The Modified Dot Library for SX1272
Embed:
(wiki syntax)
Show/hide line numbers
Lora.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora namespace defines global settings, structures and enums for the lora library 00011 * 00012 * @details 00013 * 00014 * 00015 * 00016 */ 00017 00018 #ifndef __LORA_H__ 00019 #define __LORA_H__ 00020 00021 #include "mbed.h" 00022 #include <assert.h> 00023 #include "MTSLog.h" 00024 //#include <cstring> 00025 #include <inttypes.h> 00026 00027 namespace lora { 00028 00029 /** 00030 * Frequency bandwidth of a Datarate, higher bandwidth gives higher datarate 00031 */ 00032 enum Bandwidth { 00033 BW_125, 00034 BW_250, 00035 BW_500, 00036 BW_FSK = 50 00037 }; 00038 00039 /** 00040 * Spreading factor of a Datarate, lower spreading factor gives higher datarate 00041 */ 00042 enum SpreadingFactors { 00043 SF_6 = 6, 00044 SF_7, 00045 SF_8, 00046 SF_9, 00047 SF_10, 00048 SF_11, 00049 SF_12, 00050 SF_FSK, 00051 SF_INVALID 00052 }; 00053 00054 /** 00055 * Datarates for use with ChannelPlan 00056 */ 00057 enum Datarates { 00058 DR_0 = 0, 00059 DR_1, 00060 DR_2, 00061 DR_3, 00062 DR_4, 00063 DR_5, 00064 DR_6, 00065 DR_7, 00066 DR_8, 00067 DR_9, 00068 DR_10, 00069 DR_11, 00070 DR_12, 00071 DR_13, 00072 DR_14, 00073 DR_15 00074 }; 00075 00076 const uint8_t MIN_DATARATE = (uint8_t) DR_0; //!< Minimum datarate 00077 00078 00079 const uint8_t MAX_PHY_PACKET_SIZE = 255; //!< Maximum size for a packet 00080 const uint8_t MAX_APPS = 8; //!< Maximum number of apps sessions to save 00081 const uint8_t MAX_MULTICAST_SESSIONS = 8; //!< Maximum number of multicast sessions to save 00082 const uint8_t EUI_SIZE = 8; //!< Number of bytes in an EUI 00083 const uint8_t KEY_SIZE = 16; //!< Number of bytes in an AES key 00084 00085 const uint8_t DEFAULT_NUM_CHANNELS = 16; //!< Default number of channels in a plan 00086 const uint8_t DEFAULT_RX1_DR_OFFSET = 0; //!< Default datarate offset for first rx window 00087 const uint8_t DEFAULT_RX2_DATARATE = 0; //!< Default datarate for second rx window 00088 const uint8_t DEFAULT_TX_POWER = 14; //!< Default transmit power 00089 const uint8_t DEFAULT_CODE_RATE = 1; //!< Default coding rate 1:4/5, 2:4/6, 3:4/7, 4:4/8 00090 const uint8_t DEFAULT_PREAMBLE_LEN = 8; //!< Default preamble length 00091 00092 const int32_t MAX_FCNT_GAP = 16384; //!< Maximum allowed gap in sequence numbers before roll-over 00093 00094 const uint16_t PRIVATE_JOIN_DELAY = 1000; //!< Default join delay used for private network 00095 const uint16_t PUBLIC_JOIN_DELAY = 5000; //!< Default join delay used for public network 00096 const uint16_t DEFAULT_JOIN_DELAY = PRIVATE_JOIN_DELAY; //!< Default join delay1 00097 const uint16_t DEFAULT_RX_DELAY = 1000; //!< Default delay for first receive window 00098 const uint16_t DEFAULT_RX_TIMEOUT = 3000; //!< Default timeout for receive windows 00099 00100 const uint8_t HI_DR_SYMBOL_TIMEOUT = 12; //!< Symbol timeout for receive at datarate with SF < 11 00101 const uint8_t LO_DR_SYMBOL_TIMEOUT = 8; //!