV=1, D=3 DISPLAY ON 01016 }; 01017 init_table16(S6D0154_regValues, sizeof(S6D0154_regValues)); 01018 01019 break; 01020 #endif 01021 01022 #ifdef SUPPORT_1289 01023 case 0x9797: 01024 is9797 = 1; 01025 // _lcd_capable = 0 | XSA_XEA_16BIT | REV_SCREEN | AUTO_READINC | READ_24BITS; 01026 // deliberately set READ_BGR to disable Software Scroll in graphictest_kbv example 01027 _lcd_capable = 0 | XSA_XEA_16BIT | REV_SCREEN | AUTO_READINC | READ_24BITS | READ_BGR; 01028 _lcd_ID = 0x1289; 01029 goto common_1289; 01030 case 0x1289: 01031 _lcd_capable = 0 | XSA_XEA_16BIT | REV_SCREEN | AUTO_READINC; 01032 common_1289: 01033 // came from MikroElektronika library http://www.hmsprojects.com/tft_lcd.html 01034 static const uint16_t SSD1289_regValues[] PROGMEM = { 01035 0x0000, 0x0001, 01036 0x0003, 0xA8A4, 01037 0x000C, 0x0000, 01038 0x000D, 0x000A, // VRH=10 01039 0x000E, 0x2B00, 01040 0x001E, 0x00B7, 01041 0x0001, 0x2B3F, // setRotation() alters 01042 0x0002, 0x0600, // B_C=1, EOR=1 01043 0x0010, 0x0000, 01044 0x0011, 0x6070, // setRotation() alters 01045 0x0005, 0x0000, 01046 0x0006, 0x0000, 01047 0x0016, 0xEF1C, 01048 0x0017, 0x0003, 01049 0x0007, 0x0233, 01050 0x000B, 0x0000, 01051 0x000F, 0x0000, 01052 0x0030, 0x0707, 01053 0x0031, 0x0204, 01054 0x0032, 0x0204, 01055 0x0033, 0x0502, 01056 0x0034, 0x0507, 01057 0x0035, 0x0204, 01058 0x0036, 0x0204, 01059 0x0037, 0x0502, 01060 0x003A, 0x0302, 01061 0x003B, 0x0302, 01062 0x0023, 0x0000, 01063 0x0024, 0x0000, 01064 0x0025, 0x8000, 01065 }; 01066 init_table16(SSD1289_regValues, sizeof(SSD1289_regValues)); 01067 break; 01068 #endif 01069 01070 case 0x1511: // Unknown from Levy 01071 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1; //extra read_8(dummy) 01072 static const uint8_t R61511_regValues[] PROGMEM = { 01073 0xB0, 1, 0x00, //Command Access Protect 01074 }; 01075 table8_ads = R61511_regValues, table_size = sizeof(R61511_regValues); 01076 p16 = (int16_t *) & HEIGHT; 01077 *p16 = 480; 01078 p16 = (int16_t *) & WIDTH; 01079 *p16 = 320; 01080 break; 01081 01082 case 0x1520: 01083 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 01084 static const uint8_t R61520_regValues[] PROGMEM = { 01085 0xB0, 1, 0x00, //Command Access Protect 01086 0xC0, 1, 0x0A, //DM=1, BGR=1 01087 }; 01088 table8_ads = R61520_regValues, table_size = sizeof(R61520_regValues); 01089 break; 01090 01091 case 0x1526: 01092 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 01093 static const uint8_t R61526_regValues[] PROGMEM = { 01094 0xB0, 1, 0x03, //Command Access 01095 0xE2, 1, 0x3F, //Command Write Access 01096 0xC0, 1, 0x22, //REV=0, BGR=1, SS=0 01097 0xE2, 1, 0x00, //Command Write Protect 01098 }; 01099 table8_ads = R61526_regValues, table_size = sizeof(R61526_regValues); 01100 break; 01101 01102 #ifdef SUPPORT_1580 01103 case 0x1580: 01104 _lcd_capable = 0 | REV_SCREEN | READ_BGR | INVERT_GS | READ_NODUMMY; //thanks vanhan123 01105 static const uint16_t R61580_regValues[] PROGMEM = { //from MCHIP Graphics Lib drvTFT001.c 01106 // Synchronization after reset 01107 TFTLCD_DELAY, 2, 01108 0x0000, 0x0000, 01109 0x0000, 0x0000, 01110 0x0000, 0x0000, 01111 0x0000, 0x0000, 01112 01113 // Setup display 01114 0x00A4, 0x0001, // CALB=1 01115 TFTLCD_DELAY, 2, 01116 0x0060, 0xA700, // Driver Output Control 01117 0x0008, 0x0808, // Display Control BP=8, FP=8 01118 0x0030, 0x0111, // y control 01119 0x0031, 0x2410, // y control 01120 0x0032, 0x0501, // y control 01121 0x0033, 0x050C, // y control 01122 0x0034, 0x2211, // y control 01123 0x0035, 0x0C05, // y control 01124 0x0036, 0x2105, // y control 01125 0x0037, 0x1004, // y control 01126 0x0038, 0x1101, // y control 01127 0x0039, 0x1122, // y control 01128 0x0090, 0x0019, // 80Hz 01129 0x0010, 0x0530, // Power Control 01130 0x0011, 0x0237, //DC1=2, DC0=3, VC=7 01131 // 0x0011, 0x17B0, //DC1=7, DC0=3, VC=0 ?b12 ?b7 vanhan123 01132 0x0012, 0x01BF, //VCMR=1, PSON=1, PON=1, VRH=15 01133 // 0x0012, 0x013A, //VCMR=1, PSON=1, PON=1, VRH=10 vanhan123 01134 0x0013, 0x1300, //VDV=19 01135 TFTLCD_DELAY, 100, 01136 01137 0x0001, 0x0100, 01138 0x0002, 0x0200, 01139 0x0003, 0x1030, 01140 0x0009, 0x0001, 01141 0x000A, 0x0008, 01142 0x000C, 0x0001, 01143 0x000D, 0xD000, 01144 0x000E, 0x0030, 01145 0x000F, 0x0000, 01146 0x0020, 0x0000, 01147 0x0021, 0x0000, 01148 0x0029, 0x0077, 01149 0x0050, 0x0000, 01150 0x0051, 0xD0EF, 01151 0x0052, 0x0000, 01152 0x0053, 0x013F, 01153 0x0061, 0x0001, 01154 0x006A, 0x0000, 01155 0x0080, 0x0000, 01156 0x0081, 0x0000, 01157 0x0082, 0x005F, 01158 0x0093, 0x0701, 01159 0x0007, 0x0100, 01160 }; 01161 static const uint16_t R61580_DEM240320C[] PROGMEM = { //from DEM 240320C TMH-PW-N 01162 0x00, 0x0000, 01163 0x00, 0x0000, 01164 TFTLCD_DELAY, 100, 01165 0x00, 0x0000, 01166 0x00, 0x0000, 01167 0x00, 0x0000, 01168 0x00, 0x0000, 01169 0xA4, 0x0001, 01170 TFTLCD_DELAY, 100, 01171 0x60, 0xA700, 01172 0x08, 0x0808, 01173 /******************************************/ 01174 //Gamma Setting: 01175 0x30, 0x0203, 01176 0x31, 0x080F, 01177 0x32, 0x0401, 01178 0x33, 0x050B, 01179 0x34, 0x3330, 01180 0x35, 0x0B05, 01181 0x36, 0x0005, 01182 0x37, 0x0F08, 01183 0x38, 0x0302, 01184 0x39, 0x3033, 01185 /******************************************/ 01186 //Power Setting: 01187 0x90, 0x0018, //80Hz 01188 0x10, 0x0530, //BT,AP 01189 0x11, 0x0237, //DC1,DC0,VC 01190 0x12, 0x01BF, 01191 0x13, 0x1000, //VCOM 01192 TFTLCD_DELAY, 200, 01193 /******************************************/ 01194 0x01, 0x0100, 01195 0x02, 0x0200, 01196 0x03, 0x1030, 01197 0x09, 0x0001, 01198 0x0A, 0x0008, 01199 0x0C, 0x0000, 01200 0x0D, 0xD000, 01201 01202 0x0E, 0x0030, 01203 0x0F, 0x0000, 01204 0x20, 0x0000, //H Start 01205 0x21, 0x0000, //V Start 01206 0x29, 0x002E, 01207 0x50, 0x0000, 01208 0x51, 0x00EF, 01209 0x52, 0x0000, 01210 0x53, 0x013F, 01211 0x61, 0x0001, 01212 0x6A, 0x0000, 01213 0x80, 0x0000, 01214 0x81, 0x0000, 01215 0x82, 0x005F, 01216 0x93, 0x0701, 01217 /******************************************/ 01218 0x07, 0x0100, 01219 TFTLCD_DELAY, 100, 01220 }; 01221 init_table16(R61580_DEM240320C, sizeof(R61580_DEM240320C)); 01222 // init_table16(R61580_regValues, sizeof(R61580_regValues)); 01223 break; 01224 #endif 01225 01226 #if defined(SUPPORT_1963) && USING_16BIT_BUS 01227 case 0x1963: 01228 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | READ_NODUMMY | INVERT_SS | INVERT_RGB; 01229 // from NHD 5.0" 8-bit 01230 static const uint8_t SSD1963_NHD_50_regValues[] PROGMEM = { 01231 (0xE0), 1, 0x01, // PLL enable 01232 TFTLCD_DELAY8, 10, 01233 (0xE0), 1, 0x03, // Lock PLL 01234 (0xB0), 7, 0x08, 0x80, 0x03, 0x1F, 0x01, 0xDF, 0x00, //LCD SPECIFICATION 01235 (0xF0), 1, 0x03, //was 00 pixel data interface 01236 // (0x3A), 1, 0x60, // SET R G B format = 6 6 6 01237 (0xE2), 3, 0x1D, 0x02, 0x54, //PLL multiplier, set PLL clock to 120M 01238 (0xE6), 3, 0x02, 0xFF, 0xFF, //PLL setting for PCLK, depends on resolution 01239 (0xB4), 8, 0x04, 0x20, 0x00, 0x58, 0x80, 0x00, 0x00, 0x00, //HSYNC 01240 (0xB6), 7, 0x02, 0x0D, 0x00, 0x20, 0x01, 0x00, 0x00, //VSYNC 01241 (0x13), 0, //Enter Normal mode 01242 (0x38), 0, //Exit Idle mode 01243 }; 01244 // from NHD 7.0" 8-bit 01245 static const uint8_t SSD1963_NHD_70_regValues[] PROGMEM = { 01246 (0xE2), 3, 0x1D, 0x02, 0x04, //PLL multiplier, set PLL clock to 120M 01247 (0xE0), 1, 0x01, // PLL enable 01248 TFTLCD_DELAY8, 10, 01249 (0xE0), 1, 0x03, // Lock PLL 01250 0x01, 0, //Soft Reset 01251 TFTLCD_DELAY8, 120, 01252 (0xB0), 7, 0x08, 0x80, 0x03, 0x1F, 0x01, 0xDF, 0x00, //LCD SPECIFICATION 01253 (0xF0), 1, 0x03, //was 00 pixel data interface 01254 // (0x3A), 1, 0x60, // SET R G B format = 6 6 6 01255 (0xE6), 3, 0x0F, 0xFF, 0xFF, //PLL setting for PCLK, depends on resolution 01256 (0xB4), 8, 0x04, 0x20, 0x00, 0x58, 0x80, 0x00, 0x00, 0x00, //HSYNC 01257 (0xB6), 7, 0x02, 0x0D, 0x00, 0x20, 0x01, 0x00, 0x00, //VSYNC 01258 (0x13), 0, //Enter Normal mode 01259 (0x38), 0, //Exit Idle mode 01260 }; 01261 // from UTFTv2.81 initlcd.h 01262 static const uint8_t SSD1963_800_regValues[] PROGMEM = { 01263 (0xE2), 3, 0x1E, 0x02, 0x54, //PLL multiplier, set PLL clock to 120M 01264 (0xE0), 1, 0x01, // PLL enable 01265 TFTLCD_DELAY8, 10, 01266 (0xE0), 1, 0x03, // 01267 TFTLCD_DELAY8, 10, 01268 0x01, 0, //Soft Reset 01269 TFTLCD_DELAY8, 100, 01270 (0xE6), 3, 0x03, 0xFF, 0xFF, //PLL setting for PCLK, depends on resolution 01271 (0xB0), 7, 0x24, 0x00, 0x03, 0x1F, 0x01, 0xDF, 0x00, //LCD SPECIFICATION 01272 // (0xB0), 7, 0x24, 0x00, 0x03, 0x1F, 0x01, 0xDF, 0x2D, //LCD SPECIFICATION 01273 (0xB4), 8, 0x03, 0xA0, 0x00, 0x2E, 0x30, 0x00, 0x0F, 0x00, //HSYNC 01274 (0xB6), 7, 0x02, 0x0D, 0x00, 0x10, 0x10, 0x00, 0x08, //VSYNC 01275 (0xBA), 1, 0x0F, //GPIO[3:0] out 1 01276 (0xB8), 2, 0x07, 0x01, //GPIO3=input, GPIO[2:0]=output 01277 (0xF0), 1, 0x03, //pixel data interface 01278 TFTLCD_DELAY8, 1, 01279 0x28, 0, //Display Off 01280 0x11, 0, //Sleep Out 01281 TFTLCD_DELAY8, 100, 01282 0x29, 0, //Display On 01283 (0xBE), 6, 0x06, 0xF0, 0x01, 0xF0, 0x00, 0x00, //set PWM for B/L 01284 (0xD0), 1, 0x0D, 01285 }; 01286 // from UTFTv2.82 initlcd.h 01287 static const uint8_t SSD1963_800NEW_regValues[] PROGMEM = { 01288 (0xE2), 3, 0x1E, 0x02, 0x54, //PLL multiplier, set PLL clock to 120M 01289 (0xE0), 1, 0x01, // PLL enable 01290 TFTLCD_DELAY8, 10, 01291 (0xE0), 1, 0x03, // 01292 TFTLCD_DELAY8, 10, 01293 0x01, 0, //Soft Reset 01294 TFTLCD_DELAY8, 100, 01295 (0xE6), 3, 0x03, 0xFF, 0xFF, //PLL setting for PCLK, depends on resolution 01296 (0xB0), 7, 0x24, 0x00, 0x03, 0x1F, 0x01, 0xDF, 0x00, //LCD SPECIFICATION 01297 (0xB4), 8, 0x03, 0xA0, 0x00, 0x2E, 0x30, 0x00, 0x0F, 0x00, //HSYNC HT=928, HPS=46, HPW=48, LPS=15 01298 (0xB6), 7, 0x02, 0x0D, 0x00, 0x10, 0x10, 0x00, 0x08, //VSYNC VT=525, VPS=16, VPW=16, FPS=8 01299 (0xBA), 1, 0x0F, //GPIO[3:0] out 1 01300 (0xB8), 2, 0x07, 0x01, //GPIO3=input, GPIO[2:0]=output 01301 (0xF0), 