CDC/ECM driver for mbed, based on USBDevice by mbed-official. Uses PicoTCP to access Ethernet USB device. License: GPLv2
Fork of USB_Ethernet by
pico_http_client.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 Author: Andrei Carp <andrei.carp@tass.be> 00006 *********************************************************************/ 00007 00008 00009 #ifndef PICO_HTTP_CLIENT_H_ 00010 #define PICO_HTTP_CLIENT_H_ 00011 00012 #include "pico_http_util.h" 00013 00014 /* 00015 * Transfer encodings 00016 */ 00017 #define HTTP_TRANSFER_CHUNKED 1u 00018 #define HTTP_TRANSFER_FULL 0u 00019 00020 /* 00021 * Parameters for the send header function 00022 */ 00023 #define HTTP_HEADER_RAW 0u 00024 #define HTTP_HEADER_DEFAULT 1u 00025 00026 /* 00027 * Data types 00028 */ 00029 00030 struct pico_http_header 00031 { 00032 uint16_t responseCode; // http response 00033 char * location; // if redirect is reported 00034 uint32_t contentLengthOrChunk; // size of the message 00035 uint8_t transferCoding; // chunked or full 00036 00037 }; 00038 00039 int pico_http_client_open(char * uri, void (*wakeup)(uint16_t ev, uint16_t conn)); 00040 int pico_http_client_sendHeader(uint16_t conn, char * header, int hdr); 00041 00042 struct pico_http_header * pico_http_client_readHeader(uint16_t conn); 00043 struct pico_http_uri * pico_http_client_readUriData(uint16_t conn); 00044 char * pico_http_client_buildHeader(const struct pico_http_uri * uriData); 00045 00046 int pico_http_client_readData(uint16_t conn, char * data, uint16_t size); 00047 int pico_http_client_close(uint16_t conn); 00048 00049 #endif /* PICO_HTTP_CLIENT_H_ */
Generated on Wed Jul 13 2022 02:20:45 by
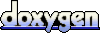