Basic 3D graphics for the MBED application-shield on-board LCD (initial/incomplete).
Embed:
(wiki syntax)
Show/hide line numbers
gfx3d.h
00001 /* 00002 * gfx3d.h -- basic 3D graphics 00003 * Copyright (C) 2015 Fred Barnes, University of Kent <frmb@kent.ac.uk> 00004 */ 00005 00006 00007 #ifndef __GFX3D_H 00008 #define __GFX3D_H 00009 00010 #include "C12832.h" 00011 #include "g3d_sintab.h " 00012 00013 /* NOTE: this assumes an ARM Cortex-M4 ish FPU, but might work elsewhere */ 00014 00015 /* some basic types */ 00016 00017 /** g3d_p3_t 3D single-precision point */ 00018 typedef struct { 00019 float x, y, z; 00020 } g3d_p3_t; 00021 00022 /** g3d_2p3_t 2D integer point with original Z scaled */ 00023 typedef struct { 00024 int16_t x, y; 00025 int16_t z; 00026 int16_t dummy; /* fill out to 64 bits */ 00027 } __attribute__ ((packed)) g3d_2p3_t; 00028 00029 #define G3D_MAX_POLY_POINTS (3) 00030 00031 /** g3d_poly_t 2D integer triangular polygon, original Z scaled */ 00032 typedef struct { 00033 g3d_2p3_t pts[G3D_MAX_POLY_POINTS]; 00034 uint16_t tx_pts[G3D_MAX_POLY_POINTS]; /* texture co-ords are 0xYYXX */ 00035 int32_t norm; /** face normal */ 00036 uint8_t *txptr; /** texture pointer (null if unset) */ 00037 } g3d_poly_t; 00038 00039 /** g3d_edgebuf_t polygon edge-buffer */ 00040 typedef struct { 00041 uint8_t start[64]; 00042 uint8_t end[64]; 00043 uint16_t zstart[64]; 00044 uint16_t zend[64]; 00045 uint16_t xoff, s_end; 00046 } g3d_edgebuf_t; 00047 00048 /** g3d_polyscan_t polygon scan-line type */ 00049 typedef struct { 00050 uint8_t scans[32][2]; /* pairs of X-start X-end for each line of the display */ 00051 int32_t zscan[32][2]; /* similar, but with Z-depth information */ 00052 int scan_s, scan_e; /* scan start and end indices */ 00053 int32_t norm; /* calculated normal for the polygon (back-face cull) */ 00054 } g3d_polyscan_t; 00055 00056 /** angle_t Type for angle (0-255 in unsigned char) */ 00057 typedef uint8_t angle_t; /* working in a coarsely grained world: circle is 256 degrees */ 00058 00059 /** g3d_cubepnts A set of 8 points representing a cube */ 00060 static const g3d_p3_t g3d_cubepnts[] = {{-1.0, -1.0, -1.0}, {1.0, -1.0, -1.0}, {1.0, 1.0, -1.0}, {-1.0, 1.0, -1.0}, 00061 {-1.0, -1.0, 1.0}, {1.0, -1.0, 1.0}, {1.0, 1.0, 1.0}, {-1.0, 1.0, 1.0}}; 00062 00063 /** g3d_xyfacepnts A set of 4 points representing a square in X-Y */ 00064 static const g3d_p3_t g3d_xyfacepnts[] = {{-1.0, -1.0, 0.0}, {1.0, -1.0, 0.0}, {1.0, 1.0, 0.0}, {-1.0, 1.0, 0.0}}; 00065 00066 /* some constants that describe the render target layout -- for the 128x32 LCD */ 00067 #define G3D_X2_SHIFT (64) 00068 #define G3D_Y2_SHIFT (16) 00069 00070 #define G3D_ZBADD (32.0f) 00071 #define G3D_ZBSCALE (256.0f) 00072 00073 #define G3D_Z_DEPTH_MIN (6.0f) 00074 #define G3D_Z_DEPTH_MAX (64.0f) 00075 #define G3D_X_SCALE_MIN (32.0f) 00076 #define G3D_X_SCALE_MAX (512.0f) 00077 #define G3D_Y_SCALE_MIN (32.0f) 00078 #define G3D_Y_SCALE_MAX (512.f) 00079 00080 #define G3D_Z_DEPTH (12.0f) 00081 #define G3D_X_SCALE (120.0f) 00082 #define G3D_Y_SCALE (80.0f) 00083 00084 extern "C" void gfx3d_set_z_depth (const float zd); 00085 00086 extern "C" void gfx3d_rotate_demo (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const angle_t a); 00087 extern "C" void gfx3d_rotate_x (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const angle_t a); 00088 extern "C" void gfx3d_rotate_y (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const angle_t a); 00089 extern "C" void gfx3d_rotate_z (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const angle_t a); 00090 extern "C" void gfx3d_scale (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const g3d_p3_t scl); 00091 extern "C" void gfx3d_translate (const g3d_p3_t *src, g3d_p3_t *dst, const int npnts, const g3d_p3_t tx); 00092 extern "C" void gfx3d_project (const g3d_p3_t *src, g3d_2p3_t *dst, const int npnts); 00093 extern "C" void gfx3d_cubify_points (const g3d_2p3_t *src, g3d_poly_t *dst, int *npoly, const int backfaces, const uint8_t **txptrs); 00094 extern "C" void gfx3d_squarify_points (const g3d_2p3_t *src, g3d_poly_t *dst, int *npoly, const int backfaces); 00095 00096 extern "C" void gfx3d_clear_zb (void); 00097 extern "C" void gfx3d_sort_poly (g3d_poly_t *p); 00098 00099 extern "C" void gfx3d_wirepoly (const g3d_poly_t *src, C12832 &lcd); 00100 extern "C" void gfx3d_wirepoly_z (const g3d_poly_t *src, C12832 &lcd); 00101 00102 extern "C" void gfx3d_edgebuf_z (const g3d_poly_t *src, g3d_edgebuf_t *dst); 00103 extern "C" void gfx3d_wireedge (const g3d_edgebuf_t *edge, C12832 &lcd); 00104 00105 extern "C" void gfx3d_polytxmap (const g3d_poly_t *src, C12832 &lcd); 00106 extern "C" void gfx3d_polynormmap (const g3d_poly_t *src, C12832 &lcd); 00107 00108 00109 #if 0 00110 extern "C" void gfx3d_polyscan (const g3d_poly_t *src, g3d_polyscan_t *dst); 00111 #endif 00112 00113 extern "C" void gfx3d_wirecube (const g3d_2p3_t *src, C12832 &lcd); 00114 00115 /* some hacky font stuff */ 00116 extern "C" int gfx3d_font04b_char_dpw (const char ch); 00117 extern "C" void gfx3d_font04b_tx_putchar (uint8_t *txbuf, const int txwidth, int *xptr, const int y, const char ch, const bool inv); 00118 extern "C" void gfx3d_font04b_tx_putstr (uint8_t *txbuf, const int txwidth, int *xptr, const int y, const char *str, const bool inv); 00119 extern "C" void gfx3d_font04b_tx_putstrn (uint8_t *txbuf, const int txwidth, int *xptr, const int y, const char *str, const int slen, const bool inv); 00120 00121 static inline uint8_t g3d_texture_bit (const uint8_t *tx, int x, int y) 00122 { 00123 return (tx[(x << 2) | (y >> 3)] & (0x01 << (y & 0x07))); 00124 } 00125 00126 00127 #endif /* !__GFX3D_H */
Generated on Wed Jul 13 2022 15:11:24 by
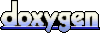