
an mbed tile music game using a capacitive touchpad and uLCD
Dependencies: SDFileSystem mbed wave_player
main.cpp
00001 //ECE 4180 Piano Tiles 00002 //Spring 2016 Georgia Tech 00003 //Kushagra Brahmbhatt 00004 //Christopher Lu 00005 00006 #include "mbed.h" 00007 #include "uLCD_4DGL.h" 00008 #include <mpr121.h> 00009 #include "SongPlayer.h" 00010 #include "SDFileSystem.h" 00011 #include "wave_player.h" 00012 00013 //LCD better 00014 uLCD_4DGL uLCD(p28,p27,p29); 00015 00016 //Speaker 00017 SongPlayer mySpeaker(p21); 00018 AnalogOut Speaker(p18); 00019 00020 // Setup the i2c bus on pins 9 and 10 00021 I2C i2c(p9, p10); 00022 00023 // Setup the Mpr121: 00024 // Create the interrupt receiver object on pin 26 00025 InterruptIn interrupt(p26); 00026 // constructor(i2c object, i2c address of the mpr121) 00027 Mpr121 mpr121(&i2c, Mpr121::ADD_VSS); 00028 00029 // the pinout on the mbed Cool Components workshop board 00030 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00031 00032 //timer 00033 Timer t; 00034 00035 //bool key0Press, key4Press, key8Press; 00036 int vals; 00037 bool interruptBool; 00038 int score, timer; 00039 00040 // Key hit/release interrupt routine 00041 void fallInterrupt() { //void 00042 00043 int value=mpr121.read(0x00); 00044 00045 //wait(2); 00046 value +=mpr121.read(0x01)<<8; 00047 interruptBool = true; 00048 vals = value; 00049 } 00050 00051 00052 int press() { 00053 int value=mpr121.read(0x00); 00054 value +=mpr121.read(0x01)<<8; 00055 //1(key 0) 2(key 1) 4 8 16 32 64 128 256 512 1024 2048(key 11) 00056 return value; 00057 } 00058 00059 bool check(int value) { 00060 if (value == 1) { //key0 00061 if(uLCD.read_pixel(15,90)==BLACK) { 00062 return true; 00063 } 00064 } 00065 if (value == 16) { //key4 00066 if(uLCD.read_pixel(50,90)==BLACK) { 00067 return true; 00068 } 00069 } 00070 if (value == 256) { //key8 00071 if(uLCD.read_pixel(90,90)==BLACK) { 00072 return true; 00073 } 00074 } 00075 return false; 00076 } 00077 00078 //initialize the graphics for the game 00079 void startGame(){ 00080 uLCD.background_color(WHITE); 00081 uLCD.cls(); 00082 //draw walls 00083 uLCD.filled_rectangle(0,103,10,0, BLUE); 00084 uLCD.filled_rectangle(117,103,127,0, BLUE); 00085 uLCD.filled_rectangle(10,78,117,103, 0xFDF983); 00086 uLCD.locate(0,13); 00087 uLCD.textbackground_color(WHITE); 00088 uLCD.color(RED); 00089 uLCD.printf("Score: "); 00090 uLCD.locate(9,13); 00091 uLCD.printf("Time:"); 00092 uLCD.locate(4,14); 00093 uLCD.text_width(1); //4X size text 00094 uLCD.text_height(2); 00095 uLCD.printf("Piano Tiles"); 00096 00097 00098 //draw the lines 00099 uLCD.line(10, 26, 117, 26, BLACK); 00100 uLCD.line(10, 52, 117, 52, BLACK); 00101 uLCD.line(10, 78, 117, 78, BLACK); 00102 uLCD.line(10, 103, 117, 103, BLACK); 00103 00104 uLCD.line(46, 0, 46, 103, BLACK); 00105 uLCD.line(82, 0, 82, 103, BLACK); 00106 00107 } 00108 00109 //moves block down 00110 int updateBlockY1(int y) { 00111 y = y + 26; 00112 if (y > 81) { 00113 y = 3; 00114 } 00115 return y; 00116 } 00117 00118 int updateBlockY2(int y) { 00119 y = y + 26; 00120 if (y > 101) { 00121 y = 23; 00122 } 00123 return y; 00124 } 00125 00126 //Frequency of notes for Fur Elise 