< Symbol timeout for receive at datarate with SF > 10 00102 00103 const uint16_t RX2_DELAY_OFFSET = 1000; //!< Delay between first and second window 00104 const uint16_t RXC_OFFSET = 50; //!< Time between end of RXC after TX and RX1 00105 00106 const uint16_t BEACON_PREAMBLE_LENGTH = 10U; //!< Beacon preamble length 00107 const uint16_t DEFAULT_BEACON_PERIOD = 128U; //!< Default period of the beacon (in seconds) 00108 const uint16_t PING_SLOT_LENGTH = 30U; //!< Duration of each class B ping slot (in milliseconds) 00109 const uint16_t BEACON_RESERVED_TIME = 2120U; //!< Time reserved for beacon broadcast (in milliseconds) 00110 const uint16_t BEACON_GUARD_TIME = 3000U; //!< Guard time before beacon transmission where no ping slots can be scheduled (in milliseconds) 00111 const uint32_t MAX_BEACONLESS_OP_TIME = 7200U; //!< Maximum time to operate in class B since last beacon received (in seconds) 00112 const uint16_t MAX_CLASS_B_WINDOW_GROWTH = 3U; //!< Maximum window growth factor for beacons and ping slots in beacon-less operation 00113 const uint16_t DEFAULT_PING_NB = 1U; //!< Default number of ping slots per beacon interval 00114 const uint16_t CLS_B_PAD = 15U; //!< Pad added to the beginning of ping slot rx windows (in milliseconds) 00115 00116 const int16_t DEFAULT_FREE_CHAN_RSSI_THRESHOLD = -90; //!< Threshold for channel activity detection (CAD) dBm 00117 00118 const uint8_t CHAN_MASK_SIZE = 16; //!< Number of bits in a channel mask 00119 const uint8_t COMMANDS_BUFFER_SIZE = 15; //!< Size of Mac Command buffer 00120 00121 const uint8_t PKT_HEADER = 0; //!< Index to packet mHdr field 00122 const uint8_t PKT_ADDRESS = 1; //!< Index to first byte of packet address field 00123 const uint8_t PKT_FRAME_CONTROL = PKT_ADDRESS + 4; //!< Index to packet fCtrl field @see UplinkControl 00124 const uint8_t PKT_FRAME_COUNTER = PKT_FRAME_CONTROL + 1; //!< Index to packet frame counter field 00125 const uint8_t PKT_OPTIONS_START = PKT_FRAME_COUNTER + 2; //!< Index to start of optional mac commands 00126 00127 const uint8_t PKT_JOIN_APP_NONCE = 1; //!< Index to application nonce in Join Accept message 00128 const uint8_t PKT_JOIN_NETWORK_ID = 4; //!< Index to network id in Join Accept message 00129 const uint8_t PKT_JOIN_NETWORK_ADDRESS = 7; //!< Index to network address in Join Accept message 00130 const uint8_t PKT_JOIN_DL_SETTINGS = 11; //!< Index to downlink settings in Join Accept message 00131 const uint8_t PKT_JOIN_RX_DELAY = 12; //!< Index to rx delay in Join Accept message 00132 00133 const uint8_t DEFAULT_ADR_ACK_LIMIT = 64; //!< Number of packets without ADR ACK Request 00134 const uint8_t DEFAULT_ADR_ACK_DELAY = 32; //!< Number of packets to expect ADR ACK Response within 00135 00136 const uint16_t ACK_TIMEOUT = 2000; //!< Base millisecond timeout to resend after missed ACK 00137 const uint16_t ACK_TIMEOUT_RND = 1000; //!< Random millisecond adjustment to resend after missed ACK 00138 00139 const uint8_t FRAME_OVERHEAD = 13; //!< Bytes of network info overhead in a frame 00140 00141 const uint16_t MAX_OFF_AIR_WAIT = 5000U; //!