Library for interfacing with the Parallax Ping))) sensor.
Embed:
(wiki syntax)
Show/hide line numbers
ping.h
00001 #ifndef PING_H 00002 #define PING_H 00003 00004 #include "mbed.h" 00005 #include "FPointer.h" 00006 00007 #define DEFAULT_MEASUREMENT_DELAY_US 5000 00008 #define SPEED_OF_SOUND_CM_PER_US 0.034029 00009 #define SPEED_OF_SOUND_IN_PER_US 0.0133972441 00010 00011 /** 00012 * Class for interfacing with the Parallax Ping))) Ultrasonic Sensor 00013 * 00014 * See the Ping)) documentation for more information. 00015 * http://www.parallax.com/sites/default/files/downloads/28015-PING-Documentation-v1.6.pdf 00016 */ 00017 class Ping { 00018 public: 00019 /** 00020 * @param pin Digital I/O pin used for communicating with the Ping))) 00021 */ 00022 Ping (PinName pin); 00023 00024 /** 00025 * Starts a one shot reading. 00026 * 00027 * @return True if the reading was successfuly started, false if not. 00028 */ 00029 bool startReading(); 00030 00031 /** 00032 * Starts a reading. 00033 * 00034 * @param continuous Continuous readings if true, one-shot if false. 00035 * @return True if the reading was successfuly started, false if not. 00036 */ 00037 bool startReading(bool continuous); 00038 00039 /** 00040 * Returns whether the sensor is busy getting a reading 00041 * 00042 * @return True if busy, false if not. 00043 */ 00044 bool isBusy() { return mBusy; } 00045 00046 /** 00047 * Returns whether the valid reading is available 00048 * 00049 * @return True if valid, false if not. 00050 */ 00051 bool isValid() { return mValid; } 00052 00053 /** 00054 * Returns the raw reading from the sensor. 00055 * 00056 * @return The time in uS that the sound travelled from the sensor to an object. 00057 */ 00058 uint32_t getRawReading() { return mRawReading; } 00059 00060 /** 00061 * Sets the delay between continuous readings. 00062 * 00063 * @param delay_us Delay between readings in microseconds. 00064 */ 00065 void setContinuousReadingDelay(uint32_t delay_us) { mDelayBetweenReadings = delay_us; } 00066 00067 /** 00068 * Gets the distance sound can travel, in centimeters, for a given time in uS. 00069 * 00070 * @param time_us Time traveled in microseconds. 00071 * @return The distance traveled in centimeters. 00072 */ 00073 static float getDistanceCm(uint32_t time_us) { return time_us * SPEED_OF_SOUND_CM_PER_US; } 00074 00075 /** 00076 * Gets the distance sound can travel, in inches, for a given time in uS. 00077 * 00078 * @param time_us Time traveled in microseconds. 00079 * @return The distance traveled in inches. 00080 */ 00081 static float getDistanceIn(uint32_t time_us) { return time_us * SPEED_OF_SOUND_IN_PER_US; } 00082 00083 /** 00084 * Attach a callback function that will be called when a valid reading is available. 00085 * 00086 * @param function Function pointer for callback. 00087 */ 00088 void attach(uint32_t (*function)(uint32_t) = 0) { mCallback.attach(function); } 00089 00090 /** 00091 * Attach a class method that will be called when a valid reading is available. 00092 * 00093 * @param item Class object that contains the method to call. 00094 * @param method Method to call. 00095 */ 00096 template<class T> 00097 void attach(T* item, uint32_t (T::*method)(uint32_t)) { mCallback.attach(item, method); } 00098 00099 private: 00100 DigitalInOut mSignalIo; 00101 InterruptIn mEvent; 00102 00103 bool mBusy; 00104 bool mValid; 00105 bool mContinuous; 00106 uint32_t mDelayBetweenReadings; 00107 00108 Timer mTimer; 00109 Ticker mMeasureDelayTicker; 00110 00111 uint32_t mRawReading; 00112 00113 FPointer mCallback; 00114 00115 void start(); 00116 void stop(); 00117 void nextMeasurement(); 00118 }; 00119 00120 #endif
Generated on Fri Jul 15 2022 13:23:33 by
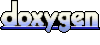