PCA9635 16-bit I2C-bus LED driver
Dependents: digitalThermometer Counter SimpleClock printNumber ... more
PCA9635.cpp
00001 //NXP PCA9635 library 00002 //mbed Team - 1st June 2011 00003 //Ioannis Kedros 00004 //updated by Daniel Worrall - 28th June 2011 00005 00006 #include "mbed.h" 00007 #include "PCA9635.h" 00008 00009 PCA9635::PCA9635(PinName sda, PinName scl) : m_i2c(sda, scl) 00010 { 00011 init(0x02); 00012 } 00013 00014 00015 //Driver Software Reset 00016 void PCA9635::reset(void){ 00017 cmd[0] = 0x06; 00018 int ack = m_i2c.write(m_addr, cmd, 1); 00019 if(!ack); 00020 else return; 00021 cmd[0] = 0xA5; 00022 cmd[1] = 0x5A; 00023 m_i2c.write(m_addr, cmd, 2); 00024 } 00025 00026 void PCA9635::init(int address){ 00027 m_addr = address; 00028 00029 reset(); 00030 00031 //Mode-1 Register: 00032 cmd[0] = 0x00; 00033 cmd[1] = 0x00; 00034 m_i2c.write(address, cmd, 2); 00035 00036 //Mode-2 Register: 00037 cmd[0] = 0x01; 00038 cmd[1] = 0x22; 00039 m_i2c.write(address, cmd, 2); 00040 00041 //LED Registers into PWM Control 00042 for(char i=0x14; i<0x18; i++) 00043 { 00044 cmd[0] = i; 00045 cmd[1] = 0xAA; 00046 m_i2c.write(address, cmd, 2); 00047 } 00048 } 00049 00050 void PCA9635::setAddress(int address){ 00051 m_addr = address; 00052 } 00053 00054 //Single LED On 00055 void PCA9635::on(char led) 00056 { 00057 cmd[0] = led + 2; //led position 00058 cmd[1] = 0xFF; //brightness value 00059 m_i2c.write(m_addr, cmd, 2); 00060 } 00061 00062 00063 //Single LED Off 00064 void PCA9635::off(char led) 00065 { 00066 cmd[0] = led + 2; //led position 00067 cmd[1] = 0x00; //brightness value 00068 m_i2c.write(m_addr, cmd, 2); 00069 } 00070 00071 00072 void PCA9635::bus(short leds) 00073 { 00074 short temp = leds; //check each bit of leds 00075 for(int j=0; j<16; j++) { 00076 if(temp & 1){ //set output according to LSB 00077 on(j); 00078 } 00079 else { 00080 off(j); 00081 } 00082 temp = (temp >> 1); //bitwise shift right by 1 to scan next bit 00083 } 00084 } 00085 00086 //Brightness control for single or all LEDs 00087 void PCA9635::brightness(char led, char value) 00088 { 00089 if(led == ALL) 00090 { 00091 for(char allLeds=0x02; allLeds<0x12; allLeds++) 00092 { 00093 cmd[0] = allLeds; 00094 cmd[1] = value; 00095 m_i2c.write(m_addr, cmd, 2); 00096 } 00097 } 00098 else 00099 { 00100 cmd[0] = led + 2; 00101 cmd[1] = value; 00102 m_i2c.write(m_addr, cmd, 2); 00103 } 00104 } 00105 00106 00107 00108 00109 00110
Generated on Tue Jul 19 2022 03:54:59 by
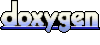