< Max time in ms to block for a duty cycle restriction to expire before erroring out 00142 /** 00143 * Settings for type of network 00144 * 00145 * PRIVATE_MTS - Sync Word 0x12, US/AU Downlink frequencies per Frequency Sub Band 00146 * PUBLIC_LORAWAN - Sync Word 0x34 00147 * PRIVATE_LORAWAN - Sync Word 0x12 00148 * PEER_TO_PEER - Sync Word 0x56 used for Dot to Dot communication 00149 * 00150 * Join Delay window settings are independent of Network Type setting 00151 */ 00152 enum NetworkType { 00153 PRIVATE_MTS = 0, 00154 PUBLIC_LORAWAN = 1, 00155 PRIVATE_LORAWAN = 2, 00156 PEER_TO_PEER = 4 00157 }; 00158 00159 /** 00160 * Enum for on/off settings 00161 */ 00162 enum Enabled { 00163 OFF = 0, 00164 ON = 1 00165 }; 00166 00167 /** 00168 * Return status of mac functions 00169 */ 00170 enum MacStatus { 00171 LORA_OK = 0, 00172 LORA_ERROR = 1, 00173 LORA_JOIN_ERROR = 2, 00174 LORA_SEND_ERROR = 3, 00175 LORA_MIC_ERROR = 4, 00176 LORA_ADDRESS_ERROR = 5, 00177 LORA_NO_CHANS_ENABLED = 6, 00178 LORA_COMMAND_BUFFER_FULL = 7, 00179 LORA_UNKNOWN_MAC_COMMAND = 8, 00180 LORA_ADR_OFF = 9, 00181 LORA_BUSY = 10, 00182 LORA_LINK_BUSY = 11, 00183 LORA_RADIO_BUSY = 12, 00184 LORA_BUFFER_FULL = 13, 00185 LORA_JOIN_BACKOFF = 14, 00186 LORA_NO_FREE_CHAN = 15, 00187 LORA_AGGREGATED_DUTY_CYCLE = 16, 00188 LORA_MAC_COMMAND_ERROR = 17, 00189 LORA_MAX_PAYLOAD_EXCEEDED = 18, 00190 LORA_LBT_CHANNEL_BUSY = 19 00191 }; 00192 00193 /** 00194 * State for Link 00195 */ 00196 enum LinkState { 00197 LINK_IDLE = 0, //!< Link ready to send or receive 00198 LINK_TX, //!< Link is busy sending 00199 LINK_ACK_TX, //!< Link is busy resending after missed ACK 00200 LINK_REP_TX, //!< Link is busy repeating 00201 LINK_RX, //!< Link has receive window open 00202 LINK_RX1, //!< Link has first received window open 00203 LINK_RX2, //!< Link has second received window open 00204 LINK_RXC, //!< Link has class C received window open 00205 LINK_RX_BEACON, //!< Link has a beacon receive window open 00206 LINK_RX_PING, //!< Link has a ping slot receive window open 00207 LINK_P2P //!< Link is busy sending 00208 }; 00209 00210 /** 00211 * State for MAC 00212 */ 00213 enum MacState { 00214 MAC_IDLE, 00215 MAC_RX1, 00216 MAC_RX2, 00217 MAC_RXC, 00218 MAC_TX, 00219 MAC_JOIN 00220 }; 00221 00222 /** 00223 * Operation class for device 00224 */ 00225 enum MoteClass { 00226 CLASS_A = 0x00, //!< Device can only receive in windows opened after a transmit 00227 CLASS_B = 0x01, //!< Device can receive in windows sychronized with gateway beacon 00228 CLASS_C = 0x02 //!< Device can receive any time when not transmitting 00229 }; 00230 00231 /** 00232 * Direction of a packet 00233 */ 00234 enum Direction { 00235 DIR_UP = 0, //!< Packet is sent from mote to gateway 00236 DIR_DOWN = 1, //!< Packet was received from gateway 00237 DIR_PEER = 2 //!< Packet was received from peer 00238 }; 00239 00240 00241 /** 00242 * Receive window used by Link 00243 */ 00244 enum ReceiveWindows { 00245 RX_1 = 1, //!< First receive window 00246 RX_2, //!< Second receive window 00247 RX_SLOT, //!