
HAND
Dependencies: mbed BMI160 MLX90614 max32630fthr Adafruit_FeatherOLED MAX30100
ble_functions.cpp
00001 #include "functions.h" 00002 #include "ble_functions.h" 00003 #include "RawSerial.h" 00004 #include <InterruptIn.h> 00005 #include <InterruptManager.h> 00006 #include "bmi160.h" 00007 #include "max32630fthr.h" 00008 #include "max3263x.h" 00009 #include "MAX14690.h" 00010 #include "Adafruit_SSD1306.h" 00011 #include "MAX30100_PulseOximeter.h" 00012 #include "mlx90614.h" 00013 #include <BLE.h> 00014 #include "ble/BLE.h" 00015 #include "ble/Gap.h" 00016 #include "ble/services/BatteryService.h" 00017 #include "ble/blecommon.h" 00018 #include "UUID.h" 00019 #include "ble/gap/AdvertisingDataTypes.h" 00020 #include "ble/gap/Types.h" 00021 #include "SDBlockDevice.h" 00022 #include "FATFileSystem.h" 00023 #include <errno.h> 00024 #include <stdio.h> 00025 #include <time.h> 00026 #include <stdint.h> 00027 00028 extern char device_id[]; 00029 extern unsigned short int measure_id; 00030 extern unsigned short int log_id; 00031 00032 extern Serial pan1326b; 00033 extern DigitalOut bt_rst; 00034 00035 extern volatile int conn_state; 00036 extern bool in_BT; 00037 00038 extern AnalogIn battery; 00039 00040 extern DigitalOut r; 00041 extern DigitalOut g; 00042 extern DigitalOut b; 00043 extern DigitalOut RGBs[]; 00044 00045 extern MAX32630FTHR pegasus; 00046 00047 extern I2C i2cm2; 00048 extern MAX14690 max14690; 00049 00050 extern InterruptIn button; 00051 00052 extern FATFileSystem fileSystem; 00053 extern SDBlockDevice blockDevice; 00054 00055 extern I2C i2c; 00056 extern int sensor_temp; 00057 extern Adafruit_SSD1306_I2c featherOLED; 00058 00059 extern Timer t; 00060 extern PulseOximeter pox; 00061 extern uint32_t tsLastReport; 00062 00063 extern DigitalIn ain; 00064 extern double anSignal; 00065 extern int bpm; 00066 extern int signal; 00067 extern int ibi; 00068 extern MLX90614 IR_thermometer; 00069 extern float ir_temp; 00070 00071 extern volatile int quit; 00072 extern volatile int ble_return; 00073 extern volatile int conn_state; 00074 extern int counter_ble; 00075 00076 extern bool error_status; 00077 extern bool BT_error; 00078 extern bool shipping; 00079 extern bool pause; 00080 extern bool init_status; 00081 extern bool after_BT; 00082 00083 extern Timeout after_BLE; 00084 extern Timeout turnoff; 00085 extern Timeout flipper; 00086 extern Timeout advertise_cancel; 00087 extern Timeout connected_cancel; 00088 extern Ticker mess_timer; 00089 extern Ticker ticker; 00090 extern time_t now; 00091 extern time_t raw; 00092 00093 extern float buffer_temp; 00094 extern struct tm current; 00095 extern struct tm actual; 00096 00097 extern struct user_config_struct user_config_para; 00098 extern struct tm user_config_time; 00099 00100 extern Timeout done_rcv; 00101 00102 extern int next_state; 00103 00104 extern int unsigned long t_diff; 00105 extern int unsigned long unix_time; 00106 00107 extern int default_advertise_time; 00108 extern int default_connected_time; 00109 00110 extern int alert_count; 00111 00112 extern Timer t; 00113 extern PulseOximeter pox; 00114 extern uint32_t tsLastReport; 00115 00116 int ble_buff_line = 0; 00117 char ble_buff[1024][64]; 00118 00119 char response = '0'; 00120 char messungen[7]; 00121 int messwerte[5000]; 00122 char logs_array[7]; 00123 char seconds[12]; 00124 float min_temp = 0; 00125 float max_temp = 0; 00126 float avr_temp = 0; 00127 unsigned short counter_m = 0; 00128 unsigned short counter_l = 0; 00129 int sendLedCounter = 0; 00130 00131 //const static char* SERVICE_UUID = "32372fb9-5f73-4c32-a17b-25e17f52a99a"; 00132 static uint8_t service_data[16]; 00133 00134 //const static char* CHARACTERISTIC_UUID = "1d6ba3db-d405-4034-96fc-78942ef7075f"; 00135 00136 uint16_t customServiceUUID = 0xA000; 00137 uint16_t readCharUUID = 0xA001; 00138 uint16_t writeCharUUID = 0xA002; 00139 uint16_t battService = 0x180F; 00140 00141 const static char DEVICE_NAME[] = "Cold Chain Logger"; 00142 00143 static const uint16_t uuid16_list[] = {0xFFFF}; 00144 //static const uint8_t advData[26]; 00145 00146 //UUID service_uuid = UUID(SERVICE_UUID); 00147 //UUID characteristic_uuid = UUID(CHARACTERISTIC_UUID); 00148 00149 //Gap::ConnectionParams_t fast; 00150 00151 // Set Up custom Charatiristics 00152 static uint8_t readValue[360]; 00153 //ReadOnlyArrayGattCharacteristic<uint8_t, sizeof(readValue)> readChar(readCharUUID, readValue); 00154 ReadOnlyArrayGattCharacteristic<uint8_t, sizeof(readValue)> readChar(readCharUUID, readValue, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY ,NULL,0); 00155 00156 static uint8_t writeValue[20]; 00157 //WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeValue)> writeChar(writeCharUUID, writeValue); 00158 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeValue)> writeChar(writeCharUUID, writeValue, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE ,NULL,0); 00159 00160 // Set up custom service 00161 GattCharacteristic *characteristics[] = {&readChar, &writeChar}; 00162 GattService customService(customServiceUUID, characteristics, sizeof(characteristics) / sizeof(GattCharacteristic *)); 00163 00164 //Battery service 00165 static uint8_t batteryLevel = 50; 00166 static BatteryService* batteryServicePtr; 00167 00168 void periodicCallback(void) 00169 { 00170 /* 00171 if(error_status == true){ 00172 r = !r; 00173 } 00174 */ 00175 b = !b; 00176 } 00177 00178 void writeLedCallback(void) 00179 {/* 00180 if(error_status == true){ 00181 r = !r; 00182 } 00183 */ 00184 b = !b; 00185 ticker.detach(); 00186 } 00187 00188 void