
QRSS Rx Network receiver. A receiver to sample a segment of RF spectrum and send this data to a server for further processing and display. NXP mbed Design Challenge entry (Honorable Mention). Published in Circuit Cellar, Feb 2012
Dependencies: NetServices mbed DNSResolver
main.cpp
00001 /*--------------------------------------------------------------------------- 00002 00003 QRSS Receiver Application 00004 00005 by Clayton ZL3TKA/VK1TKA 00006 clayton@isnotcrazy.com 00007 00008 main function 00009 00010 ---------------------------------------------------------------------------*/ 00011 // include files 00012 00013 #include "mbed.h" 00014 #include "global.h" 00015 #include "EthernetNetIf.h" 00016 #include "UDPSocket.h" 00017 #include "dnsresolve.h" 00018 #include "gps.h" 00019 #include "BufferSys.h" 00020 #include "I2S_Rx.h" 00021 #include "DSP.h" 00022 #include "comms.h" 00023 00024 // Definitions 00025 00026 // Macros 00027 00028 // Local Data 00029 00030 // Global Data 00031 00032 // Serial port interface to the PC 00033 Serial pc( USBTX, USBRX ); 00034 00035 // LEDs 00036 DigitalOut OnLED( LED1 ); 00037 00038 // IO 00039 DigitalOut TestOscillator( p21 ); 00040 #define TESTOSC_ON 0 00041 #define TESTOSC_OFF 1 00042 00043 // The Buffer pool for audio data 00044 TBufferPool BufferPool; 00045 00046 // The array of buffers for the pool 00047 TBufferData BufferDataArray[NUM_OF_BUFFERS]; 00048 00049 // I2S Object 00050 TI2SReceiver AudioInput( BufferPool ); 00051 00052 // Communications Object 00053 TCommunications Comms; 00054 00055 00056 // Function Prototypes 00057 00058 //--------------------------------------------------------------------------- 00059 // MAIN 00060 //--------------------------------------------------------------------------- 00061 00062 //--------------------------------------------------------------------------- 00063 // 00064 // Main start routine 00065 // Just starts everything running 00066 // 00067 int main() 00068 { 00069 TDSPProcessor ADCBuffer; 00070 uint32_t uiCurrentTestmode = 0x1234; 00071 00072 // Set up IOs 00073 OnLED = 1; 00074 TestOscillator = TESTOSC_OFF; 00075 00076 // Set up PC port 00077 pc.baud( 38400 ); 00078 00079 // Report 00080 printf( "\r\n\r\nQRSS_Rx Application running\r\n" ); 00081 printf( "Built " __DATE__ " " __TIME__ "\r\n" ); 00082 printf( "System Core Clock = %ld\r\n", SystemCoreClock ); 00083 00084 // Add array of buffers to the pool 00085 pc.printf ("Setting up buffer pool with %d buffer of %d samples ...", NUM_OF_BUFFERS, BUFFERSYS_SIZE ); 00086 BufferPool.AddNewMsgsToPool( BufferDataArray, NUM_OF_BUFFERS ); 00087 printf (" OK\r\n"); 00088 00089 // Set up audio receiver 00090 pc.printf ("Initialise the I2S Receiver ..."); 00091 AudioInput.Init(); 00092 printf (" OK\r\n"); 00093 00094 // Set up GPS 00095 pc.printf ("Initialise GPS ..."); 00096 GPSModule.Init(); 00097 printf (" OK\r\n"); 00098 00099 // Set up communications 00100 pc.printf ("Initialise Communications ..."); 00101 Comms.Init(); 00102 Comms.SetServer( "192.168.0.15" ); // default QRSS Server Address (could be a DNS name) 00103 printf (" OK\r\n"); 00104 00105 ADCBuffer.NCOFrequency( TEST_NCO_FREQ ); 00106 00107 // echo GPS data out the PC port 00108 while(1) 00109 { 00110 // Poll the sub-systems 00111 Net::poll(); 00112 GPSModule.Poll(); 00113 Comms.Poll(); 00114 00115 // Handle Comms Mode 00116 if ( Comms.Running() ) 00117 { // online comms 00118 if ( !AudioInput.Running() ) 00119 { 00120 printf( "Starting Processing\r\n" ); 00121 ADCBuffer.NCOPhaseInc( Comms.NCOPhaseInc() ); 00122 AudioInput.Start(); 00123 } 00124 // process and output any received samples 00125 if ( !AudioInput.Empty() ) 00126 { 00127 // ADC buffer will be automatically released 00128 AudioInput.Read( ADCBuffer ); 00129 // Mix the LO 00130 ADCBuffer.MixLO(); 00131 // Filter the result 00132 ADCBuffer.LPF(); 00133 // Output the data to the comms module 00134 for ( int ii=0; ii<8; ii++ ) 00135 Comms.SendSamples( ADCBuffer.Timestamp(), ADCBuffer.FilteredOutput(ii) ); 00136 } 00137 } 00138 else 00139 { // offline comms 00140 if ( AudioInput.Running() ) 00141 { 00142 AudioInput.Stop(); 00143 printf( "Stopping Processing\r\n" ); 00144 } 00145 if ( !AudioInput.Empty() ) 00146 { // discard messages 00147 AudioInput.Read( ADCBuffer ); 00148 ADCBuffer.Release(); 00149 } 00150 } 00151 00152 // Handle Test Modes 00153 if ( Comms.TestMode()!=uiCurrentTestmode ) 00154 { 00155 uiCurrentTestmode = Comms.TestMode(); 00156 if ( uiCurrentTestmode!=0 ) 00157 { // enable test Osc 00158 printf( "Turn ON Test Osc\r\n" ); 00159 TestOscillator = TESTOSC_ON; 00160 } 00161 else 00162 { // disable test Osc 00163 printf( "Turn OFF Test Osc\r\n" ); 00164 TestOscillator = TESTOSC_OFF; 00165 } 00166 } 00167 00168 // Process Keystrokes - debug only 00169 if( pc.readable() ) 00170 { 00171 int iCharIn = pc.getc(); 00172 switch ( iCharIn ) 00173 { 00174 case'1': 00175 printf( "Turn on Test Osc\r\n" ); 00176 TestOscillator = TESTOSC_ON; 00177 break; 00178 case '2': 00179 printf( "Turn off Test Osc\r\n" ); 00180 TestOscillator = TESTOSC_OFF; 00181 break; 00182 case '7': 00183 printf( "AudioInput Start\r\n" ); 00184 AudioInput.Start(); 00185 break; 00186 case '8': 00187 printf( "AudioInput Stop\r\n" ); 00188 AudioInput.Stop(); 00189 break; 00190 case '9': 00191 AudioInput.Report(); 00192 break; 00193 case 'x': 00194 ADCBuffer.NCOFrequency( 15000 ); 00195 break; 00196 case 'y': 00197 ADCBuffer.NCOFrequency( -15000 ); 00198 break; 00199 case 'z': 00200 ADCBuffer.NCOFrequency( 15600 ); 00201 break; 00202 } 00203 } 00204 } 00205 00206 } 00207 00208 //--------------------------------------------------------------------------- 00209 // END 00210 //--------------------------------------------------------------------------- 00211 00212
Generated on Wed Jul 13 2022 23:08:21 by
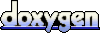