< Ping slot receive window 00248 RX_BEACON, //!< Beacon receive window 00249 RXC, //!< Class C continuous window 00250 RX_TEST 00251 }; 00252 00253 /** 00254 * Beacon info descriptors for the GwSpecific Info field 00255 */ 00256 enum BeaconInfoDesc { 00257 GPS_FIRST_ANTENNA = 0, //!< GPS coordinates of the gateway's first antenna 00258 GPS_SECOND_ANTENNA, //!< GPS coordinates of the gateway's second antenna 00259 GPS_THIRD_ANTENNA, //!< GPS coordinates of the gateway's third antenna 00260 }; 00261 00262 /** 00263 * Datarate range for a Channel 00264 */ 00265 typedef union { 00266 int8_t Value; 00267 struct { 00268 int8_t Min :4; 00269 int8_t Max :4; 00270 } Fields; 00271 } DatarateRange; 00272 00273 /** 00274 * Datarate used for transmitting and receiving 00275 */ 00276 typedef struct Datarate { 00277 uint8_t Index; 00278 uint8_t Bandwidth; 00279 uint8_t Coderate; 00280 uint8_t PreambleLength; 00281 uint8_t SpreadingFactor; 00282 uint8_t Crc; 00283 uint8_t TxIQ; 00284 uint8_t RxIQ; 00285 uint8_t SymbolTimeout(uint16_t pad_ms = 0); 00286 float Timeout(); 00287 Datarate(); 00288 } Datarate; 00289 00290 /** 00291 * Channel used for transmitting 00292 */ 00293 typedef struct { 00294 uint8_t Index; 00295 uint32_t Frequency; 00296 DatarateRange DrRange; 00297 } Channel; 00298 00299 /** 00300 * Receive window 00301 */ 00302 typedef struct { 00303 uint8_t Index; 00304 uint32_t Frequency; 00305 uint8_t DatarateIndex; 00306 } RxWindow; 00307 00308 /** 00309 * Duty band for limiting time-on-air for regional regulations 00310 */ 00311 typedef struct { 00312 uint8_t Index; 00313 uint32_t FrequencyMin; 00314 uint32_t FrequencyMax; 00315 uint8_t PowerMax; 00316 uint16_t DutyCycle; //!< Multiplier of time on air, 0:100%, 1:50%, 2:33%, 10:10%, 100:1%, 1000,0.1% 00317 uint32_t TimeOffEnd; //!< Timestamp when this band will be available 00318 } DutyBand; 00319 00320 /** 00321 * Beacon data content (w/o CRCs and RFUs) 00322 */ 00323 typedef struct { 00324 uint32_t Time; 00325 uint8_t InfoDesc; 00326 uint32_t Latitude; 00327 uint32_t Longitude; 00328 } BeaconData_t; 00329 00330 /** 00331 * Device configuration 00332 */ 00333 typedef struct { 00334 uint8_t FrequencyBand; //!< Used to choose ChannelPlan 00335 uint8_t EUI[8]; //!< Unique identifier assigned to device 00336 } DeviceConfig; 00337 00338 /** 00339 * Network configuration 00340 */ 00341 typedef struct { 00342 uint8_t Mode; //!< PUBLIC, PRIVATE or PEER_TO_PEER network mode 00343 uint8_t Class; //!< Operating class of device 00344 uint8_t EUI[8]; //!< Network ID or AppEUI 00345 uint8_t Key[16]; //!< Network Key or AppKey 00346 uint8_t JoinDelay; //!< Number of seconds to wait before 1st RX Window 00347 uint8_t RxDelay; //!< Number of seconds to wait before 1st RX Window 00348 uint8_t FrequencySubBand; //!< FrequencySubBand used for US915 hybrid operation 0:72 channels, 1:1-8 channels ... 00349 uint8_t AckAttempts; //!< Number of attempts to send packet and receive an ACK from server 00350 uint8_t Retries; //!< Number of times to resend a packet without receiving an ACK, redundancy 00351 uint8_t ADREnabled; //!