ThingPlug Test
Dependents: WizFi310_ThingPlug_Test WizFi310_ThingPlug_Test_P
Fork of WizFi310Interface by
WizFi310.cpp
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi310 00021 */ 00022 00023 #include "mbed.h" 00024 #include "WizFi310.h" 00025 00026 WizFi310 * WizFi310::_inst; 00027 00028 00029 WizFi310::WizFi310(PinName tx,PinName rx,PinName cts, PinName rts,PinName reset, PinName alarm, int baud): 00030 _wizfi(tx,rx), _reset(reset) 00031 { 00032 _inst = this; 00033 memset(&_state, 0, sizeof(_state)); 00034 memset(&_con, 0, sizeof(_con)); 00035 _state.initialized = false; 00036 _state.status = STAT_READY; 00037 _state.cid = -1; 00038 _state.buf = new CircBuffer<char>(CFG_DATA_SIZE); 00039 00040 initUart(cts, rts, alarm, baud); 00041 _reset.output(); 00042 00043 setRts(true); // release 00044 /* 00045 wait_ms(500); 00046 cmdAT(); 00047 cmdMECHO(false); 00048 if(cts != NC && rts != NC) 00049 cmdUSET(baud,"HW"); 00050 else 00051 cmdUSET(baud,"N"); 00052 00053 // WizFi310 will restart by cmdUSET command. 00054 wait_ms(1000); 00055 cmdAT(); 00056 */ 00057 } 00058 00059 int WizFi310::join(WiFiMode mode) 00060 { 00061 char sec[10]; 00062 00063 if( cmdMMAC() ) return -1; 00064 00065 if(mode == WM_AP) 00066 _state.wm = WM_AP; 00067 else 00068 _state.wm = WM_STATION; 00069 00070 if ( cmdWNET(_state.dhcp) ) return -1; 00071 if ( cmdWSET(_state.wm, _state.ssid) ) return -1; 00072 00073 00074 switch (_state.sec) 00075 { 00076 case NSAPI_SECURITY_NONE: 00077 strcpy(sec,"OPEN"); 00078 break; 00079 case NSAPI_SECURITY_WEP: 00080 strcpy(sec,"WEP"); 00081 break; 00082 case NSAPI_SECURITY_WPA: 00083 strcpy(sec,"WPA"); 00084 break; 00085 case NSAPI_SECURITY_WPA2: 00086 strcpy(sec,"WPA2"); 00087 break; 00088 default: 00089 strcpy(sec,""); 00090 break; 00091 } 00092 00093 if ( cmdWSEC(_state.wm, _state.pass, sec) ) return -1; 00094 if ( cmdWJOIN() ) return -1;; 00095 _state.associated = true; 00096 00097 return 0; 00098 } 00099 00100 bool WizFi310::isAssociated() 00101 { 00102 return _state.associated; 00103 } 00104 00105 int WizFi310::setMacAddress (const char *mac) 00106 { 00107 if (cmdMMAC(mac)) return -1; 00108 strncpy(_state.mac, mac, sizeof(_state.mac)); 00109 return 0; 00110 } 00111 00112 int WizFi310::getMacAddress (char *mac) 00113 { 00114 if (cmdMMAC()) return -1; 00115 strcpy(mac, _state.mac); 00116 return 0; 00117 } 00118 00119 int WizFi310::setAddress (const char *name) 00120 { 00121 _state.dhcp = true; 00122 strncpy(_state.name, name, sizeof(_state.name)); 00123 return 0; 00124 } 00125 00126 int WizFi310::setAddress (const char *ip, const char *netmask, const char *gateway, const char *dns, const char *name) 00127 { 00128 _state.dhcp = false; 00129 strncpy(_state.ip, ip, sizeof(_state.ip)); 00130 strncpy(_state.netmask, netmask, sizeof(_state.netmask)); 00131 strncpy(_state.gateway, gateway, sizeof(_state.gateway)); 00132 strncpy(_state.nameserver, dns, sizeof(_state.nameserver)); 00133 strncpy(_state.name, name, sizeof(_state.name)); 00134 return 0; 00135 } 00136 00137 int WizFi310::getAddress (char *ip, char *netmask, char *gateway) 00138 { 00139 strcpy(ip, _state.ip); 00140 strcpy(netmask, _state.netmask); 00141 strcpy(gateway, _state.gateway); 00142 return 0; 00143 } 00144 00145 int WizFi310::setSsid (const char *ssid) 00146 { 00147 strncpy(_state.ssid, ssid, sizeof(_state.ssid)); 00148 return 0; 00149 } 00150 00151 //daniel 00152 //int WizFi310::setSec ( Security sec, const char *phrase ) 00153 int WizFi310::setSec ( nsapi_security_t sec, const char *phrase ) 00154 { 00155 _state.sec = sec; 00156 strncpy(_state.pass, phrase, strlen(phrase)); 00157 return 0; 00158 } 00159 00160 const char *WizFi310::getIPAddress(void) 00161 { 00162 return _state.ip; 00163 } 00164 00165 const char *WizFi310::getMACAddress(void) 00166 { 00167 return _state.mac; 00168 } 00169 00170 00171 int WizFi310::joinTP(const char *clientId, const char *credentialId, const char *serviceId, const char *devId, const char *containerNm) 00172 { 00173 WIZ_INFO("ThingPlug Start\r\n"); 00174 00175 if(cmdSKTPCON("1", clientId, credentialId, serviceId, devId)) return -1; 00176 WIZ_INFO("ThingPlug Connected"); 00177 00178 if(cmdSKTPDEVICE("1", devId)) return -1; 00179 WIZ_INFO("Device Registered"); 00180 00181 if(cmdSKTPCONTAINER("1", containerNm)) return -1; 00182 WIZ_INFO("Created Container"); 00183 00184 if(cmdSKTPSEND(containerNm, "010600000026020600000022")) return -1; 00185 WIZ_INFO("Sending Data"); 00186 00187 if(cmdSKTPCON("0", clientId, credentialId, serviceId, devId)) return -1; 00188 WIZ_INFO("ThingPlug Disconnected"); 00189 00190 return 0; 00191 }
Generated on Sat Jul 16 2022 03:26:38 by
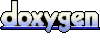