JSON parsing library by Andrii Mamchur https://github.com/amamchur/jsonlite
Dependents: M2X_dev MTS_M2x_Example1 MTS_M2x_Example m2x-demo-all ... more
jsonlite.h
00001 // 00002 // Copyright 2012-2013, Andrii Mamchur 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License 00015 00016 #ifndef JSONLITE_H 00017 #define JSONLITE_H 00018 00019 // #include "jsonlite_builder.h" 00020 // 00021 // Copyright 2012-2013, Andrii Mamchur 00022 // 00023 // Licensed under the Apache License, Version 2.0 (the "License"); 00024 // you may not use this file except in compliance with the License. 00025 // You may obtain a copy of the License at 00026 // 00027 // http://www.apache.org/licenses/LICENSE-2.0 00028 // 00029 // Unless required by applicable law or agreed to in writing, software 00030 // distributed under the License is distributed on an "AS IS" BASIS, 00031 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00032 // See the License for the specific language governing permissions and 00033 // limitations under the License 00034 00035 #ifndef JSONLITE_BUILDER_H 00036 #define JSONLITE_BUILDER_H 00037 00038 #include <stdio.h> 00039 // #include "jsonlite_types.h" 00040 // 00041 // Copyright 2012-2013, Andrii Mamchur 00042 // 00043 // Licensed under the Apache License, Version 2.0 (the "License"); 00044 // you may not use this file except in compliance with the License. 00045 // You may obtain a copy of the License at 00046 // 00047 // http://www.apache.org/licenses/LICENSE-2.0 00048 // 00049 // Unless required by applicable law or agreed to in writing, software 00050 // distributed under the License is distributed on an "AS IS" BASIS, 00051 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00052 // See the License for the specific language governing permissions and 00053 // limitations under the License 00054 00055 #ifndef JSONLITE_TYPES_H 00056 #define JSONLITE_TYPES_H 00057 00058 typedef enum { 00059 jsonlite_result_unknown = -1, 00060 jsonlite_result_ok, 00061 jsonlite_result_end_of_stream, 00062 jsonlite_result_depth_limit, 00063 jsonlite_result_invalid_argument, 00064 jsonlite_result_expected_object_or_array, 00065 jsonlite_result_expected_value, 00066 jsonlite_result_expected_key_or_end, 00067 jsonlite_result_expected_key, 00068 jsonlite_result_expected_colon, 00069 jsonlite_result_expected_comma_or_end, 00070 jsonlite_result_invalid_escape, 00071 jsonlite_result_invalid_number, 00072 jsonlite_result_invalid_token, 00073 jsonlite_result_invalid_utf8, 00074 jsonlite_result_suspended, 00075 00076 jsonlite_result_not_allowed 00077 } jsonlite_result; 00078 00079 00080 #endif 00081 00082 // #include "jsonlite_stream.h" 00083 // 00084 // Copyright 2012-2013, Andrii Mamchur 00085 // 00086 // Licensed under the Apache License, Version 2.0 (the "License"); 00087 // you may not use this file except in compliance with the License. 00088 // You may obtain a copy of the License at 00089 // 00090 // http://www.apache.org/licenses/LICENSE-2.0 00091 // 00092 // Unless required by applicable law or agreed to in writing, software 00093 // distributed under the License is distributed on an "AS IS" BASIS, 00094 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00095 // See the License for the specific language governing permissions and 00096 // limitations under the License 00097 00098 #ifndef JSONLITE_STREAM_H 00099 #define JSONLITE_STREAM_H 00100 00101 #include <stdio.h> 00102 #include <stdint.h> 00103 00104 #ifdef __cplusplus 00105 extern "C" { 00106 #endif 00107 00108 struct jsonlite_stream_struct; 00109 typedef struct jsonlite_stream_struct const * jsonlite_stream; 00110 00111 typedef int (*jsonlite_stream_write_fn)(jsonlite_stream stream, const void *data, size_t length); 00112 typedef void (*jsonlite_stream_release_fn)(jsonlite_stream stream); 00113 00114 int jsonlite_stream_write(jsonlite_stream stream, const void *data, size_t length); 00115 void