< Enable adaptive datarate 00352 uint16_t AdrAckLimit; //!< Number of uplinks without a downlink to allow before setting ADRACKReq 00353 uint16_t AdrAckDelay; //!< Number of downlinks to expect ADR ACK Response within 00354 uint8_t CADEnabled; //!< Enable listen before talk/channel activity detection 00355 uint8_t RepeaterMode; //!< Limit payloads to repeater compatible sizes 00356 uint8_t TxPower; //!< Default radio output power in dBm 00357 uint8_t TxPowerMax; //!< Max transmit power 00358 uint8_t TxDatarate; //!< Datarate for P2P transmit 00359 uint32_t TxFrequency; //!< Frequency for P2P transmit 00360 int8_t AntennaGain; //!< Antenna Gain 00361 uint8_t DisableEncryption; //!< Disable Encryption 00362 uint8_t DisableCRC; //!< Disable CRC on uplink packets 00363 uint16_t P2PACKTimeout; 00364 uint16_t P2PACKBackoff; 00365 uint8_t JoinRx1DatarateOffset; //!< Offset for datarate for first window 00366 uint32_t JoinRx2Frequency; //!< Frequency used in second window 00367 uint8_t JoinRx2DatarateIndex; //!< Datarate for second window 00368 uint8_t PingPeriodicity; //!< Number of ping slots to open in a beacon interval (2^(7-PingPeriodicity)) 00369 } NetworkConfig; 00370 00371 /** 00372 * Network session info 00373 * Some settings are acquired in join message and others may be changed through Mac Commands from server 00374 */ 00375 typedef struct { 00376 uint8_t Joined; //!< State of session 00377 uint8_t Class; //!< Operating class of device 00378 uint8_t Rx1DatarateOffset; //!< Offset for datarate for first window 00379 uint32_t Rx2Frequency; //!< Frequency used in second window 00380 uint8_t Rx2DatarateIndex; //!< Datarate for second window 00381 uint32_t BeaconFrequency; //!< Frequency used for the beacon window 00382 bool BeaconFreqHop; //!< Beacon frequency hopping enable 00383 uint32_t PingSlotFrequency; //!< Frequency used for ping slot windows 00384 uint8_t PingSlotDatarateIndex; //!< Datarate for the ping slots 00385 bool PingSlotFreqHop; //!< Ping slot frequency hopping enable 00386 uint8_t TxPower; //!< Current total radiated output power in dBm 00387 uint8_t TxDatarate; //!< Current datarate can be changed when ADR is enabled 00388 uint32_t Address; //!< Network address 00389 uint32_t NetworkID; //!< Network ID 24-bits 00390 uint8_t NetworkSessionKey[16]; //!< Network session key 00391 uint8_t ApplicationSessionKey[16]; //!< Data session key 00392 uint16_t ChannelMask[4]; //!< Current channel mask 00393 uint16_t ChannelMask500k; //!< Current channel mask for 500k channels 00394 uint32_t DownlinkCounter; //!< Downlink counter of last packet received from server 00395 uint32_t UplinkCounter; //!< Uplink counter of last packet received from server 00396 uint8_t Redundancy; //!< Number of time to repeat an uplink 00397 uint8_t MaxDutyCycle; //!< Current Max Duty Cycle value 00398 uint32_t JoinTimeOnAir; //!< Balance of time on air used during join attempts 00399 uint32_t JoinTimeOffEnd; //!< RTC time of next join attempt 00400 uint32_t JoinFirstAttempt; //!