
HAND
Dependencies: mbed BMI160 MLX90614 max32630fthr Adafruit_FeatherOLED MAX30100
functions.cpp
00001 #include "functions.h" 00002 #include "ble_functions.h" 00003 #include "RawSerial.h" 00004 #include <InterruptIn.h> 00005 #include <InterruptManager.h> 00006 #include "bmi160.h" 00007 #include "max32630fthr.h" 00008 #include "max3263x.h" 00009 #include "MAX14690.h" 00010 #include "MAX30100_PulseOximeter.h" 00011 #include "mlx90614.h" 00012 #include "Adafruit_SSD1306.h" 00013 #include <BLE.h> 00014 #include "ble/BLE.h" 00015 #include "ble/Gap.h" 00016 #include "SDBlockDevice.h" 00017 #include "FATFileSystem.h" 00018 #include <errno.h> 00019 #include <stdio.h> 00020 #include <time.h> 00021 #include <stdint.h> 00022 00023 extern char device_id[]; 00024 extern unsigned short int measure_id; 00025 extern unsigned short int log_id; 00026 00027 extern Serial pan1326b; 00028 extern DigitalOut bt_rst; 00029 00030 extern volatile int conn_state; 00031 extern bool in_BT; 00032 00033 extern AnalogIn battery; 00034 00035 extern DigitalOut r; 00036 extern DigitalOut g; 00037 extern DigitalOut b; 00038 extern DigitalOut RGBs[]; 00039 00040 extern MAX32630FTHR pegasus; 00041 00042 extern I2C i2cm2; 00043 extern MAX14690 max14690; 00044 00045 extern InterruptIn button; 00046 00047 extern FATFileSystem fileSystem; 00048 extern SDBlockDevice blockDevice; 00049 00050 extern I2C i2c; 00051 extern int sensor_temp; 00052 extern Adafruit_SSD1306_I2c featherOLED; 00053 00054 extern Timer t; 00055 extern PulseOximeter pox; 00056 extern uint32_t tsLastReport; 00057 00058 extern DigitalIn ain; 00059 extern double anSignal; 00060 extern int bpm; 00061 extern int signal; 00062 extern int ibi; 00063 extern MLX90614 IR_thermometer; 00064 extern float ir_temp; 00065 00066 extern volatile int quit; 00067 extern volatile int ble_return; 00068 extern volatile int conn_state; 00069 extern int counter_ble; 00070 00071 extern bool error_status; 00072 extern bool BT_error; 00073 extern bool shipping; 00074 extern bool pause; 00075 extern bool init_status; 00076 extern bool after_BT; 00077 00078 extern Timeout after_BLE; 00079 extern Timeout turnoff; 00080 extern Timeout flipper; // 00081 extern Timeout advertise_cancel; // Zum abbrechen von BT bei keinem Verbindungsaufbau 00082 extern Timeout connected_cancel; // Zum abbrechen von BT bei kein Befehlempfang 00083 extern Ticker mess_timer; // ticker eventuell nur bis 30 Minuten geeignet 00084 extern Ticker ticker; 00085 extern time_t now; 00086 extern time_t raw; 00087 00088 extern float buffer_temp; 00089 extern struct tm current; 00090 extern struct tm actual; 00091 00092 extern struct user_config_struct user_config_para; 00093 extern struct tm user_config_time; 00094 00095 extern Timeout done_rcv; 00096 00097 extern int next_state; 00098 00099 extern int unsigned long t_diff; 00100 extern int unsigned long unix_time; 00101 00102 extern int default_advertise_time; 00103 extern int default_connected_time; 00104 00105 extern int alert_count; 00106 00107 extern Timer t; 00108 extern PulseOximeter pox; 00109 extern uint32_t tsLastReport; 00110 00111 bool newValueMAX30100 = 0; 00112 extern float heartRate; 00113 float finalHeartRate; 00114 extern uint8_t sp02; 00115 uint16_t finalSp02; 00116 uint32_t REPORTING_PERIOD_MS = 1000; 00117 std::vector<float> valuesHeartRate; 00118 std::vector<uint8_t> valuesSp02; 00119 uint8_t samplesMAX30100 = 10; 00120 uint8_t counterMAX30100 = 0; 00121 00122 void turnON(){ 00123 max14690.writeReg(MAX14690::REG_PWR_CFG, 0x1D); 00124 char string[] = "H.A.N.D. turned on"; 00125 write_to_sd_log_single(string); 00126 } 00127 00128 void reboot(){ 00129 char string[] = "Rebooting H.A.N.D. ..."; 00130 write_to_sd_log_single(string); 00131 printf("\n__________________________\n\nRebooting H.A.N.D.