Library for interfacing with the Parallax Ping))) sensor.
Embed:
(wiki syntax)
Show/hide line numbers
ping.cpp
00001 #include "ping.h" 00002 00003 Ping::Ping (PinName signalPin) : mSignalIo(signalPin), mEvent(signalPin), 00004 mBusy(0), mValid(0), mContinuous(0), 00005 mDelayBetweenReadings(DEFAULT_MEASUREMENT_DELAY_US), mTimer(), mRawReading(0) { 00006 mEvent.rise(this, &Ping::start); 00007 mEvent.fall(this, &Ping::stop); 00008 } 00009 00010 bool Ping::startReading() { 00011 return this->startReading(false); 00012 } 00013 00014 bool Ping::startReading(bool continuous) { 00015 mContinuous = continuous; 00016 if (mBusy) return false; 00017 00018 mSignalIo.output(); 00019 mSignalIo.write(0); 00020 wait_us(3); 00021 mSignalIo.write(1); 00022 wait_us(3); 00023 mSignalIo.write(0); 00024 mSignalIo.input(); 00025 00026 return true; 00027 } 00028 00029 void Ping::start() { 00030 mBusy = true; 00031 mValid = false; 00032 mTimer.reset(); 00033 mTimer.start(); 00034 } 00035 00036 void Ping::stop() { 00037 mBusy = false; 00038 mValid = true; 00039 // The time it takes is for a round trip. We divide by two to get the time for one way. 00040 mRawReading = mTimer.read_us() >> 1; 00041 mTimer.stop(); 00042 mCallback.call(mRawReading); 00043 if (mContinuous) mMeasureDelayTicker.attach_us(this, &Ping::nextMeasurement, mDelayBetweenReadings); 00044 } 00045 00046 void Ping::nextMeasurement() { 00047 mMeasureDelayTicker.detach(); 00048 this->startReading(mContinuous); 00049 }
Generated on Fri Jul 15 2022 13:23:33 by
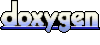