< RTC time of first failed join attempt 00401 uint32_t AggregatedTimeOffEnd; //!< Time off air expiration for aggregate duty cycle 00402 uint16_t AggregateDutyCycle; //!< Used for enforcing time-on-air 00403 uint8_t AckCounter; //!< Current number of packets sent without ACK from server 00404 uint8_t AdrCounter; //!< Current number of packets received without downlink from server 00405 uint8_t RxDelay; //!< Number of seconds to wait before 1st RX Window 00406 uint8_t CommandBuffer[COMMANDS_BUFFER_SIZE]; //!< Buffer to hold Mac Commands and parameters to be sent in next packet 00407 uint8_t CommandBufferIndex; //!< Index to place next Mac Command, also current size of Command Buffer 00408 bool SrvRequestedAck; //!< Indicator of ACK requested by server in last packet received 00409 bool DataPending; //!< Indicator of data pending at server 00410 uint8_t RxTimingSetupReqReceived; //!< Indicator that RxTimingSetupAns should be included in uplink 00411 uint8_t RxParamSetupReqAnswer; //!< Indicator that RxParamSetupAns should be included in uplink 00412 uint8_t DlChannelReqAnswer; //!< Indicator that DlChannelAns should be included in uplink 00413 uint8_t DownlinkDwelltime; //!< On air dwell time for downlink packets 0:NONE,1:400ms 00414 uint8_t UplinkDwelltime; //!< On air dwell time for uplink packets 0:NONE,1:400ms 00415 uint8_t Max_EIRP; //!< Maximum allowed EIRP for uplink 00416 } NetworkSession; 00417 00418 /** 00419 * Multicast session info 00420 */ 00421 typedef struct { 00422 uint32_t Address; //!< Network address 00423 uint8_t NetworkSessionKey[16]; //!< Network session key 00424 uint8_t DataSessionKey[16]; //!< Data session key 00425 uint32_t DownlinkCounter; //!< Downlink counter of last packet received from server 00426 } MulticastSession; 00427 00428 /** 00429 * Statistics of current network session 00430 */ 00431 typedef struct Statistics { 00432 uint32_t Up; //!< Number of uplink packets sent 00433 uint32_t Down; //!< Number of downlink packets received 00434 uint32_t Joins; //!< Number of join requests sent 00435 uint32_t JoinFails; //!< Number of join requests without response or invalid response 00436 uint32_t MissedAcks; //!< Number of missed acknowledgement attempts of confirmed packets 00437 uint32_t CRCErrors; //!< Number of CRC errors in received packets 00438 int32_t AvgCount; //!< Number of packets used to compute rolling average of RSSI and SNR 00439 int16_t Rssi; //!< RSSI of last packet received 00440 int16_t RssiMin; //!< Minimum RSSI of last AvgCount packets 00441 int16_t RssiMax; //!< Maximum RSSI of last AvgCount packets 00442 int16_t RssiAvg; //!< Rolling average RSSI of last AvgCount packets 00443 int16_t Snr; //!< SNR of last packet received 00444 int16_t SnrMin; //!< Minimum SNR of last AvgCount packets 00445 int16_t SnrMax; //!< Maximum SNR of last AvgCount packets 00446 int16_t SnrAvg; //!