\n__________________________\n\n"); 00132 log_id -= 2; 00133 BT_false(); 00134 wait(0.2); 00135 NVIC_SystemReset(); 00136 } 00137 00138 void set_time(){ 00139 unix_time = 946684800; //946684800: 2000-01-01 00:00:00 00140 now = time(0); 00141 time(&now); 00142 set_time(unix_time); 00143 } 00144 00145 int set_app_time(unsigned long device_time){ 00146 printf("\nDevice time: %ld\n", device_time); 00147 if(device_time >= 946684800){ 00148 unix_time = device_time; 00149 now = time(0); 00150 time(&now); 00151 set_time(unix_time); 00152 00153 tm *current; 00154 time(&now); 00155 current = localtime (&now); 00156 char string[] = "Device time updated"; 00157 write_to_sd_log_single(string); 00158 printf ("\nDate: 20%02d-%02d-%02d %02d:%02d:%02d\r\n", current->tm_year - 100, current->tm_mon + 1, current->tm_mday, current->tm_hour, current->tm_min, current->tm_sec); 00159 printf("\n__________________________\n\nNew device time applied\n__________________________\n\n"); 00160 00161 return 1; 00162 }else{ 00163 printf("\n__________________________\n\nCan not applie new device time\n__________________________\n\n"); 00164 00165 return -1; 00166 } 00167 } 00168 00169 void write_to_sd_log_single(char log_string[]) 00170 { 00171 fileSystem.unmount(); 00172 fflush(stdout); 00173 00174 fileSystem.mount(&blockDevice); 00175 00176 char file_name[14] = "/fs/log"; 00177 char file_type[10] = ".csv"; 00178 char buff_file[25]; 00179 sprintf(buff_file, "%s%s", file_name, file_type); 00180 FILE * f = fopen(buff_file, "a"); 00181 00182 log_id++; 00183 00184 tm *current; 00185 time(&now); 00186 current = localtime (&now); 00187 fprintf(f, "%d;20%02d-%02d-%02d;%02d:%02d:%02d;%s;\r\n", log_id, current->tm_year - 100, current->tm_mon + 1, current->tm_mday, current->tm_hour, current->tm_min, current->tm_sec, log_string); 00188 00189 fclose(f); 00190 fileSystem.unmount(); 00191 } 00192 00193 void write_to_sd_messdaten_single(float buffer_temp) 00194 { 00195 fileSystem.unmount(); 00196 fflush(stdout); 00197 00198 fileSystem.mount(&blockDevice); 00199 00200 char file_name[14] = "/fs/messdaten"; 00201 char file_type[10] = ".csv"; 00202 char buff_file[25]; 00203 sprintf(buff_file, "%s%s", file_name, file_type); 00204 FILE * f = fopen(buff_file, "a"); 00205 00206 int buffer_conv = (int)(100*buffer_temp); 00207 00208 measure_id++; 00209 00210 tm *current; 00211 time(&now); 00212 current = localtime (&now); 00213 fprintf(f, "%d;20%02d-%02d-%02d;%02d:%02d:%02d;%d;%4d;\r\n", measure_id, current->tm_year - 100, current->tm_mon + 1, current->tm_mday, current->tm_hour, current->tm_min, current->tm_sec, sensor_temp, buffer_conv); 00214 00215 fclose(f); 00216 fileSystem.unmount(); 00217 } 00218 00219 void get_save_Messpunkt(float temperatur) 00220 { 00221 buffer_temp = temperatur; 00222 00223 tm *current; 00224 time(&now); 00225 current = localtime (&now); 00226 write_to_sd_messdaten_single(buffer_temp); 00227 sensor_temp = 2; 00228 write_to_sd_messdaten_single(finalHeartRate); 00229 write_to_sd_messdaten_single((float)finalSp02); 00230 sensor_temp = 1; 00231 printf ("Measurement %d saved 20%02d-%02d-%02d %02d:%02d:%02d\r\n", measure_id, current->tm_year - 100, current->tm_mon + 1, current->tm_mday, current->tm_hour, current->tm_min, current->tm_sec); 00232 } 00233 00234 float get_Messwert() 00235 { 00236 float temperatur = 0; 00237 00238 IR_thermometer.getTemp(&temperatur); 00239 00240 wait(0.3); 00241 00242 if(user_config_para.alert == 1){ 00243 if(user_config_para.minimum > temperatur){ 00244 char string[] = "ALERT: lower threshold limit below"; 00245 write_to_sd_log_single(string); 00246 00247 printf("ALERT: lower threshold limit below (lower limit: %d C)\n", user_config_para.minimum); 00248 00249 alert_count++; 00250 }else if(user_config_para.maximum < temperatur){ 00251 char string[] = "ALERT: upper threshold exceeded"; 00252 write_to_sd_log_single(string); 00253 00254 printf("ALERT: upper threshold exceeded (upper limit: %d C)\n", user_config_para.maximum); 00255 00256 alert_count++; 00257 } 00258 } 00259 00260 get_save_Messpunkt(temperatur); 00261 00262 return temperatur; 00263 } 00264 00265 int create_file_messdaten(){ 00266 fileSystem.unmount(); 00267 fflush(stdout); 00268 00269 int file_err = fileSystem.mount(&blockDevice); 00270 if(file_err){ 00271 fileSystem.unmount(); 00272 00273 char string[] = "SD mount error"; 00274 write_to_sd_log_single(string); 00275 00276 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00277 00278 return -1; 00279 }else{ 00280 00281 char file_name[14] = "/fs/messdaten"; 00282 char file_type[10] = ".csv"; 00283 char buff_file[25]; 00284 sprintf(buff_file, "%s%s", file_name, file_type); 00285 FILE * f = fopen(buff_file, "a"); 00286 00287 fclose(f); 00288 fileSystem.unmount(); 00289 00290 return 1; 00291 } 00292 } 00293 00294 int create_file_log() 00295 { 00296 fileSystem.unmount(); 00297 fflush(stdout); 00298 00299 int file_err = fileSystem.mount(&blockDevice); 00300 if(file_err){ 00301 fileSystem.unmount(); 00302 00303 char string[] = "SD mount error"; 00304 write_to_sd_log_single(string); 00305 00306 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00307 00308 return -1; 00309 }else{ 00310 00311 char file_name[14] = "/fs/log"; 00312 char file_type[10] = ".csv"; 00313 char buff_file[25]; 00314 sprintf(buff_file, "%s%s", file_name, file_type); 00315 FILE * f = fopen(buff_file, "a"); 00316 00317 fclose(f); 00318 fileSystem.unmount(); 00319 00320 return 1; 00321 } 00322 } 00323 00324 int delete_file_messdaten(){ 00325 fileSystem.unmount(); 00326 fflush(stdout); 00327 00328 int file_err = fileSystem.mount(&blockDevice); 00329 if(file_err) 00330 { 00331 char string[] = "SD mount error"; 00332 write_to_sd_log_single(string); 00333 00334 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00335 00336 fileSystem.unmount(); 00337 00338 return -1; 00339 }else{ 00340 00341 remove("/fs/messdaten.csv"); 00342 wait(0.2); 00343 fileSystem.unmount(); 00344 fflush(stdout); 00345 00346 char string_1[] = "Measuring data file deleted"; 00347 write_to_sd_log_single(string_1); 00348 00349 if(create_file_messdaten() == 1){ 00350 00351 char string[] = "New measuring data file created"; 00352 write_to_sd_log_single(string); 00353 00354 fileSystem.unmount(); 00355 00356 measure_id = 0; 00357 00358 printf("\n__________________________\n\nMeasuring data deleted\n__________________________\n\n"); 00359 return 1; 00360 }else{ 00361 fileSystem.unmount(); 00362 printf("\nError while creating new file\n"); 00363 return -1; 00364 } 00365 } 00366 } 00367 00368 int delete_file_log(){ 00369 fileSystem.unmount(); 00370 fflush(stdout); 00371 00372 int file_err = fileSystem.mount(&blockDevice); 