00127 float note[85]= {329.63,311.13,329.63,311.13,329.63,246.94,293.66,261.63,220.00, 00128 146.83,174.61,220.0,246.94,174.61,233.08,246.94,261.63, 329.63, 00129 311.13, 329.63, 246.94, 293.66, 261.63, 220.00, 146.83, 174.61, 00130 220.00, 246.94, 174.61, 261.63, 246.94, 220.00, 246.94, 261.63, 00131 293.66, 329.63, 196.00, 329.23, 329.23, 293.63, 164.81, 329.63, 00132 293.63, 261.63, 146.83, 293.63, 261.63, 246.94, 329.63, 311.13, 00133 329.63, 311.13, 329.63, 246.94, 293.66, 261.63, 220.00, 146.83, 00134 174.61, 220.00, 246.94, 174.61, 233.08, 246.94, 261.63, 329.63, 00135 311.13, 329.63, 311.13, 329.63, 311.13, 329.63, 246.94, 293.66, 00136 261.63, 220.00, 146.83, 174.61, 220.00, 246.94, 174.61, 261.63, 00137 246.94, 220.0, 0.0}; 00138 //How long each note plays for 00139 float duration[85]= {0.3,0.3,0.3,0.3,0.30,0.30,0.30,0.30,0.30, 00140 0.30,0.30,0.30,0.30,0.30,0.30,0.30,0.30, 00141 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00142 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00143 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00144 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00145 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00146 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00147 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00148 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00149 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 0.30, 00150 0.30, 0.30, 0.30, 0.30, 0.0}; 00151 00152 00153 int main() { 00154 00155 //wait 4 seconds then moves on to graphics of the game 00156 uLCD.locate(3,4); 00157 uLCD.text_width(1); 00158 uLCD.text_height(2); 00159 uLCD.printf("Piano Tiles!!!\n"); 00160 uLCD.text_height(1); 00161 uLCD.locate(5,8); 00162 uLCD.printf("Playing:"); 00163 uLCD.locate(5,9); 00164 uLCD.printf("Beethoven"); 00165 uLCD.locate(5,10); 00166 uLCD.printf("Fur Elise"); 00167 uLCD.locate(0,12); 00168 uLCD.printf("Get a score above "); 00169 uLCD.locate(4,13); 00170 uLCD.printf("100 to win!"); 00171 wait(4); 00172 00173 interrupt.fall(&fallInterrupt); 00174 interrupt.mode(PullUp); 00175 startGame(); 00176 00177 //initialization to track tiles 00178 int a[16]; 00179 int y1 = 81; //starting y coordinate to fill in rectangle from the bottom 00180 int y2 = 101; 00181 int position = 1; //tracks placement of the previous tile for tone so tiles are placed by tones 00182 00183 00184 //initializing the first four set of piano tiles 00185 for (int i = 0; i < 4; i++) { 00186 if (note[i] < note[i-1] && i != 0){ //if (note<previousnote) then next tile is to the left 00187 uLCD.filled_rectangle(49,y1,79,y2, BLACK); 00188 a[4*i + 0] = 49; 00189 a[4*i + 1] = y1; 00190 a[4*i + 2] = 79; 00191 a[4*i + 3] = y2; 00192 position = 2; 00193 } 00194 else if (i == 0 || note[i] > note[i-1]) { //initial start is on right side of screen 00195 uLCD.filled_rectangle(85,y1,115,y2, BLACK); 00196 a[4*i + 0] = 85; 00197 a[4*i + 1] = y1; 00198 a[4*i + 2] = 115; 00199 a[4*i + 3] = y2; 00200 position = 3; 00201 } 00202 //move from bottom of the screen to the top 00203 y1 = y1 - 26; 00204 y2 = y2 - 26; 00205 } 00206 //array used for holding all the tiles 00207 int block1_x1 = a[0]; 