sendLedCallback(void) 00189 {/* 00190 if(error_status == true){ 00191 r = !r; 00192 }*/ 00193 b = !b; 00194 if(sendLedCounter == 2){ 00195 ticker.detach(); 00196 sendLedCounter = 0; 00197 }else{ 00198 sendLedCounter++; 00199 } 00200 } 00201 00202 void clear_buffer(){ 00203 if(response != 's'){ 00204 counter_m = 0; 00205 }else if(response != 'l'){ 00206 counter_l = 0; 00207 } 00208 ble_buff_line = 0; 00209 memset(&ble_buff[0][0], 0, sizeof(ble_buff)); 00210 memset(&readValue[0], 0, sizeof(readValue)); 00211 memset(&writeValue[0], 0, sizeof(writeValue)); 00212 } 00213 00214 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00215 { 00216 printf("\nDisconnection callback\n"); 00217 clear_buffer(); 00218 connected_cancel.detach(); 00219 ticker.attach(periodicCallback, 0.5); 00220 advertise_cancel.attach(&BT_false, user_config_para.advertise); 00221 char string[] = "Bluetooth disconnected"; 00222 write_to_sd_log_single(string); 00223 conn_state = 0; 00224 counter_m = 0; 00225 counter_l = 0; 00226 BLE::Instance(BLE::DEFAULT_INSTANCE).startAdvertising(); 00227 printf("\nAdvertising\n"); 00228 } 00229 00230 void updateSensorValue() { 00231 batteryLevel = (battery.read() * 100 - 47) / 0.3971; 00232 if(batteryLevel > 99.95){ 00233 batteryLevel = 100; 00234 } 00235 batteryServicePtr->updateBatteryLevel(batteryLevel); 00236 } 00237 00238 void printMacAddress() 00239 { 00240 Gap::AddressType_t addr_type; 00241 Gap::Address_t address; 00242 BLE::Instance().gap().getAddress(&addr_type, address); 00243 printf("Device MAC address: "); 00244 for (int i = 5; i >= 1; i--){ 00245 printf("%02x:", address[i]); 00246 } 00247 printf("%02x\r\n", address[0]); 00248 } 00249 00250 void writeCharCallback(const GattWriteCallbackParams *params) 00251 { 00252 printf("\nData received\n"); 00253 b = 1; 00254 ticker.attach(writeLedCallback, 0.5); 00255 memset(&readValue[0], 0, sizeof(readValue)); 00256 memset(&writeValue[0], 0, sizeof(writeValue)); 00257 BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), params->data, params->len); 00258 for(int i = 0; i < 15; i++){ 00259 ble_buff[0][i + ble_buff_line] = readValue[i]; 00260 } 00261 printf("\n%s received (ble_buff)\n", ble_buff[0]); 00262 ble_buff_line = ble_buff_line + 15; 00263 if(readValue[0] > 0x60){ 00264 response = readValue[0]; 00265 } 00266 } 00267 00268 void disconnect(){ 00269 Gap::DisconnectionReason_t res=Gap::LOCAL_HOST_TERMINATED_CONNECTION; 00270 BLE::Instance(BLE::DEFAULT_INSTANCE).gap().disconnect(res); 00271 } 00272 00273 void whenConnected(const Gap::ConnectionCallbackParams_t *) 00274 { 00275 printf("\nConnected\n"); 00276 advertise_cancel.detach(); 00277 ticker.detach(); 00278 connected_cancel.attach(&disconnect, user_config_para.connected); 00279 conn_state = 1; 00280 if(error_status == true){ 00281 r = 1; 00282 } 00283 b = 0; 00284 response = '0'; 00285 char string[] = "Bluetooth connected"; 00286 write_to_sd_log_single(string); 00287 } 00288 00289 void onDataSent(unsigned count) 00290 { 00291 printf("%c", ble_buff[ble_buff_line][count]); 00292 readValue[count] = ble_buff[ble_buff_line][count]; 00293 } 00294 00295 void after_BL(){ 00296 after_BT = false; 00297 after_BLE.detach(); 00298 } 00299 00300 void BT_false() 00301 { 00302 BLE &ble = BLE::Instance(); 00303 in_BT = false; 00304 b = 1; 00305 conn_state = 0; 00306 quit = 0; 00307 clear_buffer(); 00308 ticker.detach(); 00309 advertise_cancel.detach(); 00310 connected_cancel.detach(); 00311 disconnect(); 00312 wait(0.5); 00313 if(error_status == true){ 00314 r = 0; 00315 } 00316 if(shipping == true && pause == false){ 00317 mess_timer.attach(&mess_handler, (float)user_config_para.interval); 00318 } 00319 after_BT = true; 00320 after_BLE.attach(after_BL, 2); 00321 response = '0'; 00322 } 00323 00324 void BT_true() 00325 { 00326 in_BT = true; 00327 next_state = 2; 00328 } 00329 00330 void log_count(){ 00331 fileSystem.unmount(); 00332 fflush(stdout); 00333 00334 fileSystem.mount(&blockDevice); 00335 FILE * log = fopen ("/fs/log.csv", "rb"); 00336 00337 int ch = 0; 00338 int lines = 0; 00339 00340 while(!feof(log)){ 00341 ch = fgetc(log); 00342 if(ch == '\n') 00343 { 00344 lines++; 00345 } 00346 } 00347 00348 log_id = lines; 00349 00350 fclose(log); 00351 fileSystem.unmount(); 00352 00353 sprintf(logs_array,"%d", lines); 00354 } 00355 00356 void messdaten_count(){ 00357 fileSystem.unmount(); 00358 fflush(stdout); 00359 00360 fileSystem.mount(&blockDevice); 00361 FILE * messdaten = fopen ("/fs/messdaten.csv", "rb"); 00362 00363 int ch = 0; 00364 int lines = 0; 00365 00366 while(!feof(messdaten)){ 00367 ch = fgetc(messdaten); 00368 if(ch == '\n') 00369 { 00370 lines++; 00371 } 00372 } 00373 00374 fclose(messdaten); 00375 fileSystem.unmount(); 00376 00377 measure_id = lines; 00378 00379 sprintf(messungen,"%d", lines); 00380 } 00381 00382 void unixtime_to_char_array(){ 00383 tm *current; 00384 time(&now); 00385 current = localtime (&now); 00386 printf ("\nDate: 20%02d-%02d-%02d %02d:%02d:%02d\r\n", current->tm_year - 100, current->tm_mon + 1, current->tm_mday, current->tm_hour, current->tm_min, current->tm_sec); 00387 00388 printf("\nSekunden als Dezimal: %d\n", time(&now)); 00389 00390 sprintf(seconds,"%d", time(&now)); 00391 00392 printf("\nSekunden als String: %s\n", seconds); 00393 } 00394 00395 int array_to_int(int z){ 00396 int zahl[z]; 00397 00398 for(int i = 0; i < z; i++){ 00399 zahl[i] = readValue[i]; 00400 zahl[i] = zahl[i] - 48; 00401 } 00402 00403 unsigned long ganzes = 0; 00404 unsigned long log = 1; 00405 int j = z; 00406 do{ 00407 j--; 00408 ganzes = ganzes + log * zahl[j]; 00409 log = log * 10; 00410 }while(j > 0); 00411 00412 if((int)ganzes < 0){ 00413 ganzes = 0; 00414 } 00415 return ganzes; 00416 } 00417 00418 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00419 { 00420 BLE &ble = params->ble; 00421 ble_error_t error = params->error; 00422 00423 if (error != BLE_ERROR_NONE) { 00424 return; 00425 } 00426 00427 read_id(); 00428 00429 /* Setup advertising */ 00430 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); // BLE only, no classic BT 00431 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); // advertising type 00432 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, (const uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); // add name 00433 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::DEVICE_ID, (uint8_t *)device_id, 5); // add name 00434 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); // UUID's broadcast in advertising packet 00435 ble.gap().setAdvertisingInterval(100); // 100ms. 00436 00437 00438 /* Add custom service */ 00439 ble.gattServer().addService(customService); 00440 00441 /* Add battery service */ 00442 batteryServicePtr = new BatteryService(ble, batteryLevel); 00443 00444 ble.gap().onDisconnection(disconnectionCallback); 00445 ble.gap().onConnection(whenConnected); 00446 ble.gattServer().onDataWritten(writeCharCallback); 00447 ble.gattServer().onDataSent(onDataSent); 00448 00449 /* Start advertising */ 00450 ble.gap().startAdvertising(); 00451 } 00452 00453 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00454 BLE &ble = BLE::Instance(); 00455 updateSensorValue(); 00456 } 00457 00458 void dataSentSend(){ 00459 response = '0'; 00460 connected_cancel.attach(&disconnect, user_config_para.connected); 00461 } 00462 00463 void dataSent(){ 00464 wait(1); 00465 b = 1; 00466 ticker.attach(sendLedCallback, 0.5); 00467 response = '0'; 00468 connected_cancel.attach(&disconnect, user_config_para.connected); 00469 } 00470 00471 void swap(int num1, int num2){ 00472 int temp = messwerte[num1]; 00473 messwerte[num1] = messwerte[num2]; 00474 messwerte[num2] = temp; 00475 } 00476 00477 int partition(int left, int right, int pivot){ 00478 int leftPointer = left - 1; 00479 int rightPointer = right; 00480 00481 while(true){ 00482 while(messwerte[++leftPointer] < pivot){ 00483 //do nothing 00484 } 00485 00486 while(rightPointer > 0 && messwerte[--rightPointer] > pivot){ 00487 //do nothing 00488 } 00489 00490 if(leftPointer >= rightPointer){ 00491 break; 00492 }else{ 00493 swap(leftPointer, rightPointer); 00494 } 00495 } 00496 swap(leftPointer, right); 00497 00498 return leftPointer; 00499 } 00500 00501 void quickSort(int left, int right){ 00502 if(right - left <= 0){ 00503 return; 00504 }else{ 00505 int pivot = messwerte[right]; 00506 int partionPoint = partition(left, right, pivot); 00507 quickSort(left, partionPoint - 1); 00508 quickSort(partionPoint + 1, right); 00509 } 00510 } 00511 00512 void BLE_handler() 00513 { 00514 BLE &ble = BLE::Instance(); 00515 r = 1; 00516 g = 1; 00517 b = 0; 00518 ticker.attach(periodicCallback, 0.5); 00519 advertise_cancel.attach(&BT_false, user_config_para.advertise); 00520 clear_buffer(); 00521 next_state = 0; 00522 printf("\nBLE - ON\n"); 00523 printf("\nAdvertising\n"); 00524 00525 while( quit ) 00526 { 00527 ble.waitForEvent(); 00528 ble.onEventsToProcess(scheduleBleEventsProcessing); 00529 00530 while ( conn_state ) //Abfrage zum Verbindungsstatus 00531 { 00532 ble.waitForEvent(); 00533 ble.onEventsToProcess(scheduleBleEventsProcessing); 00534 00535 switch ( response ) 00536 { 00537 /* 00538 case 's': // Messwerte übertragen 00539 { 00540 connected_cancel.detach(); 00541 clear_buffer(); 00542 00543 unsigned int length = 0; 00544 00545 connected_cancel.attach(&disconnect, user_config_para.connected); 00546 while(readValue[0] == NULL || readValue[0] < 0x31|| readValue[0] > 0x39){ 00547 clear_buffer(); 00548 ble.waitForEvent(); 00549 if(conn_state == 0){ 00550 break; 00551 } 00552 } 00553 connected_cancel.detach(); 00554 if(conn_state == 1){ 00555 int a = 0; 00556 while(readValue[a] != NULL){ 00557 a++; 00558 } 00559 int id = array_to_int(a); 00560 clear_buffer(); 00561 00562 fileSystem.mount(&blockDevice); 00563 FILE * messdaten = fopen ("/fs/messdaten.csv", "rb"); 00564 00565 fseek (messdaten, 0, SEEK_END); 00566 length = ftell (messdaten); 00567 fseek (messdaten, 0, SEEK_SET); 00568 00569 if(id > measure_id){ 00570 printf("\nMeasuremnt ID %d doesn't exist!\n", id); 00571 char string[] = "Call of an inexistent measurement ID"; 00572 write_to_sd_log_single(string); 00573 00574 ble_buff[0][0] = '0'; 00575 onDataSent(0); 00576 }else{ 00577 unsigned long ch = 0; 00578 unsigned long lines = 0; 00579 unsigned long i = 0; 00580 unsigned long j = 1; 00581 unsigned long c = 0; 00582 00583 if(id == 1){ 00584 fseek(messdaten, 0, SEEK_SET); 00585 }else{ 00586 while((id - 1) != lines){ 00587 ch = fgetc(messdaten); 00588 if(ch == '\n'){ 00589 lines++; 00590 } 00591 i++; 00592 } 00593 fseek(messdaten, i, SEEK_SET); 00594 c = i; 00595 } 00596 if(id == measure_id){ 00597 while(fgetc(messdaten) != EOF){ 00598 i++; 00599 j++; 00600 } 00601 }else{ 00602 while(fgetc(messdaten) != '\n'){ 00603 i++; 00604 j++; 00605 } 00606 } 00607 fseek(messdaten, c, SEEK_SET); 00608 fread (ble_buff[ble_buff_line], 1, j, messdaten); 00609 00610 for(int l = 0; l < j; l++){ 00611 onDataSent(l); 00612 } 00613 } 00614 00615 fclose(messdaten); 00616 fileSystem.unmount(); 00617 fflush(stdout); 00618 00619 dataSentSend(); 00620 } 00621 break; 00622 } 00623 */ 00624 00625 case 's': // Messwerte übertragen 00626 { 00627 