
Demo program for the MPL3115A2 library.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MPL3115A2.h" 00003 00004 #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00005 00006 DigitalOut myled(LED1); 00007 MPL3115A2 wigo_sensor1( PTE0, PTE1, MPL3115A2_I2C_ADDRESS); 00008 Serial pc(USBTX, USBRX); 00009 00010 /* pos [0] = altimeter or pressure value */ 00011 /* pos [1] = temperature value */ 00012 float sensor_data[2]; 00013 00014 void dataready( void); // callback function for data streaming using Interrupt 00015 void alttrigger( void); 00016 00017 /* Helper functions to print out as float the raw data */ 00018 float print_PressureValue( unsigned char *dt); 00019 float print_AltimiterValue( unsigned char *dt); 00020 float print_TemperatureValue( unsigned char *dt); 00021 00022 int main() { 00023 00024 pc.baud( 230400); 00025 pc.printf("MPL3115A2 Sensor. [%X]\r\n", wigo_sensor1.getDeviceID()); 00026 00027 #if 0 00028 // ***** Data acquisition using Interrupt 00029 00030 // Configure the sensor as Barometer. 00031 wigo_sensor1.Barometric_Mode(); 00032 // Set callback function and over sampling value (see MPL3115A2.h for details) 00033 wigo_sensor1.DataReady( &dataready, OVERSAMPLE_RATIO_64); 00034 // just loop... 00035 while( 1) 00036 { 00037 wait( 1.0); 00038 pc.printf("."); 00039 } 00040 #endif 00041 00042 #if 0 00043 // ***** Data acquisition using polling method 00044 00045 // Configure the sensor as Barometer. 00046 unsigned int mode=1; 00047 00048 // Set over sampling value (see MPL3115A2.h for details) 00049 wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_64); 00050 // Configure the sensor as Barometer. 00051 wigo_sensor1.Barometric_Mode(); 00052 00053 while(1) { 00054 // 00055 if ( wigo_sensor1.getAllData( &sensor_data[0])) { 00056 if ( mode & 0x0001) { 00057 pc.printf("\tPressure: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00058 wigo_sensor1.Altimeter_Mode(); 00059 } else { 00060 pc.printf("\tAltitude: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00061 wigo_sensor1.Barometric_Mode(); 00062 } 00063 mode++; 00064 } 00065 // 00066 wait( 1.0); 00067 } 00068 #endif 00069 00070 #if 0 00071 // ***** Data acquisition using polling method with Max and Min values visualization 00072 00073 // Configure the sensor as Barometer. 00074 unsigned int mode=1; 00075 00076 // Set over sampling value (see MPL3115A2.h for details) 00077 wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_64); 00078 // Configure the sensor as Barometer. 00079 wigo_sensor1.Barometric_Mode(); 00080 00081 while(1) { 00082 // 00083 if ( wigo_sensor1.getAllData( &sensor_data[0])) { 00084 pc.printf("\tPressure: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00085 if ( (mode % 20)==0) { 00086 sensor_data[0] = sensor_data[1] = 0.0; 00087 wigo_sensor1.getAllMaximumData( &sensor_data[0]); 00088 pc.printf("\tMaxPress: %f\tMaxTemp: %f\r\n", sensor_data[0], sensor_data[1]); 00089 sensor_data[0] = sensor_data[1] = 0.0; 00090 wigo_sensor1.getAllMinimumData( &sensor_data[0]); 00091 pc.printf("\tMinPress: %f\tMinTemp: %f\r\n", sensor_data[0], sensor_data[1]); 00092 } 00093 mode++; 00094 } 00095 // 00096 wait( 1.0); 00097 } 00098 #endif 00099 00100 #if 1 00101 // ***** Data acquisition using polling method and delta values 00102 00103 // Array for the dela values 00104 float delta[2]; 00105 00106 // Set over sampling value (see MPL3115A2.h for details) 00107 wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_128); 00108 // Configure the sensor as Barometer. 