The Modified Dot Library for SX1272
Embed:
(wiki syntax)
Show/hide line numbers
mDot.h
00001 /********************************************************************** 00002 * COPYRIGHT 2015 MULTI-TECH SYSTEMS, INC. 00003 * 00004 * Redistribution and use in source and binary forms, with or without modification, 00005 * are permitted provided that the following conditions are met: 00006 * 1. Redistributions of source code must retain the above copyright notice, 00007 * this list of conditions and the following disclaimer. 00008 * 2. Redistributions in binary form must reproduce the above copyright notice, 00009 * this list of conditions and the following disclaimer in the documentation 00010 * and/or other materials provided with the distribution. 00011 * 3. Neither the name of MULTI-TECH SYSTEMS, INC. nor the names of its contributors 00012 * may be used to endorse or promote products derived from this software 00013 * without specific prior written permission. 00014 * 00015 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00016 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00017 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00018 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00019 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00020 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00021 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00022 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00023 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00024 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00025 * 00026 ****************************************************************************** 00027 */ 00028 00029 #ifndef MDOT_H 00030 #define MDOT_H 00031 00032 #include "mbed.h" 00033 #include "rtos.h" 00034 #include "Mote.h" 00035 #include <vector> 00036 #include <map> 00037 #include <string> 00038 00039 class mDotEvent; 00040 class LoRaConfig; 00041 00042 00043 class mDot { 00044 friend class mDotEvent; 00045 00046 private: 00047 00048 mDot(lora::ChannelPlan* plan); 00049 ~mDot(); 00050 00051 void initLora(); 00052 00053 void setLastError(const std::string& str); 00054 00055 static bool validateBaudRate(const uint32_t& baud); 00056 static bool validateFrequencySubBand(const uint8_t& band); 00057 bool validateDataRate(const uint8_t& dr); 00058 00059 int32_t joinBase(const uint32_t& retries); 00060 int32_t sendBase(const std::vector<uint8_t>& data, const bool& confirmed = false, const bool& blocking = true, const bool& highBw = false); 00061 void waitForPacket(); 00062 void waitForLinkCheck(); 00063 00064 00065 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00066 void setActivityLedState(const uint8_t& state); 00067 00068 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00069 uint8_t getActivityLedState(); 00070 00071 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00072 void blinkActivityLed(void) { 00073 if (_activity_led) { 00074 int val = _activity_led->read(); 00075 _activity_led->write(!val); 00076 } 00077 } 00078 00079 mDot(const mDot&); 00080 mDot& operator=(const mDot&); 00081 00082 uint32_t RTC_ReadBackupRegister(uint32_t RTC_BKP_DR); 00083 void RTC_WriteBackupRegister(uint32_t RTC_BKP_DR, uint32_t Data); 00084 00085 void RTC_DisableWakeupTimer(); 00086 void RTC_EnableWakeupTimer(); 00087 00088 void enterStopMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00089 void enterStandbyMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00090 00091 static mDot* _instance; 00092 00093 lora::Mote* _mote; 00094 LoRaConfig* _config; 00095 lora::Settings _settings; 00096 mDotEvent* _events; 00097 00098 std::string _last_error; 00099 static const uint32_t _baud_rates[]; 00100 uint8_t _activity_led_state; //deprecated will be removed 00101 Ticker _tick; 00102 DigitalOut* _activity_led; //deprecated will be removed 00103 bool _activity_led_enable; //deprecated will be removed 00104 PinName _activity_led_pin; //deprecated will be removed 00105 bool _activity_led_external; //deprecated will be removed 00106 uint8_t _linkFailCount; 00107 uint8_t _class; 00108 InterruptIn* _wakeup; 00109 PinName _wakeup_pin; 00110 00111 typedef enum { 00112 OFF, 00113 ON, 00114 BLINK, 00115 } state; 00116 00117 public: 00118 00119 #if defined(TARGET_MTS_MDOT_F411RE) 00120 typedef enum { 00121 FM_APPEND = (1 << 0), 00122 FM_TRUNC = (1 << 1), 00123 FM_CREAT = (1 << 2), 00124 FM_RDONLY = (1 << 3), 00125 FM_WRONLY = (1 << 4), 00126 FM_RDWR = (FM_RDONLY | FM_WRONLY), 00127 FM_DIRECT = (1 << 5) 00128 } FileMode; 00129 #endif /* TARGET_MTS_MDOT_F411RE */ 00130 00131 typedef enum { 00132 MDOT_OK = 0, 00133 MDOT_INVALID_PARAM = -1, 00134 MDOT_TX_ERROR = -2, 00135 MDOT_RX_ERROR = -3, 00136 MDOT_JOIN_ERROR = -4, 00137 MDOT_TIMEOUT = -5, 00138 MDOT_NOT_JOINED = -6, 00139 MDOT_ENCRYPTION_DISABLED = -7, 00140 MDOT_NO_FREE_CHAN = -8, 00141 MDOT_TEST_MODE = -9, 00142 MDOT_NO_ENABLED_CHAN = -10, 00143 MDOT_AGGREGATED_DUTY_CYCLE = -11, 00144 MDOT_MAX_PAYLOAD_EXCEEDED = -12, 00145 MDOT_LBT_CHANNEL_BUSY = -13, 00146 MDOT_NOT_IDLE = -14, 00147 MDOT_ERROR = -1024, 00148 } mdot_ret_code; 00149 00150 enum JoinMode { 00151 MANUAL = 0, 00152 OTA, 00153 AUTO_OTA, 00154 PEER_TO_PEER 00155 }; 00156 00157 enum Mode { 00158 COMMAND_MODE, 00159 SERIAL_MODE 00160 }; 00161 00162 enum RX_Output { 00163 HEXADECIMAL, 00164 BINARY, 00165 EXTENDED 00166 }; 00167 00168 enum DataRates { 00169 DR0, 00170 DR1, 00171 DR2, 00172 DR3, 00173 DR4, 00174 DR5, 00175 DR6, 00176 DR7, 00177 DR8, 00178 DR9, 00179 DR10, 00180 DR11, 00181 DR12, 00182 DR13, 00183 DR14, 00184 DR15 00185 }; 00186 00187 enum FrequencySubBands { 00188 FSB_ALL, 00189 FSB_1, 00190 FSB_2, 00191 FSB_3, 00192 FSB_4, 00193 FSB_5, 00194 FSB_6, 00195 FSB_7, 00196 FSB_8 00197 }; 00198 00199 enum wakeup_mode { 00200 RTC_ALARM, 00201 INTERRUPT, 00202 RTC_ALARM_OR_INTERRUPT 00203 }; 00204 00205 enum UserBackupRegs { 00206 UBR0, 00207 UBR1, 00208 UBR2, 00209 UBR3, 00210 UBR4, 00211 UBR5, 00212 UBR6, 00213 UBR7, 00214 UBR8, 00215 UBR9 00216 #if defined (TARGET_XDOT_L151CC) 00217 ,UBR10, 00218 UBR11, 00219 UBR12, 00220 UBR13, 00221 UBR14, 00222 UBR15, 00223 UBR16, 00224 UBR17, 00225 UBR18, 00226 UBR19, 00227 UBR20, 00228 UBR21 00229 #endif /* TARGET_XDOT_L151CC */ 00230 }; 00231 00232 #if defined(TARGET_MTS_MDOT_F411RE) 00233 typedef struct { 00234 int16_t fd; 00235 char name[33]; 00236 uint32_t size; 00237 } mdot_file; 00238 #endif /* TARGET_MTS_MDOT_F411RE */ 00239 00240 typedef struct { 00241 uint32_t Up; 00242 uint32_t Down; 00243 uint32_t Joins; 00244 uint32_t JoinFails; 00245 uint32_t MissedAcks; 00246 uint32_t CRCErrors; 00247 } mdot_stats; 00248 00249 typedef struct { 00250 