
; vergeten ergens?
Dependencies: HIDScope biquadFilter mbed
Fork of a_check_emg_filtered_new_lib by
main.cpp
00001 //libraries 00002 #include "mbed.h" 00003 #include "HIDScope.h" 00004 #include "BiQuad.h" 00005 00006 //Define objects 00007 AnalogIn emg_biceps_right_in( A0 ); //analog in to get EMG in to c++ 00008 Ticker sample_timer; //ticker 00009 HIDScope scope( 3); //open 3 channels in hidscope 00010 DigitalOut richting_motor1(D4); //motor1 direction output 00011 PwmOut pwm_motor1(D5); //motor1 velocity output 00012 DigitalOut led(LED_GREEN); 00013 DigitalIn button (D9); 00014 00015 //define variables 00016 double emg_biceps_right; 00017 double emg_filtered_high_biceps_right; 00018 double emg_abs_biceps_right; 00019 double emg_filtered_biceps_right; 00020 int onoffsignal=0; 00021 double cut_off_value=0.20; 00022 double max_right_biceps; 00023 00024 BiQuad filterhigh(9.5654e-01,-1.9131e+00,9.5654e-01,-1.9112e+00,9.1498e-01); 00025 BiQuad filterlow (6.2942e-06, 1.2588e-05,6.2942e-06,-1.9929e+00,9.9292e-01); 00026 00027 //functions which are called in ticker 00028 void filter(){ 00029 emg_biceps_right=emg_biceps_right_in.read(); //read the emg value from the elektrodes 00030 emg_filtered_high_biceps_right= filterhigh.step(emg_biceps_right); 00031 emg_abs_biceps_right=fabs(emg_filtered_high_biceps_right); //fabs because float 00032 emg_filtered_biceps_right=filterlow.step(emg_abs_biceps_right); 00033 led=!led; 00034 00035 if (emg_filtered_biceps_right>cut_off_value) 00036 {onoffsignal=1;} 00037 00038 else 00039 {onoffsignal=0;} 00040 00041 //send signals to scope 00042 scope.set(0, emg_biceps_right ); //set emg signal to scope in channel 1 00043 scope.set(1, emg_filtered_biceps_right); 00044 scope.set(2, onoffsignal); 00045 scope.send(); //send all the signals to the scope 00046 } 00047 00048 void calibration(){ 00049 for(int n =0; n<5000;n++){ //read for 5000 samples as calibration 00050 emg_biceps_right=emg_biceps_right_in.read(); //read the emg value from the elektrodes 00051 emg_filtered_high_biceps_right= filterhigh.step(emg_biceps_right); //highpass 00052 emg_abs_biceps_right=fabs(emg_filtered_high_biceps_right); //fabs because float 00053 emg_filtered_biceps_right=filterlow.step(emg_abs_biceps_right); //lowpass to envelope 00054 if (emg_filtered_biceps_right > max_right_biceps){ //determine what the highest reachable emg signal is 00055 max_right_biceps = emg_filtered_biceps_right; 00056 } 00057 00058 cut_off_value=0.2*max_right_biceps; 00059 } 00060 //program 00061 00062 int main() 00063 { 00064 sample_timer.attach(&filter, 0.001); //continously execute the EMG reader and filter, it ensures that filter and sampling is executed every 1/frequency seconds 00065 00066 //endless loop 00067 while(1) { 00068 if(button==0){ 00069 &calibri(); } //if button pressed calibration starts again! 00070 00071 if (onoffsignal==1) 00072 { 00073 richting_motor1 = 0; //motordirection (ccw) 00074 pwm_motor1 = 1; //motorspeed 1 00075 00076 } 00077 else if(onoffsignal==0) 00078 { 00079 richting_motor1 = 0; //motordirection (ccw) 00080 pwm_motor1 = 0; //motorspeed 0 00081 } 00082 00083 } 00084 }
Generated on Tue Aug 9 2022 19:00:10 by
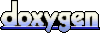