CanInterface Dispatcher, it depends on MyThread (MyThings lib)
Embed:
(wiki syntax)
Show/hide line numbers
CANInterface.h
00001 00002 #ifndef CAN_INTERFACE_H 00003 #define CAN_INTERFACE_H 00004 00005 #include "MyThread.h" 00006 #include "MyCallBack.h" 00007 #include "CAN.h" 00008 #include "CANFifoMessage.h" 00009 00010 #define CAN_ID_PROMISCUOUS_MODE 0xffffffff 00011 00012 #define CAN_SIMULTANEOUS_CBS 8 00013 #define CAN_SIMULTANEOUS_MSG 15 00014 00015 class CANInterface: public MyThread { 00016 protected: 00017 typedef struct _idCbEntry{ 00018 uint32_t id; // 29b and 11b can ids are supported 00019 MyCallBack *cb; 00020 } idCbEntry; 00021 00022 Mutex idCbTableMutex; 00023 Mutex sendMutex; 00024 00025 uint8_t nCurrentCbs; // How much entries we got in our table 00026 idCbEntry idCbTable[CAN_SIMULTANEOUS_CBS]; // This mean that we allow at most 8 sensors to receive events simultaneously 00027 00028 CAN *bus1; 00029 CAN *bus2; 00030 00031 bool rxOvf; 00032 CANFifoMessage fifoRxMsg; 00033 CANMessage msgTx; 00034 00035 public: 00036 CANInterface(); 00037 virtual void Configure(uint8_t ifaceNumber, int speed); 00038 virtual void AddCallBackForId(int id, MyCallBack *cb); 00039 virtual void DellCallBackForId(int id); 00040 virtual void DispatchCANMessage(CANMessage *msg); 00041 virtual int Send(uint8_t ifaceNumber,int id, char *data, uint8_t len); 00042 virtual void Main(); 00043 00044 virtual void RxCbCan1(void); 00045 virtual void RxCbCan2(void); 00046 00047 virtual CAN *GetCan1() { return bus1; } 00048 virtual CAN *GetCan2() { return bus2; } 00049 }; 00050 00051 #endif
Generated on Sat Jul 16 2022 01:00:20 by
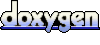