int16_t last; 00251 int16_t min; 00252 int16_t max; 00253 int16_t avg; 00254 } rssi_stats; 00255 00256 typedef struct { 00257 int16_t last; 00258 int16_t min; 00259 int16_t max; 00260 int16_t avg; 00261 } snr_stats; 00262 00263 typedef struct { 00264 bool status; 00265 uint8_t dBm; 00266 uint32_t gateways; 00267 std::vector<uint8_t> payload; 00268 } link_check; 00269 00270 typedef struct { 00271 int32_t status; 00272 int16_t rssi; 00273 int16_t snr; 00274 } ping_response; 00275 00276 static std::string JoinModeStr(uint8_t mode); 00277 static std::string ModeStr(uint8_t mode); 00278 static std::string RxOutputStr(uint8_t format); 00279 static std::string DataRateStr(uint8_t rate); 00280 static std::string FrequencyBandStr(uint8_t band); 00281 static std::string FrequencySubBandStr(uint8_t band); 00282 00283 #if defined(TARGET_MTS_MDOT_F411RE) 00284 uint32_t UserRegisters[10]; 00285 #else 00286 uint32_t UserRegisters[22]; 00287 #endif /* TARGET_MTS_MDOT_F411RE */ 00288 00289 /** 00290 * Get a handle to the singleton object 00291 * @param plan the channel plan to use 00292 * @returns pointer to mDot object 00293 */ 00294 static mDot* getInstance(lora::ChannelPlan* plan); 00295 00296 /** 00297 * Can only be used after a dot has 00298 * configured with a plan 00299 * @returns pointer to mDot object 00300 */ 00301 static mDot* getInstance(); 00302 00303 void setEvents(mDotEvent* events); 00304 00305 /** 00306 * 00307 * Get library version information 00308 * @returns string containing library version information 00309 */ 00310 std::string getId(); 00311 00312 /** 00313 * Get MTS LoRa version information 00314 * @returns string containing MTS LoRa version information 00315 */ 00316 std::string getMtsLoraId(); 00317 00318 /** 00319 * Perform a soft reset of the system 00320 */ 00321 void resetCpu(); 00322 00323 /** 00324 * Reset config to factory default 00325 */ 00326 void resetConfig(); 00327 00328 /** 00329 * Save config data to non volatile memory 00330 * @returns true if success, false if failure 00331 */ 00332 bool saveConfig(); 00333 00334 /** 00335 * Set the log level for the library 00336 * options are: 00337 * NONE_LEVEL - logging is off at this level 00338 * FATAL_LEVEL - only critical errors will be reported 00339 * ERROR_LEVEL 00340 * WARNING_LEVEL 00341 * INFO_LEVEL 00342 * DEBUG_LEVEL 00343 * TRACE_LEVEL - every detail will be reported 00344 * @param level the level to log at 00345 * @returns MDOT_OK if success 00346 */ 00347 int32_t setLogLevel(const uint8_t& level); 00348 00349 /** 00350 * Get the current log level for the library 00351 * @returns current log level 00352 */ 00353 uint8_t getLogLevel(); 00354 00355 /** 00356 * Seed pseudo RNG in LoRaMac layer, uses random value from radio RSSI reading by default 00357 * @param seed for RNG 00358 */ 00359 void seedRandom(uint32_t seed); 00360 00361 /** 00362 * @returns true if MAC command answers are ready to be sent 00363 */ 00364 bool hasMacCommands(); 00365 00366 00367 uint8_t setChannelPlan(lora::ChannelPlan* plan); 00368 00369 lora::Settings* getSettings(); 00370 00371 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00372 void setActivityLedEnable(const bool& enable); 00373 00374 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00375 bool getActivityLedEnable(); 00376 00377 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00378 void setActivityLedPin(const PinName& pin); 00379 00380 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00381 void setActivityLedPin(DigitalOut* pin); 00382 00383 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00384 PinName getActivityLedPin(); 00385 00386 /** 00387 * Returns boolean indicative of start-up from standby mode 00388 * @returns true if dot woke from standby 00389 */ 00390 bool getStandbyFlag(); 00391 00392 std::vector<uint16_t> getChannelMask(); 00393 00394 int32_t setChannelMask(uint8_t offset, uint16_t mask); 00395 00396 /** 00397 * Add a channel 00398 * @returns MDOT_OK 00399 */ 00400 int32_t addChannel(uint8_t index, uint32_t frequency, uint8_t datarateRange); 00401 00402 /** 00403 * Add a downlink channel 00404 * @returns MDOT_OK 00405 */ 00406 int32_t addDownlinkChannel(uint8_t index, uint32_t frequency); 00407 00408 /** 00409 * Get list of channel frequencies currently in use 00410 * @returns vector of channels currently in use 00411 */ 00412 std::vector<uint32_t> getChannels(); 00413 00414 /** 00415 * Get list of downlink channel frequencies currently in use 00416 * @returns vector of channels currently in use 00417 */ 00418 std::vector<uint32_t> getDownlinkChannels(); 00419 00420 /** 00421 * Get list of channel datarate ranges currently in use 00422 * @returns vector of datarate ranges currently in use 00423 */ 00424 std::vector<uint8_t> getChannelRanges(); 00425 00426 /** 00427 * Get list of channel frequencies in config file to be used as session defaults 00428 * @returns vector of channels in config file 00429 */ 00430 std::vector<uint32_t> getConfigChannels(); 00431 00432 /** 00433 * Get list of channel datarate ranges in config file to be used as session defaults 00434 * @returns vector of datarate ranges in config file 00435 */ 00436 std::vector<uint8_t> getConfigChannelRanges(); 00437 00438 /** 00439 * Get default frequency band 00440 * @returns frequency band the device was manufactured for 00441 */ 00442 uint8_t getDefaultFrequencyBand(); 00443 00444 /** 00445 * Set frequency sub band 00446 * only applicable if frequency band is set for United States (FB_915) 00447 * sub band 0 will allow the radio to use all 64 channels 00448 * sub band 1 - 8 will allow the radio to use the 8 channels in that sub band 00449 * for use with Conduit gateway and MTAC_LORA, use sub bands 1 - 8, not sub band 0 00450 * @param band the sub band to use (0 - 8) 00451 * @returns MDOT_OK if success 00452 */ 00453 int32_t setFrequencySubBand(const uint8_t& band); 00454 00455 /** 00456 * Get frequency sub band 00457 * @returns frequency sub band currently in use 00458 */ 00459 uint8_t getFrequencySubBand(); 00460 00461 /** 00462 * Get frequency band 00463 * @returns frequency band (channel plan) currently in use 00464 */ 00465 uint8_t getFrequencyBand(); 00466 00467 /** 00468 * Get channel plan name 00469 * @returns name of channel plan currently in use 00470 */ 00471 std::string getChannelPlanName(); 00472 00473 /** 00474 * Get the datarate currently in use within the MAC layer 00475 * returns 0-15 00476 */ 00477 uint8_t getSessionDataRate(); 00478 00479 00480 /** 00481 * Get the current max EIRP used in the channel plan 00482 * May be changed by the network server 00483 * returns 0-36 00484 */ 00485 uint8_t getSessionMaxEIRP(); 