connected_cancel.detach(); 00628 clear_buffer(); 00629 00630 if(counter_m == 0){ 00631 char string[] = "Command 's' (send measuremnts) received"; 00632 write_to_sd_log_single(string); 00633 } 00634 00635 connected_cancel.attach(&disconnect, user_config_para.connected); 00636 while(readValue[0] == NULL || readValue[0] < 0x31|| readValue[0] > 0x39){ 00637 clear_buffer(); 00638 ble.waitForEvent(); 00639 if(conn_state == 0){ 00640 break; 00641 } 00642 } 00643 connected_cancel.detach(); 00644 if(conn_state == 1){ 00645 00646 if(counter_m == 0){ 00647 char string1[] = "Sending measuremnts..."; 00648 write_to_sd_log_single(string1); 00649 } 00650 00651 int a = 0; 00652 while(readValue[a] != NULL){ 00653 a++; 00654 } 00655 int id = array_to_int(a); 00656 clear_buffer(); 00657 00658 fileSystem.mount(&blockDevice); 00659 FILE * messdaten = fopen ("/fs/messdaten.csv", "rb"); 00660 00661 /* Daten senden */ 00662 00663 if(id > measure_id){ 00664 printf("\nMeasuremnt ID %d doesn't exist!\n", id); 00665 char string[] = "Call of an inexistent measurement ID"; 00666 write_to_sd_log_single(string); 00667 00668 ble_buff[0][0] = '0'; 00669 onDataSent(0); 00670 }else{ 00671 unsigned long ch = 0; 00672 unsigned long lines = 0; 00673 unsigned long i = 0; 00674 unsigned long j = 1; 00675 unsigned long c = 0; 00676 00677 if(id == 1){ 00678 fseek(messdaten, 0, SEEK_SET); 00679 }else{ 00680 while((id - 1) != lines){ 00681 ch = fgetc(messdaten); 00682 if(ch == '\n'){ 00683 lines++; 00684 } 00685 i++; 00686 } 00687 fseek(messdaten, i, SEEK_SET); 00688 c = i; 00689 } 00690 if(id == measure_id){ 00691 while(fgetc(messdaten) != EOF){ 00692 i++; 00693 j++; 00694 } 00695 }else{ 00696 int y = 0; 00697 while(y < 10){ 00698 i++; 00699 j++; 00700 ch = fgetc(messdaten); 00701 if(ch == '\n'){ 00702 y++; 00703 } 00704 if(ch == EOF){ 00705 break; 00706 } 00707 } 00708 i--; 00709 j--; 00710 } 00711 fseek(messdaten, c, SEEK_SET); 00712 fread (ble_buff[ble_buff_line], 1, j, messdaten); 00713 00714 for(int l = 0; l < j; l++){ 00715 onDataSent(l); 00716 } 00717 } 00718 00719 fclose(messdaten); 00720 fileSystem.unmount(); 00721 fflush(stdout); 00722 00723 counter_m += 10; 00724 if(counter_m >= measure_id){ 00725 char string2[] = "Sending measuremnts complete"; 00726 write_to_sd_log_single(string2); 00727 counter_m = 0; 00728 dataSent(); 00729 }else{ 00730 dataSentSend(); 00731 } 00732 } 00733 break; 00734 } 00735 00736 case 'g': //Config senden 00737 { 00738 connected_cancel.detach(); 00739 clear_buffer(); 00740 00741 char string[] = "Command 'g' (send config settings) received"; 00742 write_to_sd_log_single(string); 00743 00744 unsigned int length = 0; 00745 00746 char string_1[] = "Sending config.."; 00747 write_to_sd_log_single(string_1); 00748 00749 fileSystem.mount(&blockDevice); 00750 FILE * user_config = fopen ("/fs/user_config.csv", "rb"); 00751 00752 fseek (user_config, 0, SEEK_END); 00753 length = ftell (user_config); 00754 fseek (user_config, 0, SEEK_SET); 00755 00756 fread (ble_buff, 1, length, user_config); 00757 00758 fclose(user_config); 00759 fileSystem.unmount(); 00760 00761 fflush(stdout); 00762 00763 for(int i = 0; i < length; i++){ 00764 onDataSent(i); 00765 } 00766 00767 char string_2[] = "Sending config complete"; 00768 write_to_sd_log_single(string_2); 00769 00770 dataSent(); 00771 00772 break; 00773 } 00774 00775 case 'c': // Config auf Werkeinstellungen zurücksetzen 00776 { 00777 connected_cancel.detach(); 00778 clear_buffer(); 00779 00780 char string[] = "Command 'c' (reset config to standard) received"; 00781 write_to_sd_log_single(string); 00782 00783 if(load_standard_config() == 1){ 00784 char string[] = "Config reseted to factory settings"; 00785 write_to_sd_log_single(string); 00786 00787 ble_buff[0][0] = '1'; 00788 onDataSent(0); 00789 }else{ 00790 char string[] = "Failed to reset Config to factory settings"; 00791 write_to_sd_log_single(string); 00792 00793 ble_buff[0][0] = '0'; 00794 onDataSent(0); 00795 } 00796 00797 dataSent(); 00798 00799 break; 00800 } 00801 00802 case 'd': // Messwerte löschen 00803 { 00804 connected_cancel.detach(); 00805 clear_buffer(); 00806 00807 char string[] = "Command 'd' (delete measurement data) received"; 00808 write_to_sd_log_single(string); 00809 00810 if(delete_file_messdaten() == 1){ 00811 char string_1[] = "Measuerements deleted succesfully"; 00812 write_to_sd_log_single(string_1); 00813 00814 ble_buff[0][0] = '1'; 00815 onDataSent(0); 00816 }else{ 00817 char string_1[] = "Measuerements data delete failed"; 00818 write_to_sd_log_single(string_1); 00819 00820 ble_buff[0][0] = '0'; 00821 onDataSent(0); 00822 } 00823 00824 dataSent(); 00825 00826 break; 00827 } 00828 /* 00829 case 'l': //Log-Datei übertragen 00830 { 00831 00832 connected_cancel.detach(); 00833 clear_buffer(); 00834 00835 unsigned int length = 0; 00836 00837 connected_cancel.attach(&disconnect, user_config_para.connected); 00838 while(readValue[0] == NULL || readValue[0] < 0x31|| readValue[0] > 0x39){ 00839 clear_buffer(); 00840 ble.waitForEvent(); 00841 if(conn_state == 0){ 00842 break; 00843 } 00844 } 00845 connected_cancel.detach(); 00846 if(conn_state == 1){ 00847 int a = 0; 00848 while(readValue[a] != NULL){ 00849 a++; 00850 } 00851 int id = array_to_int(a); 00852 clear_buffer(); 00853 00854 fileSystem.mount(&blockDevice); 00855 FILE * log = fopen ("/fs/log.csv", "rb"); 00856 00857 fseek (log, 0, SEEK_END); 00858 length = ftell (log); 00859 fseek (log, 0, SEEK_SET); 00860 00861 00862 00863 if(id > log_id){ 00864 printf("\nLog ID %d doesn't exist!