
Wheelchair control
Fork of wheelchaircontrol by
Embed:
(wiki syntax)
Show/hide line numbers
wheelchair.h
00001 #ifndef wheelchair 00002 #define wheelchair 00003 00004 #include "chair_imu.h" 00005 00006 #define def (2.5f/3.3f) 00007 #define high 3.3f 00008 #define offset .02f 00009 #define low (1.7f/3.3f) 00010 #define process .1 00011 #define xDir D12 //top right two pins 00012 #define yDir D13 //top left two pins 00013 00014 class Wheelchair 00015 { 00016 public: 00017 Wheelchair(PinName xPin, PinName yPin); 00018 void move(float x_coor, float y_coor); 00019 void turn_right(); 00020 void turn_left(); 00021 void forward(); 00022 void backward(); 00023 void right(); 00024 void left(); 00025 void stop(); 00026 00027 private: 00028 PwmOut* x; 00029 PwmOut* y; 00030 chair_imu* imu; 00031 00032 }; 00033 #endif
Generated on Thu Jul 14 2022 08:30:56 by
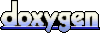