00486 00487 /** 00488 * Set the current max EIRP used in the channel plan 00489 * May be changed by the network server 00490 * accepts 0-36 00491 */ 00492 void setSessionMaxEIRP(uint8_t max); 00493 00494 /** 00495 * Get the current downlink dwell time used in the channel plan 00496 * May be changed by the network server 00497 * returns 0-1 00498 */ 00499 uint8_t getSessionDownlinkDwelltime(); 00500 00501 /** 00502 * Set the current downlink dwell time used in the channel plan 00503 * May be changed by the network server 00504 * accepts 0-1 00505 */ 00506 void setSessionDownlinkDwelltime(uint8_t dwell); 00507 00508 /** 00509 * Get the current uplink dwell time used in the channel plan 00510 * May be changed by the network server 00511 * returns 0-1 00512 */ 00513 uint8_t getSessionUplinkDwelltime(); 00514 00515 /** 00516 * Set the current uplink dwell time used in the channel plan 00517 * May be changed by the network server 00518 * accepts 0-1 00519 */ 00520 void setSessionUplinkDwelltime(uint8_t dwell); 00521 00522 /** 00523 * Set the current downlink dwell time used in the channel plan 00524 * May be changed by the network server 00525 * accepts 0-1 00526 */ 00527 uint32_t getListenBeforeTalkTime(uint8_t ms); 00528 00529 /** 00530 * Set the current downlink dwell time used in the channel plan 00531 * May be changed by the network server 00532 * accepts 0-1 00533 */ 00534 void setListenBeforeTalkTime(uint32_t ms); 00535 00536 /** 00537 * Set public network mode 00538 * 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00539 * PRIVATE_MTS - Sync Word 0x12, US/AU Downlink frequencies per Frequency Sub Band 00540 * PUBLIC_LORAWAN - Sync Word 0x34 00541 * PRIVATE_LORAWAN - Sync Word 0x12 00542 * 00543 * The default Join Delay is 5 seconds 00544 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00545 * The Join Delay must be changed independently of Public Network setting 00546 * 00547 * @see lora::NetworkType 00548 * @returns MDOT_OK if success 00549 */ 00550 int32_t setPublicNetwork(const uint8_t& val); 00551 00552 /** 00553 * Get public network mode 00554 * 00555 * The default Join Delay is 5 seconds 00556 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00557 * The Join Delay must be changed independently of Public Network setting 00558 * 00559 * @see lora:NetworkType 00560 * @returns 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00561 */ 00562 uint8_t getPublicNetwork(); 00563 00564 /** 00565 * Get the device ID 00566 * @returns vector containing the device ID (size 8) 00567 */ 00568 std::vector<uint8_t> getDeviceId(); 00569 00570 /** 00571 * Get the device port to be used for lora application data (1-223) 00572 * @returns port 00573 */ 00574 uint8_t getAppPort(); 00575 00576 /** 00577 * Set the device port to be used for lora application data (1-223) 00578 * @returns MDOT_OK if success 00579 */ 00580 int32_t setAppPort(uint8_t port); 00581 00582 /** 00583 * Set the device class A, B or C 00584 * @returns MDOT_OK if success 00585 */ 00586 int32_t setClass(std::string newClass); 00587 00588 /** 00589 * Get the device class A, B or C 00590 * @returns MDOT_OK if success 00591 */ 00592 std::string getClass(); 00593 00594 /** 00595 * Get the max packet length with current settings 00596 * @returns max packet length 00597 */ 00598 uint8_t getMaxPacketLength(); 00599 00600 /** 00601 * Set network address 00602 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00603 * @param addr a vector of 4 bytes 00604 * @returns MDOT_OK if success 00605 */ 00606 int32_t setNetworkAddress(const std::vector<uint8_t>& addr); 00607 00608 /** 00609 * Get network address 00610 * @returns vector containing network address (size 4) 00611 */ 00612 std::vector<uint8_t> getNetworkAddress(); 00613 00614 /** 00615 * Set network session key 00616 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00617 * @param key a vector of 16 bytes 00618 * @returns MDOT_OK if success 00619 */ 00620 int32_t setNetworkSessionKey(const std::vector<uint8_t>& key); 00621 00622 /** 00623 * Get network session key 00624 * @returns vector containing network session key (size 16) 00625 */ 00626 std::vector<uint8_t> getNetworkSessionKey(); 00627 00628 /** 00629 * Set data session key 00630 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00631 * @param key a vector of 16 bytes 00632 * @returns MDOT_OK if success 00633 */ 00634 int32_t setDataSessionKey(const std::vector<uint8_t>& key); 00635 00636 /** 00637 * Get data session key 00638 * @returns vector containing data session key (size 16) 00639 */ 00640 std::vector<uint8_t> getDataSessionKey(); 00641 00642 /** 00643 * Set network name 00644 * for use with OTA & AUTO_OTA network join modes 00645 * generates network ID (crc64 of name) automatically 00646 * @param name a string of of at least 8 bytes and no more than 128 bytes 00647 * @return MDOT_OK if success 00648 */ 00649 int32_t setNetworkName(const std::string& name); 00650 00651 /** 00652 * Get network name 00653 * @return string containing network name (size 8 to 128) 00654 */ 00655 std::string getNetworkName(); 00656 00657 /** 00658 * Set network ID 00659 * for use with OTA & AUTO_OTA network join modes 00660 * setting network ID via this function sets network name to empty 00661 * @param id a vector of 8 bytes 00662 * @returns MDOT_OK if success 00663 */ 00664 int32_t setNetworkId(const std::vector<uint8_t>& id); 00665 00666 /** 00667 * Get network ID 00668 * @returns vector containing network ID (size 8) 00669 */ 00670 std::vector<uint8_t> getNetworkId(); 00671 00672 /** 00673 * Set network passphrase 00674 * for use with OTA & AUTO_OTA network join modes 00675 * generates network key (cmac of passphrase) automatically 00676 * @param name a string of of at least 8 bytes and no more than 128 bytes 00677 * @return MDOT_OK if success 00678 */ 00679 int32_t setNetworkPassphrase(const std::string& passphrase); 00680 00681 /** 00682 * Get network passphrase 00683 * @return string containing network passphrase (size 8 to 128) 00684 */ 00685 std::string getNetworkPassphrase(); 00686 00687 /** 00688 * Set network key 00689 * for use with OTA & AUTO_OTA network join modes 00690 * setting network key via this function sets network passphrase to empty 00691 * @param id a vector of 16 bytes 00692 * @returns MDOT_OK if success 00693 */ 00694 int32_t setNetworkKey(const std::vector<uint8_t>& id); 00695 00696 /** 00697 * Get network key 00698 * @returns a vector containing network key (size 16) 00699 */ 00700 std::vector<uint8_t> getNetworkKey(); 00701 00702 /** 00703 * Set lorawan application EUI 00704 * equivalent to setNetworkId 00705 * @param eui