00109 wigo_sensor1.Barometric_Mode(); 00110 00111 while(1) { 00112 // 00113 if ( wigo_sensor1.getAllData( &sensor_data[0], &delta[0])) { 00114 pc.printf("\tPressure: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00115 pc.printf("\tDelatPress: %f\tDeltaTemp: %f\r\n", delta[0], delta[1]); 00116 } 00117 // 00118 wait( 1.0); 00119 } 00120 #endif 00121 00122 #if 0 00123 // ***** Data acquisition in RAW mode using polling method 00124 00125 // Create a buffer for raw data 00126 unsigned char raw_data[5]; 00127 // Configure the sensor as Barometer. 00128 unsigned int mode=1; 00129 00130 // Set over sampling value (see MPL3115A2.h for details) 00131 wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_64); 00132 // Configure the sensor as Barometer. 00133 wigo_sensor1.Barometric_Mode(); 00134 00135 while(1) { 00136 // 00137 if ( wigo_sensor1.getAllDataRaw( &raw_data[0])) { 00138 if ( mode & 0x0001) { 00139 pc.printf("\tPressure: %f\tTemperature: %f\r\n", print_PressureValue( &raw_data[0]), print_TemperatureValue( &raw_data[3])); 00140 wigo_sensor1.Altimeter_Mode(); 00141 } else { 00142 pc.printf("\tAltitude: %f\tTemperature: %f\r\n", print_AltimiterValue( &raw_data[0]), print_TemperatureValue( &raw_data[3])); 00143 wigo_sensor1.Barometric_Mode(); 00144 } 00145 mode++; 00146 } 00147 // 00148 wait( 1.0); 00149 } 00150 00151 #endif 00152 00153 } 00154 00155 void dataready( void) 00156 { 00157 wigo_sensor1.getAllData( &sensor_data[0]); 00158 pc.printf("\tPressure: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00159 } 00160 00161 float print_PressureValue( unsigned char *dt) 00162 { 00163 unsigned int prs; 00164 float fprs; 00165 00166 /* 00167 * dt[0] = Bits 12-19 of 20-bit real-time Pressure sample. (b7-b0) 00168 * dt[1] = Bits 4-11 of 20-bit real-time Pressure sample. (b7-b0) 00169 * dt[2] = Bits 0-3 of 20-bit real-time Pressure sample (b7-b4) 00170 */ 00171 prs = (dt[0]<<10) | (dt[1]<<2) | (dt[2]>>6); 00172 // 00173 fprs = (float)prs * 1.0f; 00174 00175 if ( dt[2] & 0x20) 00176 fprs += 0.25f; 00177 if ( dt[2] & 0x10) 00178 fprs += 0.5f; 00179 00180 return fprs; 00181 } 00182 00183 float print_AltimiterValue( unsigned char *dt) 00184 { 00185 unsigned short altm; 00186 float faltm; 00187 00188 /* 00189 * dt[0] = Bits 12-19 of 20-bit real-time Altitude sample. (b7-b0) 00190 * dt[1] = Bits 4-11 of 20-bit real-time Altitude sample. (b7-b0) 00191 * dt[2] = Bits 0-3 of 20-bit real-time Altitude sample (b7-b4) 00192 */ 00193 altm = (dt[0]<<8) | dt[1]; 00194 // 00195 if ( dt[0] > 0x7F) { 00196 altm = ~altm + 1; 00197 faltm = (float)altm * -1.0f; 00198 } else { 00199 faltm = (float)altm * 1.0f; 00200 } 00201 // 00202 faltm = faltm+((float)(dt[2]>>4) * 0.0625f); 00203 return faltm; 00204 } 00205 00206 float print_TemperatureValue( unsigned char *dt) 00207 { 00208 unsigned short temp; 00209 float ftemp; 00210 00211 /* 00212 * dt[0] = Bits 4-11 of 16-bit real-time temperature sample. (b7-b0) 00213 * dt[1] = Bits 0-3 of 16-bit real-time temperature sample. (b7-b4) 00214 */ 00215 temp = dt[0]; 00216 // 00217 if ( dt[0] > 0x7F) { 00218 temp = ~temp + 1; 00219 ftemp = (float)temp * -1.0f; 00220 } else { 00221 ftemp = (float)temp * 1.0f; 00222 } 00223 // 00224 ftemp = ftemp+((float)(dt[1]>>4) * 0.0625f); 00225 return ftemp; 00226 00227 } 00228
Generated on Tue Jul 12 2022 21:59:47 by
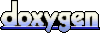