\n", id); 00865 char string[] = "Call of an inexistent log ID"; 00866 write_to_sd_log_single(string); 00867 00868 ble_buff[0][0] = '0'; 00869 onDataSent(0); 00870 }else{ 00871 unsigned long ch = 0; 00872 unsigned long lines = 0; 00873 unsigned long i = 0; 00874 unsigned long j = 1; 00875 unsigned long c = 0; 00876 00877 if(id == 1){ 00878 fseek(log, 0, SEEK_SET); 00879 }else{ 00880 while((id - 1) != lines){ 00881 ch = fgetc(log); 00882 if(ch == '\n'){ 00883 lines++; 00884 } 00885 i++; 00886 } 00887 fseek(log, i, SEEK_SET); 00888 c = i; 00889 } 00890 if(id == log_id){ 00891 while(fgetc(log) != EOF){ 00892 i++; 00893 j++; 00894 } 00895 }else{ 00896 while(fgetc(log) != '\n'){ 00897 i++; 00898 j++; 00899 } 00900 } 00901 fseek(log, c, SEEK_SET); 00902 fread (ble_buff[ble_buff_line], 1, j, log); 00903 00904 for(int l = 0; l < j; l++){ 00905 onDataSent(l); 00906 } 00907 } 00908 00909 fclose(log); 00910 fileSystem.unmount(); 00911 fflush(stdout); 00912 00913 dataSentSend(); 00914 } 00915 break; 00916 } 00917 */ 00918 00919 case 'l': //Log-Datei übertragen 00920 { 00921 connected_cancel.detach(); 00922 clear_buffer(); 00923 00924 if(counter_l == 0){ 00925 char string[] = "Command 'l' (send logs) received"; 00926 write_to_sd_log_single(string); 00927 } 00928 00929 connected_cancel.attach(&disconnect, user_config_para.connected); 00930 while(readValue[0] == NULL || readValue[0] < 0x31|| readValue[0] > 0x39){ 00931 clear_buffer(); 00932 ble.waitForEvent(); 00933 if(conn_state == 0){ 00934 break; 00935 } 00936 } 00937 connected_cancel.detach(); 00938 if(conn_state == 1){ 00939 00940 if(counter_l == 0){ 00941 char string1[] = "Sending logs..."; 00942 write_to_sd_log_single(string1); 00943 } 00944 00945 int a = 0; 00946 while(readValue[a] != NULL){ 00947 a++; 00948 } 00949 int id = array_to_int(a); 00950 clear_buffer(); 00951 00952 fileSystem.mount(&blockDevice); 00953 FILE * log = fopen ("/fs/log.csv", "rb"); 00954 00955 /* Daten senden */ 00956 00957 if(id > log_id){ 00958 printf("\nLog ID %d doesn't exist!\n", id); 00959 char string[] = "Call of an inexistent log ID"; 00960 write_to_sd_log_single(string); 00961 00962 ble_buff[0][0] = '0'; 00963 onDataSent(0); 00964 }else{ 00965 unsigned long ch = 0; 00966 unsigned long lines = 0; 00967 unsigned long i = 0; 00968 unsigned long j = 1; 00969 unsigned long c = 0; 00970 00971 if(id == 1){ 00972 fseek(log, 0, SEEK_SET); 00973 }else{ 00974 while((id - 1) != lines){ 00975 ch = fgetc(log); 00976 if(ch == '\n'){ 00977 lines++; 00978 } 00979 i++; 00980 } 00981 fseek(log, i, SEEK_SET); 00982 c = i; 00983 } 00984 if(id == log_id){ 00985 while(fgetc(log) != EOF){ 00986 i++; 00987 j++; 00988 } 00989 }else{ 00990 int y = 0; 00991 while(y < 5){ 00992 i++; 00993 j++; 00994 ch = fgetc(log); 00995 if(ch == '\n'){ 00996 y++; 00997 } 00998 if(ch == EOF){ 00999 break; 01000 } 01001 } 01002 i--; 01003 j--; 01004 } 01005 fseek(log, c, SEEK_SET); 01006 fread (ble_buff[ble_buff_line], 1, j, log); 01007 01008 for(int l = 0; l < j; l++){ 01009 onDataSent(l); 01010 } 01011 } 01012 01013 fclose(log); 01014 fileSystem.unmount(); 01015 fflush(stdout); 01016 01017 counter_l += 5; 01018 if(counter_l >= measure_id){ 01019 char string2[] = "Sending logs complete"; 01020 write_to_sd_log_single(string2); 01021 counter_l = 0; 01022 dataSent(); 01023 }else{ 01024 dataSentSend(); 01025 } 01026 } 01027 break; 01028 } 01029 01030 case 'r': // Log Datei löschen 01031 { 01032 connected_cancel.detach(); 01033 clear_buffer(); 01034 01035 char string[] = "Command 'r' (delete log file) received"; 01036 write_to_sd_log_single(string); 01037 01038 if(delete_file_log() == 1){ 01039 char string_1[] = "Log file deleted"; 01040 write_to_sd_log_single(string_1); 01041 01042 ble_buff[0][0] = '1'; 01043 onDataSent(0); 01044 }else{ 01045 char string_1[] = "Log file delete failed"; 01046 write_to_sd_log_single(string_1); 01047 01048 ble_buff[0][0] = '0'; 01049 onDataSent(0); 01050 } 01051 01052 dataSent(); 01053 01054 break; 01055 } 01056 01057 case 'n': //Reset 01058 { 01059 connected_cancel.detach(); 01060 clear_buffer(); 01061 01062 char string[] = "Command 'n' (reboot) received"; 01063 write_to_sd_log_single(string); 01064 BT_false(); 01065 wait(0.5); 01066 reboot(); 01067 break; 01068 } 01069 01070 case 'w': // Werkeinstellungen 01071 { 01072 connected_cancel.detach(); 01073 clear_buffer(); 01074 01075 char string[] = "Command 'w' (reset to factory settings) received"; 01076 write_to_sd_log_single(string); 01077 01078 if(delete_file_messdaten() && load_standard_config() && delete_file_log() == 1){ 01079 char string_1[] = "System reseted to factory settings"; 01080 write_to_sd_log_single(string_1); 01081 01082 ble_buff[0][0] = '1'; 01083 onDataSent(0); 01084 01085 printf("\n__________________________\n\nColdchainlogger reseted \nto factory settings\n__________________________\n\n"); 01086 }else{ 01087 char string_1[] = "System reset to factory settings failed"; 01088 write_to_sd_log_single(string_1); 01089 01090 ble_buff[0][0] = '0'; 01091 onDataSent(0); 01092 } 01093 01094 dataSent(); 01095 01096 break; 01097 } 01098 01099 case 'a': // Datenaufnahme pausieren / stoppen 01100 { 01101 connected_cancel.detach(); 01102 clear_buffer(); 01103 01104 char string[] = "Command 'a' (pause / stop measurement) received"; 01105 write_to_sd_log_single(string); 01106 01107 mess_timer.detach(); 01108 next_state = 0; 01109 01110 if(pause == true){ 01111 char string_1[] = "Measurement stopped"; 01112 write_to_sd_log_single(string_1); 01113 printf("\nMeasurement stopped\n"); 01114 shipping = false; 01115 01116 ble_buff[0][0] = '0'; 01117 onDataSent(0); 01118 }else{ 01119 pause = true; 01120 char string_1[] = "Measurement paused"; 01121 write_to_sd_log_single(string_1); 01122 printf("\nMeasurement puased\n"); 01123 01124 ble_buff[0][0] = '1'; 01125 onDataSent(0); 01126 } 01127 01128 mess_timer.detach(); 01129 01130 dataSent(); 01131 01132 break; 01133 } 01134 01135 case 'f': // Datenaufnahme starten / fortsetzen 01136 { 01137 connected_cancel.detach(); 01138 clear_buffer(); 01139 01140 char string[] = "Command 'f' (start / continue measurement) received"; 01141 write_to_sd_log_single(string); 01142 01143 if(shipping == false){ 01144 char string_1[] = "Measurement started"; 01145 write_to_sd_log_single(string_1); 01146 shipping = true; 01147 pause = false; 01148 01149 if(user_config_para.wait_mode == 1){ 01150 next_state = 3; 01151 }else{ 01152 next_state = 1; 01153 } 01154 printf("\nMeasurement started\n"); 01155 01156 ble_buff[0][0] = '1'; 01157 onDataSent(0); 01158 }else{ 01159 next_state = 1; 01160 char string_1[] = "Measurement continued"; 01161 write_to_sd_log_single(string_1); 01162 printf("\nMeasurement continued\n"); 01163 pause = false; 01164 01165 ble_buff[0][0] = '0'; 01166 onDataSent(0); 01167 } 01168 01169 dataSent(); 01170 01171 break; 01172 } 01173 01174 case 'e': //Config erhalten 01175 { 01176 connected_cancel.detach(); 01177 clear_buffer(); 01178 01179 char string[] = "Command 'e' (receive and overwrite config) received"; 01180 write_to_sd_log_single(string); 01181 01182 connected_cancel.attach(&disconnect, user_config_para.connected); 01183 printf("\nReady to receive new config settings\n"); 01184 01185 while(readValue[0] == NULL){ 01186 ble.waitForEvent(); 01187 if(conn_state == 0){ 01188 break; 01189 } 01190 } 01191 memset(&readValue[0], 0, sizeof(readValue)); 01192 memset(&writeValue[0], 0, sizeof(writeValue)); 01193 while(readValue[0] == NULL){ 01194 ble.waitForEvent(); 01195 if(conn_state == 0){ 01196 break; 01197 } 01198 } 01199 connected_cancel.detach(); 01200 if(conn_state == 1){ 01201 char params[50]; 01202 printf("\n%s\n", ble_buff[0]); 01203 strcpy(params, ble_buff[0]); 01204 01205 if(create_user_config(params) == 1){ 01206 clear_buffer(); 01207 01208 char string_1[] = "User config changed"; 01209 write_to_sd_log_single(string_1); 01210 printf("\n__________________________\n\nUser config changed\n__________________________\n\n"); 01211 01212 ble_buff[0][0] = '1'; 01213 onDataSent(0); 01214 }else{ 01215 clear_buffer(); 01216 01217 char string_1[] = "Changing user config failed"; 01218 write_to_sd_log_single(string_1); 01219 printf("\n__________________________\n\n!!! Error while changing the user config !!!\n__________________________\n\n"); 01220 01221 ble_buff[0][0] = '0'; 01222 onDataSent(0); 01223 } 01224 01225 dataSent(); 01226 } 01227 break; 01228 } 01229 01230 case 'x': // Verbindung beenden 01231 { 01232 connected_cancel.detach(); 01233 clear_buffer(); 01234 01235 char string[] = "Command 'x' (shut down bluetooth) received"; 01236 write_to_sd_log_single(string); 01237 01238 BT_false(); 01239 char string_1[] = "Bluetooth shuted down"; 01240 write_to_sd_log_single(string_1); 01241 printf("\n\nBluetooth shuted down\n\n"); 01242 01243 response = '0'; 01244 connected_cancel.attach(&disconnect, user_config_para.connected); 01245 01246 break; 01247 } 01248 01249 case 'b': //Akku-Zustand 01250 { 01251 connected_cancel.detach(); 01252 clear_buffer(); 01253 01254 char string[] = "Command 'b' (send battery info) received"; 01255 write_to_sd_log_single(string); 01256 01257 updateSensorValue(); 01258 int battery = batteryLevel; 01259 sprintf(ble_buff[0],"%d", battery); 01260 for(int i = 0; i < 2; i++){ 01261 onDataSent(i); 01262 } 01263 printf("\nBattery level: %d %%\n", battery); 01264 01265 dataSent(); 01266 01267 break; 01268 } 01269 01270 case 'm': //Speicher-Belegung 01271 { 01272 connected_cancel.detach(); 01273 clear_buffer(); 01274 01275 char string[] = "Command 'm' (send memmory info) received"; 01276 write_to_sd_log_single(string); 01277 01278 printf("\nTotal SD memory: %llu Bytes\n", blockDevice.size()); 01279 01280 unsigned long long length = 0; 01281 01282 //ID Größe 01283 fileSystem.mount(&blockDevice); 01284 FILE * id = fopen ("/fs/id.csv", "rb"); 01285 01286 fseek (id, 0, SEEK_END); 01287 length = ftell (id); 01288 fseek (id, 0, SEEK_SET); 01289 01290 fclose(id); 01291 fileSystem.unmount(); 01292 fflush(stdout); 01293 01294 unsigned long long idn = length; 01295 length = 0; 01296 01297 //Log Größe 01298 fileSystem.mount(&blockDevice); 01299 FILE * log = fopen ("/fs/log.csv", "rb"); 01300 01301 fseek (log, 0, SEEK_END); 01302 length = ftell (log); 01303 fseek (log, 0, SEEK_SET); 01304 01305 fclose(log); 01306 fileSystem.unmount(); 01307 fflush(stdout); 01308 01309 unsigned long long logs = length; 01310 length = 0; 01311 01312 //Messdaten Größe 01313 fileSystem.mount(&blockDevice); 01314 FILE * messdaten = fopen ("/fs/messdaten.csv", "rb"); 01315 01316 fseek (messdaten, 0, SEEK_END); 01317 length = ftell (messdaten); 01318 fseek (messdaten, 0, SEEK_SET); 01319 01320 fclose(messdaten); 01321 fileSystem.unmount(); 01322 fflush(stdout); 01323 01324 unsigned long long mes = length; 01325 length = 0; 01326 01327 //Standard Config Größe 01328 fileSystem.mount(&blockDevice); 01329 FILE * stcf = fopen ("/fs/standard_config.csv", "rb"); 01330 01331 fseek (stcf, 0, SEEK_END); 01332 length = ftell (stcf); 01333 fseek (stcf, 0, SEEK_SET); 01334 01335 fclose(stcf); 01336 fileSystem.unmount(); 01337 fflush(stdout); 01338 01339 unsigned long long stcfg = length; 01340 length = 0; 01341 01342 //User Config Größe 