The Modified Dot Library for SX1272
Embed:
(wiki syntax)
Show/hide line numbers
FragmentationSession.h
00001 #ifndef _FRAGMENTATION_SESSION_H 00002 #define _FRAGMENTATION_SESSION_H 00003 #ifdef FOTA 00004 00005 #include "mbed.h" 00006 #include "FragmentationMath.h" 00007 #include "mDot.h" 00008 #include "WriteFile.h" 00009 00010 #define MAX_PARITY 300 00011 #define FRAG_OFFSET 3 00012 #define MULTICAST_SESSIONS 3 00013 00014 class FragmentationSession { 00015 00016 public: 00017 FragmentationSession(mDot* dot, std::vector<uint8_t>* ret, bool* filled, uint32_t* delay); 00018 ~FragmentationSession(); 00019 void processCmd(uint8_t* payload, uint8_t size); 00020 void reset(); 00021 00022 private: 00023 00024 typedef struct { 00025 uint8_t Padding; 00026 uint8_t FragmentSize; 00027 uint16_t NumberOfFragments; 00028 } FragmentationSessionOpts_t; 00029 00030 typedef struct { 00031 bool reset; 00032 bool failed; 00033 uint8_t fragMatrix; 00034 uint8_t blockAckDelay; 00035 uint8_t total_sessions; 00036 uint8_t mcGroupBitMask; 00037 uint8_t current_session; 00038 uint16_t index; 00039 uint16_t last_frag_num; 00040 uint32_t total_frags; 00041 FragmentationMath* math; 00042 FragmentationSessionOpts_t opts; 00043 } fragGroup; 00044 00045 00046 enum FragResult { 00047 FRAG_OK, 00048 FRAG_SIZE_INCORRECT, 00049 FRAG_FLASH_WRITE_ERROR, 00050 FRAG_NO_MEMORY, 00051 FRAG_COMPLETE 00052 }; 00053 00054 enum FragCmd { 00055 PACKAGE_VERSION_FRAG, 00056 FRAG_STATUS, 00057 FRAG_SESSION_SETUP, 00058 FRAG_SESSION_DELETE, 00059 DATA_FRAGMENT = 0x08, 00060 CHECKSUM = 0x80 00061 }; 00062 00063 void reset(uint16_t num); 00064 void upgradeFile(uint8_t fragIndex); 00065 bool process_frame(uint8_t fragIndex,uint16_t index, uint8_t buffer[], size_t size); 00066 00067 mDot* _dot; 00068 WriteFile* _fh; 00069 fragGroup fg[MULTICAST_SESSIONS]; 00070 00071 uint8_t ans; 00072 bool* _filled; 00073 uint32_t* _delay; 00074 uint8_t fragIndex; 00075 std::vector<uint8_t>* _ret; 00076 std::string _org_class; 00077 }; 00078 #endif 00079 #endif // _FRAGMENTATION_SESSION_H
Generated on Wed Jul 13 2022 16:42:53 by
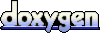