CDC/ECM driver for mbed, based on USBDevice by mbed-official. Uses PicoTCP to access Ethernet USB device. License: GPLv2
Fork of USB_Ethernet by
USBCDC_ECM.cpp
00001 /********************************************************************* 00002 PicoTCP. Copyright (c) 2012 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. 00004 Do not redistribute without a written permission by the Copyright 00005 holders. 00006 00007 Authors: Daniele Lacamera, Julien Duraj 00008 *********************************************************************/ 00009 #include "stdint.h" 00010 #include "USBCDC_ECM.h" 00011 #define DEFAULT_CONFIGURATION (1) 00012 #define MAX_CDC_REPORT_SIZE MAX_PACKET_SIZE_EPBULK 00013 00014 USBCDC_ECM::USBCDC_ECM(uint16_t vendor_id, uint16_t product_id, uint16_t product_release): USBDevice(vendor_id, product_id, product_release) { 00015 terminal_connected = false; 00016 USBDevice::connect(); 00017 } 00018 00019 bool USBCDC_ECM::USBCallback_request(void) { 00020 /* Called in ISR context */ 00021 00022 bool success = false; 00023 CONTROL_TRANSFER * transfer = getTransferPtr(); 00024 00025 if (transfer->setup.bmRequestType.Type == STANDARD_TYPE) { 00026 printf("In USBCallback_request: GENERIC Request: %02x\n", transfer->setup.bRequest); 00027 } else if (transfer->setup.bmRequestType.Type == CLASS_TYPE) { 00028 printf("In USBCallback_request: CLASS specific Request: %02x\n", transfer->setup.bRequest); 00029 switch (transfer->setup.bRequest) { 00030 default: 00031 break; 00032 } 00033 } 00034 00035 return success; 00036 } 00037 00038 bool USBCDC_ECM::USBCallback_setInterface(uint16_t interface, uint8_t alternate) 00039 { 00040 printf("Host selected interface %d, alternate %d\n", interface, alternate); 00041 return true; 00042 } 00043 00044 00045 // Called in ISR context 00046 // Set configuration. Return false if the 00047 // configuration is not supported. 00048 bool USBCDC_ECM::USBCallback_setConfiguration(uint8_t configuration) { 00049 printf("In USBCallback_SetConfiguration: %02x\n", configuration); 00050 if (configuration != DEFAULT_CONFIGURATION) { 00051 printf("Set config: failed\n"); 00052 return false; 00053 } 00054 00055 // Configure endpoints > 0 00056 addEndpoint(EPINT_IN, MAX_PACKET_SIZE_EPINT); 00057 addEndpoint(EPBULK_IN, MAX_PACKET_SIZE_EPBULK); 00058 addEndpoint(EPBULK_OUT, MAX_PACKET_SIZE_EPBULK); 00059 00060 // We activate the endpoint to be able to recceive data 00061 readStart(EPBULK_OUT, MAX_PACKET_SIZE_EPBULK); 00062 printf("Set config: OK!\n"); 00063 00064 return true; 00065 } 00066 00067 bool USBCDC_ECM::send(uint8_t * buffer, uint32_t size) { 00068 if (!configured()) 00069 return false; 00070 return USBDevice::write(EPBULK_IN, buffer, size, MAX_CDC_REPORT_SIZE); 00071 } 00072 00073 bool USBCDC_ECM::readEP(uint8_t * buffer, uint32_t * size) { 00074 if (!configured()) 00075 return false; 00076 if (!USBDevice::readEP(EPBULK_OUT, buffer, size, MAX_CDC_REPORT_SIZE)) 00077 return false; 00078 if (!readStart(EPBULK_OUT, MAX_CDC_REPORT_SIZE)) 00079 return false; 00080 return true; 00081 } 00082 00083 bool USBCDC_ECM::readEP_NB(uint8_t * buffer, uint32_t * size) { 00084 if (!configured()) 00085 return false; 00086 if (!USBDevice::readEP_NB(EPBULK_OUT, buffer, size, MAX_CDC_REPORT_SIZE)) 00087 return false; 00088 if (!readStart(EPBULK_OUT, MAX_CDC_REPORT_SIZE)) 00089 return false; 00090 return true; 00091 } 00092 00093 00094 uint8_t * USBCDC_ECM::deviceDesc() { 00095 static uint8_t deviceDescriptor[] = { 00096 18, // bLength 00097 1, // bDescriptorType 00098 0x10, 0x01, // bcdUSB 00099 2, // bDeviceClass 00100 0, // bDeviceSubClass 00101 0, // bDeviceProtocol 00102 MAX_PACKET_SIZE_EP0, // bMaxPacketSize0 00103 LSB(VENDOR_ID), MSB(VENDOR_ID), // idVendor 00104 LSB(PRODUCT_ID), MSB(PRODUCT_ID),// idProduct 00105 0x00, 0x01, // bcdDevice 00106 1, // iManufacturer 00107 2, // iProduct 00108 3, // iSerialNumber 00109 4, // iMacAddress 00110 1 // bNumConfigurations 00111 }; 00112 return deviceDescriptor; 00113 } 00114 00115 uint8_t * USBCDC_ECM::stringIinterfaceDesc() { 00116 static uint8_t stringIinterfaceDescriptor[] = { 00117 0x08, 00118 STRING_DESCRIPTOR, 00119 'C',0,'D',0,'C',0, 