
First attempt at some form of autodiscovery of an mbed based device by periodically broadcasting our IP in a UDP packet.
main.cpp
00001 /* 00002 * Auto Discovery 00003 * Broadcasts information about this mbed on port 2010 00004 * Enables other devices on the same network to find it 00005 */ 00006 00007 #define LWIP_NETIF_HOSTNAME "mbed_dms" 00008 00009 #include "mbed.h" 00010 #include "EthernetNetIf.h" 00011 00012 #include "AutoDiscoveryBroadcaster.h" 00013 00014 // Our Ethernet interface 00015 EthernetNetIf eth; 00016 // Static IP address information 00017 /* 00018 EthernetNetIf eth( 00019 IpAddr(192,168,1,158), //IP Address 00020 IpAddr(255,255,255,0), //Network Mask 00021 IpAddr(192,168,1,254), //Gateway 00022 IpAddr(192,168,1,2) //DNS 00023 ); 00024 */ 00025 // Our AutoDiscoveryBroadcaster server 00026 AutoDiscoveryBroadcaster adb; 00027 00028 /* 00029 Function: main 00030 00031 Sets up the Ethernet interface using DHCP, sets 00032 up the AutoDiscoveryBroadcaster which then 00033 fires out UDP datagrams with info about the mbed 00034 */ 00035 int main() { 00036 00037 printf("\r\nSetting up...\r\n"); 00038 EthernetErr ethErr = eth.setup(); 00039 if (ethErr) { 00040 printf("Error %d in setup on DHCP.\r\n", ethErr); 00041 return -1; 00042 } 00043 printf("Trying to get IP address\r\n"); 00044 IpAddr ip = eth.getIp(); 00045 printf("mbed IP Address is %d.%d.%d.%d\r\n", ip[0], ip[1], ip[2], ip[3]); 00046 00047 adb.start(); 00048 00049 printf("Entering while loop Net::poll()ing\r\n"); 00050 while (1) { 00051 Net::poll(); 00052 } 00053 }
Generated on Sat Jul 16 2022 15:44:47 by
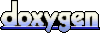