
Embed:
(wiki syntax)
Show/hide line numbers
SerialBuffered.cpp
00001 #include "mbed.h" 00002 #include "LPC.h" 00003 00004 Serial pc2(USBTX, USBRX); 00005 00006 SerialBuffered::SerialBuffered( size_t bufferSize, PinName tx, PinName rx ) : target( tx, rx ) { 00007 m_buffSize = 0; 00008 m_contentStart = 0; 00009 m_contentEnd = 0; 00010 m_timeout = 1.0; 00011 target.attach( this, &SerialBuffered::handleInterrupt ); 00012 m_buff = (uint8_t *) malloc( bufferSize ); 00013 if ( m_buff == NULL ) { 00014 pc2.printf("\n\rERROR: Failed to alloc serial buffer size of %d\r\n", (int) bufferSize ); 00015 } else { 00016 m_buffSize = bufferSize; 00017 } 00018 } 00019 00020 SerialBuffered::~SerialBuffered() { 00021 if ( m_buff ) 00022 free( m_buff ); 00023 } 00024 00025 void SerialBuffered::InitUART(int baud) { //Sets the baud rates 00026 pc2.baud(baud); 00027 target.baud(baud); 00028 } 00029 00030 void SerialBuffered::TargetSendString(char * str) { //Sends string to the board for console communication 00031 for (int i=0; i<strlen(str); i++) { 00032 target.putc(str[i]); 00033 } 00034 } 00035 00036 void SerialBuffered::TargetSendStringAndCR(char * str) { //Sends string to the board for console communication followed by the carriage return character 00037 for (int i=0; i<strlen(str); i++) 00038 target.putc(str[i]); 00039 target.putc(0x0D); 00040 } 00041 00042 void SerialBuffered::setTimeout( float seconds ) { 00043 m_timeout = seconds; 00044 } 00045 00046 size_t SerialBuffered::readBytes(char *bytes, size_t requested) { 00047 int i = 0; 00048 for (int a=0; a<m_buffSize; a++) 00049 bytes[a] = 0; 00050 for (; i<requested;) { 00051 int c = getc(); 00052 if (c<0) 00053 break; 00054 bytes[i] = c; 00055 i++; 00056 } 00057 return i; 00058 } 00059 00060 int SerialBuffered::getc() { 00061 m_timer.reset(); 00062 m_timer.start(); 00063 while (m_contentStart == m_contentEnd) { 00064 wait_ms(1); 00065 if (m_timeout>0 && m_timer.read()>m_timeout) 00066 return EOF; 00067 } 00068 m_timer.stop(); 00069 uint8_t result = m_buff[m_contentStart++]; 00070 m_contentStart = m_contentStart % m_buffSize; 00071 return result; 00072 } 00073 00074 int SerialBuffered::readable() { 00075 return m_contentStart != m_contentEnd ; 00076 } 00077 00078 void SerialBuffered::handleInterrupt() { 00079 while (target.readable()) { 00080 if (m_contentStart == (m_contentEnd +1) % m_buffSize) { 00081 pc2.printf("\n\rERROR: Serial buffer overrun, data lost!\r\n" ); 00082 exit(0); 00083 } else { 00084 m_buff[m_contentEnd++] = target.getc(); 00085 m_contentEnd = m_contentEnd % m_buffSize; 00086 } 00087 } 00088 }
Generated on Tue Jul 19 2022 22:34:47 by
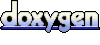