jsonlite_stream_release(jsonlite_stream stream); 00116 00117 jsonlite_stream jsonlite_mem_stream_init(size_t block_size); 00118 size_t jsonlite_mem_stream_data(jsonlite_stream stream, uint8_t **data, size_t extra_bytes); 00119 00120 jsonlite_stream jsonlite_static_mem_stream_init(void *buffer, size_t size); 00121 size_t jsonlite_static_mem_stream_written_bytes(jsonlite_stream stream); 00122 00123 jsonlite_stream jsonlite_file_stream_init(FILE *file); 00124 00125 extern jsonlite_stream jsonlite_null_stream; 00126 extern jsonlite_stream jsonlite_stdout_stream; 00127 00128 #ifdef __cplusplus 00129 } 00130 #endif 00131 00132 #endif 00133 00134 00135 #ifdef __cplusplus 00136 extern "C" { 00137 #endif 00138 00139 struct jsonlite_builder_struct; 00140 typedef struct jsonlite_builder_struct* jsonlite_builder; 00141 00142 /** @brief Creates and initializes new instance of builder object. 00143 * 00144 * You should release jsonlite_builder object using ::jsonlite_builder_release. 00145 * @see jsonlite_builder 00146 * @see jsonlite_builder_release 00147 * @param depth the builder depth 00148 * @return jsonlite_builder object 00149 */ 00150 jsonlite_builder jsonlite_builder_init(size_t depth, jsonlite_stream stream); 00151 00152 /** \brief Releases builder object. 00153 * 00154 * If builder is NULL, jsonlite_builder_release does nothing. 00155 * @see jsonlite_builder 00156 * @see jsonlite_result 00157 * @param builder the builder object 00158 * @return jsonlite_result_invalid_argument when builder is NULL; otherwise jsonlite_result_ok. 00159 */ 00160 jsonlite_result jsonlite_builder_release(jsonlite_builder builder); 00161 00162 /** \brief Sets beautify indentation. Default is 0. 00163 * 00164 * @see jsonlite_builder 00165 * @see jsonlite_result 00166 * @param builder the builder object 00167 * @param indentation the beautify indentation; 0 - disabled 00168 * @return jsonlite_result_invalid_argument when builder is NULL; otherwise jsonlite_result_ok. 00169 */ 00170 jsonlite_result jsonlite_builder_set_indentation(jsonlite_builder builder, size_t indentation); 00171 00172 /** \brief Sets format for double values. Default is "%.16g". 00173 * 00174 * jsonlite_builder_set_double_format copies format parameter and you can safety release it. 00175 * @see jsonlite_builder 00176 * @see jsonlite_result 00177 * @param builder the builder object 00178 * @param format the double format; see sprintf function for details 00179 * @return jsonlite_result_invalid_argument when builder or format are NULL; otherwise jsonlite_result_ok. 00180 */ 00181 jsonlite_result jsonlite_builder_set_double_format(jsonlite_builder builder, const char *format); 00182 00183 /** \brief Begin JSON object. 00184 * 00185 * @see jsonlite_builder 00186 * @see jsonlite_result 00187 * @param builder the builder object 00188 * @return jsonlite_result_invalid_argument when builder is NULL; 00189 * jsonlite_result_not_allowed when operation is not allowed; 00190 * otherwise jsonlite_result_ok. 00191 */ 00192 jsonlite_result jsonlite_builder_object_begin(jsonlite_builder builder); 00193 00194 /** \brief End JSON object. 00195 * 00196 * @see jsonlite_builder 00197 * @see jsonlite_result 00198 * @param builder the builder object 00199 * @return jsonlite_result_invalid_argument when builder is NULL; 00200 * jsonlite_result_not_allowed when operation is not allowed; 00201 * otherwise jsonlite_result_ok. 00202 */ 00203 jsonlite_result jsonlite_builder_object_end(jsonlite_builder builder); 00204 00205 /** \brief Begin JSON array. 00206 * 00207 * @see jsonlite_builder 00208 * @see jsonlite_result 00209 * @param builder the builder object 00210 * @return jsonlite_result_invalid_argument when builder is NULL; 00211 * jsonlite_result_not_allowed when operation is not allowed; 00212 * otherwise jsonlite_result_ok. 00213 */ 00214 jsonlite_result jsonlite_builder_array_begin(jsonlite_builder builder); 00215 00216 /** \brief End JSON array. 