CDC/ECM driver for mbed, based on USBDevice by mbed-official. Uses PicoTCP to access Ethernet USB device. License: GPLv2
Fork of USB_Ethernet by
pico_constants.h
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 00005 *********************************************************************/ 00006 #ifndef _INCLUDE_PICO_CONST 00007 #define _INCLUDE_PICO_CONST 00008 /* Included from pico_config.h */ 00009 /** Endian-dependant constants **/ 00010 00011 extern volatile unsigned long pico_tick; 00012 00013 #ifdef PICO_BIGENDIAN 00014 00015 # define PICO_IDETH_IPV4 0x0800 00016 # define PICO_IDETH_ARP 0x0806 00017 # define PICO_IDETH_IPV6 0x86DD 00018 00019 # define PICO_ARP_REQUEST 0x0001 00020 # define PICO_ARP_REPLY 0x0002 00021 # define PICO_ARP_HTYPE_ETH 0x0001 00022 00023 #define short_be(x) (x) 00024 #define long_be(x) (x) 00025 00026 static inline uint16_t short_from(void *_p) 00027 { 00028 unsigned char *p = (unsigned char *)_p; 00029 uint16_t r, p0, p1; 00030 p0 = p[0]; 00031 p1 = p[1]; 00032 r = (p0 << 8) + p1; 00033 return r; 00034 } 00035 00036 static inline uint32_t long_from(void *_p) 00037 { 00038 unsigned char *p = (unsigned char *)_p; 00039 uint32_t r, p0, p1, p2, p3; 00040 p0 = p[0]; 00041 p1 = p[1]; 00042 p2 = p[2]; 00043 p3 = p[3]; 00044 r = (p0 << 24) + (p1 << 16) + (p2 << 8) + p3; 00045 return r; 00046 } 00047 00048 #else 00049 00050 static inline uint16_t short_from(void *_p) 00051 { 00052 unsigned char *p = (unsigned char *)_p; 00053 uint16_t r, p0, p1; 00054 p0 = p[0]; 00055 p1 = p[1]; 00056 r = (p1 << 8) + p0; 00057 return r; 00058 } 00059 00060 static inline uint32_t long_from(void *_p) 00061 { 00062 unsigned char *p = (unsigned char *)_p; 00063 uint32_t r, p0, p1, p2, p3; 00064 p0 = p[0]; 00065 p1 = p[1]; 00066 p2 = p[2]; 00067 p3 = p[3]; 00068 r = (p3 << 24) + (p2 << 16) + (p1 << 8) + p0; 00069 return r; 00070 } 00071 00072 00073 # define PICO_IDETH_IPV4 0x0008 00074 # define PICO_IDETH_ARP 0x0608 00075 # define PICO_IDETH_IPV6 0xDD86 00076 00077 # define PICO_ARP_REQUEST 0x0100 00078 # define PICO_ARP_REPLY 0x0200 00079 # define PICO_ARP_HTYPE_ETH 0x0100 00080 00081 static inline uint16_t short_be(uint16_t le) 00082 { 00083 return ((le & 0xFF) << 8) | ((le >> 8) & 0xFF); 00084 } 00085 00086 static inline uint32_t long_be(uint32_t le) 00087 { 00088 uint8_t *b = (uint8_t *)≤ 00089 uint32_t be = 0; 00090 uint32_t b0, b1, b2; 00091 b0 = b[0]; 00092 b1 = b[1]; 00093 b2 = b[2]; 00094 be = b[3] + (b2 << 8) + (b1 << 16) + (b0 << 24); 00095 return be; 00096 } 00097 #endif 00098 00099 00100 /* Add well-known host numbers here. (bigendian constants only beyond this point) */ 00101 #define PICO_IP4_ANY (0x00000000U) 00102 #define PICO_IP4_BCAST (0xffffffffU) 00103 00104 /* defined in modules/pico_ipv6.c */ 00105 #ifdef PICO_SUPPORT_IPV6 00106 extern const uint8_t PICO_IPV6_ANY[PICO_SIZE_IP6]; 00107 #endif 00108 00109 static inline uint32_t pico_hash(char *name) 00110 { 00111 unsigned long hash = 5381; 00112 int c; 00113 while ((c = *name++)) 00114 hash = ((hash << 5) + hash) + c; /* hash * 33 + c */ 00115 return hash; 00116 } 00117 00118 /* Debug */ 00119 //#define PICO_SUPPORT_DEBUG_MEMORY 00120 //#define PICO_SUPPORT_DEBUG_TOOLS 00121 #endif
Generated on Wed Jul 13 2022 02:20:45 by
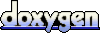