MAXIM DS3231 accurate Real Time Clock Library
Dependents: I2C-LCD-DHT22 wifigpslcd HomeStatus project ... more
DS3231.h
00001 /** mbded library for driving the PMAXIM DS3231 Real Time Clock 00002 * datasheet link : http://datasheets.maximintegrated.com/en/ds/DS3231.pdf 00003 * breakout : MACETECH ChronoDot V2.1 High Precision RTC 00004 * remi cormier 2012 00005 * WARNING : sda and sdl should be pulled up with 2.2k resistor 00006 */ 00007 00008 /** Example code 00009 * @code 00010 // DS3231 Library test program 00011 // remi cormier 2012 00012 00013 #include "mbed.h" 00014 #include "DS3231.h" 00015 00016 Serial pc(USBTX, USBRX); 00017 00018 int hour; 00019 int minute; 00020 int second; 00021 00022 int dayOfWeek; 00023 int date; 00024 int month; 00025 int year; 00026 00027 DS3231 RTC(p28,p27); 00028 00029 00030 int main() 00031 {printf("\r\n\nDS3231 Library test program\r\nremi cormier 2012\r\n\n"); 00032 00033 RTC.setI2Cfrequency(400000); 00034 00035 //RTC.writeRegister(DS3231_Aging_Offset,0); // uncomment to set Aging Offset 1LSB = approx. 0.1 ppm according from datasheet = 0.05 ppm @ 21 °C from my measurments 00036 00037 RTC.convertTemperature(); 00038 00039 int reg=RTC.readRegister(DS3231_Aging_Offset); 00040 if (reg>127) 00041 {reg=reg-256;} 00042 pc.printf("Aging offset : %i\r\n",reg); 00043 00044 pc.printf("OSF flag : %i",RTC.OSF()); 00045 pc.printf("\r\n"); 00046 00047 RTC.readDate(&date,&month,&year); 00048 pc.printf("date : %02i-%02i-%02i",date,month,year); 00049 pc.printf("\r\n"); 00050 00051 //RTC.setTime(19,48,45); // uncomment to set time 00052 00053 RTC.readTime(&hour,&minute,&second); 00054 pc.printf("time : %02i:%02i:%02i",hour,minute,second); 00055 pc.printf("\r\n"); 00056 00057 //RTC.setDate(6,22,12,2012); // uncomment to set date 00058 00059 RTC.readDateTime(&dayOfWeek,&date,&month,&year,&hour,&minute,&second); 00060 pc.printf("date time : %i / %02i-%02i-%02i %02i:%02i:%02i",dayOfWeek,date,month,year,hour,minute,second); 00061 pc.printf("\r\n"); 00062 00063 pc.printf("temperature :%6.2f",RTC.readTemp()); 00064 pc.printf("\r\n"); 00065 } 00066 * @endcode 00067 */ 00068 00069 00070 #include "mbed.h" 00071 00072 #ifndef MBED_DS3231_H 00073 #define MBED_DS3231_H 00074 00075 //DS3231 8 bit adress 00076 #define DS3231_Address 0xD0 00077 00078 //DS3231 registers 00079 #define DS3231_Seconds 0x00 00080 #define DS3231_Minutes 0x01 00081 #define DS3231_Hours 0x02 00082 // DS3231 Hours bits 00083 #define DS3231_bit_AM_PM 0x20 00084 #define DS3231_bit_12_24 0x40 00085 00086 #define DS3231_Day 0x03 00087 #define DS3231_Date 0x04 00088 #define DS3231_Month_Century 0x05 00089 #define DS3231_Year 0x06 00090 #define DS3231_Alarm1_Seconds 0x07 00091 #define DS3231_Alarm1_Minutes 0x08 00092 #define DS3231_Alarm1_Hours 0x09 00093 #define DS3231_Alarm1_Day_Date 0x0A 00094 #define DS3231_Alarm2_Minutes 0x0B 00095 #define DS3231_Alarm2_Hours 0x0C 00096 #define DS3231_Alarm_2_Day_Date 0x0D 00097 00098 #define DS3231_Control 0x0E 00099 // DS3231 Control bits 00100 #define DS3231_bit_A1IE 1 00101 #define DS3231_bit_A2IE 2 00102 #define DS3231_bit_INTCN 4 00103 #define DS3231_bit_SQW_1Hz 0 00104 #define DS3231_bit_SQW_1024Hz 8 00105 #define DS3231_bit_SQW_4096Hz 16 00106 #define DS3231_bit_SQW_8192Hz 24 00107 #define DS3231_bit_CONV 32 