CDC/ECM driver for mbed, based on USBDevice by mbed-official. Uses PicoTCP to access Ethernet USB device. License: GPLv2
Fork of USB_Ethernet by
pico_http_util.c
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 Author: Andrei Carp <andrei.carp@tass.be> 00006 *********************************************************************/ 00007 00008 #include <stdint.h> 00009 #include "pico_config.h" 00010 #include "pico_stack.h" 00011 #include "pico_protocol.h" 00012 #include "pico_http_util.h" 00013 00014 #define TRUE 1 00015 #define FALSE 0 00016 00017 #define HTTP_PROTO_TOK "http://" 00018 #define HTTP_PROTO_LEN 7u 00019 00020 #if defined PICO_SUPPORT_HTTP_CLIENT || defined PICO_SUPPORT_HTTP_SERVER 00021 00022 int pico_itoaHex(uint16_t port, char * ptr) 00023 { 00024 int size = 0; 00025 int index; 00026 00027 // transform to from number to string [ in backwards ] 00028 while(port) 00029 { 00030 ptr[size] = ((port & 0xF) < 10) ? ((port & 0xF) + '0') : ((port & 0xF) - 10 + 'a'); 00031 port = port>>4u; //divide by 16 00032 size++; 00033 } 00034 00035 // invert positions 00036 for(index=0 ;index < size>>1u ;index++) 00037 { 00038 char c = ptr[index]; 00039 ptr[index] = ptr[size-index-1]; 00040 ptr[size-index-1] = c; 00041 } 00042 ptr[size] = '\0'; 00043 return size; 00044 } 00045 00046 int pico_itoa(uint16_t port, char * ptr) 00047 { 00048 int size = 0; 00049 int index; 00050 00051 // transform to from number to string [ in backwards ] 00052 while(port) 00053 { 00054 ptr[size] = port%10 + '0'; 00055 port = port/10; 00056 size++; 00057 } 00058 00059 // invert positions 00060 for(index=0 ;index < size>>1u ;index++) 00061 { 00062 char c = ptr[index]; 00063 ptr[index] = ptr[size-index-1]; 00064 ptr[size-index-1] = c; 00065 } 00066 ptr[size] = '\0'; 00067 return size; 00068 } 00069 00070 00071 int pico_processURI(const char * uri, struct pico_http_uri * urikey) 00072 { 00073 00074 uint16_t lastIndex = 0, index; 00075 00076 if(!uri || !urikey || uri[0] == '/') 00077 { 00078 pico_err = PICO_ERR_EINVAL; 00079 goto error; 00080 } 00081 00082 // detect protocol => search for "://" 00083 if(memcmp(uri,HTTP_PROTO_TOK,HTTP_PROTO_LEN) == 0) // could be optimized 00084 { // protocol identified, it is http 00085 urikey->protoHttp = TRUE; 00086 lastIndex = HTTP_PROTO_LEN; 00087 } 00088 else 00089 { 00090 if(strstr(uri,"://")) // different protocol specified 00091 { 00092 urikey->protoHttp = FALSE; 00093 goto error; 00094 } 00095 // no protocol specified, assuming by default it's http 00096 urikey->protoHttp = TRUE; 00097 } 00098 00099 // detect hostname 00100 index = lastIndex; 00101 while(uri[index] && uri[index]!='/' && uri[index]!=':') index++; 00102 00103 if(index == lastIndex) 00104 { 00105 // wrong format 00106 urikey->host = urikey->resource = NULL; 00107 urikey->port = urikey->protoHttp = 0u; 00108 00109 goto error; 00110 } 00111 else 00112 { 00113 // extract host 00114 urikey->host = (char *)pico_zalloc(index-lastIndex+1); 00115 00116 if(!urikey->host) 00117 { 00118 // no memory 00119 goto error; 00120 } 00121 memcpy(urikey->host,uri+lastIndex,index-lastIndex); 00122 } 00123 00124 if(!uri[index]) 00125 { 00126 // nothing specified 00127 urikey->port = 80u; 00128 urikey->resource = pico_zalloc(2u); 00129 urikey->resource[0] = '/'; 00130 return HTTP_RETURN_OK; 00131 } 00132 else if(uri[index] == '/') 00133 { 00134 urikey->port = 80u; 00135 } 00136 else if(uri[index] == ':') 00137 { 00138 urikey->port = 0u; 00139 index++; 00140 while(uri[index] && uri[index]!='/') 00141 { 00142 // should check if every component is a digit 00143 urikey->port = urikey->port*10 + (uri[index] - '0'); 00144 index++; 00145 } 00146 } 00147 00148 // extract resource 00149 if(!uri[index]) 00150 { 00151 urikey->resource = pico_zalloc(2u); 00152 urikey->resource[0] = '/'; 00153 } 00154 else 00155 { 00156 lastIndex = index; 00157 while(uri[index] && uri[index]!='?' && uri[index]!='&' && uri[index]!='#') index++; 00158 urikey->resource = (char *)pico_zalloc(index-lastIndex+1); 00159 00160 if(!urikey->resource) 00161 { 00162 // no memory 00163 pico_err = PICO_ERR_ENOMEM; 00164 goto error; 00165 } 00166 00167 memcpy(urikey->resource,uri+lastIndex,index-lastIndex); 00168 } 00169 00170 return HTTP_RETURN_OK; 00171 00172 error : 00173 if(urikey->resource) 00174 { 00175 pico_free(urikey->resource); 00176 urikey->resource = NULL; 00177 } 00178 if(urikey->host) 00179 { 00180 pico_free(urikey->host); 00181 urikey->host = NULL; 00182 } 00183 00184 return HTTP_RETURN_ERROR; 00185 } 00186 #endif
Generated on Wed Jul 13 2022 02:20:45 by
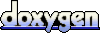