application EUI (size 8) 00706 */ 00707 int32_t setAppEUI(const uint8_t* eui); 00708 00709 /** 00710 * Get lorawan application EUI 00711 * equivalent to getNetworkId 00712 * @returns vector containing application EUI (size 8) 00713 */ 00714 const uint8_t* getAppEUI(); 00715 00716 /** 00717 * Set lorawan application key 00718 * equivalent to setNetworkKey 00719 * @param eui application key (size 16) 00720 */ 00721 int32_t setAppKey(const uint8_t* key); 00722 00723 /** 00724 * Set lorawan application key 00725 * equivalent to getNetworkKey 00726 * @returns eui application key (size 16) 00727 */ 00728 const uint8_t* getAppKey(); 00729 00730 /** 00731 * Add a multicast session address and keys 00732 * Downlink counter is set to 0 00733 * Up to 3 MULTICAST_SESSIONS can be set 00734 */ 00735 int32_t setMulticastSession(uint8_t index, uint32_t addr, const uint8_t* nsk, const uint8_t* dsk); 00736 00737 /** 00738 * Set a multicast session counter 00739 * Up to 3 MULTICAST_SESSIONS can be set 00740 */ 00741 int32_t setMulticastDownlinkCounter(uint8_t index, uint32_t count); 00742 00743 /** 00744 * Attempt to join network 00745 * each attempt will be made with a random datarate up to the configured datarate 00746 * JoinRequest backoff between tries is enforced to 1% for 1st hour, 0.1% for 1-10 hours and 0.01% after 10 hours 00747 * Check getNextTxMs() for time until next join attempt can be made 00748 * @returns MDOT_OK if success 00749 */ 00750 int32_t joinNetwork(); 00751 00752 /** 00753 * Attempts to join network once 00754 * @returns MDOT_OK if success 00755 */ 00756 int32_t joinNetworkOnce(); 00757 00758 /** 00759 * Resets current network session, essentially disconnecting from the network 00760 * has no effect for MANUAL network join mode 00761 */ 00762 void resetNetworkSession(); 00763 00764 /** 00765 * Restore saved network session from flash 00766 * has no effect for MANUAL network join mode 00767 */ 00768 void restoreNetworkSession(); 00769 00770 /** 00771 * Save current network session to flash 00772 * has no effect for MANUAL network join mode 00773 */ 00774 void saveNetworkSession(); 00775 00776 /** 00777 * Set number of times joining will retry each sub-band before changing 00778 * to the next subband in US915 and AU915 00779 * @param retries must be between 0 - 255 00780 * @returns MDOT_OK if success 00781 */ 00782 int32_t setJoinRetries(const uint8_t& retries); 00783 00784 /** 00785 * Get number of times joining will retry each sub-band 00786 * @returns join retries (0 - 255) 00787 */ 00788 uint8_t getJoinRetries(); 00789 00790 /** 00791 * Set network join mode 00792 * MANUAL: set network address and session keys manually 00793 * OTA: User sets network name and passphrase, then attempts to join 00794 * AUTO_OTA: same as OTA, but network sessions can be saved and restored 00795 * @param mode MANUAL, OTA, or AUTO_OTA 00796 * @returns MDOT_OK if success 00797 */ 00798 int32_t setJoinMode(const uint8_t& mode); 00799 00800 /** 00801 * Get network join mode 00802 * @returns MANUAL, OTA, or AUTO_OTA 00803 */ 00804 uint8_t getJoinMode(); 00805 00806 /** 00807 * Get network join status 00808 * @returns true if currently joined to network 00809 */ 00810 bool getNetworkJoinStatus(); 00811 00812 /** 00813 * Do a network link check 00814 * application data may be returned in response to a network link check command 00815 * @returns link_check structure containing success, dBm above noise floor, gateways in range, and packet payload 00816 */ 00817 link_check networkLinkCheck(); 00818 00819 /** 00820 * Set network link check count to perform automatic link checks every count packets 00821 * only applicable if ACKs are disabled 00822 * @param count must be between 0 - 255 00823 * @returns MDOT_OK if success 00824 */ 00825 int32_t setLinkCheckCount(const uint8_t& count); 00826 00827 /** 00828 * Get network link check count 00829 * @returns count (0 - 255) 00830 */ 00831 uint8_t getLinkCheckCount(); 00832 00833 /** 00834 * Set network link check threshold, number of link check failures or missed acks to tolerate 00835 * before considering network connection lost 00836 * @pararm count must be between 0 - 255 00837 * @returns MDOT_OK if success 00838 */ 00839 int32_t setLinkCheckThreshold(const uint8_t& count); 00840 00841 /** 00842 * Get network link check threshold 00843 * @returns threshold (0 - 255) 00844 */ 00845 uint8_t getLinkCheckThreshold(); 00846 00847 /** 00848 * Get/set number of failed link checks in the current session 00849 * @returns count (0 - 255) 00850 */ 00851 uint8_t getLinkFailCount(); 00852 int32_t setLinkFailCount(uint8_t count); 00853 00854 /** 00855 * Set UpLinkCounter number of packets sent to the gateway during this network session (sequence number) 00856 * @returns MDOT_OK 00857 */ 00858 int32_t setUpLinkCounter(uint32_t count); 00859 00860 /** 00861 * Get UpLinkCounter 00862 * @returns number of packets sent to the gateway during this network session (sequence number) 00863 */ 00864 uint32_t getUpLinkCounter(); 00865 00866 /** 00867 * Set UpLinkCounter number of packets sent by the gateway during this network session (sequence number) 00868 * @returns MDOT_OK 00869 */ 00870 int32_t setDownLinkCounter(uint32_t count); 00871 00872 /** 00873 * Get DownLinkCounter 00874 * @returns number of packets sent by the gateway during this network session (sequence number) 00875 */ 00876 uint32_t getDownLinkCounter(); 00877 00878 /** 00879 * Enable/disable AES encryption 00880 * AES encryption must be enabled for use with Conduit gateway and MTAC_LORA card 00881 * @param on true for AES encryption to be enabled 00882 * @returns MDOT_OK if success 00883 */ 00884 int32_t setAesEncryption(const bool& on); 00885 00886 /** 00887 * Get AES encryption 00888 * @returns true if AES encryption is enabled 00889 */ 00890 bool getAesEncryption(); 00891 00892 /** 00893 * Get RSSI stats 00894 * @returns rssi_stats struct containing last, min, max, and avg RSSI in dB 00895 */ 00896 rssi_stats getRssiStats(); 00897 00898 /** 00899 * Get SNR stats 00900 * @returns snr_stats struct containing last, min, max, and avg SNR in cB 00901 */ 00902 snr_stats getSnrStats(); 00903 00904 /** 00905 * Get ms until next free channel 00906 * only applicable for European models, US models return 0 00907 * @returns time (ms) until a channel is free to use for transmitting 00908 */ 00909 uint32_t getNextTxMs(); 00910 00911 /** 00912 * Get available bytes for payload 00913 * @returns bytes 00914 */ 00915 uint8_t getNextTxMaxSize(); 00916 00917 /** 00918 * Get join delay in seconds 00919 * Defaults to 5 seconds 00920 * Must match join delay setting of the network server 