1, 0x03, //pixel data interface 01302 TFTLCD_DELAY8, 1, 01303 0x28, 0, //Display Off 01304 0x11, 0, //Sleep Out 01305 TFTLCD_DELAY8, 100, 01306 0x29, 0, //Display On 01307 (0xBE), 6, 0x06, 0xF0, 0x01, 0xF0, 0x00, 0x00, //set PWM for B/L 01308 (0xD0), 1, 0x0D, 01309 }; 01310 // from UTFTv2.82 initlcd.h 01311 static const uint8_t SSD1963_800ALT_regValues[] PROGMEM = { 01312 (0xE2), 3, 0x23, 0x02, 0x04, //PLL multiplier, set PLL clock to 120M 01313 (0xE0), 1, 0x01, // PLL enable 01314 TFTLCD_DELAY8, 10, 01315 (0xE0), 1, 0x03, // 01316 TFTLCD_DELAY8, 10, 01317 0x01, 0, //Soft Reset 01318 TFTLCD_DELAY8, 100, 01319 (0xE6), 3, 0x04, 0x93, 0xE0, //PLL setting for PCLK, depends on resolution 01320 (0xB0), 7, 0x00, 0x00, 0x03, 0x1F, 0x01, 0xDF, 0x00, //LCD SPECIFICATION 01321 (0xB4), 8, 0x03, 0xA0, 0x00, 0x2E, 0x30, 0x00, 0x0F, 0x00, //HSYNC HT=928, HPS=46, HPW=48, LPS=15 01322 (0xB6), 7, 0x02, 0x0D, 0x00, 0x10, 0x10, 0x00, 0x08, //VSYNC VT=525, VPS=16, VPW=16, FPS=8 01323 (0xBA), 1, 0x0F, //GPIO[3:0] out 1 01324 (0xB8), 2, 0x07, 0x01, //GPIO3=input, GPIO[2:0]=output 01325 (0xF0), 1, 0x03, //pixel data interface 01326 TFTLCD_DELAY8, 1, 01327 0x28, 0, //Display Off 01328 0x11, 0, //Sleep Out 01329 TFTLCD_DELAY8, 100, 01330 0x29, 0, //Display On 01331 (0xBE), 6, 0x06, 0xF0, 0x01, 0xF0, 0x00, 0x00, //set PWM for B/L 01332 (0xD0), 1, 0x0D, 01333 }; 01334 // from UTFTv2.82 initlcd.h 01335 static const uint8_t SSD1963_480_regValues[] PROGMEM = { 01336 (0xE2), 3, 0x23, 0x02, 0x54, //PLL multiplier, set PLL clock to 120M 01337 (0xE0), 1, 0x01, // PLL enable 01338 TFTLCD_DELAY8, 10, 01339 (0xE0), 1, 0x03, // 01340 TFTLCD_DELAY8, 10, 01341 0x01, 0, //Soft Reset 01342 TFTLCD_DELAY8, 100, 01343 (0xE6), 3, 0x01, 0x1F, 0xFF, //PLL setting for PCLK, depends on resolution 01344 (0xB0), 7, 0x20, 0x00, 0x01, 0xDF, 0x01, 0x0F, 0x00, //LCD SPECIFICATION 01345 (0xB4), 8, 0x02, 0x13, 0x00, 0x08, 0x2B, 0x00, 0x02, 0x00, //HSYNC 01346 (0xB6), 7, 0x01, 0x20, 0x00, 0x04, 0x0C, 0x00, 0x02, //VSYNC 01347 (0xBA), 1, 0x0F, //GPIO[3:0] out 1 01348 (0xB8), 2, 0x07, 0x01, //GPIO3=input, GPIO[2:0]=output 01349 (0xF0), 1, 0x03, //pixel data interface 01350 TFTLCD_DELAY8, 1, 01351 0x28, 0, //Display Off 01352 0x11, 0, //Sleep Out 01353 TFTLCD_DELAY8, 100, 01354 0x29, 0, //Display On 01355 (0xBE), 6, 0x06, 0xF0, 0x01, 0xF0, 0x00, 0x00, //set PWM for B/L 01356 (0xD0), 1, 0x0D, 01357 }; 01358 // table8_ads = SSD1963_480_regValues, table_size = sizeof(SSD1963_480_regValues); 01359 table8_ads = SSD1963_800_regValues, table_size = sizeof(SSD1963_800_regValues); 01360 // table8_ads = SSD1963_NHD_50_regValues, table_size = sizeof(SSD1963_NHD_50_regValues); 01361 // table8_ads = SSD1963_NHD_70_regValues, table_size = sizeof(SSD1963_NHD_70_regValues); 01362 // table8_ads = SSD1963_800NEW_regValues, table_size = sizeof(SSD1963_800NEW_regValues); 01363 // table8_ads = SSD1963_800ALT_regValues, table_size = sizeof(SSD1963_800ALT_regValues); 01364 p16 = (int16_t *) & HEIGHT; 01365 *p16 = 480; 01366 p16 = (int16_t *) & WIDTH; 01367 *p16 = 800; 01368 break; 01369 #endif 01370 01371 #ifdef SUPPORT_4532 01372 //Support for LG Electronics LGDP4532 (also 4531 i guess) by Leodino v1.0 2-Nov-2016 01373 //based on data by waveshare and the datasheet of LG Electronics 01374 //My approach to get it working: the parameters by waveshare did no make it function allright 01375 //I started with remming lines to see if this helped. Basically the stuff in range 41-93 01376 //gives problems. 01377 //The other lines that are REMmed give no problems, but it seems default values are OK as well. 01378 case 0x4532: // thanks Leodino 01379 _lcd_capable = 0 | REV_SCREEN; // | INVERT_GS; 01380 static const uint16_t LGDP4532_regValues[] PROGMEM = { 01381 0x0000,0x0001, //Device code read 01382 0x0010,0x0628, //Power control 1 SAP[2:0] BT[3:0] AP[2:0] DK DSTB SLP 01383 0x0012,0x0006, //Power control 3 PON VRH[3:0] 01384 //0x0013,0x0A32, //Power control 4 VCOMG VDV[4:0] VCM[6:0] 01385 0x0011,0x0040, //Power control 2; DC1[2:0] DC0[2:0] VC[2:0] 01386 //0x0015,0x0050, //Regulator control RSET RI[2:0] RV[2:0] RCONT[2:0] 01387 0x0012,0x0016, //Power control 3 PON VRH[3:0] 01388 TFTLCD_DELAY,50, 01389 0x0010,0x5660, //Power control 1 SAP[2:0] BT[3:0] AP[2:0] DK DSTB SLP 01390 TFTLCD_DELAY,50, 01391 //0x0013,0x2A4E, //Power control 4 VCOMG VDV[4:0] VCM[6:0] 01392 //0x0001,0x0100, //Driver output control SM SS 01393 //0x0002,0x0300, //LCD Driving Wave Control 01394 //0x0003,0x1030, //Entry mode TRI DFM BGR ORG I/D[1:0] AM 01395 //0x0007,0x0202, //Display Control 1 PTDE[1:0] BASEE GON DTE COL D[1:0] 01396 TFTLCD_DELAY,50, 01397 //0x0008,0x0202, //Display Control 2 FP[3:0] BP[3:0] front and back porch (blank period at begin and end..) 01398 //0x000A,0x0000, //Test Register 1 (RA0h) 01399 //Gamma adjustment 01400 0x0030,0x0000, 01401 0x0031,0x0402, 01402 0x0032,0x0106, 01403 0x0033,0x0700, 01404 0x0034,0x0104, 01405 0x0035,0x0301, 01406 0x0036,0x0707, 01407 0x0037,0x0305, 01408 0x0038,0x0208, 01409 0x0039,0x0F0B, 01410 TFTLCD_DELAY,50, 01411 //some of this stuff in range 41-93 really throws things off.... 01412 //0x0041,0x0002, 01413 //0x0060,0x2700, //Driver Output Control (R60h) 01414 //0x0061,0x0001, //Base Image Display Control (R61h) 01415 //0x0090,0x0119, //Panel Interface Control 1 (R90h) DIVI[1:0] RTNI[4:0] 01416 //0x0092,0x010A, //Panel Interface Control 2 (R92h) NOWI[2:0] EQI2[1:0] EQI1[1:0] 01417 //0x0093,0x0004, //Panel Interface Control 3 (R93h) MCPI[2:0] 01418 //0x00A0,0x0100, //Test Register 1 (RA0h) 01419 TFTLCD_DELAY,50, 01420 0x0007,0x0133, //Display Control 1 PTDE[1:0] BASEE GON DTE COL D[1:0] 01421 TFTLCD_DELAY,50, 01422 //0x00A0,0x0000, //Test Register 1 (RA0h) 01423 }; 01424 init_table16(LGDP4532_regValues, sizeof(LGDP4532_regValues)); 01425 break; 01426 #endif 01427 01428 #ifdef SUPPORT_4535 01429 case 0x4535: 01430 _lcd_capable = 0 | REV_SCREEN; // | INVERT_GS; 01431 static const uint16_t LGDP4535_regValues[] PROGMEM = { 01432 0x0015, 0x0030, // Set the internal vcore voltage 01433 0x009A, 0x0010, // Start internal OSC 01434 0x0011, 0x0020, // set SS and SM bit 01435 0x0010, 0x3428, // set 1 line inversion 01436 0x0012, 0x0002, // set GRAM write direction and BGR=1 01437 0x0013, 0x1038, // Resize register 01438 TFTLCD_DELAY, 40, 01439 0x0012, 0x0012, // set the back porch and front porch 01440 TFTLCD_DELAY, 40, 01441 0x0010, 0x3420, // set non-display area refresh cycle ISC[3:0] 01442 0x0013, 0x3045, // FMARK function 01443 TFTLCD_DELAY, 70, 01444 0x0030, 0x0000, // RGB interface setting 01445 0x0031, 0x0402, // Frame marker Position 01446 0x0032, 0x0307, // RGB interface polarity 01447 0x0033, 0x0304, // SAP, BT[3:0], AP, DSTB, SLP, STB 01448 0x0034, 0x0004, // DC1[2:0], DC0[2:0], VC[2:0] 01449 0x0035, 0x0401, // VREG1OUT voltage 01450 0x0036, 0x0707, // VDV[4:0] for VCOM amplitude 01451 0x0037, 0x0305, // SAP, BT[3:0], AP, DSTB, SLP, STB 01452 0x0038, 0x0610, // DC1[2:0], DC0[2:0], VC[2:0] 01453 0x0039, 0x0610, // VREG1OUT voltage 01454 0x0001, 0x0100, // VDV[4:0] for VCOM amplitude 01455 0x0002, 0x0300, // VCM[4:0] for VCOMH 01456 0x0003, 0x1030, // GRAM horizontal Address 01457 0x0008, 0x0808, // GRAM Vertical Address 01458 0x000A, 0x0008, 01459 0x0060, 0x2700, // Gate Scan Line 01460 0x0061, 0x0001, // NDL,VLE, REV 01461 0x0090, 0x013E, 01462 0x0092, 0x0100, 01463 0x0093, 0x0100, 01464 0x00A0, 0x3000, 01465 0x00A3, 0x0010, 01466 0x0007, 0x0001, 01467 0x0007, 0x0021, 01468 0x0007, 0x0023, 01469 0x0007, 0x0033, 01470 0x0007, 0x0133, 01471 }; 01472 init_table16(LGDP4535_regValues, sizeof(LGDP4535_regValues)); 01473 break; 01474 #endif 01475 01476 case 0x5310: 01477 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | INVERT_SS | INVERT_RGB | READ_24BITS; 01478 static const uint8_t NT35310_regValues[] PROGMEM = { // 01479 TFTLCD_DELAY8, 10, //just some dummy 01480 }; 01481 table8_ads = NT35310_regValues, table_size = sizeof(NT35310_regValues); 01482 p16 = (int16_t *) & HEIGHT; 01483 *p16 = 480; 01484 p16 = (int16_t *) & WIDTH; 01485 *p16 = 320; 01486 break; 01487 01488 #ifdef SUPPORT_68140 01489 case 0x6814: 01490 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; 01491 static const uint8_t RM68140_regValues_max[] PROGMEM = { // 01492 0x3A, 1, 0x55, //Pixel format .kbv my Mega Shield 01493 }; 01494 table8_ads = RM68140_regValues_max, table_size = sizeof(RM68140_regValues_max); 01495 p16 = (int16_t *) & HEIGHT; 01496 *p16 = 480; 01497 p16 = (int16_t *) & WIDTH; 01498 *p16 = 320; 01499 break; 01500 #endif 01501 01502 #ifdef SUPPORT_7735 01503 case 0x7735: // 01504 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | REV_SCREEN | READ_24BITS; 01505 static const uint8_t PROGMEM table7735S[] = { 01506 // (COMMAND_BYTE), n, data_bytes.... 