CDC/ECM driver for mbed, based on USBDevice by mbed-official. Uses PicoTCP to access Ethernet USB device. License: GPLv2
Fork of USB_Ethernet by
pico_frame.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 *********************************************************************/ 00006 #ifndef _INCLUDE_PICO_FRAME 00007 #define _INCLUDE_PICO_FRAME 00008 #include "pico_config.h" 00009 00010 00011 #define PICO_FRAME_FLAG_BCAST (0x01) 00012 #define PICO_FRAME_FLAG_SACKED (0x80) 00013 #define IS_BCAST(f) ((f->flags & PICO_FRAME_FLAG_BCAST) == PICO_FRAME_FLAG_BCAST) 00014 00015 00016 struct pico_socket; 00017 00018 00019 struct pico_frame { 00020 00021 /* Connector for queues */ 00022 struct pico_frame *next; 00023 00024 /* Start of the whole buffer, total frame length. */ 00025 unsigned char *buffer; 00026 uint32_t buffer_len; 00027 00028 /* For outgoing packets: this is the meaningful buffer. */ 00029 unsigned char *start; 00030 uint32_t len; 00031 00032 /* Pointer to usage counter */ 00033 uint32_t *usage_count; 00034 00035 /* Pointer to protocol headers */ 00036 uint8_t *datalink_hdr; 00037 00038 uint8_t *net_hdr; 00039 int net_len; 00040 uint8_t *transport_hdr; 00041 int transport_len; 00042 uint8_t *app_hdr; 00043 int app_len; 00044 00045 /* Pointer to the phisical device this packet belongs to. 00046 * Should be valid in both routing directions 00047 */ 00048 struct pico_device *dev; 00049 00050 unsigned long timestamp; 00051 00052 /* Failures due to bad datalink addressing. */ 00053 uint16_t failure_count; 00054 00055 /* Protocol over IP */ 00056 uint8_t proto; 00057 00058 /* PICO_FRAME_FLAG_* */ 00059 uint8_t flags; 00060 00061 /* Pointer to payload */ 00062 unsigned char *payload; 00063 int payload_len; 00064 00065 #ifdef PICO_SUPPORT_IPFRAG 00066 /* Payload fragmentation info (big endian)*/ 00067 uint16_t frag; 00068 #endif 00069 00070 /* Pointer to socket */ 00071 struct pico_socket *sock; 00072 00073 /* Pointer to transport info, used to store remote UDP duple (IP + port) */ 00074 void *info; 00075 00076 /*Priority. "best-effort" priority, the default value is 0. Priority can be in between -10 and +10*/ 00077 int8_t priority; 00078 }; 00079 00080 /** frame alloc/dealloc/copy **/ 00081 void pico_frame_discard(struct pico_frame *f); 00082 struct pico_frame *pico_frame_copy(struct pico_frame *f); 00083 struct pico_frame *pico_frame_deepcopy(struct pico_frame *f); 00084 struct pico_frame *pico_frame_alloc(int size); 00085 uint16_t pico_checksum(void *inbuf, int len); 00086 uint16_t pico_dualbuffer_checksum(void *b1, int len1, void *b2, int len2); 00087 00088 static inline int pico_is_digit(char c) 00089 { 00090 if (c < '0' || c > '9') 00091 return 0; 00092 return 1; 00093 } 00094 00095 #endif
Generated on Wed Jul 13 2022 02:20:45 by
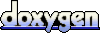