00921 * 00922 * The default Join Delay is 5 seconds 00923 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00924 * 00925 * @returns number of seconds before join accept message is expected 00926 */ 00927 uint8_t getJoinDelay(); 00928 00929 /** 00930 * Set join delay in seconds 00931 * Defaults to 5 seconds 00932 * Must match join delay setting of the network server 00933 * 00934 * The default Join Delay is 5 seconds 00935 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00936 * 00937 * @param delay number of seconds before join accept message is expected 00938 * @return MDOT_OK if success 00939 */ 00940 uint32_t setJoinDelay(uint8_t delay); 00941 00942 /** 00943 * Get join Rx1 datarate offset 00944 * defaults to 0 00945 * @returns offset 00946 */ 00947 uint8_t getJoinRx1DataRateOffset(); 00948 00949 /** 00950 * Set join Rx1 datarate offset 00951 * @param offset for datarate 00952 * @return MDOT_OK if success 00953 */ 00954 uint32_t setJoinRx1DataRateOffset(uint8_t offset); 00955 00956 /** 00957 * Get join Rx2 datarate 00958 * defaults to US:DR8, AU:DR8, EU:DR0 00959 * @returns datarate 00960 */ 00961 uint8_t getJoinRx2DataRate(); 00962 00963 /** 00964 * Set join Rx2 datarate 00965 * @param datarate 00966 * @return MDOT_OK if success 00967 */ 00968 uint32_t setJoinRx2DataRate(uint8_t datarate); 00969 00970 /** 00971 * Get join Rx2 frequency 00972 * defaults US:923.3, AU:923.3, EU:869.525 00973 * @returns frequency 00974 */ 00975 uint32_t getJoinRx2Frequency(); 00976 00977 /** 00978 * Set join Rx2 frequency 00979 * @param frequency 00980 * @return MDOT_OK if success 00981 */ 00982 uint32_t setJoinRx2Frequency(uint32_t frequency); 00983 00984 /** 00985 * Get rx delay in seconds 00986 * Defaults to 1 second 00987 * @returns number of seconds before response message is expected 00988 */ 00989 uint8_t getRxDelay(); 00990 00991 /** 00992 * Set rx delay in seconds 00993 * Defaults to 1 second 00994 * @param delay number of seconds before response message is expected 00995 * @return MDOT_OK if success 00996 */ 00997 uint32_t setRxDelay(uint8_t delay); 00998 00999 /** 01000 * Get preserve session to save network session info through reset or power down in AUTO_OTA mode 01001 * Defaults to off 01002 * @returns true if enabled 01003 */ 01004 bool getPreserveSession(); 01005 01006 /** 01007 * Set preserve session to save network session info through reset or power down in AUTO_OTA mode 01008 * Defaults to off 01009 * @param enable 01010 * @return MDOT_OK if success 01011 */ 01012 uint32_t setPreserveSession(bool enable); 01013 01014 /** 01015 * Get data pending 01016 * only valid after sending data to the gateway 01017 * @returns true if server has available packet(s) 01018 */ 01019 bool getDataPending(); 01020 01021 /** 01022 * Get ack requested 01023 * only valid after sending data to the gateway 01024 * @returns true if server has requested ack 01025 */ 01026 bool getAckRequested(); 01027 01028 /** 01029 * Get is transmitting indicator 01030 * @returns true if currently transmitting 01031 */ 01032 bool getIsTransmitting(); 01033 01034 /** 01035 * Get is idle indicator 01036 * @returns true if not currently transmitting, waiting or receiving 01037 */ 01038 bool getIsIdle(); 01039 01040 /** 01041 * Set TX data rate 01042 * data rates affect maximum payload size 01043 * @param dr DR0-DR7 for Europe, DR0-DR4 for United States 01044 * @returns MDOT_OK if success 01045 */ 01046 int32_t setTxDataRate(const uint8_t& dr); 01047 01048 /** 01049 * Get TX data rate 01050 * @returns current TX data rate (DR0-DR15) 01051 */ 01052 uint8_t getTxDataRate(); 01053 01054 /** 01055 * Get a random value from the radio based on RSSI 01056 * @returns randome value 01057 */ 01058 uint32_t getRadioRandom(); 01059 01060 /** 01061 * Get data rate spreading factor and bandwidth 01062 * EU868 Datarates 01063 * --------------- 01064 * DR0 - SF12BW125 01065 * DR1 - SF11BW125 01066 * DR2 - SF10BW125 01067 * DR3 - SF9BW125 01068 * DR4 - SF8BW125 01069 * DR5 - SF7BW125 01070 * DR6 - SF7BW250 01071 * DR7 - FSK 01072 * 01073 * US915 Datarates 01074 * --------------- 01075 * DR0 - SF10BW125 01076 * DR1 - SF9BW125 01077 * DR2 - SF8BW125 01078 * DR3 - SF7BW125 01079 * DR4 - SF8BW500 01080 * 01081 * AU915 Datarates 01082 * --------------- 01083 * DR0 - SF10BW125 01084 * DR1 - SF9BW125 01085 * DR2 - SF8BW125 01086 * DR3 - SF7BW125 01087 * DR4 - SF8BW500 01088 * 01089 * @returns spreading factor and bandwidth 01090 */ 01091 std::string getDataRateDetails(uint8_t rate); 01092 MBED_DEPRECATED("Will be removed in 3.3.0") 01093 std::string getDateRateDetails(uint8_t rate); 01094 01095 01096 /** 01097 * Set TX power output of radio before antenna gain, default: 14 dBm 01098 * actual output power may be limited by local regulations for the chosen frequency 01099 * power affects maximum range 01100 * @param power 2 dBm - 20 dBm 01101 * @returns MDOT_OK if success 01102 */ 01103 int32_t setTxPower(const uint32_t& power); 01104 01105 /** 01106 * Get TX power 01107 * @returns TX power (2 dBm - 20 dBm) 01108 */ 01109 uint32_t getTxPower(); 01110 01111 /** 01112 * Get configured gain of installed antenna, default: +3 dBi 01113 * @returns gain of antenna in dBi 01114 */ 01115 int8_t getAntennaGain(); 01116 01117 /** 01118 * Set configured gain of installed antenna, default: +3 dBi 01119 * @param gain -127 dBi - 128 dBi 01120 * @returns MDOT_OK if success 01121 */ 01122 int32_t setAntennaGain(int8_t gain); 01123 01124 /** 01125 * Enable/disable TX waiting for rx windows 01126 * when enabled, send calls will block until a packet is received or RX timeout 01127 * @param enable set to true if expecting responses to transmitted packets 01128 * @returns MDOT_OK if success 01129 */ 01130 int32_t setTxWait(const bool& enable); 01131 01132 /** 01133 * Get TX wait 01134 * @returns true if TX wait is enabled 01135 */ 01136 bool getTxWait(); 01137 01138 /** 01139 * Cancel pending rx windows 01140 */ 01141 void cancelRxWindow(); 01142 01143 /** 01144 * Get time on air 01145 * @returns the amount of time (in ms) it would take to send bytes bytes based on current configuration 01146 */ 01147 uint32_t getTimeOnAir(uint8_t bytes); 01148 01149 /** 01150 * Get min frequency 01151 * @returns minimum frequency based on current channel plan 01152 */ 01153 uint32_t getMinFrequency(); 01154 01155 /** 01156 * Get max frequency 01157 * @returns maximum frequency based on current channel plan 01158 */ 01159 uint32_t getMaxFrequency(); 01160 01161 /** 01162 * Get min datarate 01163 * @returns minimum datarate based on current channel plan 01164 */ 01165 uint8_t getMinDatarate(); 01166 01167 /** 01168 * Get max