< Rolling average SNR of last AvgCount packets 00447 } Statistics; 00448 00449 /** 00450 * Testing settings 00451 */ 00452 typedef struct { 00453 uint8_t TestMode; 00454 uint8_t SkipMICCheck; 00455 uint8_t DisableDutyCycle; 00456 uint8_t DisableRx1; 00457 uint8_t DisableRx2; 00458 uint8_t FixedUplinkCounter; 00459 uint8_t DisableRandomJoinDatarate; 00460 } Testing; 00461 00462 /** 00463 * Combination of device, network, testing settings and statistics 00464 */ 00465 typedef struct { 00466 DeviceConfig Device; 00467 NetworkConfig Network; 00468 NetworkSession Session; 00469 MulticastSession Multicast[MAX_MULTICAST_SESSIONS]; 00470 Statistics Stats; 00471 Testing Test; 00472 } Settings; 00473 00474 /** 00475 * Downlink settings sent in Join Accept message 00476 */ 00477 typedef union { 00478 uint8_t Value; 00479 struct { 00480 uint8_t Rx2Datarate :4; 00481 uint8_t Rx1Offset :3; 00482 uint8_t RFU :1; 00483 }; 00484 } DownlinkSettings; 00485 00486 /** 00487 * Frame structure for Join Request 00488 */ 00489 typedef struct { 00490 uint8_t Type; 00491 uint8_t AppEUI[8]; 00492 uint8_t DevEUI[8]; 00493 uint8_t Nonce[2]; 00494 uint8_t MIC[4]; 00495 } JoinRequestFrame; 00496 00497 /** 00498 * Mac header of uplink and downlink packets 00499 */ 00500 typedef union { 00501 uint8_t Value; 00502 struct { 00503 uint8_t Major :2; 00504 uint8_t RFU :3; 00505 uint8_t MType :3; 00506 } Bits; 00507 } MacHeader; 00508 00509 /** 00510 * Frame control field of uplink packets 00511 */ 00512 typedef union { 00513 uint8_t Value; 00514 struct { 00515 uint8_t OptionsLength :4; 00516 uint8_t ClassB :1; 00517 uint8_t Ack :1; 00518 uint8_t AdrAckReq :1; 00519 uint8_t Adr :1; 00520 } Bits; 00521 } UplinkControl; 00522 00523 /** 00524 * Frame control field of downlink packets 00525 */ 00526 typedef union { 00527 uint8_t Value; 00528 struct { 00529 uint8_t OptionsLength :4; 00530 uint8_t FPending :1; 00531 uint8_t Ack :1; 00532 uint8_t RFU :1; 00533 uint8_t Adr :1; 00534 } Bits; 00535 } DownlinkControl; 00536 00537 /** 00538 * Frame type of packet 00539 */ 00540 typedef enum { 00541 FRAME_TYPE_JOIN_REQ = 0x00, 00542 FRAME_TYPE_JOIN_ACCEPT = 0x01, 00543 FRAME_TYPE_DATA_UNCONFIRMED_UP = 0x02, 00544 FRAME_TYPE_DATA_UNCONFIRMED_DOWN = 0x03, 00545 FRAME_TYPE_DATA_CONFIRMED_UP = 0x04, 00546 FRAME_TYPE_DATA_CONFIRMED_DOWN = 0x05, 00547 FRAME_TYPE_RFU = 0x06, 00548 FRAME_TYPE_PROPRIETARY = 0x07, 00549 } FrameType; 00550 00551 /** 00552 * LoRaWAN mote MAC commands 00553 */ 00554 typedef enum { 00555 /* Class A */ 00556 MOTE_MAC_LINK_CHECK_REQ = 0x02, 00557 MOTE_MAC_LINK_ADR_ANS = 0x03, 00558 MOTE_MAC_DUTY_CYCLE_ANS = 0x04, 00559 MOTE_MAC_RX_PARAM_SETUP_ANS = 0x05, 00560 MOTE_MAC_DEV_STATUS_ANS = 0x06, 00561 MOTE_MAC_NEW_CHANNEL_ANS = 0x07, 00562 MOTE_MAC_RX_TIMING_SETUP_ANS = 0x08, 00563 MOTE_MAC_TX_PARAM_SETUP_ANS = 0x09, 00564 MOTE_MAC_DL_CHANNEL_ANS = 0x0A, 00565 MOTE_MAC_REKEY_IND = 0x0B, 00566 MOTE_MAC_ADR_PARAM_SETUP_ANS = 0x0C, 00567 MOTE_MAC_DEVICE_TIME_REQ = 0x0D, 00568 MOTE_MAC_REJOIN_PARAM_SETUP_ANS = 0x0F, 00569 00570 /* Class B */ 00571 MOTE_MAC_PING_SLOT_INFO_REQ = 0x10, 00572 MOTE_MAC_PING_SLOT_CHANNEL_ANS = 0x11, 00573 MOTE_MAC_BEACON_TIMING_REQ = 0x12, 00574 MOTE_MAC_BEACON_FREQ_ANS = 0x13, 00575 00576 /* Multitech */ 00577 MOTE_MAC_PING_REQ = 0x80, 00578 MOTE_MAC_CHANGE_CLASS = 0x81, 00579 MOTE_MAC_MULTIPART_START_REQ = 0x82, 00580 MOTE_MAC_MULTIPART_START_ANS = 0x83, 00581 MOTE_MAC_MULTIPART_CHUNK = 0x84, 00582 MOTE_MAC_MULTIPART_END_REQ = 0x85, 00583 MOTE_MAC_MULTIPART_END_ANS = 0x86 00584 } MoteCommand; 00585 00586 /*! 