00373 if(file_err) 00374 { 00375 char string[] = "SD mount error"; 00376 write_to_sd_log_single(string); 00377 00378 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00379 00380 fileSystem.unmount(); 00381 00382 return -1; 00383 }else{ 00384 00385 remove("/fs/log.csv"); 00386 wait(0.2); 00387 /* 00388 char string_1[] = "Log file deleted"; 00389 write_to_sd_log_single(string_1); 00390 */ 00391 if(create_file_log() == 1){ 00392 00393 log_id = 0; 00394 00395 char string[] = "New log data file created"; 00396 write_to_sd_log_single(string); 00397 00398 fileSystem.unmount(); 00399 00400 printf("\n__________________________\n\nLog deleted\n__________________________\n\n"); 00401 return 1; 00402 }else{ 00403 fileSystem.unmount(); 00404 printf("\nError while creating new file\n"); 00405 return -1; 00406 } 00407 } 00408 } 00409 00410 int file_copy(const char *src, const char *dst) 00411 { 00412 fileSystem.unmount(); 00413 fflush(stdout); 00414 00415 int retval = 0; 00416 int ch; 00417 00418 int file_err = fileSystem.mount(&blockDevice); 00419 if(file_err) 00420 { 00421 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00422 fileSystem.unmount(); 00423 00424 return -1; 00425 }else{ 00426 00427 FILE *fpsrc = fopen(src, "r"); // src file 00428 FILE *fpdst = fopen(dst, "w"); // dest file 00429 00430 while (1) { // Copy src to dest 00431 ch = fgetc(fpsrc); // until src EOF read. 00432 if (ch == EOF) break; 00433 fputc(ch, fpdst); 00434 } 00435 fclose(fpsrc); 00436 fclose(fpdst); 00437 00438 fpdst = fopen(dst, "r"); // Reopen dest to insure 00439 if (fpdst == NULL) { // that it was created. 00440 retval = -1; // Return error. 00441 } else { 00442 fclose(fpdst); 00443 retval = 0; // Return success. 00444 } 00445 return retval; 00446 } 00447 } 00448 00449 int load_user_config() 00450 { 00451 printf("\n__________________________\n\nReading the config\n__________________________\n\n"); 00452 00453 fileSystem.unmount(); 00454 fflush(stdout); 00455 00456 int file_err = fileSystem.mount(&blockDevice); 00457 if(file_err) 00458 { 00459 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00460 fileSystem.unmount(); 00461 00462 return -1; 00463 }else{ 00464 00465 FILE * conf = fopen ("/fs/user_config.csv", "rb"); 00466 char buffer[700]; 00467 int length; 00468 00469 if (conf) 00470 { 00471 fseek (conf, 0, SEEK_END); 00472 length = ftell (conf); 00473 fseek (conf, 0, SEEK_SET); 00474 00475 if (buffer) 00476 { 00477 fread (buffer, 1, length, conf); 00478 } 00479 fclose(conf); 00480 fileSystem.unmount(); 00481 00482 if (buffer) 00483 { 00484 char* single_word[100]; 00485 int word_count = 0; 00486 char delim[] = ";\r\n"; 00487 char *ptr = strtok(buffer, delim); 00488 00489 for(int j = 0; j < 100; j++) 00490 { 00491 single_word[j] = new char[15]; 00492 } 00493 00494 while (ptr != NULL) 00495 { 00496 strcpy(single_word[word_count], ptr); 00497 ptr = strtok(NULL, delim); 00498 word_count++; 00499 } 00500 00501 const char *params[] = {"interval", "alert", "minimum", "maximum", "advertise", "connected"}; 00502 for(int k = 0; k < 100; k++) 00503 { 00504 for(int l = 0; l < 5; l++) 00505 { 00506 if(strcmp(single_word[k], params[0]) == 0){ 00507 user_config_para.interval = atoi(single_word[k+1]); 00508 } 00509 else if(strcmp(single_word[k], params[1]) == 0){ 00510 user_config_para.alert = atoi(single_word[k+1]); 00511 } 00512 else if(strcmp(single_word[k], params[2]) == 0){ 00513 user_config_para.minimum = atoi(single_word[k+1]); 00514 } 00515 else