00217 * 00218 * @see jsonlite_builder 00219 * @see jsonlite_result 00220 * @param builder the builder object 00221 * @return jsonlite_result_invalid_argument when builder is NULL; 00222 * jsonlite_result_not_allowed when operation is not allowed; 00223 * otherwise jsonlite_result_ok. 00224 */ 00225 jsonlite_result jsonlite_builder_array_end(jsonlite_builder builder); 00226 00227 /** \brief Write JSON key. 00228 * 00229 * jsonlite_builder_key performs two-character sequence escape for 00230 * U+0022, U+005C, U+002F, U+0008, U+000C, U+000A, U+000D and U+0009 00231 * @see jsonlite_builder 00232 * @see jsonlite_result 00233 * @param builder the builder object 00234 * @param data the UTF-8 encoded string 00235 * @param length the string length 00236 * @return jsonlite_result_invalid_argument when builder or data are NULL; 00237 * jsonlite_result_not_allowed when operation is not allowed; 00238 * otherwise jsonlite_result_ok. 00239 */ 00240 jsonlite_result jsonlite_builder_key(jsonlite_builder builder, const char *data, size_t length); 00241 00242 /** \brief Write string value. 00243 * 00244 * jsonlite_builder_key performs two-character sequence escape for 00245 * U+0022, U+005C, U+002F, U+0008, U+000C, U+000A, U+000D and U+0009 00246 * @see jsonlite_builder 00247 * @see jsonlite_result 00248 * @param builder the builder object 00249 * @param data the UTF-8 encoded string 00250 * @param length the string length 00251 * @return jsonlite_result_invalid_argument when builder or data are NULL; 00252 * jsonlite_result_not_allowed when operation is not allowed; 00253 * otherwise jsonlite_result_ok. 00254 */ 00255 jsonlite_result jsonlite_builder_string(jsonlite_builder builder, const char *data, size_t length); 00256 00257 /** \brief Write integer value. 00258 * 00259 * @see jsonlite_builder 00260 * @see jsonlite_result 00261 * @param builder the builder object 00262 * @param value the integer value 00263 * @return jsonlite_result_invalid_argument when builder is NULL; 00264 * jsonlite_result_not_allowed when operation is not allowed; 00265 * otherwise jsonlite_result_ok. 00266 */ 00267 jsonlite_result jsonlite_builder_int(jsonlite_builder builder, long long value); 00268 00269 /** \brief Write double value. 00270 * 00271 * @see jsonlite_builder 00272 * @see jsonlite_result 00273 * @param builder the builder object 00274 * @param value the double value 00275 * @return jsonlite_result_invalid_argument when builder is NULL; 00276 * jsonlite_result_not_allowed when operation is not allowed; 00277 * otherwise jsonlite_result_ok. 00278 */ 00279 jsonlite_result jsonlite_builder_double(jsonlite_builder builder, double value); 00280 00281 /** \brief Write true value. 00282 * 00283 * @see jsonlite_builder 00284 * @see jsonlite_result 00285 * @param builder the builder object 00286 * @return jsonlite_result_invalid_argument when builder is NULL; 00287 * jsonlite_result_not_allowed when operation is not allowed; 00288 * otherwise jsonlite_result_ok. 00289 */ 00290 jsonlite_result jsonlite_builder_true(jsonlite_builder builder); 00291 00292 /** \brief Write false value. 00293 * 00294 * @see jsonlite_builder 00295 * @see jsonlite_result 00296 * @param builder the builder object 00297 * @return jsonlite_result_invalid_argument when builder is NULL; 00298 * jsonlite_result_not_allowed when operation is not allowed; 00299 * otherwise jsonlite_result_ok. 00300 */ 00301 jsonlite_result jsonlite_builder_false(jsonlite_builder builder); 00302 00303 /** \brief Write null value. 00304 * 00305 * @see jsonlite_builder 00306 * @see jsonlite_result 00307 * @param builder the builder object 00308 * @return jsonlite_result_invalid_argument when builder is NULL; 00309 * jsonlite_result_not_allowed when operation is not allowed; 00310 * otherwise jsonlite_result_ok. 00311 */ 00312 jsonlite_result jsonlite_builder_null(jsonlite_builder builder); 00313 00314 /** \brief Write raw key. 00315 * 00316 * jsonlite_builder_raw_key does not perform any transformation. 