
An Echo server as described in RFC862. Written as a learning exercise for using Donatien's network stack. Hopefully of some use to others to get started with socket programming.
EchoServer.h
00001 #ifndef ECHO_SERVER_H 00002 #define ECHO_SERVER_H 00003 00004 #include "mbed.h" 00005 #include "TCPSocket.h" 00006 #include "UDPSocket.h" 00007 00008 #include "TCPEchoHandler.h" 00009 00010 /* 00011 Class: EchoServer 00012 Binds itself to port 7 on TCP and UDP and listens for 00013 incoming connections 00014 */ 00015 class EchoServer { 00016 public: 00017 // Constructor: EchoServer 00018 // Creates the TCP and UDP sockets and wires up 00019 // the event callback methods 00020 EchoServer(); 00021 // Destructor: ~EchoServer 00022 // Deletes the TCP and UDP sockets 00023 ~EchoServer(); 00024 /* 00025 Function: bind 00026 Binds the sockets to the specified ports 00027 Parameters: 00028 tcpPort - The TCP port to bind to (default 7 as per RFC862) 00029 udpPort - The UDP port to bind to (default 7 as per RFC862) 00030 */ 00031 void bind(int tcpPort=7, int udpPort=7); 00032 private: 00033 // Variable: tcpSock 00034 // The TCP server socket 00035 TCPSocket* tcpSock; 00036 // Variable: udpSock 00037 // The UDP socket 00038 UDPSocket* udpSock; 00039 00040 // Function: onNetTcpSocketEvent 00041 // The callback function called by the network stack whenever an 00042 // event occurs on the TCP socket 00043 // Parameter: e - The event that has occurred 00044 void onNetTcpSocketEvent(TCPSocketEvent e); 00045 // Function: onNetUdpSocketEvent 00046 // The callback function called by the network stack whenever an 00047 // event occurs on the UDP socket 00048 // Parameter: e - The event that has occurred 00049 void onNetUdpSocketEvent(UDPSocketEvent e); 00050 }; 00051 00052 #endif
Generated on Wed Jul 13 2022 01:13:14 by
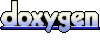