01507 0xB1, 3, 0x01, 0x2C, 0x2D, // [05 3C 3C] FRMCTR1 if GM==11 01508 0xB2, 3, 0x01, 0x2C, 0x2D, // [05 3C 3C] 01509 0xB3, 6, 0x01, 0x2C, 0x2D, 0x01, 0x2C, 0x2D, // [05 3C 3C 05 3C 3C] 01510 0xB4, 1, 0x07, // [07] INVCTR Column inversion 01511 //ST7735XR Power Sequence 01512 0xC0, 3, 0xA2, 0x02, 0x84, // [A8 08 84] PWCTR1 01513 0xC1, 1, 0xC5, // [C0] 01514 0xC2, 2, 0x0A, 0x00, // [0A 00] 01515 0xC3, 2, 0x8A, 0x2A, // [8A 26] 01516 0xC4, 2, 0x8A, 0xEE, // [8A EE] 01517 0xC5, 1, 0x0E, // [05] VMCTR1 VCOM 01518 }; 01519 table8_ads = table7735S, table_size = sizeof(table7735S); // 01520 p16 = (int16_t *) & HEIGHT; 01521 *p16 = 160; 01522 p16 = (int16_t *) & WIDTH; 01523 *p16 = 128; 01524 break; 01525 #endif 01526 01527 #ifdef SUPPORT_7781 01528 case 0x7783: 01529 _lcd_capable = AUTO_READINC | REV_SCREEN | INVERT_GS; 01530 static const uint16_t ST7781_regValues[] PROGMEM = { 01531 0x00FF, 0x0001, //can we do 0xFF 01532 0x00F3, 0x0008, 01533 // LCD_Write_COM(0x00F3, 01534 01535 0x00, 0x0001, 01536 0x0001, 0x0100, // Driver Output Control Register (R01h) 01537 0x0002, 0x0700, // LCD Driving Waveform Control (R02h) 01538 0x0003, 0x1030, // Entry Mode (R03h) 01539 0x0008, 0x0302, 01540 0x0009, 0x0000, 01541 0x0010, 0x0000, // Power Control 1 (R10h) 01542 0x0011, 0x0007, // Power Control 2 (R11h) 01543 0x0012, 0x0000, // Power Control 3 (R12h) 01544 0x0013, 0x0000, // Power Control 4 (R13h) 01545 TFTLCD_DELAY, 50, 01546 0x0010, 0x14B0, // Power Control 1 SAP=1, BT=4, APE=1, AP=3 01547 TFTLCD_DELAY, 10, 01548 0x0011, 0x0007, // Power Control 2 VC=7 01549 TFTLCD_DELAY, 10, 01550 0x0012, 0x008E, // Power Control 3 VCIRE=1, VRH=14 01551 0x0013, 0x0C00, // Power Control 4 VDV=12 01552 0x0029, 0x0015, // NVM read data 2 VCM=21 01553 TFTLCD_DELAY, 10, 01554 0x0030, 0x0000, // Gamma Control 1 01555 0x0031, 0x0107, // Gamma Control 2 01556 0x0032, 0x0000, // Gamma Control 3 01557 0x0035, 0x0203, // Gamma Control 6 01558 0x0036, 0x0402, // Gamma Control 7 01559 0x0037, 0x0000, // Gamma Control 8 01560 0x0038, 0x0207, // Gamma Control 9 01561 0x0039, 0x0000, // Gamma Control 10 01562 0x003C, 0x0203, // Gamma Control 13 01563 0x003D, 0x0403, // Gamma Control 14 01564 0x0060, 0xA700, // Driver Output Control (R60h) .kbv was 0xa700 01565 0x0061, 0x0001, // Driver Output Control (R61h) 01566 0x0090, 0X0029, // Panel Interface Control 1 (R90h) 01567 01568 // Display On 01569 0x0007, 0x0133, // Display Control (R07h) 01570 TFTLCD_DELAY, 50, 01571 }; 01572 init_table16(ST7781_regValues, sizeof(ST7781_regValues)); 01573 break; 01574 #endif 01575 01576 case 0x7789: 01577 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 01578 static const uint8_t ST7789_regValues[] PROGMEM = { 01579 (0xB2), 5, 0x0C, 0x0C, 0x00, 0x33, 0x33, //PORCTRK: Porch setting [08 08 00 22 22] PSEN=0 anyway 01580 (0xB7), 1, 0x35, //GCTRL: Gate Control [35] 01581 (0xBB), 1, 0x2B, //VCOMS: VCOM setting VCOM=1.175 [20] VCOM=0.9 01582 (0xC0), 1, 0x04, //LCMCTRL: LCM Control [2C] 01583 (0xC2), 2, 0x01, 0xFF, //VDVVRHEN: VDV and VRH Command Enable [01 FF] 01584 (0xC3), 1, 0x11, //VRHS: VRH Set VAP=4.4, VAN=-4.4 [0B] 01585 (0xC4), 1, 0x20, //VDVS: VDV Set [20] 01586 (0xC6), 1, 0x0F, //FRCTRL2: Frame Rate control in normal mode [0F] 01587 (0xD0), 2, 0xA4, 0xA1, //PWCTRL1: Power Control 1 [A4 A1] 01588 (0xE0), 14, 0xD0, 0x00, 0x05, 0x0E, 0x15, 0x0D, 0x37, 0x43, 0x47, 0x09, 0x15, 0x12, 0x16, 0x19, //PVGAMCTRL: Positive Voltage Gamma control 01589 (0xE1), 14, 0xD0, 0x00, 0x05, 0x0D, 0x0C, 0x06, 0x2D, 0x44, 0x40, 0x0E, 0x1C, 0x18, 0x16, 0x19, //NVGAMCTRL: Negative Voltage Gamma control 01590 }; 01591 static const uint8_t ST7789_regValues_arcain6[] PROGMEM = { 01592 (0xB2), 5, 0x0C, 0x0C, 0x00, 0x33, 0x33, //PORCTRK: Porch setting [08 08 00 22 22] PSEN=0 anyway 01593 (0xB7), 1, 0x35, //GCTRL: Gate Control [35] 01594 (0xBB), 1, 0x35, //VCOMS: VCOM setting VCOM=??? [20] VCOM=0.9 01595 (0xC0), 1, 0x2C, //LCMCTRL: LCM Control [2C] 01596 (0xC2), 2, 0x01, 0xFF, //VDVVRHEN: VDV and VRH Command Enable [01 FF] 01597 (0xC3), 1, 0x13, //VRHS: VRH Set VAP=???, VAN=-??? [0B] 01598 (0xC4), 1, 0x20, //VDVS: VDV Set [20] 01599 (0xC6), 1, 0x0F, //FRCTRL2: Frame Rate control in normal mode [0F] 01600 (0xCA), 1, 0x0F, //REGSEL2 [0F] 01601 (0xC8), 1, 0x08, //REGSEL1 [08] 01602 (0x55), 1, 0x90, //WRCACE [00] 01603 (0xD0), 2, 0xA4, 0xA1, //PWCTRL1: Power Control 1 [A4 A1] 01604 (0xE0), 14, 0xD0, 0x00, 0x06, 0x09, 0x0B, 0x2A, 0x3C, 0x55, 0x4B, 0x08, 0x16, 0x14, 0x19, 0x20, //PVGAMCTRL: Positive Voltage Gamma control 01605 (0xE1), 14, 0xD0, 0x00, 0x06, 0x09, 0x0B, 0x29, 0x36, 0x54, 0x4B, 0x0D, 0x16, 0x14, 0x21, 0x20, //NVGAMCTRL: Negative Voltage Gamma control 01606 }; 01607 table8_ads = ST7789_regValues, table_size = sizeof(ST7789_regValues); // 01608 break; 01609 01610 case 0x8031: //Unknown BangGood thanks PrinceCharles 01611 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | REV_SCREEN; 01612 static const uint8_t FK8031_regValues[] PROGMEM = { 01613 // 0xF2:8.2 = SM, 0xF2:8.0 = REV. invertDisplay(), vertScroll() do not work 01614 0xF2,11, 0x16, 0x16, 0x03, 0x08, 0x08, 0x08, 0x08, 0x10, 0x04, 0x16, 0x16, // f.k. 0xF2:8.2 SM=1 01615 0xFD, 3, 0x11, 0x02, 0x35, //f.k 0xFD:1.1 creates contiguous scan lins 01616 }; 01617 table8_ads = FK8031_regValues, table_size = sizeof(FK8031_regValues); 01618 break; 01619 01620 #ifdef SUPPORT_8347D 01621 case 0x4747: //HX8347-D 01622 _lcd_capable = REV_SCREEN | MIPI_DCS_REV1 | MV_AXIS | INVERT_SS | AUTO_READINC | READ_24BITS; 01623 goto common_8347DGI; 01624 case 0x6767: //HX8367-A 01625 case 0x7575: //HX8347-G 01626 case 0x9595: //HX8347-I 01627 _lcd_capable = REV_SCREEN | MIPI_DCS_REV1 | MV_AXIS; 01628 common_8347DGI: 01629 is8347 = 1; 01630 static const uint8_t HX8347G_2_regValues[] PROGMEM = { 01631 0xEA, 2, 0x00, 0x20, //PTBA[15:0] 01632 0xEC, 2, 0x0C, 0xC4, //STBA[15:0] 01633 0xE8, 1, 0x38, //OPON[7:0] 01634 0xE9, 1, 0x10, //OPON1[7:0] 01635 0xF1, 1, 0x01, //OTPS1B 01636 0xF2, 1, 0x10, //GEN 01637 //Gamma 2.2 Setting 01638 0x40, 13, 0x01, 0x00, 0x00, 0x10, 0x0E, 0x24, 0x04, 0x50, 0x02, 0x13, 0x19, 0x19, 0x16, 01639 0x50, 14, 0x1B, 0x31, 0x2F, 0x3F, 0x3F, 0x3E, 0x2F, 0x7B, 0x09, 0x06, 0x06, 0x0C, 0x1D, 0xCC, 01640 //Power Voltage Setting 01641 0x1B, 1, 0x1B, //VRH=4.65V 01642 0x1A, 1, 0x01, //BT (VGH~15V,VGL~-10V,DDVDH~5V) 01643 0x24, 1, 0x2F, //VMH(VCOM High voltage ~3.2V) 01644 0x25, 1, 0x57, //VML(VCOM Low voltage -1.2V) 01645 //****VCOM offset**/// 01646 0x23, 1, 0x88, //for Flicker adjust //can reload from OTP 01647 //Power on Setting 01648 0x18, 1, 0x34, //I/P_RADJ,N/P_RADJ, Normal mode 60Hz 01649 0x19, 1, 0x01, //OSC_EN='1', start Osc 01650 0x01, 1, 0x00, //DP_STB='0', out deep sleep 01651 0x1F, 1, 0x88, // GAS=1, VOMG=00, PON=0, DK=1, XDK=0, DVDH_TRI=0, STB=0 01652 TFTLCD_DELAY8, 5, 01653 0x1F, 1, 0x80, // GAS=1, VOMG=00, PON=0, DK=0, XDK=0, DVDH_TRI=0, STB=0 01654 TFTLCD_DELAY8, 3, 01655 0x1F, 1, 0x90, // GAS=1, VOMG=00, PON=1, DK=0, XDK=0, DVDH_TRI=0, STB=0 01656 TFTLCD_DELAY8, 5, 01657 0x1F, 1, 0xD0, // GAS=1, VOMG=10, PON=1, DK=0, XDK=0, DDVDH_TRI=0, STB=0 01658 TFTLCD_DELAY8, 5, 01659 //262k/65k color selection 01660 0x17, 1, 0x05, //default 0x06 262k color // 0x05 65k color 01661 //SET PANEL 01662 0x36, 1, 0x00, //SS_P, GS_P,REV_P,BGR_P 01663 //Display ON Setting 01664 0x28, 1, 0x38, //GON=1, DTE=1, D=1000 01665 TFTLCD_DELAY8, 40, 01666 0x28, 1, 0x3F, //GON=1, DTE=1, D=1100 01667 01668 0x16, 1, 0x18, 01669 }; 01670 init_table(HX8347G_2_regValues, sizeof(HX8347G_2_regValues)); 01671 break; 01672 #endif 01673 01674 #ifdef SUPPORT_8352A 01675 case 0x5252: //HX8352-A 01676 _lcd_capable = MIPI_DCS_REV1 | MV_AXIS; 01677 is8347 = 1; 01678 static const uint8_t HX8352A_regValues[] PROGMEM = { 01679 0x83, 1, 0x02, //Test Mode: TESTM=1 01680 0x85, 1, 0x03, //VDD ctl : VDC_SEL=3 [05] 01681 0x8B, 1, 0x01, //VGS_RES 1: RES_VGS1=1 01682 0x8C, 1, 0x93, //VGS_RES 2: RES_VGS2=1, anon=0x13 [93] 01683 0x91, 1, 0x01, //PWM control: SYNC=1 01684 0x83, 1, 0x00, //Test Mode: TESTM=0 01685 //Gamma Setting 01686 0x3E, 12, 0xB0, 0x03, 0x10, 0x56, 0x13, 0x46, 0x23, 0x76, 0x00, 0x5E, 0x4F, 0x40, 01687 //Power Voltage Setting 01688 0x17, 1, 0x91, //OSC 1: RADJ=9, OSC_EN=1 [F0] 01689 0x2B, 1, 0xF9, //Cycle 1: N_DC=F9 [BE] 01690 TFTLCD_DELAY8, 10, 01691 0x1B, 1, 0x14, //Power 3: BT=1, ??=1, AP=0 [42] 01692 0x1A, 1, 0x11, //Power 2: VC3=1, VC1=1 [05] 01693 0x1C, 1, 0x06, //Power 4: VRH=6 [0D] 01694 0x1F, 1, 0x42, //VCOM : VCM=42 [55] 01695 TFTLCD_DELAY8, 20, 01696 0x19, 1, 0x0A, //Power 1: DK=1, VL_TR1=1 [09] 01697 0x19, 1, 0x1A, //Power 1: PON=1, DK=1, VL_TR1=1 [09] 01698 TFTLCD_DELAY8, 40, 01699 0x19, 1, 0x12, //Power 1: PON=1, DK=1, STB=1 [09] 01700 TFTLCD_DELAY8, 40, 01701 0x1E, 1, 0x27, //Power 6: VCOMG=1, VDV=7 [10] 01702 TFTLCD_DELAY8, 100, 01703 //Display ON Setting 01704 0x24, 1, 0x60, //Display 2: PT=1, GON=1 [A0] 01705 0x3D, 1, 0x40, //Source 1: N_SAP=40 [C0] 01706 0x34, 1, 0x38, //Cycle 10: EQS=0x38 [38] 01707 0x35, 1, 0x38, //Cycle 11: EQP=0x38 [38] 01708 0x24, 1, 0x38, //Display 2: GON=1 D=2 [A0] 01709 TFTLCD_DELAY8, 40, 01710 0x24, 1, 0x3C, //Display 2: GON=1 D=3 [A0] 01711 0x16, 1, 0x1C, //Memaccess: GS=1, BGR=1, SS=1 01712 0x01, 1, 0x06, //Disp Mode: INVON=1, NORON=1 [02] 01713 0x55, 1, 0x06, //SM_PANEL=0, SS_PANEL=0, GS_PANEL=1, REV_PANEL=1, BGR_PANEL=0 01714 }; 01715 init_table(HX8352A_regValues, sizeof(HX8352A_regValues)); 01716 p16 = (int16_t *) & HEIGHT; 01717 *p16 = 400; 01718 break; 01719 #endif 01720 01721 #ifdef SUPPORT_8352B 01722 case 0x0065: //HX8352-B 01723 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | REV_SCREEN; 01724 is8347 = 1; 01725 static const uint8_t HX8352B_regValues[] PROGMEM = { 01726 // Register setting for EQ setting 01727 0xe5, 1, 0x10, // 01728 0xe7, 1, 0x10, // 01729 0xe8, 1, 0x48, // 01730 0xec, 1, 0x09, // 01731 0xed, 1, 0x6c, // 01732 // Power on Setting 01733 0x23, 1, 0x6F, //VMF 01734 0x24, 1, 0x57, //VMH 01735 0x25, 1, 0x71, //VML 01736 0xE2, 1, 0x18, // 01737 0x1B, 1, 0x15, //VRH 01738 0x01, 1, 0x00, // 01739 0x1C, 1, 0x03, //AP=3 01740 // Power on sequence 01741 0x19, 1, 0x01, //OSCEN=1 01742 TFTLCD_DELAY8, 5, 01743 0x1F, 1, 0x8C, //GASEN=1, DK=1, XDK=1 01744 0x1F, 1, 0x84, //GASEN=1, XDK=1 01745 TFTLCD_DELAY8, 10, 01746 0x1F, 1, 0x94, //GASEN=1, PON=1, XDK=1 01747 TFTLCD_DELAY8, 10, 01748 0x1F, 1, 0xD4, //GASEN=1, VCOMG=1, PON=1, XDK=1 01749 TFTLCD_DELAY8, 5, 01750 // Gamma Setting 01751 0x40, 13, 0x00, 0x2B, 0x29, 0x3E, 0x3D, 0x3F, 0x24, 0x74, 0x08, 0x06, 0x07, 0x0D, 0x17, 01752 0x50, 13, 0x00, 0x02, 0x01, 0x16, 0x14, 0x3F, 0x0B, 0x5B, 0x08, 0x12, 0x18, 0x19, 0x17, 01753 0x5D, 1, 0xFF, // 01754 01755 0x16, 1, 0x08, //MemoryAccess BGR=1 01756 0x28, 1, 0x20, //GON=1 01757 TFTLCD_DELAY8, 40, 01758 0x28, 1, 0x38, //GON=1, DTE=1, D=2 01759 TFTLCD_DELAY8, 40, 01760 0x28, 1, 0x3C, //GON=1, DTE=1, D=3 01761 01762 0x02, 2, 0x00, 0x00, //SC 01763 0x04, 2, 0x00, 0xEF, //EC 01764 0x06, 2, 0x00, 0x00, //SP 01765 0x08, 2, 