
First attempt at some form of autodiscovery of an mbed based device by periodically broadcasting our IP in a UDP packet.
AutoDiscoveryBroadcaster.h
00001 #ifndef AUTO_DISCOVERY_BROADCASTER_H 00002 #define AUTO_DISCOVERY_BROADCASTER_H 00003 00004 #include "mbed.h" 00005 #include "UDPSocket.h" 00006 #include "BroadcastMessage.h" 00007 00008 /* 00009 Class: AutoDiscoveryBroadcaster 00010 Periodically sends out hostname 00011 and IP address on UDP port 2010 00012 */ 00013 class AutoDiscoveryBroadcaster { 00014 public: 00015 // Constructor: AutoDiscoveryBroadcaster 00016 // Creates the UDP sockets 00017 AutoDiscoveryBroadcaster(); 00018 // Destructor: ~AutoDiscoveryBroadcaster 00019 // Deletes the UDP socket 00020 ~AutoDiscoveryBroadcaster(); 00021 /* 00022 Function: start 00023 Starts periodically sending out the information datagrams 00024 Parameters: 00025 udpPort - The UDP port to send on (default 2010) 00026 period - The period between broadcasts 00027 */ 00028 void start(int udpPort=2010, int period=5); 00029 /* 00030 Function: stop 00031 Stops sending out the information datagrams 00032 */ 00033 void stop(); 00034 private: 00035 // Variable: udpSock 00036 // The UDP socket 00037 UDPSocket* udpSock; 00038 // Variable: running 00039 // Flag to indicate whether we're running or not 00040 char running; 00041 // Variable: ticker 00042 // Ticker used to periodically call broadcast() 00043 Ticker ticker; 00044 Host host; 00045 00046 // Function: broadcast 00047 // The method that actually broadcasts the information 00048 // about this mbed 00049 void broadcast(); 00050 00051 BroadcastMessage msg; 00052 }; 00053 00054 #endif
Generated on Sat Jul 16 2022 15:44:47 by
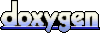