Fork of Chris Styles' C12832 LCD driver
Dependents: co657_lcdplay co657_nrf52_beacons door_lock co657_IoT
Fork of C12832 by
C12832.h
00001 /* mbed library for the mbed Lab Board 128*32 pixel LCD 00002 * use C12832 controller 00003 * Copyright (c) 2012 Peter Drescher - DC2PD 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00007 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00008 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00009 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00010 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00011 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00012 * THE SOFTWARE. 00013 */ 00014 00015 #ifndef C12832_H 00016 #define C12832_H 00017 00018 #include "mbed.h" 00019 #include "GraphicsDisplay.h" 00020 00021 00022 /** optional Defines : 00023 * #define debug_lcd 1 enable infos to PC_USB 00024 */ 00025 00026 // some defines for the DMA use 00027 #define DMA_CHANNEL_ENABLE 1 00028 #define DMA_TRANSFER_TYPE_M2P (1UL << 11) 00029 #define DMA_CHANNEL_TCIE (1UL << 31) 00030 #define DMA_CHANNEL_SRC_INC (1UL << 26) 00031 #define DMA_MASK_IE (1UL << 14) 00032 #define DMA_MASK_ITC (1UL << 15) 00033 #define DMA_SSP1_TX (1UL << 2) 00034 #define DMA_SSP0_TX (0) 00035 #define DMA_DEST_SSP1_TX (2UL << 6) 00036 #define DMA_DEST_SSP0_TX (0UL << 6) 00037 00038 /** Draw mode 00039 * NORMAl 00040 * XOR set pixel by xor the screen 00041 */ 00042 enum {NORMAL,XOR}; 00043 00044 /** Bitmap 00045 */ 00046 struct Bitmap{ 00047 int xSize; 00048 int ySize; 00049 int Byte_in_Line; 00050 char* data; 00051 }; 00052 00053 class C12832 : public GraphicsDisplay 00054 { 00055 public: 00056 /** Create a C12832 object connected to SPI1 00057 * 00058 */ 00059 00060 C12832(PinName mosi, PinName sck, PinName reset, PinName a0, PinName ncs, const char* name = "LCD"); 00061 00062 00063 /** Get the width of the screen in pixel 00064 * 00065 * @param 00066 * @returns width of screen in pixel 00067 * 00068 */ 00069 virtual int width(); 00070 00071 /** Get the height of the screen in pixel 00072 * 00073 * @returns height of screen in pixel 00074 * 00075 */ 00076 virtual int height(); 00077 00078 /** Draw a pixel at x,y black or white 00079 * 00080 * @param x horizontal position 00081 * @param y vertical position 00082 * @param colour ,1 set pixel ,0 erase pixel 00083 */ 00084 virtual void pixel (int x, int y, int colour); 00085 00086 /** draw a circle 00087 * 00088 * @param x0,y0 center 00089 * @param r radius 00090 * @param colour ,1 set pixel ,0 erase pixel 00091 * 00092 */ 00093 void circle(int x, int y, int r, int colour); 00094 00095 /** draw a filled circle 00096 * 00097 * @param x0,y0 center 00098 * @param r radius 00099 * @param color ,1 set pixel ,0 erase pixel 00100 * 00101 * use circle with different radius, 00102 * can miss some pixel 00103 */ 00104 void fillcircle(int x, int y, int r, int colour); 00105 00106 /** draw a 1 pixel line 00107 * 00108 * @param x0,y0 start point 00109 * @param x1,y1 stop point 00110 * @param colour 1 set pixel, 0 erase pixel 00111 * 00112 */ 00113 void line (int x0, int y0, int x1, int y1, int colour); 00114 00115 00116 /** draw a horizontal line. Note x1 must be >= x0. 00117 * 00118 * @param x0 horizontal start 00119 * @param y0 vertical position 00120 * @param x1 horizontal stop 00121 * @param colour 1 set pixel, 0 erase pixel 00122 * 00123 */ 00124 void hline (int x0, int y0, int x1, int colour); 00125 00126 00127 /** draw a vertical line. Note y1 must be >= y0. 00128 * 00129 * @param x0 horizontal position 00130 * @param y0 vertical start 00131 * @param y1 vertical stop 00132 * @param colour 1 set pixel, 0 erase pixel 00133 * 00134 */ 00135 void vline (int x0, int y0, int y1, int colour); 00136 00137 00138 /** draw a rectangle 00139 * 00140 * @param x0,y0 top left corner 00141 * @param x1,y1 down right corner 00142 * @param color 1 set pixel ,0 erase pixel 00143 * * 00144 */ 00145 void rect(int x0, int y0, int x1, int y1, int colour); 00146 00147 /** draw a filled rectangle 00148 * 00149 * @param x0,y0 top left corner 00150 * @param x1,y1 down right corner 00151 * @param color 1 set pixel ,0 erase pixel 00152 * 00153 */ 00154 void fillrect(int x0, int y0, int x1, int y1, int colour); 00155 00156 /** copy display buffer to lcd 00157 * 00158 */ 00159 00160 void copy_to_lcd(void); 00161 00162 /** set the orienation of the screen 00163 * 00164 */ 00165 00166 00167 void set_contrast(unsigned int o); 00168 00169 /** read the contrast level 00170 * 00171 */ 00172 unsigned int get_contrast(void); 00173 00174 00175 /** invert the screen 00176 * 00177 * @param o = 0 normal, 1 invert 00178 */ 00179 void invert(unsigned int o); 00180 00181 /** clear the screen 00182 * 00183 */ 00184 virtual void cls(void); 00185 00186 /** set the drawing mode 00187 * 00188 * @param mode NORMAl or XOR 00189 */ 00190 00191 void setmode(int mode); 00192 00193 virtual int columns(void); 00194 00195 /** calculate the max number of columns 00196 * 00197 * @returns max column 00198 * depends on actual font size 00199 * 00200 */ 00201 virtual int rows(void); 00202 00203 /** put a char on the screen 00204 * 00205 * @param value char to print 00206 * @returns printed char 00207 * 00208 */ 00209 virtual int _putc(int value); 00210 00211 /** draw a character on given position out of the active font to the LCD 00212 * 00213 * @param x x-position of char (top left) 00214 * @param y y-position 00215 * @param c char to print 00216 * 00217 */ 00218 virtual void character(int x, int y, int c); 00219 00220 /** setup cursor position 00221 * 00222 * @param x x-position (top left) 00223 * @param y y-position 00224 */ 00225 virtual void locate(int x, int y); 00226 00227 /** setup auto update of screen 00228 * 00229 * @param up 1 = on , 0 = off 00230 * if switched off the program has to call copy_to_lcd() 00231 * to update screen from framebuffer 00232 */ 00233 void set_auto_up(unsigned int up); 00234 00235 /** get status of the auto update function 00236 * 00237 * @returns if auto update is on 00238 */ 00239 unsigned int get_auto_up(void); 00240 00241 /** Vars */ 00242 SPI _spi; 00243 DigitalOut _reset; 00244 DigitalOut _A0; 00245 DigitalOut _CS; 00246 unsigned char* font; 00247 unsigned int draw_mode; 00248 00249 00250 /** select the font to use 00251 * 00252 * @param f pointer to font array 00253 * 00254 * font array can created with GLCD Font Creator from http://www.mikroe.com 00255 * you have to add 4 parameter at the beginning of the font array to use: 00256 * - the number of byte / char 00257 * - the vertial size in pixel 00258 * - the horizontal size in pixel 00259 * - the number of byte per vertical line 00260 * you also have to change the array to char[] 00261 * 00262 */ 00263 void set_font(unsigned char* f); 00264 00265 /** print bitmap to buffer 00266 * 00267 * @param bm Bitmap in flash 00268 * @param x x start 00269 * @param y y start 00270 * 00271 */ 00272 00273 void print_bm(Bitmap bm, int x, int y); 00274 00275 /** Plot a pixel without bounds checking 00276 * 00277 * @param x X position [0..127] 00278 * @param y Y position [0..31] 00279 * @param colour colour (1 = set, 0 = clear) 00280 */ 00281 void pixel_nochk (int x, int y, int colour); 00282 00283 /** Plots a pixel, but without bounds checking (assumed to be done elsewhere). Fixed normal-mode. */ 00284 inline void pixel_nochk_norm (int x, int y, int colour) 00285 { 00286 if (colour == 0) { 00287 buffer[x + ((y & ~7) << 4)] &= ~(1 << (y & 0x07)); // erase pixel 00288 } else { 00289 buffer[x + ((y & ~7) << 4)] |= (1 << (y & 0x07)); // set pixel 00290 } 00291 } 00292 00293 /** Plots a pixel, but without bounds checking (assumed to be done elsewhere). Fixed XOR mode. */ 00294 inline void pixel_nochk_xor (int x, int y, int colour) 00295 { 00296 if (colour == 1) { 00297 buffer[x + ((y & ~7) << 4)] ^= (1 << (y & 0x07)); // xor pixel 00298 } 00299 } 00300 00301 protected: 00302 00303 /** Init the C12832 LCD controller 00304 * 00305 */ 00306 void lcd_reset(); 00307 00308 /** Write data to the LCD controller 00309 * 00310 * @param dat data written to LCD controller 00311 * 00312 */ 00313 void wr_dat(unsigned char value); 00314 00315 /** Write a command the LCD controller 00316 * 00317 * @param cmd: command to be written 00318 * 00319 */ 00320 void wr_cmd(unsigned char value); 00321 00322 void wr_cnt(unsigned char cmd); 00323 00324 unsigned int orientation; 00325 unsigned int char_x; 00326 unsigned int char_y; 00327 unsigned char buffer[512]; 00328 unsigned int contrast; 00329 unsigned int auto_up; 00330 00331 }; 00332 00333 00334 00335 00336 #endif
Generated on Tue Jul 12 2022 17:25:58 by
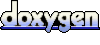