0x01, 0x8F, //EP 01766 01767 0x80, 2, 0x00, 0x00, //CAC 01768 0x82, 2, 0x00, 0x00, //RAC 01769 0x17, 1, 0x05, //COLMOD = 565 01770 01771 }; 01772 init_table(HX8352B_regValues, sizeof(HX8352B_regValues)); 01773 p16 = (int16_t *) & HEIGHT; 01774 *p16 = 400; 01775 break; 01776 #endif 01777 01778 #ifdef SUPPORT_8347A 01779 case 0x8347: 01780 _lcd_capable = REV_SCREEN | MIPI_DCS_REV1 | MV_AXIS; 01781 // AN.01 The reference setting of CMO 3.2” Panel 01782 static const uint8_t HX8347A_CMO32_regValues[] PROGMEM = { 01783 // VENDOR Gamma for 3.2" 01784 (0x46), 12, 0xA4, 0x53, 0x00, 0x44, 0x04, 0x67, 0x33, 0x77, 0x12, 0x4C, 0x46, 0x44, 01785 // Display Setting 01786 (0x01), 1, 0x06, // IDMON=0, INVON=1, NORON=1, PTLON=0 01787 (0x16), 1, 0x48, // MY=0, MX=0, MV=0, ML=1, BGR=0, TEON=0 01788 (0x23), 3, 0x95, 0x95, 0xFF, // N_DC=1001 0101, PI_DC=1001 0101, I_DC=1111 1111 01789 01790 (0x27), 4, 0x02, 0x02, 0x02, 0x02, // N_BP=2, N_FP=2, PI_BP=2, PI_FP=2 01791 (0x2C), 2, 0x02, 0x02, // I_BP=2, I_FP=2 01792 01793 (0x3a), 4, 0x01, 0x01, 0xF0, 0x00, // N_RTN=0, N_NW=1, P_RTN=0, P_NW=1, I_RTN=15, I_NW=0, DIV=0 01794 TFTLCD_DELAY8, 5, 01795 (0x35), 2, 0x38, 0x78, // EQS=38h, EQP=78h 01796 (0x3E), 1, 0x38, // SON=38h 01797 (0x40), 2, 0x0F, 0xF0, // GDON=0Fh, GDOFF 01798 // Power Supply Setting 01799 (0x19), 1, 0x49, // CADJ=0100, CUADJ=100, OSD_EN=1 ,60Hz 01800 (0x93), 1, 0x0F, // RADJ=1111, 100% 01801 TFTLCD_DELAY8, 5, 01802 (0x20), 1, 0x40, // BT=0100 01803 (0x1D), 3, 0x07, 0x00, 0x04, // VC1=7, VC3=0, VRH=?? 01804 //VCOM SETTING for 3.2" 01805 (0x44), 2, 0x4D, 0x11, // VCM=100 1101, VDV=1 0001 01806 TFTLCD_DELAY8, 10, 01807 (0x1C), 1, 0x04, // AP=100 01808 TFTLCD_DELAY8, 20, 01809 (0x1B), 1, 0x18, // GASENB=0, PON=0, DK=1, XDK=0, VLCD_TRI=0, STB=0 01810 TFTLCD_DELAY8, 40, 01811 (0x1B), 1, 0x10, // GASENB=0, PON=1, DK=0, XDK=0, VLCD_TRI=0, STB=0 01812 TFTLCD_DELAY8, 40, 01813 (0x43), 1, 0x80, //set VCOMG=1 01814 TFTLCD_DELAY8, 100, 01815 // Display ON Setting 01816 (0x90), 1, 0x7F, // SAP=0111 1111 01817 (0x26), 1, 0x04, //GON=0, DTE=0, D=01 01818 TFTLCD_DELAY8, 40, 01819 (0x26), 1, 0x24, //GON=1, DTE=0, D=01 01820 (0x26), 1, 0x2C, //GON=1, DTE=0, D=11 01821 TFTLCD_DELAY8, 40, 01822 (0x26), 1, 0x3C, //GON=1, DTE=1, D=11 01823 // INTERNAL REGISTER SETTING 01824 (0x57), 1, 0x02, // TEST_Mode=1: into TEST mode 01825 (0x55), 1, 0x00, // VDC_SEL=000, VDDD=1.95V 01826 (0xFE), 1, 0x5A, // For ESD protection 01827 (0x57), 1, 0x00, // TEST_Mode=0: exit TEST mode 01828 }; 01829 // AN.01 The reference setting of CMO 2.4” Panel 01830 static const uint8_t HX8347A_CMO24_regValues[] PROGMEM = { 01831 // VENDOR Gamma for 2.4" 01832 (0x46), 12, 0x94, 0x41, 0x00, 0x33, 0x23, 0x45, 0x44, 0x77, 0x12, 0xCC, 0x46, 0x82, 01833 // Display Setting 01834 (0x01), 1, 0x06, // IDMON=0, INVON=1, NORON=1, PTLON=0 01835 (0x16), 1, 0x48, // MY=0, MX=0, MV=0, ML=1, BGR=0, TEON=0 01836 (0x23), 3, 0x95, 0x95, 0xFF, // N_DC=1001 0101, PI_DC=1001 0101, I_DC=1111 1111 01837 01838 (0x27), 4, 0x02, 0x02, 0x02, 0x02, // N_BP=2, N_FP=2, PI_BP=2, PI_FP=2 01839 (0x2C), 2, 0x02, 0x02, // I_BP=2, I_FP=2 01840 01841 (0x3a), 4, 0x01, 0x01, 0xF0, 0x00, // N_RTN=0, N_NW=1, P_RTN=0, P_NW=1, I_RTN=15, I_NW=0, DIV=0 01842 TFTLCD_DELAY8, 5, 01843 (0x35), 2, 0x38, 0x78, // EQS=38h, EQP=78h 01844 (0x3E), 1, 0x38, // SON=38h 01845 (0x40), 2, 0x0F, 0xF0, // GDON=0Fh, GDOFF 01846 // Power Supply Setting 01847 (0x19), 1, 0x49, // CADJ=0100, CUADJ=100, OSD_EN=1 ,60Hz 01848 (0x93), 1, 0x0F, // RADJ=1111, 100% 01849 TFTLCD_DELAY8, 5, 01850 (0x20), 1, 0x40, // BT=0100 01851 (0x1D), 3, 0x07, 0x00, 0x04, // VC1=7, VC3=0, VRH=?? 01852 //VCOM SETTING for 2.4" 01853 (0x44), 2, 0x40, 0x12, // VCM=100 0000, VDV=1 0001 01854 TFTLCD_DELAY8, 10, 01855 (0x1C), 1, 0x04, // AP=100 01856 TFTLCD_DELAY8, 20, 01857 (0x1B), 1, 0x18, // GASENB=0, PON=0, DK=1, XDK=0, VLCD_TRI=0, STB=0 01858 TFTLCD_DELAY8, 40, 01859 (0x1B), 1, 0x10, // GASENB=0, PON=1, DK=0, XDK=0, VLCD_TRI=0, STB=0 01860 TFTLCD_DELAY8, 40, 01861 (0x43), 1, 0x80, //set VCOMG=1 01862 TFTLCD_DELAY8, 100, 01863 // Display ON Setting 01864 (0x90), 1, 0x7F, // SAP=0111 1111 01865 (0x26), 1, 0x04, //GON=0, DTE=0, D=01 01866 TFTLCD_DELAY8, 40, 01867 (0x26), 1, 0x24, //GON=1, DTE=0, D=01 01868 (0x26), 1, 0x2C, //GON=1, DTE=0, D=11 01869 TFTLCD_DELAY8, 40, 01870 (0x26), 1, 0x3C, //GON=1, DTE=1, D=11 01871 // INTERNAL REGISTER SETTING 01872 (0x57), 1, 0x02, // TEST_Mode=1: into TEST mode 01873 (0x55), 1, 0x00, // VDC_SEL=000, VDDD=1.95V 01874 (0xFE), 1, 0x5A, // For ESD protection 01875 (0x57), 1, 0x00, // TEST_Mode=0: exit TEST mode 01876 }; 01877 static const uint8_t HX8347A_ITDB02_regValues[] PROGMEM = { 01878 // VENDOR Gamma ITDB02 same as CMO32. Delays are shorter than AN01 01879 (0x46), 12, 0xA4, 0x53, 0x00, 0x44, 0x04, 0x67, 0x33, 0x77, 0x12, 0x4C, 0x46, 0x44, 01880 // Display Setting 01881 (0x01), 1, 0x06, // IDMON=0, INVON=1, NORON=1, PTLON=0 01882 (0x16), 1, 0xC8, // MY=0, MX=0, MV=0, ML=1, BGR=0, TEON=0 .itead 01883 (0x23), 3, 0x95, 0x95, 0xFF, // N_DC=1001 0101, PI_DC=1001 0101, I_DC=1111 1111 01884 01885 (0x27), 4, 0x02, 0x02, 0x02, 0x02, // N_BP=2, N_FP=2, PI_BP=2, PI_FP=2 01886 (0x2C), 2, 0x02, 0x02, // I_BP=2, I_FP=2 01887 01888 (0x3a), 4, 0x01, 0x00, 0xF0, 0x00, // N_RTN=0, N_NW=1, P_RTN=0, ?? P_NW=1, I_RTN=15, I_NW=0, DIV=0 .itead 01889 TFTLCD_DELAY8, 5, 01890 (0x35), 2, 0x38, 0x78, // EQS=38h, EQP=78h 01891 (0x3E), 1, 0x38, // SON=38h 01892 (0x40), 2, 0x0F, 0xF0, // GDON=0Fh, GDOFF 01893 // Power Supply Setting 01894 (0x19), 1, 0x49, // CADJ=0100, CUADJ=100, OSD_EN=1 ,60Hz 01895 (0x93), 1, 0x0F, // RADJ=1111, 100% 01896 TFTLCD_DELAY8, 5, 01897 (0x20), 1, 0x40, // BT=0100 01898 (0x1D), 3, 0x07, 0x00, 0x04, // VC1=7, VC3=0, VRH=?? 01899 //VCOM SETTING for ITDB02 01900 (0x44), 2, 0x4D, 0x0E, // VCM=101 0000 4D, VDV=1 0001 .itead 01901 TFTLCD_DELAY8, 5, 01902 (0x1C), 1, 0x04, // AP=100 01903 TFTLCD_DELAY8, 5, 01904 (0x1B), 1, 0x18, // GASENB=0, PON=0, DK=1, XDK=0, VLCD_TRI=0, STB=0 01905 TFTLCD_DELAY8, 5, 01906 (0x1B), 1, 0x10, // GASENB=0, PON=1, DK=0, XDK=0, VLCD_TRI=0, STB=0 01907 TFTLCD_DELAY8, 5, 01908 (0x43), 1, 0x80, //set VCOMG=1 01909 TFTLCD_DELAY8, 5, 01910 // Display ON Setting 01911 (0x90), 1, 0x7F, // SAP=0111 1111 01912 (0x26), 1, 0x04, //GON=0, DTE=0, D=01 01913 TFTLCD_DELAY8, 5, 01914 (0x26), 1, 0x24, //GON=1, DTE=0, D=01 01915 (0x26), 1, 0x2C, //GON=1, DTE=0, D=11 01916 TFTLCD_DELAY8, 5, 01917 (0x26), 1, 0x3C, //GON=1, DTE=1, D=11 01918 // INTERNAL REGISTER SETTING for ITDB02 01919 (0x57), 1, 0x02, // TEST_Mode=1: into TEST mode 01920 (0x95), 1, 0x01, // SET DISPLAY CLOCK AND PUMPING CLOCK TO SYNCHRONIZE .itead 01921 (0x57), 1, 0x00, // TEST_Mode=0: exit TEST mode 01922 }; 01923 static const uint8_t HX8347A_NHD_regValues[] PROGMEM = { 01924 //Gamma Setting NHD 01925 (0x46), 12, 0x94, 0x41, 0x00, 0x33, 0x23, 0x45, 0x44, 0x77, 0x12, 0xCC, 0x46, 0x82, 01926 (0x01), 1, 0x06, //Display Mode [06] 01927 (0x16), 1, 0xC8, //MADCTL [00] MY=1, MX=1, BGR=1 01928 // (0x70), 1, 0x05, //Panel [06] 16-bit 01929 (0x23), 3, 0x95, 0x95, 0xFF, //Cycle Control 1-3 [95 95 FF] 01930 (0x27), 4, 0x02, 0x02, 0x02, 0x02, //Display Control 2-5 [02 02 02 02] 01931 (0x2C), 2, 0x02, 0x02, //Display Control 6-7 [02 02] 01932 (0x3A), 4, 0x01, 0x01, 0xF0, 0x00, //Cycle Control 1-4 [01 01 F0 00] 01933 TFTLCD_DELAY8, 80, 01934 (0x35), 2, 0x38, 0x78, //Display Control 9-10 [09 09] EQS=56, EQP=120 01935 (0x3E), 1, 0x38, //Cycle Control 5 [38] 01936 (0x40), 1, 0x0F, //Cycle Control 6 [03] GDON=15 01937 (0x41), 1, 0xF0, //Cycle Control 14 [F8] GDOF=248 01938 01939 (0x19), 1, 0x2D, //OSC Control 1 [86] CADJ=2, CUADJ=6, OSCEN=1 01940 (0x93), 1, 0x06, //SAP Idle mode [00] ??? .nhd 01941 TFTLCD_DELAY8, 80, 01942 (0x20), 1, 0x40, //Power Control 6 [40] 01943 (0x1D), 3, 0x07, 0x00, 0x04, //Power Control 3-5 [04 00 06] VC=7 01944 (0x44), 2, 0x3C, 0x12, //VCOM Control 2-3 [5A 11] VCM=60, VDV=18 01945 TFTLCD_DELAY8, 80, 01946 (0x1C), 1, 0x04, //Power Control 2 [04] 01947 TFTLCD_DELAY8, 80, 01948 (0x43), 1, 0x80, //VCOM Control 1 [80] 01949 TFTLCD_DELAY8, 80, 01950 (0x1B), 1, 0x08, //Power Control 1 [00] DK=1 01951 TFTLCD_DELAY8, 80, 01952 (0x1B), 1, 0x10, //Power Control 1 [00] PON=1 01953 TFTLCD_DELAY8, 80, 01954 (0x90), 1, 0x7F, //Display Control 8 [0A] 01955 (0x26), 1, 0x04, //Display Control 1 [A0] D=1 01956 TFTLCD_DELAY8, 80, 01957 (0x26), 1, 0x24, //Display Control 1 [A0] GON=1, D=1 01958 (0x26), 1, 0x2C, //Display Control 1 [A0] GON=1, D=3 01959 TFTLCD_DELAY8, 80, 01960 (0x26), 1, 0x3C, //Display Control 1 [A0] GON=1, DTE=1, D=3 01961 (0x57), 1, 0x02, //? 01962 (0x55), 1, 0x00, //? 01963 (0x57), 1, 0x00, //? 01964 }; 01965 // Atmel ASF code uses VCOM2-3: 0x38, 0x12. 50ms delays and no TEST mode changes. 