datarate 01169 * @returns maximum datarate based on current channel plan 01170 */ 01171 uint8_t getMaxDatarate(); 01172 01173 /** 01174 * Get min datarate offset 01175 * @returns minimum datarate offset based on current channel plan 01176 */ 01177 uint8_t getMinDatarateOffset(); 01178 01179 /** 01180 * Get max datarate offset 01181 * @returns maximum datarate based on current channel plan 01182 */ 01183 uint8_t getMaxDatarateOffset(); 01184 01185 /** 01186 * Get min datarate 01187 * @returns minimum datarate based on current channel plan 01188 */ 01189 uint8_t getMinRx2Datarate(); 01190 01191 /** 01192 * Get max rx2 datarate 01193 * @returns maximum rx2 datarate based on current channel plan 01194 */ 01195 uint8_t getMaxRx2Datarate(); 01196 01197 /** 01198 * Get max tx power 01199 * @returns maximum tx power based on current channel plan 01200 */ 01201 uint8_t getMaxTxPower(); 01202 01203 /** 01204 * Get min tx power 01205 * @returns minimum tx power based on current channel plan 01206 */ 01207 uint8_t getMinTxPower(); 01208 01209 /** 01210 * Set ping slot periodicity 01211 * Specify the the number of ping slots in a given beacon interval 01212 * Note: Must switch back to class A for the change to take effect 01213 * @param exp - number_of_pings = 2^(7 - exp) where 0 <= exp <= 7 01214 * @returns MDOT_OK if success 01215 */ 01216 uint32_t setPingPeriodicity(uint8_t exp); 01217 01218 /** 01219 * Get ping slot periodicity 01220 * @returns exp = 7 - log2(number_of_pings) 01221 */ 01222 uint8_t getPingPeriodicity(); 01223 01224 /** 01225 * 01226 * get/set adaptive data rate 01227 * configure data rates and power levels based on signal to noise of packets received at gateway 01228 * true == adaptive data rate is on 01229 * set function returns MDOT_OK if success 01230 */ 01231 int32_t setAdr(const bool& on); 01232 bool getAdr(); 01233 01234 /** 01235 * Set the ADR ACK Limit 01236 * @param limit - ADR ACK limit 01237 */ 01238 void setAdrAckLimit(uint16_t limit); 01239 01240 /** 01241 * Get the ADR ACK Limit 01242 * @returns ADR ACK limit 01243 */ 01244 uint16_t getAdrAckLimit(); 01245 01246 /** 01247 * Set the ADR ACK Delay 01248 * @param delay - ADR ACK delay 01249 */ 01250 void setAdrAckDelay(uint16_t delay); 01251 01252 /** 01253 * Get the ADR ACK Delay 01254 * @returns ADR ACK delay 01255 */ 01256 uint16_t getAdrAckDelay(); 01257 01258 /** 01259 * Enable/disable CRC checking of packets 01260 * CRC checking must be enabled for use with Conduit gateway and MTAC_LORA card 01261 * @param on set to true to enable CRC checking 01262 * @returns MDOT_OK if success 01263 */ 01264 int32_t setCrc(const bool& on); 01265 01266 /** 01267 * Get CRC checking 01268 * @returns true if CRC checking is enabled 01269 */ 01270 bool getCrc(); 01271 01272 /** 01273 * Set ack 01274 * @param retries 0 to disable acks, otherwise 1 - 8 01275 * @returns MDOT_OK if success 01276 */ 01277 int32_t setAck(const uint8_t& retries); 01278 01279 /** 01280 * Get ack 01281 * @returns 0 if acks are disabled, otherwise retries (1 - 8) 01282 */ 01283 uint8_t getAck(); 01284 01285 /** 01286 * Set number of packet repeats for unconfirmed frames 01287 * @param repeat 0 or 1 for no repeats, otherwise 2-15 01288 * @returns MDOT_OK if success 01289 */ 01290 int32_t setRepeat(const uint8_t& repeat); 01291 01292 /** 01293 * Get number of packet repeats for unconfirmed frames 01294 * @returns 0 or 1 if no repeats, otherwise 2-15 01295 */ 01296 uint8_t getRepeat(); 01297 01298 /** 01299 * Send data to the gateway 01300 * validates data size (based on spreading factor) 01301 * @param data a vector of up to 242 bytes (may be less based on spreading factor) 01302 * @returns MDOT_OK if packet was sent successfully (ACKs disabled), or if an ACK was received (ACKs enabled) 01303 */ 01304 int32_t send(const std::vector<uint8_t>& data, const bool& blocking = true, const bool& highBw = false); 01305 01306 /** 01307 * Inject mac command 01308 * @param data a vector containing mac commands 01309 * @returns MDOT_OK 01310 */ 01311 int32_t injectMacCommand(const std::vector<uint8_t>& data); 01312 01313 /** 01314 * Clear MAC command buffer to be sent in next uplink 01315 * @returns MDOT_OK 01316 */ 01317 int32_t clearMacCommands(); 01318 01319 /** 01320 * Get MAC command buffer to be sent in next uplink 01321 * @returns command bytes 01322 */ 01323 std::vector<uint8_t> getMacCommands(); 01324 01325 /** 01326 * Fetch data received from the gateway 01327 * this function only checks to see if a packet has been received - it does not open a receive window 01328 * send() must be called before recv() 01329 * @param data a vector to put the received data into 01330 * @returns MDOT_OK if packet was successfully received 01331 */ 01332 int32_t recv(std::vector<uint8_t>& data); 01333 01334 /** 01335 * Ping 01336 * status will be MDOT_OK if ping succeeded 01337 * @returns ping_response struct containing status, RSSI, and SNR 01338 */ 01339 ping_response ping(); 01340 01341 /** 01342 * Get return code string 01343 * @returns string containing a description of the given error code 01344 */ 01345 static std::string getReturnCodeString(const int32_t& code); 01346 01347 /** 01348 * Get last error 01349 * @returns string explaining the last error that occured 01350 */ 01351 std::string getLastError(); 01352 01353 /** 01354 * Go to sleep 01355 * @param interval the number of seconds to sleep before waking up if wakeup_mode == RTC_ALARM or RTC_ALARM_OR_INTERRUPT, else ignored 01356 * @param wakeup_mode RTC_ALARM, INTERRUPT, RTC_ALARM_OR_INTERRUPT 01357 * if RTC_ALARM the real time clock is configured to wake the device up after the specified interval 01358 * if INTERRUPT the device will wake up on the rising edge of the interrupt pin 01359 * if RTC_ALARM_OR_INTERRUPT the device will wake on the first event to occur 01360 * @param deepsleep if true go into deep sleep mode (lowest power, all memory and registers are lost, peripherals turned off) 01361 * else go into sleep mode (low power, memory and registers are maintained, peripherals stay on) 01362 * 01363 * For the MDOT 01364 * in sleep mode, the device can be woken up on an XBEE_DI (2-8) pin or by the RTC alarm 01365 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, XBEE_DIO7) or by the RTC alarm 01366 * For the XDOT 01367 * in sleep mode, the device can be woken up on GPIO (0-3), UART1_RX, WAKE or by the RTC alarm 01368 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, WAKE) or by the RTC alarm 01369 * @returns MDOT_OK on success 01370 */ 01371 int32_t sleep(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM, const bool& deepsleep = true); 01372 01373 /** 01374 * Set auto sleep mode 01375 * Auto sleep mode will automatically put the MCU to sleep after tx and in between receive windows 01376 * Note: The MCU will go into a stop mode sleep in between rx windows. This means that 01377 * peripherals such as timers will not function during the sleep intervals. 