if(strcmp(single_word[k], params[3]) == 0){ 00516 user_config_para.maximum = atoi(single_word[k+1]); 00517 } 00518 else if(strcmp(single_word[k], params[4]) == 0){ 00519 user_config_para.advertise = atoi(single_word[k+1]); 00520 } 00521 else if(strcmp(single_word[k], params[5]) == 0){ 00522 user_config_para.connected = atoi(single_word[k+1]); 00523 } 00524 } 00525 } 00526 00527 //printf("\nID: #%s\n", user_config_para.id); 00528 00529 //char idnummer[10]; 00530 //sscanf(idnummer, "%d", &user_config_para.id); 00531 00532 printf("\nH.A.N.D. ID #%s\n", device_id); 00533 if(user_config_para.interval == 1){ 00534 printf("Mesureual interval: %d second\n", user_config_para.interval); 00535 }else{ 00536 printf("Mesureual interval: %d seconds\n", user_config_para.interval); 00537 } 00538 if(user_config_para.alert == 1){ 00539 printf("Alert-function - ON\n"); 00540 printf("Minimum temperature: %d C | Maximum temperature: %d C\n", user_config_para.minimum, user_config_para.maximum); 00541 }else{ 00542 printf("Alert-function - OFF\n"); 00543 } 00544 printf("BLE advertise timer: %d seconds\n", user_config_para.advertise); 00545 printf("BLE when connected timer: %d seconds\n", user_config_para.connected); 00546 } 00547 else{ 00548 printf("Buffer empty: %s", buffer); 00549 } 00550 return 1; 00551 } 00552 else{ 00553 printf("!!! Config file not found, error: %s (%d)\n", strerror(errno), -errno); 00554 fileSystem.unmount(); 00555 user_config_para.advertise = default_advertise_time; 00556 user_config_para.connected = default_connected_time; 00557 return -1; 00558 } 00559 } 00560 } 00561 00562 int load_standard_config(){ 00563 fileSystem.unmount(); 00564 fflush(stdout); 00565 00566 printf("\n__________________________\n\nReseting config settings \nto default\n__________________________\n\n"); 00567 00568 int file_err = fileSystem.mount(&blockDevice); 00569 00570 if(file_err){ 00571 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00572 fileSystem.unmount(); 00573 00574 return -1; 00575 }else{ 00576 remove("/fs/user_config.csv"); 00577 wait(0.2); 00578 00579 if(file_copy("/fs/standard_config.csv", "/fs/user_config.csv") == 0){ 00580 load_user_config(); 00581 00582 printf("\n__________________________\n\nConfig settings reseted\n__________________________\n\n"); 00583 char string[] = "Standard user config file created"; 00584 write_to_sd_log_single(string); 00585 fileSystem.unmount(); 00586 00587 return 1; 00588 }else{ 00589 printf("\n__________________________\n\nFailed to reset config file\n__________________________\n\n"); 00590 char string[] = "Failed to copy standard config file"; 00591 write_to_sd_log_single(string); 00592 fileSystem.unmount(); 00593 00594 return -1; 00595 } 00596 } 00597 } 00598 00599 int read_id() 00600 { 00601 fileSystem.unmount(); 00602 fflush(stdout); 00603 00604 int file_err = fileSystem.mount(&blockDevice); 00605 if(file_err) 00606 { 00607 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00608 fileSystem.unmount(); 00609 00610 return -1; 00611 }else{ 00612 00613 FILE * id = fopen ("/fs/id.csv", "rb"); 00614 char buffer[14]; 00615 int length; 00616 00617 if (id) 00618 { 00619 fseek (id, 0, SEEK_END); 00620 length = ftell (id); 00621 fseek (id, 0, SEEK_SET); 00622 00623 if (buffer) 00624 { 00625 fread (buffer, 1, length, id); 00626 } 00627 fclose(id); 00628 fileSystem.unmount(); 00629 00630 if (buffer) 00631 { 00632 char* single_word[10]; 00633 int word_count = 0; 00634 char delim[] = ";\r\n"; 00635 char *ptr = strtok(buffer, delim); 00636 00637 for(int j = 0; j < 10; j++) 00638 { 00639 single_word[j] = new char[10]; 00640 } 00641 00642 while (ptr != NULL) 00643 { 00644 strcpy(single_word[word_count], ptr); 00645 ptr = strtok(NULL, delim); 00646 word_count++; 00647 } 00648 00649 strcpy(device_id, single_word[1]); 00650 return 1; 00651 } 00652 else{ 00653 printf("Buffer empty: %s", buffer); 00654 return -1; 00655 } 00656 } 00657 else{ 00658 printf("!!! Config file not found, error: %s (%d)\n", strerror(errno), -errno); 00659 fileSystem.unmount(); 00660 return -1; 00661 } 00662 } 00663 } 00664 00665 int create_user_config(char params[]){ 00666 fileSystem.unmount(); 00667 fflush(stdout); 00668 00669 int file_err = fileSystem.mount(&blockDevice); 00670 if(file_err){ 00671 printf("\n__________________________\n\n! No SD-card detected !\n__________________________\n\n"); 00672 fileSystem.unmount(); 00673 00674 return -1; 00675 }else{ 00676 remove("/fs/user_config.csv"); 00677 wait(0.2); 00678 char string[] = "User config file deleted"; 00679 write_to_sd_log_single(string); 00680 fileSystem.unmount(); 00681 00682 fileSystem.mount(&blockDevice); 00683 char file_name[16] = "/fs/user_config"; 00684 char file_type[10] = ".csv"; 00685 char buff_file[27]; 00686 sprintf(buff_file, "%s%s", file_name, file_type); 00687 FILE * f = fopen(buff_file, "a"); 00688 00689 fclose(f); 00690 00691 char string_1[] = "User config file created"; 00692 write_to_sd_log_single(string_1); 00693 00694 fileSystem.unmount(); 00695 00696 char *single_word[15]; 00697 int word_count = 0; 00698 char delim[] = ";\r\n"; 00699 char *ptr = strtok(params, delim); 00700 for(int j = 0; j < 9; j++) 00701 { 00702 single_word[j] = new char[6]; 00703 } 00704 00705 while (ptr != NULL) 00706 { 00707 strcpy(single_word[word_count], ptr); 00708 ptr = strtok(NULL, delim); 00709 word_count++; 00710 } 00711 printf("interval;%s;\r\nalert;%s;\r\nminimum;%s;\r\nmaximum;%s;\r\nadvertise;%s;\r\nconnected;%s;", 00712 single_word[0], single_word[1], single_word[2], single_word[3], single_word[4]); 00713 00714 fileSystem.mount(&blockDevice); 00715 FILE * fp = fopen("/fs/user_config.csv", "w"); 00716 00717 fprintf(fp, "interval;%s;\r\n", single_word[0]); 00718 fprintf(fp, "alert;%s;\r\n", single_word[1]); 00719 fprintf(fp, "minimum;%s;\r\n", single_word[2]); 00720 fprintf(fp, "maximum;%s;\r\n", single_word[3]); 00721 fprintf(fp, "advertise;%s;\r\n", single_word[4]); 00722 fprintf(fp, "connected;%s;\r\n", single_word[5]); 00723 00724 fclose(fp); 00725 fileSystem.unmount(); 00726 00727 if(load_user_config() == 1){ 00728 return 1; 00729 }else{ 00730 printf("\nFaild to read the config!\n"); 00731 return -1; 00732 } 00733 } 00734 } 00735 00736 void led_blink(int led, int anzahl, int lang, int pause){ 00737 for(int i = 0; anzahl > i; i++){ 00738 RGBs[led] = 0; 00739 if(lang == 1){ 00740 wait(1.5); 00741 }else{ 00742 wait(0.5); 00743 } 00744 RGBs[led] = 1; 00745 wait(0.5); 00746 } 00747 if(pause == 1){ 00748 wait(1); 00749 } 00750 } 00751 00752 void error_handler(int error) 00753 { 00754 g = 1; 00755 wait(1); 00756 00757 int file_err = fileSystem.mount(&blockDevice); 00758 if(file_err){ 00759 switch (error) { 00760 case 10:{ led_blink(0, 2, 1, 1); } 00761 break; 00762 case 11:{ BT_error = true; led_blink(0, 1, 1, 1); led_blink(0, 2, 1, 1); } 00763 break; 00764 case 110:{ led_blink(0, 2, 1, 1); led_blink(0, 3, 1, 1); } 00765 break; 00766 case 111:{ BT_error = true; led_blink(0, 1, 1, 1); led_blink(0, 2, 1, 1); led_blink(0, 