01966 init_table(HX8347A_NHD_regValues, sizeof(HX8347A_NHD_regValues)); 01967 // init_table(HX8347A_CMO32_regValues, sizeof(HX8347A_CMO32_regValues)); 01968 // init_table(HX8347A_CMO24_regValues, sizeof(HX8347A_CMO24_regValues)); 01969 // init_table(HX8347A_ITDB02_regValues, sizeof(HX8347A_ITDB02_regValues)); 01970 // init_table(HX8347G_2_regValues, sizeof(HX8347G_2_regValues)); 01971 break; 01972 #endif 01973 01974 case 0x8357: //BIG CHANGE: HX8357-B is now 0x8357 01975 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | REV_SCREEN; 01976 goto common_8357; 01977 case 0x9090: //BIG CHANGE: HX8357-D was 0x8357 01978 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | REV_SCREEN | READ_24BITS; 01979 common_8357: 01980 static const uint8_t HX8357C_regValues[] PROGMEM = { 01981 TFTLCD_DELAY8, 1, //dummy table 01982 }; 01983 table8_ads = HX8357C_regValues, table_size = sizeof(HX8357C_regValues); 01984 p16 = (int16_t *) & HEIGHT; 01985 *p16 = 480; 01986 p16 = (int16_t *) & WIDTH; 01987 *p16 = 320; 01988 break; 01989 01990 case 0x0099: //HX8357-D matches datasheet 01991 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | REV_SCREEN | READ_24BITS; 01992 static const uint8_t HX8357_99_regValues[] PROGMEM = { 01993 (0xB9), 3, 0xFF, 0x83, 0x57, // SETEXTC 01994 TFTLCD_DELAY8, 150, 01995 TFTLCD_DELAY8, 150, 01996 (0xB6), 1, 0x25, // SETCOM [4B 00] -2.5V+37*0.02V=-1.76V [-1.00V] 01997 (0xC0), 6, 0x50, 0x50, 0x01, 0x3C, 0x1E, 0x08, // SETSTBA [73 50 00 3C C4 08] 01998 (0xB4), 7, 0x02, 0x40, 0x00, 0x2A, 0x2A, 0x0D, 0x78, // SETCYC [02 40 00 2A 2A 0D 96] 01999 #ifdef SUPPORT_8357D_GAMMA 02000 // HX8357D_SETGAMMA [0B 0C 11 1D 25 37 43 4B 4E 47 41 39 35 31 2E 21 1C 1D 1D 26 31 44 4E 56 44 3F 39 33 31 2E 28 1D E0 01] 02001 (0xE0),34, 0x02, 0x0A, 0x11, 0x1D, 0x23, 0x35, 0x41, 0x4B, 0x4B, 0x42, 0x3A, 0x27, 0x1B, 0x08, 0x09, 0x03, 0x02, 0x0A, 0x11, 0x1D, 0x23, 0x35, 0x41, 0x4B, 0x4B, 0x42, 0x3A, 0x27, 0x1B, 0x08, 0x09, 0x03, 0x00, 0x01, 02002 #endif 02003 }; 02004 table8_ads = HX8357_99_regValues, table_size = sizeof(HX8357_99_regValues); 02005 p16 = (int16_t *) & HEIGHT; 02006 *p16 = 480; 02007 p16 = (int16_t *) & WIDTH; 02008 *p16 = 320; 02009 break; 02010 02011 #ifdef SUPPORT_8230 02012 case 0x8230: //thanks Auzman 02013 _lcd_capable = 0 | REV_SCREEN | INVERT_GS | INVERT_RGB | READ_BGR; 02014 static const uint16_t UC8230_regValues[] PROGMEM = { 02015 //After pin Reset wait at least 100ms 02016 TFTLCD_DELAY, 100, //at least 100ms 02017 0x0046, 0x0002, //MTP Disable 02018 0x0010, 0x1590, //SAP=1, BT=5, APE=1, AP=1 02019 0x0011, 0x0227, //DC1=2, DC0=2, VC=7 02020 0x0012, 0x80ff, //P5VMD=1, PON=7, VRH=15 02021 0x0013, 0x9c31, //VDV=28, VCM=49 02022 TFTLCD_DELAY, 10, //at least 10ms 02023 0x0002, 0x0300, //set N-line = 1 02024 0x0003, 0x1030, //set GRAM writing direction & BGR=1 02025 0x0060, 0xa700, //GS; gate scan: start position & drive line Q'ty 02026 0x0061, 0x0001, //REV, NDL, VLE 02027 /*--------------------Gamma control------------------------*/ 02028 0x0030, 0x0303, 02029 0x0031, 0x0303, 02030 0x0032, 0x0303, 02031 0x0033, 0x0300, 02032 0x0034, 0x0003, 02033 0x0035, 0x0303, 02034 0x0036, 0x1400, 02035 0x0037, 0x0303, 02036 0x0038, 0x0303, 02037 0x0039, 0x0303, 02038 0x003a, 0x0300, 02039 0x003b, 0x0003, 02040 0x003c, 0x0303, 02041 0x003d, 0x1400, 02042 //-----------------------------------------------------------// 02043 0x0020, 0x0000, //GRAM horizontal address 02044 0x0021, 0x0000, //GRAM vertical address 02045 //************** Partial Display control*********************// 02046 0x0080, 0x0000, 02047 0x0081, 0x0000, 02048 0x0082, 0x0000, 02049 0x0083, 0x0000, 02050 0x0084, 0x0000, 02051 0x0085, 0x0000, 02052 //-----------------------------------------------------------// 02053 0x0092, 0x0200, 02054 0x0093, 0x0303, 02055 0x0090, 0x0010, //set clocks/Line 02056 0x0000, 0x0001, 02057 TFTLCD_DELAY, 200, // Delay 200 ms 02058 0x0007, 0x0173, //Display on setting 02059 }; 02060 init_table16(UC8230_regValues, sizeof(UC8230_regValues)); 02061 break; 02062 #endif 02063 02064 #ifdef SUPPORT_9163 02065 case 0x9163: // 02066 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 02067 static const uint8_t PROGMEM table9163C[] = { 02068 // (COMMAND_BYTE), n, data_bytes.... 02069 0x26, 1, 0x02, // [01] GAMMASET use CURVE=1, 2, 4, 8 02070 0xB1, 2, 0x08, 0x02, // [0E 14] FRMCTR1 if GM==011 61.7Hz 02071 0xB4, 1, 0x07, // [02] INVCTR 02072 0xB8, 1, 0x01, // [00] GSCTRL 02073 0xC0, 2, 0x0A, 0x02, // [0A 05] PWCTR1 if LCM==10 02074 0xC1, 1, 0x02, // [07] PWCTR2 02075 0xC5, 2, 0x50, 0x63, // [43 4D] VMCTR1 02076 0xC7, 1, 0, // [40] VCOMOFFS 02077 }; 02078 table8_ads = table9163C, table_size = sizeof(table9163C); // 02079 p16 = (int16_t *) & HEIGHT; 02080 *p16 = 160; 02081 p16 = (int16_t *) & WIDTH; 02082 *p16 = 128; 02083 break; 02084 #endif 02085 02086 #ifdef SUPPORT_9225 02087 #define ILI9225_DRIVER_OUTPUT_CTRL (0x01u) // Driver Output Control 02088 #define ILI9225_LCD_AC_DRIVING_CTRL (0x02u) // LCD AC Driving Control 02089 #define ILI9225_ENTRY_MODE (0x03u) // Entry Mode 02090 #define ILI9225_DISP_CTRL1 (0x07u) // Display Control 1 02091 #define ILI9225_BLANK_PERIOD_CTRL1 (0x08u) // Blank Period Control 02092 #define ILI9225_FRAME_CYCLE_CTRL (0x0Bu) // Frame Cycle Control 02093 #define ILI9225_INTERFACE_CTRL (0x0Cu) // Interface Control 02094 #define ILI9225_OSC_CTRL (0x0Fu) // Osc Control 02095 #define ILI9225_POWER_CTRL1 (0x10u) // Power Control 1 02096 #define ILI9225_POWER_CTRL2 (0x11u) // Power Control 2 02097 #define ILI9225_POWER_CTRL3 (0x12u) // Power Control 3 02098 #define ILI9225_POWER_CTRL4 (0x13u) // Power Control 4 02099 #define ILI9225_POWER_CTRL5 (0x14u) // Power Control 5 02100 #define ILI9225_VCI_RECYCLING (0x15u) // VCI Recycling 02101 #define ILI9225_RAM_ADDR_SET1 (0x20u) // Horizontal GRAM Address Set 02102 #define ILI9225_RAM_ADDR_SET2 (0x21u) // Vertical GRAM Address Set 02103 #define ILI9225_GRAM_DATA_REG (0x22u) // GRAM Data Register 02104 #define ILI9225_GATE_SCAN_CTRL (0x30u) // Gate Scan Control Register 02105 #define ILI9225_VERTICAL_SCROLL_CTRL1 (0x31u) // Vertical Scroll Control 1 Register 02106 #define ILI9225_VERTICAL_SCROLL_CTRL2 (0x32u) // Vertical Scroll Control 2 Register 02107 #define ILI9225_VERTICAL_SCROLL_CTRL3 (0x33u) // Vertical Scroll Control 3 Register 02108 #define ILI9225_PARTIAL_DRIVING_POS1 (0x34u) // Partial Driving Position 1 Register 02109 #define ILI9225_PARTIAL_DRIVING_POS2 (0x35u) // Partial Driving Position 2 Register 02110 #define ILI9225_HORIZONTAL_WINDOW_ADDR1 (0x36u) // Horizontal Address END Position HEA 02111 #define ILI9225_HORIZONTAL_WINDOW_ADDR2 (0x37u) // Horizontal Address START Position HSA 02112 #define ILI9225_VERTICAL_WINDOW_ADDR1 (0x38u) // Vertical Address END Position VEA 02113 #define ILI9225_VERTICAL_WINDOW_ADDR2 (0x39u) // Vertical Address START Position VSA 02114 #define ILI9225_GAMMA_CTRL1 (0x50u) // Gamma Control 1 02115 #define ILI9225_GAMMA_CTRL2 (0x51u) // Gamma Control 2 02116 #define ILI9225_GAMMA_CTRL3 (0x52u) // Gamma Control 3 02117 #define ILI9225_GAMMA_CTRL4 (0x53u) // Gamma Control 4 02118 #define ILI9225_GAMMA_CTRL5 (0x54u) // Gamma Control 5 02119 #define ILI9225_GAMMA_CTRL6 (0x55u) // Gamma Control 6 02120 #define ILI9225_GAMMA_CTRL7 (0x56u) // Gamma Control 7 02121 #define ILI9225_GAMMA_CTRL8 (0x57u) // Gamma Control 8 02122 #define ILI9225_GAMMA_CTRL9 (0x58u) // Gamma Control 9 02123 #define ILI9225_GAMMA_CTRL10 (0x59u) // Gamma Control 10 02124 02125 #define ILI9225C_INVOFF 0x20 02126 #define ILI9225C_INVON 0x21 02127 02128 case 0x6813: 02129 case 0x9226: 02130 _lcd_ID = 0x9225; //fall through 02131 case 0x9225: 02132 _lcd_capable = REV_SCREEN | READ_BGR; //thanks tongbajiel 02133 static const uint16_t ILI9225_regValues[] PROGMEM = { 02134 /* Start Initial Sequence */ 02135 /* Set SS bit and direction output from S528 to S1 */ 02136 ILI9225_POWER_CTRL1, 0x0000, // Set SAP,DSTB,STB 02137 ILI9225_POWER_CTRL2, 0x0000, // Set APON,PON,AON,VCI1EN,VC 02138 ILI9225_POWER_CTRL3, 0x0000, // Set BT,DC1,DC2,DC3 02139 ILI9225_POWER_CTRL4, 0x0000, // Set GVDD 02140 ILI9225_POWER_CTRL5, 0x0000, // Set VCOMH/VCOML voltage 02141 TFTLCD_DELAY, 40, 02142 02143 // Power-on sequence 02144 ILI9225_POWER_CTRL2, 0x0018, // Set APON,PON,AON,VCI1EN,VC 02145 ILI9225_POWER_CTRL3, 0x6121, // Set BT,DC1,DC2,DC3 02146 ILI9225_POWER_CTRL4, 0x006F, // Set GVDD /*007F 0088 */ 02147 ILI9225_POWER_CTRL5, 0x495F, // Set VCOMH/VCOML voltage 02148 ILI9225_POWER_CTRL1, 0x0800, // Set SAP,DSTB,STB 02149 TFTLCD_DELAY, 10, 02150 ILI9225_POWER_CTRL2, 0x103B, // Set APON,PON,AON,VCI1EN,VC 02151 TFTLCD_DELAY, 50, 02152 02153 ILI9225_DRIVER_OUTPUT_CTRL, 0x011C, // set the display line number and display direction 02154 ILI9225_LCD_AC_DRIVING_CTRL, 0x0100, // set 1 line inversion 02155 ILI9225_ENTRY_MODE, 0x1030, // set GRAM write direction and BGR=1. 02156 ILI9225_DISP_CTRL1, 0x0000, // Display off 02157 ILI9225_BLANK_PERIOD_CTRL1, 0x0808, // set the back porch and front porch 02158 ILI9225_FRAME_CYCLE_CTRL, 0x1100, // set the clocks number per line 02159 ILI9225_INTERFACE_CTRL, 0x0000, // CPU interface 02160 ILI9225_OSC_CTRL, 0x0D01, // Set Osc /*0e01*/ 02161 ILI9225_VCI_RECYCLING, 0x0020, // Set VCI recycling 02162 ILI9225_RAM_ADDR_SET1, 0x0000, // RAM Address 02163 ILI9225_RAM_ADDR_SET2, 0x0000, // RAM Address 02164 02165 /* Set GRAM area */ 02166 ILI9225_GATE_SCAN_CTRL, 0x0000, 02167 ILI9225_VERTICAL_SCROLL_CTRL1, 0x00DB, 02168 ILI9225_VERTICAL_SCROLL_CTRL2, 0x0000, 02169 ILI9225_VERTICAL_SCROLL_CTRL3, 0x0000, 02170 ILI9225_PARTIAL_DRIVING_POS1, 0x00DB, 02171 ILI9225_PARTIAL_DRIVING_POS2, 0x0000, 02172 ILI9225_HORIZONTAL_WINDOW_ADDR1, 0x00AF, 02173 ILI9225_HORIZONTAL_WINDOW_ADDR2, 0x0000, 02174 ILI9225_VERTICAL_WINDOW_ADDR1, 0x00DB, 02175 ILI9225_VERTICAL_WINDOW_ADDR2, 0x0000, 02176 02177 /* Set GAMMA curve */ 02178 ILI9225_GAMMA_CTRL1, 0x0000, 02179 ILI9225_GAMMA_CTRL2, 0x0808, 02180 ILI9225_GAMMA_CTRL3, 0x080A, 02181 ILI9225_GAMMA_CTRL4, 0x000A, 02182 ILI9225_GAMMA_CTRL5, 0x0A08, 02183 ILI9225_GAMMA_CTRL6, 0x0808, 02184 ILI9225_GAMMA_CTRL7, 0x0000, 02185 ILI9225_GAMMA_CTRL8, 0x0A00, 02186 ILI9225_GAMMA_CTRL9, 0x0710, 02187 ILI9225_GAMMA_CTRL10, 0x0710, 02188 02189 ILI9225_DISP_CTRL1, 0x0012, 02190 TFTLCD_DELAY, 50, 02191 ILI9225_DISP_CTRL1, 0x1017, 02192 }; 02193 init_table16(ILI9225_regValues, sizeof(ILI9225_regValues)); 02194 p16 = (int16_t *) & HEIGHT; 02195 *p16 = 220; 02196 p16 = (int16_t *) & WIDTH; 02197 *p16 = 176; 02198 break; 02199 #endif 02200 02201 case 0x0001: 02202 _lcd_capable = 0 | REV_SCREEN | INVERT_GS; //no RGB bug. thanks Ivo_Deshev 02203 goto common_9320; 02204 case 0x5408: 02205 _lcd_capable = 0 | REV_SCREEN | READ_BGR; //Red 2.4" thanks jorgenv, Ardlab_Gent 02206 // _lcd_capable = 0 | REV_SCREEN | READ_BGR | INVERT_GS; //Blue 2.8" might be different 02207 goto common_9320; 02208 case 0x1505: //R61505 thanks Ravi_kanchan2004. R61505V, R61505W different 02209 case 0x9320: 02210 _lcd_capable = 0 | REV_SCREEN | READ_BGR; 02211 common_9320: 02212 static const uint16_t ILI9320_regValues[] PROGMEM = { 02213 0x00e5, 0x8000, 02214 0x0000, 0x0001, 02215 0x0001, 0x100, 02216 0x0002, 0x0700, 02217 0x0003, 0x1030, 02218 0x0004, 0x0000, 02219 0x0008, 0x0202, 02220 0x0009, 0x0000, 02221 0x000A, 0x0000, 02222 0x000C, 0x0000, 02223 0x000D, 0x0000, 02224 0x000F, 0x0000, 02225 //-----Power On sequence----------------------- 02226 0x0010, 0x0000, 02227 0x0011, 0x0007, 02228 0x0012, 0x0000, 02229 0x0013, 0x0000, 02230 TFTLCD_DELAY, 50, 02231 0x0010, 0x17B0, //SAP=1, BT=7, APE=1, AP=3 02232 0x0011, 0x0007, //DC1=0, DC0=0, VC=7 02233 TFTLCD_DELAY, 10, 02234 0x0012, 0x013A, //VCMR=1, PON=3, VRH=10 02235 TFTLCD_DELAY, 10, 02236 0x0013, 0x1A00, //VDV=26 02237 0x0029, 0x000c, //VCM=12 02238 TFTLCD_DELAY, 10, 02239 //-----Gamma control----------------------- 02240 0x0030, 0x0000, 02241 0x0031, 0x0505, 02242 0x0032, 0x0004, 02243 0x0035, 0x0006, 02244 0x0036, 0x0707, 02245 0x0037, 0x0105, 02246 0x0038, 0x0002, 02247 0x0039, 0x0707, 02248 0x003C, 0x0704, 02249 0x003D, 0x0807, 02250 //-----Set RAM area----------------------- 02251 0x0060, 0xA700, //GS=1 02252 0x0061, 0x0001, 02253 0x006A, 0x0000, 02254 0x0021, 0x0000, 02255 0x0020, 0x0000, 02256 //-----Partial Display Control------------ 02257 0x0080, 0x0000, 02258 0x0081, 0x0000, 02259 0x0082, 0x0000, 02260 0x0083, 0x0000, 02261 0x0084, 0x0000, 02262 0x0085, 0x0000, 02263 //-----Panel Control---------------------- 02264 0x0090, 0x0010, 02265 0x0092, 0x0000, 02266 0x0093, 0x0003, 02267 0x0095, 0x0110, 02268 0x0097, 0x0000, 02269 0x0098, 0x0000, 02270 //-----Display on----------------------- 02271 0x0007, 0x0173, 02272 TFTLCD_DELAY, 50, 02273 }; 02274 init_table16(ILI9320_regValues, sizeof(ILI9320_regValues)); 02275 break; 02276 case 0x6809: 02277 _lcd_capable = 0 | REV_SCREEN | INVERT_GS | AUTO_READINC; 02278 goto common_93x5; 02279 case 0x9328: 02280 case 0x9325: 02281 _lcd_capable = 0 | REV_SCREEN | INVERT_GS; 02282 goto common_93x5; 02283 case 0x9331: 02284 case 0x9335: 02285 _lcd_capable = 0 | REV_SCREEN; 02286 common_93x5: 02287 static const uint16_t ILI9325_regValues[] PROGMEM = { 02288 0x00E5, 0x78F0, // set SRAM internal timing 02289 0x0001, 0x0100, // set Driver Output Control 02290 0x0002, 0x0200, // set 1 line inversion 02291 0x0003, 0x1030, // set GRAM write direction and BGR=1. 02292 0x0004, 0x0000, // Resize register 02293 0x0005, 0x0000, // .kbv 16bits Data Format Selection 02294 0x0008, 0x0207, // set the back porch and front porch 02295 0x0009, 0x0000, // set non-display area refresh cycle ISC[3:0] 02296 0x000A, 0x0000, // FMARK function 02297 0x000C, 0x0000, // RGB interface setting 02298 0x000D, 0x0000, // Frame marker Position 02299 0x000F, 0x0000, // RGB interface polarity 02300 // ----------- Power On sequence ----------- // 02301 0x0010, 0x0000, // SAP, BT[3:0], AP, DSTB, SLP, STB 02302 0x0011, 0x0007, // DC1[2:0], DC0[2:0], VC[2:0] 02303 0x0012, 0x0000, // VREG1OUT voltage 02304 0x0013, 0x0000, // VDV[4:0] for VCOM amplitude 02305 0x0007, 0x0001, 02306 TFTLCD_DELAY, 200, // Dis-charge capacitor power voltage 02307 0x0010, 0x1690, // SAP=1, BT=6, APE=1, AP=1, DSTB=0, SLP=0, STB=0 02308 0x0011, 0x0227, // DC1=2, DC0=2, VC=7 02309 TFTLCD_DELAY, 50, // wait_ms 50ms 02310 0x0012, 0x000D, // VCIRE=1, PON=0, VRH=5 02311 TFTLCD_DELAY, 50, // wait_ms 50ms 02312 0x0013, 0x1200, // VDV=28 for VCOM amplitude 02313 0x0029, 0x000A, // VCM=10 for VCOMH 02314 0x002B, 0x000D, // Set Frame Rate 02315 TFTLCD_DELAY, 50, // wait_ms 50ms 02316 0x0020, 0x0000, // GRAM horizontal Address 02317 0x0021, 0x0000, // GRAM Vertical Address 02318 // ----------- Adjust the Gamma Curve ----------// 02319 02320 0x0030, 0x0000, 02321 0x0031, 0x0404, 02322 0x0032, 0x0003, 02323 0x0035, 0x0405, 02324 0x0036, 0x0808, 02325 0x0037, 0x0407, 02326 0x0038, 0x0303, 02327 0x0039, 0x0707, 02328 0x003C, 0x0504, 02329 0x003D, 0x0808, 02330 02331 //------------------ Set GRAM area ---------------// 02332 0x0060, 0x2700, // Gate Scan Line GS=0 [0xA700] 02333 0x0061, 0x0001, // NDL,VLE, REV .kbv 02334 0x006A, 0x0000, // set scrolling line 02335 //-------------- Partial Display Control ---------// 02336 0x0080, 0x0000, 02337 0x0081, 0x0000, 02338 0x0082, 0x0000, 02339 0x0083, 0x0000, 02340 0x0084, 0x0000, 02341 0x0085, 0x0000, 02342 //-------------- Panel Control -------------------// 02343 0x0090, 0x0010, 02344 0x0092, 0x0000, 02345 0x0007, 0x0133, // 262K color and display ON 02346 }; 02347 init_table16(ILI9325_regValues, sizeof(ILI9325_regValues)); 02348 break; 02349 02350 #if defined(SUPPORT_9326_5420) 02351 case 0x5420: 02352 case 0x9326: 02353 _lcd_capable = REV_SCREEN | READ_BGR; 02354 static const uint16_t ILI9326_CPT28_regValues[] PROGMEM = { 02355 //************* Start Initial Sequence **********// 02356 0x0702, 0x3008, // Set internal timing, don’t change this value 02357 0x0705, 0x0036, // Set internal timing, don’t change this value 02358 0x070B, 0x1213, // Set internal timing, don’t change this value 02359 0x0001, 0x0100, // set SS and SM bit 02360 0x0002, 0x0100, // set 1 line inversion 02361 0x0003, 0x1030, // set GRAM write direction and BGR=1. 02362 0x0008, 0x0202, // set the back porch and front porch 02363 0x0009, 0x0000, // set non-display area refresh cycle ISC[3:0] 02364 0x000C, 0x0000, // RGB interface setting 02365 0x000F, 0x0000, // RGB interface polarity 02366 //*************Power On sequence ****************// 02367 0x0100, 0x0000, // SAP, BT[3:0], AP, DSTB, SLP, STB 02368 0x0102, 0x0000, // VREG1OUT voltage 02369 0x0103, 0x0000, // VDV[4:0] for VCOM amplitude 02370 TFTLCD_DELAY, 200, // Dis-charge capacitor power voltage 02371 0x0100, 0x1190, // SAP, BT[3:0], AP, DSTB, SLP, STB 02372 0x0101, 0x0227, // DC1[2:0], DC0[2:0], VC[2:0] 02373 TFTLCD_DELAY, 50, // Delay 50ms 02374 0x0102, 0x01BD, // VREG1OUT voltage 02375 TFTLCD_DELAY, 50, // Delay 50ms 02376 0x0103, 0x2D00, // VDV[4:0] for VCOM amplitude 02377 0x0281, 0x000E, // VCM[5:0] for VCOMH 02378 TFTLCD_DELAY, 50, // 02379 0x0200, 0x0000, // GRAM horizontal Address 02380 0x0201, 0x0000, // GRAM Vertical Address 02381 // ----------- Adjust the Gamma Curve ----------// 02382 0x0300, 0x0000, // 02383 0x0301, 0x0707, // 02384 0x0302, 0x0606, // 02385 0x0305, 0x0000, // 02386 0x0306, 0x0D00, // 02387 0x0307, 0x0706, // 02388 0x0308, 0x0005, // 02389 0x0309, 0x0007, // 02390 0x030C, 0x0000, // 02391 0x030D, 0x000A, // 02392 //------------------ Set GRAM area ---------------// 02393 0x0400, 0x3100, // Gate Scan Line 400 lines 02394 0x0401, 0x0001, // NDL,VLE, REV 02395 0x0404, 0x0000, // set scrolling line 02396 //-------------- Partial Display Control ---------// 02397 0x0500, 0x0000, // Partial Image 1 Display Position 02398 0x0501, 0x0000, // Partial Image 1 RAM Start/End Address 02399 0x0502, 0x0000, // Partial Image 1 RAM Start/End Address 02400 0x0503, 0x0000, // Partial Image 2 Display Position 02401 0x0504, 0x0000, // Partial Image 2 RAM Start/End Address 02402 0x0505, 0x0000, // Partial Image 2 RAM Start/End Address 02403 //-------------- Panel Control -------------------// 02404 0x0010, 0x0010, // DIVI[1:0];RTNI[4:0] 02405 0x0011, 0x0600, // NOWI[2:0];SDTI[2:0] 02406 0x0020, 0x0002, // DIVE[1:0];RTNE[5:0] 02407 0x0007, 0x0173, // 262K color and display ON 02408 }; 02409 init_table16(ILI9326_CPT28_regValues, sizeof(ILI9326_CPT28_regValues)); 02410 p16 = (int16_t *) & HEIGHT; 02411 *p16 = 400; 02412 p16 = (int16_t *) & WIDTH; 02413 *p16 = 240; 02414 break; 02415 #endif 02416 02417 case 0x9327: 02418 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; 02419 static const uint8_t ILI9327_regValues[] PROGMEM = { 02420 0xB0, 1, 0x00, //Disable Protect for cmds B1-DF, E0-EF, F0-FF 02421 // 0xE0, 1, 0x20, //NV Memory Write [00] 02422 // 0xD1, 3, 0x00, 0x71, 0x19, //VCOM control [00 40 0F] 02423 // 0xD0, 3, 0x07, 0x01, 0x08, //Power Setting [07 04 8C] 02424 0xC1, 4, 0x10, 0x10, 0x02, 0x02, //Display Timing [10 10 02 02] 02425 0xC0, 6, 0x00, 0x35, 0x00, 0x00, 0x01, 0x02, //Panel Drive [00 35 00 00 01 02 REV=0,GS=0,SS=0 02426 0xC5, 1, 0x04, //Frame Rate [04] 02427 0xD2, 2, 0x01, 0x04, //Power Setting [01 44] 02428 // 0xC8, 15, 0x04, 0x67, 0x35, 0x04, 0x08, 0x06, 0x24, 0x01, 0x37, 0x40, 0x03, 0x10, 0x08, 0x80, 0x00, 02429 // 0xC8, 15, 0x00, 0x77, 0x77, 0x04, 0x04, 0x00, 0x00, 0x00, 0x77, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 02430 0xCA, 1, 0x00, //DGC LUT ??? 02431 0xEA, 1, 0x80, //3-Gamma Function Enable 02432 // 0xB0, 1, 0x03, //Enable Protect 02433 }; 02434 table8_ads = ILI9327_regValues, table_size = sizeof(ILI9327_regValues); 02435 p16 = (int16_t *) & HEIGHT; 02436 *p16 = 400; 02437 p16 = (int16_t *) & WIDTH; 02438 *p16 = 240; 02439 break; 02440 case 0x1602: 02441 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; //does not readGRAM 02442 static const uint8_t XX1602_regValues[] PROGMEM = { 02443 0xB8, 1, 0x01, //GS [00] 02444 0xC0, 1, 0x0E, //??Power [0A] 02445 }; 02446 table8_ads = XX1602_regValues, table_size = sizeof(XX1602_regValues); 02447 break; 02448 02449 case 0x2053: //weird from BangGood 02450 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | REV_SCREEN | READ_BGR; 02451 goto common_9329; 02452 case 0xAC11: 02453 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | REV_SCREEN; //thanks viliam 02454 goto common_9329; 02455 case 0x9302: 02456 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; 02457 goto common_9329; 02458 case 0x9338: 02459 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 02460 goto common_9329; 02461 case 0x9329: 02462 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | INVERT_SS | REV_SCREEN; 02463 common_9329: 02464 static const uint8_t ILI9329_regValues[] PROGMEM = { 02465 // 0xF6, 3, 0x01, 0x01, 0x00, //Interface Control needs EXTC=1 MX_EOR=1, TM=0, RIM=0 02466 // 0xB6, 3, 0x0A, 0x82, 0x27, //Display Function [0A 82 27] 02467 // 0xB7, 1, 0x06, //Entry Mode Set [06] 02468 0x36, 1, 0x00, //Memory Access [00] pointless but stops an empty array 02469 }; 02470 table8_ads = ILI9329_regValues, table_size = sizeof(ILI9329_regValues); 02471 break; 02472 02473 case 0x9340: //ILI9340 thanks Ravi_kanchan2004. 