01343 fileSystem.mount(&blockDevice); 01344 FILE * uscf = fopen ("/fs/user_config.csv", "rb"); 01345 01346 fseek (uscf, 0, SEEK_END); 01347 length = ftell (uscf); 01348 fseek (uscf, 0, SEEK_SET); 01349 01350 fclose(uscf); 01351 fileSystem.unmount(); 01352 fflush(stdout); 01353 01354 unsigned long long uscfg = length; 01355 length = 0; 01356 01357 printf("\nID size: %llu Bytes\n", idn); 01358 printf("Logs size: %llu Bytes\n", logs); 01359 printf("Standard config size: %llu Bytes\n", stcfg); 01360 printf("User config size: %llu Bytes\n\n", uscfg); 01361 01362 unsigned long long not_measuremnts = idn + logs + stcfg + uscfg; 01363 unsigned long long actual_size = blockDevice.size() - not_measuremnts; 01364 printf("Available SD memory (100 %%) = total SD memory - configs & id (%llu Bytes) - logs\n", not_measuremnts); 01365 printf("Available SD memory (100 %%): %llu Bytes\n\n", actual_size); 01366 01367 messdaten_count(); 01368 printf("Measuremnts count: %s\n", messungen); 01369 printf("Measurements size: %llu Bytes\n\n", mes); 01370 01371 double hundert = 100; 01372 double occupied = hundert / actual_size * mes; 01373 printf("Occupied memory: %f %%\n", occupied); 01374 01375 double free_mem = 100 - occupied; 01376 printf("Available memory: %f %%\n", free_mem); 01377 01378 char occ[3]; 01379 char free[3]; 01380 01381 if((int)occupied != 0){ 01382 occupied = ceil( occupied * 100.0 ) / 100.0; 01383 } 01384 if((int)free_mem != 0){ 01385 free_mem = ceil( free_mem * 100.0 ) / 100.0; 01386 } 01387 01388 sprintf(occ,"%d", (int)occupied); 01389 sprintf(free,"%d", (int)free_mem); 01390 01391 printf("OCC: %s\n", occ); 01392 printf("FREE: %s\n", free); 01393 01394 messdaten_count(); 01395 01396 int i = 0; 01397 while(i < messungen[i] != NULL){ 01398 ble_buff[0][i] = messungen[i]; 01399 onDataSent(i); 01400 i++; 01401 } 01402 ble_buff[0][i] = 0x3B; 01403 onDataSent(i); 01404 i++; 01405 int j = 0; 01406 while(j < occ[j] != NULL){ 01407 ble_buff[0][i] = occ[j]; 01408 onDataSent(i); 01409 i++; 01410 j++; 01411 } 01412 ble_buff[0][i] = 0x3B; 01413 onDataSent(i); 01414 i++; 01415 int c = 0; 01416 while(c < free[c] != NULL){ 01417 ble_buff[0][i] = free[c]; 01418 onDataSent(i); 01419 i++; 01420 c++; 01421 } 01422 ble_buff[0][i] = 0x3B; 01423 onDataSent(i); 01424 i++; 01425 dataSent(); 01426 01427 break; 01428 } 01429 01430 case 'i': //ID auslesen 01431 { 01432 connected_cancel.detach(); 01433 clear_buffer(); 01434 01435 char string[] = "Command 'i' (send ID) received"; 01436 write_to_sd_log_single(string); 01437 01438 char string_1[] = "Sending device ID..."; 01439 write_to_sd_log_single(string_1); 01440 01441 printf("\nDevice ID: %s\n", device_id); 01442 01443 int j = 0; 01444 while(device_id[j] != NULL){ 01445 ble_buff[0][j] = device_id[j]; 01446 j++; 01447 } 01448 01449 int i = 0; 01450 while(device_id[i] != NULL){ 01451 onDataSent(i); 01452 i++; 01453 } 01454 01455 char string_2[] = "Sending device ID complete"; 01456 write_to_sd_log_single(string_2); 01457 01458 dataSent(); 01459 01460 break; 01461 } 01462 01463 case 'z': //Hardware Module abfragen 01464 { 01465 connected_cancel.detach(); 01466 clear_buffer(); 01467 01468 char string[] = "Command 'z' (send connected devices) received"; 01469 write_to_sd_log_single(string); 01470 01471 dataSent(); 01472 01473 break; 01474 } 01475 01476 case 'v': // Zeit in Unixformat von Datenlogger abfragen 01477 { 01478 connected_cancel.detach(); 01479 clear_buffer(); 01480 01481 char string[] = "Command 'v' (send device time) received"; 01482 write_to_sd_log_single(string); 01483 01484 unixtime_to_char_array(); 01485 for(int i = 0; i < sizeof(seconds); i++){ 01486 ble_buff[0][i] = seconds[i]; 01487 onDataSent(i); 01488 } 01489 01490 dataSent(); 01491 01492 break; 01493 } 01494 01495 case 't': //Zeit in Unixformat an Datenlogger schicken 01496 { 01497 connected_cancel.detach(); 01498 clear_buffer(); 01499 01500 char string[] = "Command 't' (receive and set device time) received"; 01501 write_to_sd_log_single(string); 01502 01503 connected_cancel.attach(&disconnect, user_config_para.connected); 01504 printf("\nReady to receive new time settings\n"); 01505 while(readValue[0] == NULL || readValue[0] < 0x31|| readValue[0] > 0x39){ 01506 clear_buffer(); 01507 ble.waitForEvent(); 01508 if(conn_state == 0){ 01509 break; 01510 } 01511 } 01512 connected_cancel.detach(); 01513 if(conn_state == 1){ 01514 int a = 0; 01515 while(readValue[a] != NULL){ 01516 a++; 01517 } 01518 int new_time = array_to_int(a); 01519 clear_buffer(); 01520 01521 if(set_app_time(new_time) == 1){ 01522 ble_buff[0][0] = '1'; 01523 onDataSent(0); 01524 }else{ 01525 ble_buff[0][0] = '0'; 01526 onDataSent(0); 01527 } 01528 01529 dataSent(); 01530 } 01531 break; 01532 } 01533 01534 case 'q': //Min- / Max- / Mittelwert schicken 01535 { 01536 connected_cancel.detach(); 01537 clear_buffer(); 01538 01539 char string[] = "Command 'q' (send min/max/average) received"; 01540 write_to_sd_log_single(string); 01541 01542 //wait(1); 01543 //min_max_avr(); 01544 01545 connected_cancel.detach(); 01546 clear_buffer(); 01547 01548 unsigned int length = 0; 01549 int a = 0; 01550 01551 char string_1[] = "Sending min/max/average.."; 01552 write_to_sd_log_single(string_1); 01553 01554 fileSystem.unmount(); 01555 fflush(stdout); 01556 01557 fileSystem.mount(&blockDevice); 01558 FILE * messdaten = fopen ("/fs/messdaten.csv", "rb"); 01559 01560 fseek (messdaten, 0, SEEK_END); 01561 length = ftell (messdaten); 01562 fseek (messdaten, 0, SEEK_SET); 01563 01564 /* Daten senden */ 01565 01566 unsigned long ch = 0; 01567 unsigned