3, 1, 1); } 00767 break; 00768 } 00769 fileSystem.unmount(); 00770 }else{ 00771 tm *current; 00772 time(&now); 00773 current = localtime (&now); 00774 00775 switch (error) { 00776 case 0:{ char string[] = "Initializing succesfull"; write_to_sd_log_single(string); led_blink(1, 1, 0, 0); } 00777 break; 00778 case 1:{ char string[] = "Initializing failed: Bluetooth not detected"; write_to_sd_log_single(string); BT_error = true; led_blink(0, 1, 1, 1); } 00779 break; 00780 case 100:{ char string[] = "Initializing failed: Temperature sensor not detected"; write_to_sd_log_single(string); led_blink(0, 3, 1, 1); } 00781 break; 00782 case 101:{ char string[] = "Initializing failed: Temperature sensor and bluetooth not detected"; write_to_sd_log_single(string); BT_error = true; led_blink(0, 1, 1, 1); led_blink(0, 3, 1, 1); } 00783 break; 00784 } 00785 fileSystem.unmount(); 00786 } 00787 r = 1; 00788 g = 1; 00789 b = 1; 00790 wait(0.5); 00791 } 00792 /* 00793 void sendDataToProcessing(char symbol, int data) 00794 { 00795 if(symbol == 'S'){ 00796 signal = data; 00797 } 00798 else if(symbol == 'B'){ 00799 bpm = data; 00800 } 00801 else if(symbol == 'Q'){ 00802 ibi = data; 00803 } 00804 } 00805 */ 00806 00807 void onBeatDetected() 00808 { 00809 //printf("Beat!\r\n"); 00810 } 00811 00812 bool setup() 00813 { 00814 // Initialize the PulseOximeter instance and register a beat-detected callback 00815 if(!pox.begin()) 00816 return false; 00817 pox.setOnBeatDetectedCallback(onBeatDetected); 00818 return true; 00819 } 00820 00821 void updateMAX30100 (){ 00822 // Make sure to call update as fast as possible 00823 pox.update(); 00824 00825 if (t.read_ms() > REPORTING_PERIOD_MS) { 00826 heartRate = pox.getHeartRate(); 00827 sp02 = pox.getSpO2(); 00828 00829 if(heartRate != 0 && sp02 != 0) { 00830 valuesHeartRate.push_back(heartRate); 00831 valuesSp02.push_back(sp02); 00832 counterMAX30100 ++; 00833 next_state = 1; 00834 }else{ 00835 next_state = 0; 00836 } 00837 00838 if(samplesMAX30100 == counterMAX30100) { 00839 00840 finalHeartRate = 0; 00841 finalSp02 = 0; 00842 for(int i=0; i<samplesMAX30100; i++){ 00843 finalHeartRate += valuesHeartRate[i]; 00844 finalSp02 += valuesSp02[i]; 00845 } 00846 00847 finalHeartRate /= samplesMAX30100; 00848 finalSp02 /= samplesMAX30100; 00849 00850 counterMAX30100 = 0; 00851 valuesHeartRate.clear(); 00852 valuesSp02.clear(); 00853 newValueMAX30100 = true; 00854 } 00855 00856 t.reset(); 00857 } 00858 } 00859 00860 void loop() 00861 { 00862 updateMAX30100(); 00863 00864 if(newValueMAX30100){ 00865 printf("--->New Value\r\n"); 00866 printf("Heart rate: %f",finalHeartRate); 00867 printf(" bpm / SpO2: %d%\r\n",finalSp02); 00868 printf("*************************\r\n"); 00869 newValueMAX30100 = false; 00870 } 00871 00872 } 00873 00874 int check_devices() 00875 { 00876 printf("\nDevices check...\n\n"); 00877 00878 int bl = 1; 00879 int sd = 10; 00880 int tempsen = 100; 00881 int pulsoxi = 1000; 00882 00883 //BLE check 00884 bt_rst = 1; 00885 bt_rst = 0; 00886 wait(0.5); 00887 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00888 ble.init(bleInitComplete); 00889 if(ble.hasInitialized() == true){ 00890 bl = 0; 00891 BT_error = false; 00892 printf("\nBLE detected\n"); 00893 printMacAddress(); 00894 }else{ 00895 BT_error = true; 00896 printf("\nBLE not detected!