02474 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | REV_SCREEN; 02475 goto common_9341; 02476 case 0x9341: 02477 common_9341: 02478 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 02479 static const uint8_t ILI9341_regValues_2_4[] PROGMEM = { // BOE 2.4" 02480 0xF6, 3, 0x01, 0x01, 0x00, //Interface Control needs EXTC=1 MV_EOR=0, TM=0, RIM=0 02481 0xCF, 3, 0x00, 0x81, 0x30, //Power Control B [00 81 30] 02482 0xED, 4, 0x64, 0x03, 0x12, 0x81, //Power On Seq [55 01 23 01] 02483 0xE8, 3, 0x85, 0x10, 0x78, //Driver Timing A [04 11 7A] 02484 0xCB, 5, 0x39, 0x2C, 0x00, 0x34, 0x02, //Power Control A [39 2C 00 34 02] 02485 0xF7, 1, 0x20, //Pump Ratio [10] 02486 0xEA, 2, 0x00, 0x00, //Driver Timing B [66 00] 02487 0xB0, 1, 0x00, //RGB Signal [00] 02488 0xB1, 2, 0x00, 0x1B, //Frame Control [00 1B] 02489 // 0xB6, 2, 0x0A, 0xA2, 0x27, //Display Function [0A 82 27 XX] .kbv SS=1 02490 0xB4, 1, 0x00, //Inversion Control [02] .kbv NLA=1, NLB=1, NLC=1 02491 0xC0, 1, 0x21, //Power Control 1 [26] 02492 0xC1, 1, 0x11, //Power Control 2 [00] 02493 0xC5, 2, 0x3F, 0x3C, //VCOM 1 [31 3C] 02494 0xC7, 1, 0xB5, //VCOM 2 [C0] 02495 0x36, 1, 0x48, //Memory Access [00] 02496 0xF2, 1, 0x00, //Enable 3G [02] 02497 0x26, 1, 0x01, //Gamma Set [01] 02498 0xE0, 15, 0x0f, 0x26, 0x24, 0x0b, 0x0e, 0x09, 0x54, 0xa8, 0x46, 0x0c, 0x17, 0x09, 0x0f, 0x07, 0x00, 02499 0xE1, 15, 0x00, 0x19, 0x1b, 0x04, 0x10, 0x07, 0x2a, 0x47, 0x39, 0x03, 0x06, 0x06, 0x30, 0x38, 0x0f, 02500 }; 02501 static const uint8_t ILI9341_regValues_ada[] PROGMEM = { // Adafruit_TFTLCD only works with EXTC=0 02502 // 0xF6, 3, 0x00, 0x01, 0x00, //Interface Control needs EXTC=1 TM=0, RIM=0 02503 // 0xF6, 3, 0x01, 0x01, 0x03, //Interface Control needs EXTC=1 RM=1, RIM=1 02504 0xF6, 3, 0x09, 0x01, 0x03, //Interface Control needs EXTC=1 RM=0, RIM=1 02505 0xB0, 1, 0x40, //RGB Signal [40] RCM=2 02506 0xB4, 1, 0x00, //Inversion Control [02] .kbv NLA=1, NLB=1, NLC=1 02507 0xC0, 1, 0x23, //Power Control 1 [26] 02508 0xC1, 1, 0x10, //Power Control 2 [00] 02509 0xC5, 2, 0x2B, 0x2B, //VCOM 1 [31 3C] 02510 0xC7, 1, 0xC0, //VCOM 2 [C0] 02511 0x36, 1, 0x88, //Memory Access [00] 02512 0xB1, 2, 0x00, 0x1B, //Frame Control [00 1B] 02513 0xB7, 1, 0x07, //Entry Mode [00] 02514 }; 02515 table8_ads = ILI9341_regValues_2_4, table_size = sizeof(ILI9341_regValues_2_4); // 02516 break; 02517 #if defined(SUPPORT_9342) 02518 case 0x9342: 02519 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS | INVERT_GS | REV_SCREEN; 02520 static const uint8_t ILI9342_regValues_CPT24[] PROGMEM = { //CPT 2.4" 02521 (0xB9), 3, 0xFF, 0x93, 0x42, //[00 00 00] 02522 (0xC0), 2, 0x1D, 0x0A, //[26 09] 02523 (0xC1), 1, 0x02, //[10] 02524 (0xC5), 2, 0x2F, 0x2F, //[31 3C] 02525 (0xC7), 1, 0xC3, //[C0] 02526 (0xB8), 1, 0x0B, //[07] 02527 (0xE0), 15, 0x0F, 0x33, 0x30, 0x0C, 0x0F, 0x08, 0x5D, 0x66, 0x4A, 0x07, 0x13, 0x05, 0x1B, 0x0E, 0x08, 02528 (0xE1), 15, 0x08, 0x0E, 0x11, 0x02, 0x0E, 0x02, 0x24, 0x33, 0x37, 0x03, 0x0A, 0x09, 0x26, 0x33, 0x0F, 02529 }; 02530 static const uint8_t ILI9342_regValues_Tianma23[] PROGMEM = { //Tianma 2.3" 02531 (0xB9), 3, 0xFF, 0x93, 0x42, 02532 (0xC0), 2, 0x1D, 0x0A, 02533 (0xC1), 1, 0x01, 02534 (0xC5), 2, 0x2C, 0x2C, 02535 (0xC7), 1, 0xC6, 02536 (0xB8), 1, 0x09, 02537 (0xE0), 15, 0x0F, 0x26, 0x21, 0x07, 0x0A, 0x03, 0x4E, 0x62, 0x3E, 0x0B, 0x11, 0x00, 0x08, 0x02, 0x00, 02538 (0xE1), 15, 0x00, 0x19, 0x1E, 0x03, 0x0E, 0x03, 0x30, 0x23, 0x41, 0x03, 0x0B, 0x07, 0x2F, 0x36, 0x0F, 02539 }; 02540 static const uint8_t ILI9342_regValues_HSD23[] PROGMEM = { //HSD 2.3" 02541 (0xB9), 3, 0xFF, 0x93, 0x42, 02542 (0xC0), 2, 0x1D, 0x0A, 02543 (0xC1), 1, 0x02, 02544 (0xC5), 2, 0x2F, 0x27, 02545 (0xC7), 1, 0xA4, 02546 (0xB8), 1, 0x0B, 02547 (0xE0), 15, 0x0F, 0x24, 0x21, 0x0C, 0x0F, 0x06, 0x50, 0x75, 0x3F, 0x07, 0x12, 0x05, 0x11, 0x0B, 0x08, 02548 (0xE1), 15, 0x08, 0x1D, 0x20, 0x02, 0x0E, 0x04, 0x31, 0x24, 0x42, 0x03, 0x0B, 0x09, 0x30, 0x36, 0x0F, 02549 }; 02550 table8_ads = ILI9342_regValues_CPT24, table_size = sizeof(ILI9342_regValues_CPT24); // 02551 // table8_ads = ILI9342_regValues_Tianma23, table_size = sizeof(ILI9342_regValues_Tianma23); // 02552 // table8_ads = ILI9342_regValues_HSD23, table_size = sizeof(ILI9342_regValues_HSD23); // 02553 p16 = (int16_t *) & HEIGHT; 02554 *p16 = 240; 02555 p16 = (int16_t *) & WIDTH; 02556 *p16 = 320; 02557 break; 02558 #endif 02559 case 0x1581: //no BGR in MADCTL. set BGR in Panel Control 02560 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; //thanks zdravke 02561 goto common_9481; 02562 case 0x9481: 02563 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_BGR; 02564 common_9481: 02565 static const uint8_t ILI9481_regValues[] PROGMEM = { // Atmel MaxTouch 02566 0xB0, 1, 0x00, // unlocks E0, F0 02567 0xB3, 4, 0x02, 0x00, 0x00, 0x00, //Frame Memory, interface [02 00 00 00] 02568 0xB4, 1, 0x00, // Frame mode [00] 02569 0xD0, 3, 0x07, 0x42, 0x18, // Set Power [00 43 18] x1.00, x6, x3 02570 0xD1, 3, 0x00, 0x07, 0x10, // Set VCOM [00 00 00] x0.72, x1.02 02571 0xD2, 2, 0x01, 0x02, // Set Power for Normal Mode [01 22] 02572 0xD3, 2, 0x01, 0x02, // Set Power for Partial Mode [01 22] 02573 0xD4, 2, 0x01, 0x02, // Set Power for Idle Mode [01 22] 02574 0xC0, 5, 0x12, 0x3B, 0x00, 0x02, 0x11, //Panel Driving BGR for 1581 [10 3B 00 02 11] 02575 0xC1, 3, 0x10, 0x10, 0x88, // Display Timing Normal [10 10 88] 02576 0xC5, 1, 0x03, //Frame Rate [03] 02577 0xC6, 1, 0x02, //Interface Control [02] 02578 0xC8, 12, 0x00, 0x32, 0x36, 0x45, 0x06, 0x16, 0x37, 0x75, 0x77, 0x54, 0x0C, 0x00, 02579 0xCC, 1, 0x00, //Panel Control [00] 02580 }; 02581 static const uint8_t ILI9481_CPT29_regValues[] PROGMEM = { // 320x430 02582 0xB0, 1, 0x00, 02583 0xD0, 3, 0x07, 0x42, 0x1C, // Set Power [00 43 18] 02584 0xD1, 3, 0x00, 0x02, 0x0F, // Set VCOM [00 00 00] x0.695, x1.00 02585 0xD2, 2, 0x01, 0x11, // Set Power for Normal Mode [01 22] 02586 0xC0, 5, 0x10, 0x35, 0x00, 0x02, 0x11, //Set Panel Driving [10 3B 00 02 11] 02587 0xC5, 1, 0x03, //Frame Rate [03] 02588 0xC8, 12, 0x00, 0x30, 0x36, 0x45, 0x04, 0x16, 0x37, 0x75, 0x77, 0x54, 0x0F, 0x00, 02589 0xE4, 1, 0xA0, 02590 0xF0, 1, 0x01, 02591 0xF3, 2, 0x02, 0x1A, 02592 }; 02593 static const uint8_t ILI9481_PVI35_regValues[] PROGMEM = { // 320x480 02594 0xB0, 1, 0x00, 02595 0xD0, 3, 0x07, 0x41, 0x1D, // Set Power [00 43 18] 02596 0xD1, 3, 0x00, 0x2B, 0x1F, // Set VCOM [00 00 00] x0.900, x1.32 02597 0xD2, 2, 0x01, 0x11, // Set Power for Normal Mode [01 22] 02598 0xC0, 5, 0x10, 0x3B, 0x00, 0x02, 0x11, //Set Panel Driving [10 3B 00 02 11] 02599 0xC5, 1, 0x03, //Frame Rate [03] 02600 0xC8, 12, 0x00, 0x14, 0x33, 0x10, 0x00, 0x16, 0x44, 0x36, 0x77, 0x00, 0x0F, 0x00, 02601 0xE4, 1, 0xA0, 02602 0xF0, 1, 0x01, 02603 0xF3, 2, 0x40, 0x0A, 02604 }; 02605 static const uint8_t ILI9481_AUO317_regValues[] PROGMEM = { // 320x480 02606 0xB0, 1, 0x00, 02607 0xD0, 3, 0x07, 0x40, 0x1D, // Set Power [00 43 18] 02608 0xD1, 3, 0x00, 0x18, 0x13, // Set VCOM [00 00 00] x0.805, x1.08 02609 0xD2, 2, 0x01, 0x11, // Set Power for Normal Mode [01 22] 02610 0xC0, 5, 0x10, 0x3B, 0x00, 0x02, 0x11, //Set Panel Driving [10 3B 00 02 11] 02611 0xC5, 1, 0x03, //Frame Rate [03] 02612 0xC8, 12, 0x00, 0x44, 0x06, 0x44, 0x0A, 0x08, 0x17, 0x33, 0x77, 0x44, 0x08, 0x0C, 02613 0xE4, 1, 0xA0, 02614 0xF0, 1, 0x01, 02615 }; 02616 static const uint8_t ILI9481_CMO35_regValues[] PROGMEM = { // 320480 02617 0xB0, 1, 0x00, 02618 0xD0, 3, 0x07, 0x41, 0x1D, // Set Power [00 43 18] 07,41,1D 02619 0xD1, 3, 0x00, 0x1C, 0x1F, // Set VCOM [00 00 00] x0.825, x1.32 1C,1F 02620 0xD2, 2, 0x01, 0x11, // Set Power for Normal Mode [01 22] 02621 0xC0, 5, 0x10, 0x3B, 0x00, 0x02, 0x11, //Set Panel Driving [10 3B 00 02 11] 02622 0xC5, 1, 0x03, //Frame Rate [03] 02623 0xC6, 1, 0x83, 02624 0xC8, 12, 0x00, 0x26, 0x21, 0x00, 0x00, 0x1F, 0x65, 0x23, 0x77, 0x00, 0x0F, 0x00, 02625 0xF0, 1, 0x01, //? 02626 0xE4, 1, 0xA0, //?SETCABC on Himax 02627 0x36, 1, 0x48, //Memory Access [00] 02628 0xB4, 1, 0x11, 02629 }; 02630 static const uint8_t ILI9481_RGB_regValues[] PROGMEM = { // 320x480 02631 0xB0, 1, 0x00, 02632 0xD0, 3, 0x07, 0x41, 0x1D, // SETPOWER [00 43 18] 02633 0xD1, 3, 0x00, 0x2B, 0x1F, // SETVCOM [00 00 00] x0.900, x1.32 02634 0xD2, 2, 0x01, 0x11, // SETNORPOW for Normal Mode [01 22] 02635 0xC0, 6, 0x10, 0x3B, 0x00, 0x02, 0x11, 0x00, //SETPANEL [10 3B 00 02 11] 02636 0xC5, 1, 0x03, //SETOSC Frame Rate [03] 02637 0xC6, 1, 0x80, //SETRGB interface control 02638 0xC8, 12, 0x00, 0x14, 0x33, 0x10, 0x00, 0x16, 0x44, 0x36, 0x77, 0x00, 0x0F, 0x00, 02639 0xF3, 2, 0x40, 0x0A, 02640 0xF0, 1, 0x08, 02641 0xF6, 1, 0x84, 02642 0xF7, 1, 0x80, 02643 0x0C, 2, 0x00, 0x55, //RDCOLMOD 02644 0xB4, 1, 0x00, //SETDISPLAY 02645 // 0xB3, 4, 0x00, 0x01, 0x06, 0x01, //SETGRAM simple example 02646 0xB3, 4, 0x00, 0x01, 0x06, 0x30, //jpegs example 02647 }; 02648 table8_ads = ILI9481_regValues, table_size = sizeof(ILI9481_regValues); 02649 // table8_ads = ILI9481_CPT29_regValues, table_size = sizeof(ILI9481_CPT29_regValues); 02650 // table8_ads = ILI9481_PVI35_regValues, table_size = sizeof(ILI9481_PVI35_regValues); 02651 // table8_ads = ILI9481_AUO317_regValues, table_size = sizeof(ILI9481_AUO317_regValues); 02652 // table8_ads = ILI9481_CMO35_regValues, table_size = sizeof(ILI9481_CMO35_regValues); 02653 // table8_ads = ILI9481_RGB_regValues, table_size = sizeof(ILI9481_RGB_regValues); 02654 p16 = (int16_t *) & HEIGHT; 02655 *p16 = 480; 02656 p16 = (int16_t *) & WIDTH; 02657 *p16 = 320; 02658 break; 02659 case 0x9486: 02660 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; //Red 