short lines = 0; 01568 unsigned long i = 0; 01569 unsigned long j = 1; 01570 unsigned long c = 0; 01571 unsigned long e = 0; 01572 01573 while(!feof(messdaten)){ 01574 ch = fgetc(messdaten); 01575 if(ch == '\n') 01576 { 01577 lines++; 01578 } 01579 } 01580 01581 printf("\n%d Eintraege\n", lines); 01582 01583 for(int a = 0; a < lines; a++) 01584 { 01585 messwerte[a] = *new int[8]; 01586 //printf("\nTEST 1\n"); 01587 } 01588 01589 char temp[8]; 01590 01591 fseek(messdaten, 0, SEEK_SET); 01592 while(!feof(messdaten)){ 01593 ch = fgetc(messdaten); 01594 if(ch == '\n'){ 01595 fseek(messdaten, i - 7, SEEK_SET); 01596 fread (temp, 1, 7, messdaten); 01597 messwerte[e] = atoi(temp); 01598 memset(&temp[0], 0, sizeof(temp)); 01599 e++; 01600 }else if(ch == EOF){ 01601 fseek(messdaten, i - 8, SEEK_SET); 01602 fread (temp, 1, 8, messdaten); 01603 messwerte[e] = atoi(temp); 01604 memset(&temp[0], 0, sizeof(temp)); 01605 } 01606 //printf("\nTEST 3\n"); 01607 i++; 01608 } 01609 01610 unsigned long summe = 0; 01611 01612 for(int q = 1; q < e; q++){ 01613 summe += messwerte[q]; 01614 } 01615 printf("\nSumme: %lu\n", summe); 01616 01617 double avr = summe / lines; 01618 01619 fclose(messdaten); 01620 fileSystem.unmount(); 01621 fflush(stdout); 01622 01623 char string_2[] = "Sending min/max/average complete"; 01624 write_to_sd_log_single(string_2); 01625 01626 quickSort(1, lines); 01627 01628 if((int)avr != 0){ 01629 avr = ceil( avr * 100.0 ) / 100.0; 01630 } 01631 01632 char min[5]; 01633 char max[5]; 01634 char durch[5]; 01635 01636 sprintf(min,"%d", messwerte[1]); 01637 sprintf(max,"%d", messwerte[lines]); 01638 sprintf(durch,"%d", (int)avr); 01639 01640 printf("\n%s, %s, %s\n", min, max, durch); 01641 01642 int k = 0; 01643 while(k < min[k] != NULL){ 01644 ble_buff[0][k] = min[k]; 01645 onDataSent(k); 01646 k++; 01647 } 01648 ble_buff[0][k] = 0x3B; 01649 onDataSent(k); 01650 k++; 01651 int p = 0; 01652 while(p < max[p] != NULL){ 01653 ble_buff[0][k] = max[p]; 01654 onDataSent(k); 01655 k++; 01656 p++; 01657 } 01658 ble_buff[0][k] = 0x3B; 01659 onDataSent(k); 01660 k++; 01661 int z = 0; 01662 while(z < durch[z] != NULL){ 01663 ble_buff[0][k] = durch[z]; 01664 onDataSent(k); 01665 k++; 01666 z++; 01667 } 01668 ble_buff[0][k] = 0x3B; 01669 onDataSent(k); 01670 k++; 01671 01672 dataSent(); 01673 01674 //printf("\nTEST 6\n"); 01675 01676 break; 01677 } 01678 01679 case 'j': //Anzahl der Messungen schicken 01680 { 01681 connected_cancel.detach(); 01682 clear_buffer(); 01683 01684 char string[] = "Command 'j' (send measurements count) received"; 01685 write_to_sd_log_single(string); 01686 01687 //Anzahl der Messdaten 01688 messdaten_count(); 01689 for(int i = 0; i < sizeof(messungen); i++){ 01690 ble_buff[0][i] = messungen[i]; 01691 onDataSent(i); 01692 } 01693 printf("\nMeasurements count: %s\n", messungen); 01694 01695 dataSent(); 01696 01697 break; 01698 } 01699 01700 case 'h': //Anzahl der Logs schicken 01701 { 01702 connected_cancel.detach(); 01703 clear_buffer(); 01704 01705 char string[] = "Command 'h' (send log count) received"; 01706 write_to_sd_log_single(string); 01707 01708 //Anzahl der Logs 01709 log_count(); 01710 for(int i = 0; i < sizeof(logs_array); i++){ 01711 ble_buff[0][i] = logs_array[i]; 01712 onDataSent(i); 01713 } 01714 printf("\nLogs count: %s\n", logs_array); 01715 01716 dataSent(); 01717 01718 break; 01719 } 01720 01721 case 'y': //Alarn AN / AUS - Anzahl der Meldungen 01722 { 01723 connected_cancel.detach(); 01724 clear_buffer(); 01725 01726 char string[] = "Command 'y' (Alert function and count) received"; 01727 write_to_sd_log_single(string); 01728 01729 if(user_config_para.alert == 1){ 01730 printf("\nAlert function - ON\n"); 01731 01732 printf("\nNumber of alerts: %d\n", alert_count); 01733 char alerts[4]; 01734 sprintf(alerts, "%d", alert_count); 01735 for(int i = 0; i < 4; i++){ 01736 ble_buff[0][i] = alerts[i]; 01737 onDataSent(i); 01738 } 01739 }else{ 01740 printf("\nAlert function - OFF\n"); 01741 01742 ble_buff[0][0] = '0'; 01743 onDataSent(0); 01744 } 01745 01746 dataSent(); 01747 01748 break; 01749 } 01750 01751 case 'k': //Initialisierungsstatus auslesen 01752 { 01753 connected_cancel.detach(); 01754 clear_buffer(); 01755 01756 char string[] = "Command 'b' (send initialize status) received"; 01757 write_to_sd_log_single(string); 01758 01759 if(init_status == true){ 01760 ble_buff[0][0] = '1'; 01761 onDataSent(0); 01762 01763 printf("\nInitialaze status: OK\n"); 01764 }else{ 01765 ble_buff[0][0] = '0'; 01766 onDataSent(0); 01767 01768 printf("\nInitialaze status: FAILED\n"); 01769 } 01770 01771 dataSent(); 01772 01773 break; 01774 } 01775 01776 case 'p': //Mess-Status auslesen 01777 { 01778 connected_cancel.detach(); 01779 clear_buffer(); 01780 01781 char string[] = "Command 'p' (send measurement status) received"; 01782 write_to_sd_log_single(string); 01783 01784 if(shipping == true){ 01785 ble_buff[0][0] = 1; 01786 onDataSent(0); 01787 01788 wait(1); 01789 clear_buffer(); 01790 01791 if(pause == false){ 01792 ble_buff[0][0] = '1'; 01793 onDataSent(0); 01794 01795 printf("\nMeasuremt status = Launched\n"); 01796 }else{ 01797 ble_buff[0][0] = '0'; 01798 onDataSent(0); 01799 01800 printf("\nMeasuremt status = Paused\n"); 01801 } 01802 01803 }else{ 01804 ble_buff[0][0] = '0'; 01805 onDataSent(0); 01806 01807 printf("\nMeasuremt status = Sttopped\n"); 01808 } 01809 01810 dataSent(); 01811 01812 break; 01813 } 01814 01815 default: { 01816 break; 01817 } 01818 } 01819 } 01820 } 01821 }
Generated on Wed Jul 27 2022 23:42:13 by
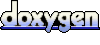