
Timer und Ticker
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // switch blue LEDs LED1 .. LED4 with external interrupt functionality 00002 /* 00003 3 classes 00004 Timeout - Call a function after a specified delay 00005 Ticker - Repeatedly call a function 00006 Timer - Create, start, stop and read a timer 00007 */ 00008 00009 #include "mbed.h" 00010 00011 Timeout toFlipper; 00012 DigitalOut doLed1(LED1); 00013 DigitalOut doLed2(LED2); 00014 Ticker tickBlinker; 00015 DigitalOut doLed3(LED3); 00016 DigitalOut doLed4(LED4); 00017 Timer tMyTimer; 00018 Serial pc(USBTX, USBRX); // tx, rx 00019 int count =0; 00020 int min=0; 00021 00022 // functions 00023 void flip() { 00024 doLed1 = !doLed1; 00025 } 00026 00027 void blink() { 00028 doLed3 = !doLed3; 00029 count ++; 00030 } 00031 00032 int main() { 00033 doLed2 = 1; 00034 toFlipper.attach(&flip, 2.0); // setup flipper to call flip after 2 seconds 00035 tickBlinker.attach(&blink, 1.0); // setup blinker to call blink avery 500 msec 00036 00037 // spin in a main loop. flipper will interrupt it to call flip 00038 while(1) { 00039 00040 if (count ==60) 00041 { 00042 min++; 00043 count=0; 00044 } 00045 tMyTimer.reset(); 00046 tMyTimer.start(); 00047 //pc.printf("Hello Timer-World! "); 00048 doLed4 = !doLed4; 00049 wait(1.0); 00050 tMyTimer.stop(); 00051 pc.printf("Uhr lauft seit %iMinuten & %i Sekunden\r",min,count); 00052 //The time taken was %f seconds\r", tMyTimer.read()); 00053 00054 } 00055 }
Generated on Sun Aug 21 2022 16:20:01 by
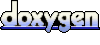