3.5", Blue 3.5" 02661 // _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | REV_SCREEN; //old Red 3.5" 02662 static const uint8_t ILI9486_regValues[] PROGMEM = { 02663 /* 02664 0xF2, 9, 0x1C, 0xA3, 0x32, 0x02, 0xB2, 0x12, 0xFF, 0x12, 0x00, //f.k 02665 0xF1, 2, 0x36, 0xA4, // 02666 0xF8, 2, 0x21, 0x04, // 02667 0xF9, 2, 0x00, 0x08, // 02668 */ 02669 0xC0, 2, 0x0d, 0x0d, //Power Control 1 [0E 0E] 02670 0xC1, 2, 0x43, 0x00, //Power Control 2 [43 00] 02671 0xC2, 1, 0x00, //Power Control 3 [33] 02672 0xC5, 4, 0x00, 0x48, 0x00, 0x48, //VCOM Control 1 [00 40 00 40] 02673 0xB4, 1, 0x00, //Inversion Control [00] 02674 0xB6, 3, 0x02, 0x02, 0x3B, // Display Function Control [02 02 3B] 02675 #define GAMMA9486 4 02676 #if GAMMA9486 == 0 02677 // default GAMMA terrible 02678 #elif GAMMA9486 == 1 02679 // GAMMA f.k. bad 02680 0xE0, 15, 0x0f, 0x31, 0x2b, 0x0c, 0x0e, 0x08, 0x4e, 0xf1, 0x37, 0x07, 0x10, 0x03, 0x0e, 0x09, 0x00, 02681 0xE1, 15, 0x00, 0x0e, 0x14, 0x03, 0x11, 0x07, 0x31, 0xC1, 0x48, 0x08, 0x0f, 0x0c, 0x31, 0x36, 0x0f, 02682 #elif GAMMA9486 == 2 02683 // 1.2 CPT 3.5 Inch Initial Code not bad 02684 0xE0, 15, 0x0F, 0x1B, 0x18, 0x0B, 0x0E, 0x09, 0x47, 0x94, 0x35, 0x0A, 0x13, 0x05, 0x08, 0x03, 0x00, 02685 0xE1, 15, 0x0F, 0x3A, 0x37, 0x0B, 0x0C, 0x05, 0x4A, 0x24, 0x39, 0x07, 0x10, 0x04, 0x27, 0x25, 0x00, 02686 #elif GAMMA9486 == 3 02687 // 2.2 HSD 3.5 Inch Initial Code not bad 02688 0xE0, 15, 0x0F, 0x1F, 0x1C, 0x0C, 0x0F, 0x08, 0x48, 0x98, 0x37, 0x0A, 0x13, 0x04, 0x11, 0x0D, 0x00, 02689 0xE1, 15, 0x0F, 0x32, 0x2E, 0x0B, 0x0D, 0x05, 0x47, 0x75, 0x37, 0x06, 0x10, 0x03, 0x24, 0x20, 0x00, 02690 #elif GAMMA9486 == 4 02691 // 3.2 TM 3.2 Inch Initial Code not bad 02692 0xE0, 15, 0x0F, 0x21, 0x1C, 0x0B, 0x0E, 0x08, 0x49, 0x98, 0x38, 0x09, 0x11, 0x03, 0x14, 0x10, 0x00, 02693 0xE1, 15, 0x0F, 0x2F, 0x2B, 0x0C, 0x0E, 0x06, 0x47, 0x76, 0x37, 0x07, 0x11, 0x04, 0x23, 0x1E, 0x00, 02694 #elif GAMMA9486 == 5 02695 // 4.2 WTK 3.5 Inch Initial Code too white 02696 0xE0, 15, 0x0F, 0x10, 0x08, 0x05, 0x09, 0x05, 0x37, 0x98, 0x26, 0x07, 0x0F, 0x02, 0x09, 0x07, 0x00, 02697 0xE1, 15, 0x0F, 0x38, 0x36, 0x0D, 0x10, 0x08, 0x59, 0x76, 0x48, 0x0A, 0x16, 0x0A, 0x37, 0x2F, 0x00, 02698 #endif 02699 }; 02700 table8_ads = ILI9486_regValues, table_size = sizeof(ILI9486_regValues); 02701 p16 = (int16_t *) & HEIGHT; 02702 *p16 = 480; 02703 p16 = (int16_t *) & WIDTH; 02704 *p16 = 320; 02705 break; 02706 case 0x7796: 02707 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS; //thanks to safari1 02708 goto common_9488; 02709 case 0x9487: //with thanks to Charlyf 02710 case 0x9488: 02711 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 02712 common_9488: 02713 static const uint8_t ILI9488_regValues_max[] PROGMEM = { // Atmel MaxTouch 02714 0xC0, 2, 0x10, 0x10, //Power Control 1 [0E 0E] 02715 0xC1, 1, 0x41, //Power Control 2 [43] 02716 0xC5, 4, 0x00, 0x22, 0x80, 0x40, //VCOM Control 1 [00 40 00 40] 02717 0x36, 1, 0x68, //Memory Access [00] 02718 0xB0, 1, 0x00, //Interface [00] 02719 0xB1, 2, 0xB0, 0x11, //Frame Rate Control [B0 11] 02720 0xB4, 1, 0x02, //Inversion Control [02] 02721 0xB6, 3, 0x02, 0x02, 0x3B, // Display Function Control [02 02 3B] .kbv NL=480 02722 0xB7, 1, 0xC6, //Entry Mode [06] 02723 0x3A, 1, 0x55, //Interlace Pixel Format [XX] 02724 0xF7, 4, 0xA9, 0x51, 0x2C, 0x82, //Adjustment Control 3 [A9 51 2C 82] 02725 }; 02726 table8_ads = ILI9488_regValues_max, table_size = sizeof(ILI9488_regValues_max); 02727 p16 = (int16_t *) & HEIGHT; 02728 *p16 = 480; 02729 p16 = (int16_t *) & WIDTH; 02730 *p16 = 320; 02731 break; 02732 case 0xB505: //R61505V 02733 case 0xC505: //R61505W 02734 _lcd_capable = 0 | REV_SCREEN | READ_LOWHIGH; 02735 static const uint16_t R61505V_regValues[] PROGMEM = { 02736 0x0000, 0x0000, 02737 0x0000, 0x0000, 02738 0x0000, 0x0000, 02739 0x0000, 0x0001, 02740 0x00A4, 0x0001, //CALB=1 02741 TFTLCD_DELAY, 10, 02742 0x0060, 0x2700, //NL 02743 0x0008, 0x0808, //FP & BP 02744 0x0030, 0x0214, //Gamma settings 02745 0x0031, 0x3715, 02746 0x0032, 0x0604, 02747 0x0033, 0x0E16, 02748 0x0034, 0x2211, 02749 0x0035, 0x1500, 02750 0x0036, 0x8507, 02751 0x0037, 0x1407, 02752 0x0038, 0x1403, 02753 0x0039, 0x0020, 02754 0x0090, 0x0015, //DIVI & RTNI 02755 0x0010, 0x0410, //BT=4,AP=1 02756 0x0011, 0x0237, //DC1=2,DC0=3, VC=7 02757 0x0029, 0x0046, //VCM1=70 02758 0x002A, 0x0046, //VCMSEL=0,VCM2=70 02759 // Sleep mode IN sequence 02760 0x0007, 0x0000, 02761 //0x0012, 0x0000, //PSON=0,PON=0 02762 // Sleep mode EXIT sequence 02763 0x0012, 0x0189, //VCMR=1,PSON=0,PON=0,VRH=9 02764 0x0013, 0x1100, //VDV=17 02765 TFTLCD_DELAY, 150, 02766 0x0012, 0x01B9, //VCMR=1,PSON=1,PON=1,VRH=9 [018F] 02767 0x0001, 0x0100, //SS=1 Other mode settings 02768 0x0002, 0x0200, //BC0=1--Line inversion 02769 0x0003, 0x1030, 02770 0x0009, 0x0001, //ISC=1 [0000] 02771 0x000A, 0x0000, // [0000] 02772 // 0x000C, 0x0001, //RIM=1 [0000] 02773 0x000D, 0x0000, // [0000] 02774 0x000E, 0x0030, //VEM=3 VCOM equalize [0000] 02775 0x0061, 0x0001, 02776 0x006A, 0x0000, 02777 0x0080, 0x0000, 02778 0x0081, 0x0000, 02779 0x0082, 0x005F, 02780 0x0092, 0x0100, 02781 0x0093, 0x0701, 02782 TFTLCD_DELAY, 80, 02783 0x0007, 0x0100, //BASEE=1--Display On 02784 }; 02785 init_table16(R61505V_regValues, sizeof(R61505V_regValues)); 02786 break; 02787 02788 #if defined(SUPPORT_B509_7793) 02789 case 0x7793: 02790 case 0xB509: 02791 _lcd_capable = REV_SCREEN; 02792 static const uint16_t R61509V_regValues[] PROGMEM = { 02793 0x0000, 0x0000, 02794 0x0000, 0x0000, 02795 0x0000, 0x0000, 02796 0x0000, 0x0000, 02797 TFTLCD_DELAY, 15, 02798 0x0400, 0x6200, //NL=0x31 (49) i.e. 400 rows 02799 0x0008, 0x0808, 02800 //gamma 02801 0x0300, 0x0C00, 02802 0x0301, 0x5A0B, 02803 0x0302, 0x0906, 02804 0x0303, 0x1017, 02805 0x0304, 0x2300, 02806 0x0305, 0x1700, 02807 0x0306, 0x6309, 02808 0x0307, 0x0C09, 02809 0x0308, 0x100C, 02810 0x0309, 0x2232, 02811 02812 0x0010, 0x0016, //69.5Hz 0016 02813 0x0011, 0x0101, 02814 0x0012, 0x0000, 02815 0x0013, 0x0001, 02816 02817 0x0100, 0x0330, //BT,AP 02818 0x0101, 0x0237, //DC0,DC1,VC 02819 0x0103, 0x0D00, //VDV 02820 0x0280, 0x6100, //VCM 02821 0x0102, 0xC1B0, //VRH,VCMR,PSON,PON 02822 TFTLCD_DELAY, 50, 02823 02824 0x0001, 0x0100, 02825 0x0002, 0x0100, 02826 0x0003, 0x1030, //1030 02827 0x0009, 0x0001, 02828 0x000C, 0x0000, 02829 0x0090, 0x8000, 02830 0x000F, 0x0000, 02831 02832 0x0210, 0x0000, 02833 0x0211, 0x00EF, 02834 0x0212, 0x0000, 02835 0x0213, 0x018F, //432=01AF,400=018F 02836 0x0500, 0x0000, 02837 0x0501, 0x0000, 02838 0x0502, 0x005F, //??? 02839 0x0401, 0x0001, //REV=1 02840 0x0404, 0x0000, 02841 TFTLCD_DELAY, 50, 02842 02843 0x0007, 0x0100, //BASEE 02844 TFTLCD_DELAY, 50, 02845 02846 0x0200, 0x0000, 02847 0x0201, 0x0000, 02848 }; 02849 init_table16(R61509V_regValues, sizeof(R61509V_regValues)); 02850 p16 = (int16_t *) & HEIGHT; 02851 *p16 = 400; 02852 break; 02853 #endif 02854 02855 #ifdef SUPPORT_9806 02856 case 0x9806: 02857 _lcd_capable = AUTO_READINC | MIPI_DCS_REV1 | MV_AXIS | READ_24BITS; 02858 // from ZinggJM 02859 static const uint8_t ILI9806_regValues[] PROGMEM = { 02860 (0xFF), 3, /* EXTC Command Set enable register*/ 0xFF, 0x98, 0x06, 02861 (0xBA), 1, /* SPI Interface Setting*/0xE0, 02862 (0xBC), 21, /* GIP 1*/0x03, 0x0F, 0x63, 0x69, 0x01, 0x01, 0x1B, 0x11, 0x70, 0x73, 0xFF, 0xFF, 0x08, 0x09, 0x05, 0x00, 0xEE, 0xE2, 0x01, 0x00, 0xC1, 02863 (0xBD), 8, /* GIP 2*/0x01, 0x23, 0x45, 0x67, 0x01, 0x23, 0x45, 0x67, 02864 (0xBE), 9, /* GIP 3*/0x00, 0x22, 0x27, 0x6A, 0xBC, 0xD8, 0x92, 0x22, 0x22, 02865 (0xC7), 1, /* Vcom*/0x1E, 02866 (0xED), 3, /* EN_volt_reg*/0x7F, 0x0F, 0x00, 02867 (0xC0), 3, /* Power Control 1*/0xE3, 0x0B, 0x00, 02868 (0xFC), 1, 0x08, 02869 (0xDF), 6, /* Engineering Setting*/0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 02870 (0xF3), 1, /* DVDD Voltage Setting*/0x74, 02871 (0xB4), 3, /* Display Inversion Control*/0x00, 0x00, 0x00, 02872 (0xF7), 1, /* 480x854*/0x81, 02873 (0xB1), 3, /* Frame Rate*/0x00, 0x10, 0x14, 02874 (0xF1), 3, /* Panel Timing Control*/0x29, 0x8A, 0x07, 02875 (0xF2), 4, /*Panel Timing Control*/0x40, 0xD2, 0x50, 0x28, 02876 (0xC1), 4, /* Power Control 2*/0x17, 0x85, 0x85, 0x20, 02877 (0xE0), 16, 0x00, 0x0C, 0x15, 0x0D, 0x0F, 0x0C, 0x07, 0x05, 0x07, 0x0B, 0x10, 0x10, 0x0D, 0x17, 0x0F, 0x00, 02878 (0xE1), 16, 0x00, 0x0D, 0x15, 0x0E, 0x10, 0x0D, 0x08, 0x06, 0x07, 0x0C, 0x11, 0x11, 0x0E, 0x17, 0x0F, 0x00, 02879 (0x35), 1, /*Tearing Effect ON*/0x00, 02880 }; 02881 table8_ads = ILI9806_regValues, table_size = sizeof(ILI9806_regValues); 02882 p16 = (int16_t *) & HEIGHT; 02883 *p16 = 480; 02884 p16 = (int16_t *) & WIDTH; 02885 *p16 = 854; 02886 break; 02887 #endif 02888 default: 02889 p16 = (int16_t *) & WIDTH; 02890 *p16 = 0; //error value for WIDTH 02891 break; 02892 } 02893 _lcd_rev = ((_lcd_capable & REV_SCREEN) != 0); 02894 if (table8_ads != NULL) { 02895 static const uint8_t reset_off[] PROGMEM = { 02896 0x01, 0, //Soft Reset 02897 TFTLCD_DELAY8, 150, // .kbv will power up with ONLY reset, sleep out, display on 02898 0x28, 0, //Display Off 02899 0x3A, 1, 0x55, //Pixel read=565, write=565. 02900 }; 02901 static const uint8_t wake_on[] PROGMEM = { 02902 0x11, 0, //Sleep Out 02903 TFTLCD_DELAY8, 150, 02904 0x29, 0, //Display On 02905 }; 02906 init_table(&reset_off, sizeof(reset_off)); 02907 init_table(table8_ads, table_size); //can change PIXFMT 02908 init_table(&wake_on, sizeof(wake_on)); 02909 } 02910 setRotation(0); //PORTRAIT 02911 invertDisplay(false); 02912 #if defined(SUPPORT_9488_555) 02913 if (_lcd_ID == 0x9488) { 02914 is555 = 0; 02915 drawPixel(0, 0, 0xFFE0); 02916 if (readPixel(0, 0) == 0xFF1F) { 02917 uint8_t pixfmt = 0x06; 02918 pushCommand(0x3A, &pixfmt, 1); 02919 _lcd_capable &= ~READ_24BITS; 02920 is555 = 1; 02921 } 02922 } 02923 #endif 02924 }
Generated on Sat Jul 23 2022 01:16:42 by
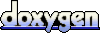