\n"); 00897 } 00898 00899 //SD check 00900 int file_err = fileSystem.mount(&blockDevice); 00901 if(file_err) 00902 { 00903 printf("\nSD-card not detected\n"); 00904 BT_error = true; 00905 } 00906 else { 00907 sd = 0; 00908 create_file_log(); 00909 create_file_messdaten(); 00910 printf("\nSD-card detected\n"); 00911 00912 fileSystem.unmount(); 00913 00914 messdaten_count(); 00915 printf("Measuremnts count: %d\n", measure_id); 00916 log_count(); 00917 printf("Logs count: %d\n", log_id); 00918 } 00919 fileSystem.unmount(); 00920 00921 //IR Thermometer check 00922 if(IR_thermometer.getTemp(&ir_temp)){ 00923 printf("\nIR Thermometer detected\n"); 00924 tempsen = 0; 00925 }else{ 00926 printf("\nIR Thermometer not detected\n"); 00927 } 00928 00929 //Pulsoximeter check 00930 if(setup()){ 00931 printf("\nPulsoximeter detected!\r\n"); 00932 t.start(); 00933 pulsoxi = 0; 00934 }else{ 00935 printf("\nPulsoximeter not detected!\r\n"); 00936 } 00937 00938 //Check output 00939 int devices = pulsoxi + tempsen + sd + bl; 00940 00941 if(devices != 0000){ 00942 printf("\nError in check_devices(): %04d\n", devices); 00943 00944 error_handler(devices); 00945 00946 return 0; 00947 00948 }else{ 00949 printf("\n__________________________\n\nAll devices connected\n__________________________\n\n"); 00950 00951 return 1; 00952 } 00953 } 00954 00955 int RTC_check(){ 00956 tm *current; 00957 time(&now); 00958 if ( (current = localtime (&now) ) == NULL) { 00959 printf ("\n\n__________________________\n\n! RTC initialize failed !\n__________________________\n"); 00960 return -1; 00961 }else{ 00962 set_time(); 00963 printf ("\n\n__________________________\n\nRTC initialized\n__________________________\n"); 00964 return 1; 00965 } 00966 } 00967 00968 void max14690_init(){ 00969 max14690.resetToDefaults(); 00970 max14690.ldo2Millivolts = 3300; 00971 max14690.ldo3Millivolts = 3300; 00972 max14690.ldo2Mode = MAX14690::LDO_ENABLED; 00973 max14690.ldo3Mode = MAX14690::LDO_ENABLED; 00974 max14690.intEnChgStatus = 1; 00975 max14690.monCfg = MAX14690::MON_SYS; //Monitoring - Multiplexer auf Battery eingestellt, um Akku-wert auszulesen 00976 max14690.monRatio = MAX14690::MON_DIV4; 00977 max14690.intEnChgStatus = 0; 00978 max14690.ldo3Mode = MAX14690::SW_EN_MPC1_DSC; 00979 if (max14690.init() == MAX14690_ERROR) { 00980 printf("Error initializing MAX14690"); 00981 } 00982 } 00983 00984 void init(){ 00985 r = 1; 00986 g = 1; 00987 b = 1; 00988 00989 printf("\n\n__________________________\n\nStarting H.A.N.D.\n__________________________\n\n"); 00990 g = 0; 00991 00992 printf("Initializing MAX32630FTHR\n\n"); 00993 pegasus.init(MAX32630FTHR::VIO_3V3); 00994 00995 printf("Initializing MAX14690N\n"); 00996 max14690_init(); 00997 00998 if(RTC_check() == 1){ 00999 if(check_devices() == 1 & read_id() == 1 & load_user_config() == 1){ 01000 printf("\n__________________________\n\nInitializing succesfull\n__________________________\n\n"); 01001 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 01002 g = 1; 01003 init_status = true; 01004 error_status = false; 01005 error_handler(000); 01006 }else{ 01007 printf("\n__________________________\n\nInitializing failed\n__________________________\n\n"); 01008 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 01009 next_state = 0; 01010 init_status = false; 01011 error_status = true; 01012 g = 1; 01013 } 01014 }else{ 01015 printf("\n__________________________\n\nRTC failure\n__________________________\n\n"); 01016 error_status = true; 01017 init_status = false; 01018 next_state = 0; 01019 g = 1; 01020 } 01021 }
Generated on Wed Jul 27 2022 23:42:13 by
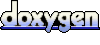