00208 int block1_y1 = a[1]; 00209 int block1_x2 = a[2]; 00210 int block1_y2 = a[3]; 00211 int block2_x1 = a[4]; 00212 int block2_y1 = a[5]; 00213 int block2_x2 = a[6]; 00214 int block2_y2 = a[7]; 00215 int block3_x1 = a[8]; 00216 int block3_y1 = a[9]; 00217 int block3_x2 = a[10]; 00218 int block3_y2 = a[11]; 00219 int block4_x1 = a[12]; 00220 int block4_y1 = a[13]; 00221 int block4_x2 = a[14]; 00222 int block4_y2 = a[15]; 00223 00224 t.start(); 00225 00226 //starting the game 00227 while(1) { 00228 if (interruptBool) { //key is pressed 00229 uLCD.locate(0,0); 00230 bool checkTrue = check(vals); 00231 uLCD.locate(0,0); 00232 00233 if (checkTrue) { //if right correct key is pressed, tiles are replaced and move down 00234 uLCD.filled_rectangle(block1_x1, block1_y1, block1_x2, block1_y2, WHITE); 00235 uLCD.filled_rectangle(block2_x1, block2_y1, block2_x2, block2_y2, WHITE); 00236 uLCD.filled_rectangle(block3_x1, block3_y1, block3_x2, block3_y2, WHITE); 00237 uLCD.filled_rectangle(block4_x1, block4_y1, block4_x2, block4_y2, WHITE); 00238 block1_y1 = updateBlockY1(block1_y1); 00239 block1_y2 = updateBlockY2(block1_y2); 00240 block2_y1 = updateBlockY1(block2_y1); 00241 block2_y2 = updateBlockY2(block2_y2); 00242 block3_y1 = updateBlockY1(block3_y1); 00243 block3_y2 = updateBlockY2(block3_y2); 00244 block4_y1 = updateBlockY1(block4_y1); 00245 block4_y2 = updateBlockY2(block4_y2); 00246 00247 //the following if statements determine position of the following tiles based on the 00248 //frequencies of the note that is being played 00249 if(block1_y1 == 3) 00250 { 00251 if (note[score%85+4] < note[score%85+3] && (position == 1 || position == 2)) { 00252 block1_x1 = 13; 00253 block1_x2 = 43; 00254 position = 1; 00255 } else if ((note[score%85+4] < note[score%85+3] && position == 3) || (note[score%85+4] > note[score%85+3] && position == 1 )) { 00256 block1_x1 = 49; 00257 block1_x2 = 79; 00258 position = 2; 00259 } else if (note[score%85+4] > note[score%85+3] && (position == 2 || position == 3)) { 00260 block1_x1 = 85; 00261 block1_x2 = 115; 00262 position = 3; 00263 } 00264 } 00265 if(block2_y1 == 3) 00266 { 00267 if (note[score%85+4] < note[score%85+3] && (position == 1 || position == 2)) { 00268 block2_x1 = 13; 00269 block2_x2 = 43; 00270 position = 1; 00271 } else if ((note[score%85+4] < note[score%85+3] && position == 3) || (note[score%85+4] > note[score%85+3] && position == 1 )) { 00272 block2_x1 = 49; 00273 block2_x2 = 79; 00274 position = 2; 00275 } else if (note[score%85+4] > note[score%85+3] && (position == 2 || position == 3)) { 00276 block2_x1 = 85; 00277 block2_x2 = 115; 00278 position = 3; 00279 } 00280 } 00281 if(block3_y1 == 3) 00282 { 00283 if (note[score%85+4] < note[score%85+3] && (position == 1 || position == 2)) { 00284 block3_x1 = 13; 00285 block3_x2 = 43; 00286 position = 1; 00287 } else if ((note[score%85+4] < note[score%85+3] && position == 3) || (note[score%85+4] > note[score%85+3] && position == 1 )) { 00288 block3_x1 = 49; 00289 block3_x2 = 79; 00290 position = 2; 00291 } else if (note[score%85+4] > note[score%85+3] && (position == 2 || position == 3)) { 00292 block3_x1 = 85; 00293 block3_x2 = 115; 00294 