00108 #define DS3231_bit_BBSQW 64 00109 #define DS3231_bit_EOSCb 128 00110 00111 00112 #define DS3231_Control_Status 0x0F 00113 // DS3231 Control/Status bits 00114 #define DS3231_bit_BSY 0x04 00115 #define DS3231_bit_EN32kHz 0x08 00116 #define DS3231_bit_OSF 0x80 00117 00118 #define DS3231_Aging_Offset 0x10 00119 #define DS3231_MSB_Temp 0x11 00120 #define DS3231_LSB_Temp 0x12 00121 00122 /* Interface to MAXIM DS3231 RTC */ 00123 class DS3231 00124 {public : 00125 /** Create an instance of the DS3231 connected to specfied I2C pins 00126 * 00127 * @param sda The I2C data pin 00128 * @param scl The I2C clock pin 00129 */ 00130 DS3231(PinName sda, PinName scl); 00131 00132 /** set I2C bus speed 00133 * @param frequency : I2C clocl frequenct (Hz) 00134 */ 00135 void setI2Cfrequency(int frequency); 00136 00137 /** Read the temperature 00138 * 00139 * @return The temperature 00140 */ 00141 float readTemp(); 00142 00143 /** Read the time registers 00144 * @param hours 00145 * @param minutes 00146 * @param seconds 00147 */ 00148 void readTime(int *hours, int *minutes, int *seconds); 00149 00150 /** force temperature conversion 00151 * 00152 */ 00153 void convertTemperature(); 00154 00155 /** Set the time registers 00156 * @param hours 00157 * @param minutes 00158 * @param seconds 00159 */ 00160 void setTime(int hours, int minutes, int seconds); 00161 00162 /** Read the date registers 00163 * @param date 00164 * @param month 00165 * @param year 00166 */ 00167 void readDate(int *date, int *month, int *year); 00168 00169 /** Set the date registers 00170 * @param dayOfWeek : day of week 00171 * @param date 00172 * @param month 00173 * @param year 00174 */ 00175 void setDate(int dayOfWeek, int date, int month, int year); 00176 00177 /** Read the date and time registers 00178 * @param dayOfWeek : day of week 00179 * @param date 00180 * @param month 00181 * @param year 00182 * @param hours 00183 * @param minutes 00184 * @param seconds 00185 */ 00186 void readDateTime(int *dayOfWeek, int *date, int *month, int *year, int *hours, int *minutes, int *seconds); 00187 00188 /** Read a register 00189 * @param reg : register address 00190 * @return The register content 00191 */ 00192 int readRegister(char reg); 00193 00194 /** Write to a register 00195 * @param reg : register address 00196 * @param The register content 00197 */ 00198 void writeRegister(int reg,char byte); 00199 00200 /** set OSF (Oscillator Stop Flag) bit to 0 in Control Status register 00201 * should be done just after power up DS3231 00202 * OSF bit is automaticaly set to 1 when on power up or when the DS3231 oscillator stops 00203 */ 00204 void eraseOSF(); 00205 00206 /** Return OSF bit. If true the oscillator stopped or the DS3231 just powered up 00207 * @return The OSF bit 00208 */ 00209 bool OSF(); 00210 00211 bool error; 00212 00213 private : 00214 I2C i2c; 00215 int bcd2dec(int k); // bcd to decimal conversion 00216 int dec2bcd(int k); // decimal to bcd conversion 00217 void decodeTime(int regHours, int regMinutes, int regSeconds,int *Hours, int *Minutes, int *Seconds); 00218 void decodeDate(int regDate,int regMonth, int regYear, int *date, int *month, int *year); 00219 }; 00220 00221 00222 #endif
Generated on Tue Jul 12 2022 19:51:03 by
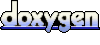