01378 * @param enable - Flag to enable auto sleep mode 01379 */ 01380 void setAutoSleep(bool enable); 01381 01382 /** 01383 * Get auto sleep mode 01384 * @returns 0 if sleep mode is disabled, 1 if it is enabled 01385 */ 01386 uint8_t getAutoSleep(); 01387 01388 /** 01389 * Set wake pin 01390 * @param pin the pin to use to wake the device from sleep mode 01391 * For MDOT, XBEE_DI (2-8) 01392 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01393 */ 01394 void setWakePin(const PinName& pin); 01395 01396 /** 01397 * Get wake pin 01398 * @returns the pin to use to wake the device from sleep mode 01399 * For MDOT, XBEE_DI (2-8) 01400 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01401 */ 01402 PinName getWakePin(); 01403 01404 /** 01405 * Write data in a user backup register 01406 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01407 * @param data user data to back up 01408 * @returns true if success 01409 */ 01410 bool writeUserBackupRegister(uint32_t reg, uint32_t data); 01411 01412 /** 01413 * Read data in a user backup register 01414 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01415 * @param data gets set to content of register 01416 * @returns true if success 01417 */ 01418 bool readUserBackupRegister(uint32_t reg, uint32_t& data); 01419 01420 /** 01421 * Set LBT time in us 01422 * @param ms time in us 01423 * @returns true if success 01424 */ 01425 bool setLbtTimeUs(uint16_t us); 01426 01427 /** 01428 * Get LBT time in us 01429 * @returns LBT time in us 01430 */ 01431 uint16_t getLbtTimeUs(); 01432 01433 /** 01434 * Set LBT threshold in dBm 01435 * @param rssi threshold in dBm 01436 * @returns true if success 01437 */ 01438 bool setLbtThreshold(int8_t rssi); 01439 01440 /** 01441 * Get LBT threshold in dBm 01442 * @returns LBT threshold in dBm 01443 */ 01444 int8_t getLbtThreshold(); 01445 01446 /** 01447 * Get Radio Frequency Offset 01448 * Used for fine calibration of radio frequencies 01449 * @returns frequency offset in MHz 01450 */ 01451 int32_t getFrequencyOffset(); 01452 /** 01453 * Get Radio Frequency Offset 01454 * Used for fine calibration of radio frequencies 01455 * @param offset frequency offset in MHz 01456 */ 01457 void setFrequencyOffset(int32_t offset); 01458 01459 /** 01460 * Get GPS time from network server 01461 * Sends a DeviceTimeReq command to the network server 01462 * @returns time since GPS epoch, 0 on failure 01463 */ 01464 uint64_t getGPSTime(); 01465 01466 #if defined(TARGET_MTS_MDOT_F411RE) 01467 /////////////////////////////////////////////////////////////////// 01468 // Filesystem (Non Volatile Memory) Operation Functions for mDot // 01469 /////////////////////////////////////////////////////////////////// 01470 01471 // Save user file data to flash 01472 // file - name of file max 30 chars 01473 // data - data of file 01474 // size - size of file 01475 // returns true if successful 01476 bool saveUserFile(const char* file, void* data, uint32_t size); 01477 01478 // Append user file data to flash 01479 // file - name of file max 30 chars 01480 // data - data of file 01481 // size - size of file 01482 // returns true if successful 01483 bool appendUserFile(const char* file, void* data, uint32_t size); 01484 01485 // Read user file data from flash 01486 // file - name of file max 30 chars 01487 // data - data of file 01488 // size - size of file 01489 // returns true if successful 01490 bool readUserFile(const char* file, void* data, uint32_t size); 01491 01492 // Move a user file in flash 01493 // file - name of file 01494 // new_name - new name of file 01495 // returns true if successful 01496 bool moveUserFile(const char* file, const char* new_name); 01497 01498 // Delete user file data from flash 01499 // file - name of file max 30 chars 01500 // returns true if successful 01501 bool deleteUserFile(const char* file); 01502 01503 // Open user file in flash, max of 4 files open concurrently 01504 // file - name of file max 30 chars 01505 // mode - combination of FM_APPEND | FM_TRUNC | FM_CREAT | 01506 // FM_RDONLY | FM_WRONLY | FM_RDWR | FM_DIRECT 01507 // returns - mdot_file struct, fd field will be a negative value if file could not be opened 01508 mDot::mdot_file openUserFile(const char* file, int mode); 01509 01510 // Seek an open file 01511 // file - mdot file struct 01512 // offset - offset in bytes 01513 // whence - where offset is based SEEK_SET, SEEK_CUR, SEEK_END 01514 // returns true if successful 01515 bool seekUserFile(mDot::mdot_file& file, size_t offset, int whence); 01516 01517 // Read bytes from open file 01518 // file - mdot file struct 01519 // data - mem location to store data 01520 // length - number of bytes to read 01521 // returns - number of bytes read, negative if error 01522 int readUserFile(mDot::mdot_file& file, void* data, size_t length); 01523 01524 // Write bytes to open file 01525 // file - mdot file struct 01526 // data - data to write 01527 // length - number of bytes to write 01528 // returns - number of bytes written, negative if error 01529 int writeUserFile(mDot::mdot_file& file, void* data, size_t length); 01530 01531 // Close open file 01532 // file - mdot file struct 01533 // returns true if successful 01534 bool closeUserFile(mDot::mdot_file& file); 01535 01536 // List user files stored in flash 01537 std::vector<mDot::mdot_file> listUserFiles(); 01538 01539 // Move file into the firmware upgrade path to be flashed on next boot 01540 // file - name of file 01541 // returns true if successful 01542 bool moveUserFileToFirmwareUpgrade(const char* file); 01543 01544 // Return total size of all files saved in FLASH 01545 // Does not include SPIFFS overhead 01546 uint32_t getUsedSpace(); 01547 #else 01548 /////////////////////////////////////////////////////////////// 01549 // EEPROM (Non Volatile Memory) Operation Functions for xDot // 01550 /////////////////////////////////////////////////////////////// 01551 01552 // Write to EEPROM 01553 // addr - address to write to (0 - 0x17FF) 01554 // data - data to write 01555 // size - size of data 01556 // returns true if successful 01557 bool nvmWrite(uint16_t addr, void* data, uint16_t size); 01558 01559 // Read from EEPROM 01560 // addr - address to read from (0 - 0x17FF) 01561 // data - buffer for data 01562 // size - size of buffer 01563 // returns true if successful 01564 bool