00587 * LoRaWAN server MAC commands 00588 */ 00589 typedef enum { 00590 /* Class A */ 00591 SRV_MAC_LINK_CHECK_ANS = 0x02, 00592 SRV_MAC_LINK_ADR_REQ = 0x03, 00593 SRV_MAC_DUTY_CYCLE_REQ = 0x04, 00594 SRV_MAC_RX_PARAM_SETUP_REQ = 0x05, 00595 SRV_MAC_DEV_STATUS_REQ = 0x06, 00596 SRV_MAC_NEW_CHANNEL_REQ = 0x07, 00597 SRV_MAC_RX_TIMING_SETUP_REQ = 0x08, 00598 SRV_MAC_TX_PARAM_SETUP_REQ = 0x09, 00599 SRV_MAC_DL_CHANNEL_REQ = 0x0A, 00600 SRV_MAC_REKEY_CONF = 0x0B, 00601 SRV_MAC_ADR_PARAM_SETUP_REQ = 0x0C, 00602 SRV_MAC_DEVICE_TIME_ANS = 0x0D, 00603 SRV_MAC_FORCE_REJOIN_REQ = 0x0E, 00604 SRV_MAC_REJOIN_PARAM_SETUP_REQ = 0x0F, 00605 00606 /* Class B */ 00607 SRV_MAC_PING_SLOT_INFO_ANS = 0x10, 00608 SRV_MAC_PING_SLOT_CHANNEL_REQ = 0x11, 00609 SRV_MAC_BEACON_TIMING_ANS = 0x12, 00610 SRV_MAC_BEACON_FREQ_REQ = 0x13, 00611 00612 /* Multitech */ 00613 SRV_MAC_PING_ANS = 0x80, 00614 SRV_MAC_CHANGE_CLASS = 0x81, 00615 SRV_MAC_MULTIPART_START_REQ = 0x82, 00616 SRV_MAC_MULTIPART_START_ANS = 0x83, 00617 SRV_MAC_MULTIPART_CHUNK = 0x84, 00618 SRV_MAC_MULTIPART_END_REQ = 0x85, 00619 SRV_MAC_MULTIPART_END_ANS = 0x86 00620 } ServerCommand; 00621 00622 /** 00623 * Radio configuration options 00624 */ 00625 typedef enum RadioCfg { 00626 NO_RADIO_CFG, 00627 TX_RADIO_CFG, 00628 RX_RADIO_CFG 00629 } RadioCfg_t; 00630 00631 /** 00632 * Random seed for software RNG 00633 */ 00634 void srand(uint32_t seed); 00635 00636 /** 00637 * Software RNG for consistent results across differing hardware 00638 */ 00639 int rand(void); 00640 00641 /** 00642 * Generate random number bounded by min and max 00643 */ 00644 int32_t rand_r(int32_t min, int32_t max); 00645 00646 uint8_t CountBits(uint16_t mask); 00647 00648 /** 00649 * Copy 3-bytes network order from array into LSB of integer value 00650 */ 00651 void CopyNetIDtoInt(const uint8_t* arr, uint32_t& val); 00652 00653 /** 00654 * Copy LSB 3-bytes from integer value into array network order 00655 */ 00656 void CopyNetIDtoArray(uint32_t val, uint8_t* arr); 00657 00658 /** 00659 * Copy 4-bytes network order from array in to integer value 00660 */ 00661 void CopyAddrtoInt(const uint8_t* arr, uint32_t& val); 00662 00663 /** 00664 * Copy 4-bytes from integer in to array network order 00665 */ 00666 void CopyAddrtoArray(uint32_t val, uint8_t* arr); 00667 00668 /** 00669 * Copy 3-bytes network order from array into integer value and multiply by 100 00670 */ 00671 void CopyFreqtoInt(const uint8_t* arr, uint32_t& freq); 00672 00673 /** 00674 * Reverse memory copy 00675 */ 00676 void memcpy_r(uint8_t *dst, const uint8_t *src, size_t n); 00677 00678 } 00679 00680 #endif 00681
Generated on Wed Jul 13 2022 16:42:53 by
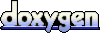