position = 3; 00295 } 00296 } 00297 if(block4_y1 == 3) 00298 { 00299 if (note[score%85+4] < note[score%85+3] && (position == 1 || position == 2)) { 00300 block4_x1 = 13; 00301 block4_x2 = 43; 00302 position = 1; 00303 } else if ((note[score%85+4] < note[score%85+3] && position == 3) || (note[score%85+4] > note[score%85+3] && position == 1 )) { 00304 block4_x1 = 49; 00305 block4_x2 = 79; 00306 position = 2; 00307 } else if (note[score%85+4] > note[score%85+3] && (position == 2 || position == 3)) { 00308 block4_x1 = 85; 00309 block4_x2 = 115; 00310 position = 3; 00311 } 00312 } 00313 00314 //fill in the tiles 00315 uLCD.filled_rectangle(block1_x1, block1_y1, block1_x2, block1_y2, BLACK); 00316 uLCD.filled_rectangle(block2_x1, block2_y1, block2_x2, block2_y2, BLACK); 00317 uLCD.filled_rectangle(block3_x1, block3_y1, block3_x2, block3_y2, BLACK); 00318 uLCD.filled_rectangle(block4_x1, block4_y1, block4_x2, block4_y2, BLACK); 00319 interruptBool = false; 00320 00321 //plays the note as the keypad is being pressed 00322 float note1[] = {note[score%85]}; 00323 float duration1[] = {duration[score%85]}; 00324 mySpeaker.PlaySong(note1,duration1); 00325 score++; 00326 00327 //updates the time and score 00328 uLCD.text_width(1); 00329 uLCD.text_height(1); 00330 uLCD.locate(0,13); 00331 uLCD.textbackground_color(WHITE); 00332 uLCD.color(RED); 00333 uLCD.printf("Score:%d", score); 00334 uLCD.locate(9,13); 00335 uLCD.printf("Time:%0.1f", t.read()); 00336 00337 00338 } 00339 else { //while loop breaks when the wrong key is pressed 00340 break; 00341 //Game Over Screen outside while loop 00342 } 00343 } //end of interruptBool 00344 }//end of while loop 00345 00346 00347 //win or lose music 00348 wave_player waver(&Speaker); 00349 //vector<string> filenames; //filenames are stored in a vector string 00350 bool PLAY = true; 00351 bool *PlayStopPtr = &PLAY; 00352 FILE *wave_file; 00353 00354 //victory and game over screen 00355 if (score >= 100) 00356 { 00357 uLCD.cls(); 00358 uLCD.locate(0,4); 00359 uLCD.text_width(2); //4X size text 00360 uLCD.text_height(2); 00361 uLCD.printf("Victory!!"); 00362 uLCD.text_width(1); //4X size text 00363 uLCD.text_height(1); 00364 uLCD.locate(4,7); 00365 uLCD.printf("SCORE:%d", score); 00366 t.stop(); 00367 uLCD.locate(4,8); 00368 uLCD.printf("Time:%0.2f", t.read()); 00369 uLCD.locate(0,10); 00370 uLCD.printf("Thanks for playing"); 00371 00372 FILE *wave_file; 00373 wave_file=fopen("/sd/wavfiles/victory.wav","r"); 00374 waver.play(wave_file,PlayStopPtr); 00375 } 00376 else 00377 { 00378 uLCD.cls(); 00379 uLCD.locate(0,4); 00380 uLCD.text_width(2); //4X size text 00381 uLCD.text_height(2); 00382 uLCD.printf("Game Over"); 00383 uLCD.text_width(1); //4X size text 00384 uLCD.text_height(1); 00385 uLCD.locate(4,7); 00386 uLCD.printf("SCORE:%d", score); 00387 t.stop(); 00388 uLCD.locate(4,8); 00389 uLCD.printf("Time:%0.2f", t.read()); 00390 uLCD.locate(0,10); 00391 uLCD.printf("Thanks for playing"); 00392 00393 FILE *wave_file; 00394 wave_file=fopen("/sd/wavfiles/defeat.wav","r"); 00395 waver.play(wave_file,PlayStopPtr); 00396 } 00397 }
Generated on Sun Jul 24 2022 12:21:37 by
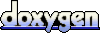