nvmRead(uint16_t addr, void* data, uint16_t size); 01565 #endif /* TARGET_MTS_MDOT_F411RE */ 01566 01567 // get current statistics 01568 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01569 mdot_stats getStats(); 01570 01571 // reset statistics 01572 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01573 void resetStats(); 01574 01575 // Convert pin number 2-8 to pin name DIO2-DI8 01576 static PinName pinNum2Name(uint8_t num); 01577 01578 // Convert pin name DIO2-DI8 to pin number 2-8 01579 static uint8_t pinName2Num(PinName name); 01580 01581 // Convert pin name DIO2-DI8 to string 01582 static std::string pinName2Str(PinName name); 01583 01584 uint64_t crc64(uint64_t crc, const unsigned char *s, uint64_t l); 01585 01586 /************************************************************************* 01587 * The following functions are only used by the AT command application and 01588 * should not be used by standard applications consuming the mDot library 01589 ************************************************************************/ 01590 01591 // set/get configured baud rate for command port 01592 // only for use in conjunction with AT interface 01593 // set function returns MDOT_OK if success 01594 int32_t setBaud(const uint32_t& baud); 01595 uint32_t getBaud(); 01596 01597 // set/get baud rate for debug port 01598 // set function returns MDOT_OK if success 01599 int32_t setDebugBaud(const uint32_t& baud); 01600 uint32_t getDebugBaud(); 01601 01602 // set/get command terminal echo 01603 // set function returns MDOT_OK if success 01604 int32_t setEcho(const bool& on); 01605 bool getEcho(); 01606 01607 // set/get command terminal verbose mode 01608 // set function returns MDOT_OK if success 01609 int32_t setVerbose(const bool& on); 01610 bool getVerbose(); 01611 01612 // set/get startup mode 01613 // COMMAND_MODE (default), starts up ready to accept AT commands 01614 // SERIAL_MODE, read serial data and send it as LoRa packets 01615 // set function returns MDOT_OK if success 01616 int32_t setStartUpMode(const uint8_t& mode); 01617 uint8_t getStartUpMode(); 01618 01619 int32_t setRxDataRate(const uint8_t& dr); 01620 uint8_t getRxDataRate(); 01621 01622 // get/set TX/RX frequency 01623 // if set to 0, device will hop frequencies 01624 // set function returns MDOT_OK if success 01625 int32_t setTxFrequency(const uint32_t& freq); 01626 uint32_t getTxFrequency(); 01627 int32_t setRxFrequency(const uint32_t& freq); 01628 uint32_t getRxFrequency(); 01629 01630 // get/set RX output mode 01631 // valid options are HEXADECIMAL, BINARY, and EXTENDED 01632 // set function returns MDOT_OK if success 01633 int32_t setRxOutput(const uint8_t& mode); 01634 uint8_t getRxOutput(); 01635 01636 // get/set serial wake interval 01637 // valid values are 2 s - INT_MAX (2147483647) s 01638 // set function returns MDOT_OK if success 01639 int32_t setWakeInterval(const uint32_t& interval); 01640 uint32_t getWakeInterval(); 01641 01642 // get/set serial wake delay 01643 // valid values are 2 ms - INT_MAX (2147483647) ms 01644 // set function returns MDOT_OK if success 01645 int32_t setWakeDelay(const uint32_t& delay); 01646 uint32_t getWakeDelay(); 01647 01648 // get/set serial receive timeout 01649 // valid values are 0 ms - 65000 ms 01650 // set function returns MDOT_OK if success 01651 int32_t setWakeTimeout(const uint16_t& timeout); 01652 uint16_t getWakeTimeout(); 01653 01654 // get/set serial wake mode 01655 // valid values are INTERRUPT or RTC_ALARM 01656 // set function returns MDOT_OK if success 01657 int32_t setWakeMode(const uint8_t& delay); 01658 uint8_t getWakeMode(); 01659 01660 // get/set serial flow control enabled 01661 // set function returns MDOT_OK if success 01662 int32_t setFlowControl(const bool& on); 01663 bool getFlowControl(); 01664 01665 // get/set serial clear on error 01666 // if enabled the data read from the serial port will be discarded if it cannot be sent or if the send fails 01667 // set function returns MDOT_OK if success 01668 int32_t setSerialClearOnError(const bool& on); 01669 bool getSerialClearOnError(); 01670 01671 // MTS_RADIO_DEBUG_COMMANDS 01672 01673 /** 01674 * Disable Duty cycle 01675 * enables or disables the duty cycle limitations 01676 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01677 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01678 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01679 * @param val true to disable duty-cycle (default:false) 01680 */ 01681 int32_t setDisableDutyCycle(bool val); 01682 01683 /** 01684 * Disable Duty cycle 01685 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01686 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01687 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01688 * @return true if duty-cycle is disabled (default:false) 01689 */ 01690 uint8_t getDisableDutyCycle(); 01691 01692 /** 01693 * LBT RSSI 01694 * @return the current RSSI on the configured frequency (SetTxFrequency) using configured LBT Time 01695 */ 01696 int16_t lbtRssi(); 01697 01698 void openRxWindow(uint32_t timeout, uint8_t bandwidth = 0); 01699 void closeRxWindow(); 01700 void sendContinuous(bool enable=true, uint32_t timeout=0, uint32_t frequency=0, int8_t txpower=-1); 01701 int32_t setDeviceId(const std::vector<uint8_t>& id); 01702 int32_t setProtectedAppEUI(const std::vector<uint8_t>& appEUI); 01703 int32_t setProtectedAppKey(const std::vector<uint8_t>& appKey); 01704 int32_t setDefaultFrequencyBand(const uint8_t& band); 01705 bool saveProtectedConfig(); 01706 // resets the radio/mac/link 01707 void resetRadio(); 01708 int32_t setRadioMode(const uint8_t& mode); 01709 std::map<uint8_t, uint8_t> dumpRegisters(); 01710 void eraseFlash(); 01711 01712 void setWakeupCallback(void (*function)(void)); 01713 01714 template<typename T> 01715 void setWakeupCallback(T *object, void (T::*member)(void)) { 01716 _wakeup_callback.attach(object, member); 01717 } 01718 01719 lora::ChannelPlan* getChannelPlan(void); 01720 01721 uint32_t setRx2DataRate(uint8_t dr); 01722 uint8_t getRx2DataRate(); 01723 01724 void mcGroupKeys(uint8_t *mcKeyEncrypt, uint32_t addr, uint8_t groupId, uint32_t frame_count); 01725 private: 01726 01727 void sleep_ms(uint32_t interval, 01728 uint8_t wakeup_mode = RTC_ALARM, 01729 bool deepsleep = true); 01730 01731 01732 void wakeup(); 01733 01734 mdot_stats _stats; 01735 01736 FunctionPointer _wakeup_callback; 01737 01738 bool _standbyFlag; 01739 bool _testMode; 01740 uint8_t _savedPort; 01741 void handleTestModePacket(); 01742 lora::ChannelPlan* _plan; 01743 }; 01744 01745 #endif
Generated on Wed Jul 13 2022 16:42:53 by
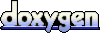