00120 }; 00121 return stringIinterfaceDescriptor; 00122 } 00123 00124 uint8_t * USBCDC_ECM::stringIproductDesc() { 00125 static uint8_t stringIproductDescriptor[] = { 00126 36, 00127 STRING_DESCRIPTOR, 00128 'M',0,'B',0,'E',0,'D',0,' ',0,'C',0,'D',0,'C',0,'-',0,'E',0,'T',0,'H',0,'E',0,'R',0,'N',0,'E',0,'T',0 00129 }; 00130 return stringIproductDescriptor; 00131 } 00132 00133 uint8_t * USBCDC_ECM::stringIserialDesc() { 00134 static uint8_t stringIserialDescriptor[] = { 00135 26, 00136 STRING_DESCRIPTOR, 00137 '0',0,'0',0, 00138 '5', 0,'a',0, 00139 'f',0,'3',0, 00140 '4',0,'1',0, 00141 'b',0,'4',0, 00142 'c',0,'7',0 00143 }; 00144 return stringIserialDescriptor; 00145 } 00146 00147 uint8_t * USBCDC_ECM::stringIConfigurationDesc() { 00148 static uint8_t stringImacAddr[] = { 00149 26, 00150 STRING_DESCRIPTOR, 00151 '0',0,'0',0, 00152 '5', 0,'a',0, 00153 'f',0,'3',0, 00154 '4',0,'1',0, 00155 'b',0,'4',0, 00156 'c',0,'7',0 00157 }; 00158 return stringImacAddr; 00159 } 00160 00161 #define CONFIG1_DESC_SIZE (9+9+5+5+13+7+9+7+7) 00162 00163 uint8_t * USBCDC_ECM::configurationDesc() { 00164 static uint8_t configDescriptor[] = { 00165 // configuration descriptor 00166 9, // bLength 00167 2, // bDescriptorType 00168 LSB(CONFIG1_DESC_SIZE), // wTotalLength 00169 MSB(CONFIG1_DESC_SIZE), 00170 2, // bNumInterfaces 00171 1, // bConfigurationValue 00172 0, // iConfiguration 00173 0xc0, // bmAttributes 00174 50, // bMaxPower 00175 00176 // interface descriptor, USB spec 9.6.5, page 267-269, Table 9-12 00177 9, // bLength 00178 4, // bDescriptorType 00179 0, // bInterfaceNumber 00180 0, // bAlternateSetting 00181 1, // bNumEndpoints 00182 0x02, // bInterfaceClass 00183 0x06, // bInterfaceSubClass 00184 0x00, // bInterfaceProtocol 00185 0, // iInterface 00186 00187 // CDC Header Functional Descriptor, CDC Spec 5.2.3.1, Table 26 00188 5, // bFunctionLength 00189 0x24, // bDescriptorType 00190 0x00, // bDescriptorSubtype 00191 0x20, 0x01, // bcdCDC 00192 00193 // CDC Union 00194 5, // bFunctionLength 00195 0x24, // bDescriptorType 00196 0x06, // bDescriptorSubType 00197 0, // bControlInterface 00198 1, // bSubordinateInterface0 00199 00200 00201 00202 // CDC/ECM Functional Descriptor 00203 13, // bFunctionLenght 00204 0x24, // bDescriptorType 00205 0x0F, // bDescriptorSubtype 00206 4, // iMacAddress 00207 0, 0, 0, 0, // bmEthernetStatistics 00208 00209 0x05, 0xEA, // wMaxSegmentSize 00210 00211 00212 0, 0, // wNumberMCFilters 00213 00214 0, // bNumberPowerFilters 00215 00216 // endpoint descriptor, USB spec 9.6.6, page 269-271, Table 9-13 00217 ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00218 ENDPOINT_DESCRIPTOR, // bDescriptorType 00219 PHY_TO_DESC(EPINT_IN), // bEndpointAddress 00220 0x03 , // bmAttributes (0x03=intr) 00221 LSB(MAX_PACKET_SIZE_EPINT), // wMaxPacketSize (LSB) 00222 MSB(MAX_PACKET_SIZE_EPINT), // wMaxPacketSize (MSB) 00223 16, 00224 00225 // interface descriptor, USB spec 9.6.5, page 267-269, Table 9-12 00226 9, // bLength 00227 4, // bDescriptorType 00228 1, // bInterfaceNumber 00229 0, // bAlternateSetting 00230 2, // bNumEndpoints 00231 0x0A, // bInterfaceClass 00232 0x00, // bInterfaceSubClass 00233 0x00, // bInterfaceProtocol 00234 0, // iInterface 00235 00236 // endpoint descriptor, USB spec 9.6.6, page 269-271, Table 9-13 00237 ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00238 ENDPOINT_DESCRIPTOR, // bDescriptorType 00239 PHY_TO_DESC(EPBULK_IN), // bEndpointAddress 00240 E_BULK, // bmAttributes (0x02=bulk) 00241 LSB(MAX_PACKET_SIZE_EPBULK),// wMaxPacketSize (LSB) 00242 MSB(MAX_PACKET_SIZE_EPBULK),// wMaxPacketSize (MSB) 00243 0, // bInterval 00244 00245 // endpoint descriptor, USB spec 9.6.6, page 269-271, Table 9-13 00246 ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00247 ENDPOINT_DESCRIPTOR, // bDescriptorType 00248 PHY_TO_DESC(EPBULK_OUT), // bEndpointAddress 00249 E_BULK, // bmAttributes (0x02=bulk) 00250 LSB(MAX_PACKET_SIZE_EPBULK),// wMaxPacketSize (LSB) 00251 MSB(MAX_PACKET_SIZE_EPBULK),// wMaxPacketSize (MSB) 00252 0 // bInterval 00253 }; 00254 return configDescriptor; 00255 }
Generated on Wed Jul 13 2022 02:20:46 by
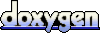