00317 * jsonlite_builder_raw_key wraps raw key with '"' (U+0022). 00318 * If data already was wrapped with '"' use following practice jsonlite_builder_raw_key(d, data + 1, size - 2); 00319 * @see jsonlite_builder 00320 * @see jsonlite_result 00321 * @param builder the builder object 00322 * @param data the raw data 00323 * @param length the data length 00324 * @return jsonlite_result_invalid_argument when builder or data are NULL; 00325 * jsonlite_result_not_allowed when operation is not allowed; 00326 * otherwise jsonlite_result_ok. 00327 */ 00328 jsonlite_result jsonlite_builder_raw_key(jsonlite_builder builder, const void *data, size_t length); 00329 00330 /** \brief Write raw string. 00331 * 00332 * jsonlite_builder_raw_string does not perform any transformation. 00333 * jsonlite_builder_raw_string wraps raw string with '"' (U+0022). 00334 * If data already was wrapped with '"' use following practice jsonlite_builder_raw_string(d, data + 1, size - 2); 00335 * @see jsonlite_builder 00336 * @see jsonlite_result 00337 * @param builder the builder object 00338 * @param data the raw data 00339 * @param length the data length 00340 * @return jsonlite_result_invalid_argument when builder or data are NULL; 00341 * jsonlite_result_not_allowed when operation is not allowed; 00342 * otherwise jsonlite_result_ok. 00343 */ 00344 jsonlite_result jsonlite_builder_raw_string(jsonlite_builder builder, const void *data, size_t length); 00345 00346 /** \brief Write raw value. 00347 * 00348 * jsonlite_builder_raw_value does not perform any transformation. 00349 * jsonlite_builder_raw_value does not wrap raw value with '"' (U+0022). 00350 * @see jsonlite_builder 00351 * @see jsonlite_result 00352 * @param builder the builder object 00353 * @param data the raw data 00354 * @param length the data length 00355 * @return jsonlite_result_invalid_argument when builder or data are NULL; 00356 * jsonlite_result_not_allowed when operation is not allowed; 00357 * otherwise jsonlite_result_ok. 00358 */ 00359 jsonlite_result jsonlite_builder_raw_value(jsonlite_builder builder, const void *data, size_t length); 00360 00361 jsonlite_result jsonlite_builder_base64_value(jsonlite_builder builder, const void *data, size_t length); 00362 00363 #ifdef __cplusplus 00364 } 00365 #endif 00366 00367 #endif 00368 00369 // #include "jsonlite_parser.h" 00370 // 00371 // Copyright 2012-2013, Andrii Mamchur 00372 // 00373 // Licensed under the Apache License, Version 2.0 (the "License"); 00374 // you may not use this file except in compliance with the License. 00375 // You may obtain a copy of the License at 00376 // 00377 // http://www.apache.org/licenses/LICENSE-2.0 00378 // 00379 // Unless required by applicable law or agreed to in writing, software 00380 // distributed under the License is distributed on an "AS IS" BASIS, 00381 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00382 // See the License for the specific language governing permissions and 00383 // limitations under the License 00384 00385 #ifndef JSONLITE_PARSER_H 00386 #define JSONLITE_PARSER_H 00387 00388 #include <stdio.h> 00389 #include <stddef.h> 00390 #include <stdint.h> 00391 // #include "jsonlite_token.h" 00392 // 00393 // Copyright 2012-2013, Andrii Mamchur 00394 // 00395 // Licensed under the Apache License, Version 2.0 (the "License"); 00396 // you may not use this file except in compliance with the License. 00397 // You may obtain a copy of the License at 00398 // 00399 // http://www.apache.org/licenses/LICENSE-2.0 00400 // 00401 // Unless required by applicable law or agreed to in writing, software 00402 // distributed under the License is distributed on an "AS IS" BASIS, 00403 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00404 // See the License for the specific language governing permissions and 00405 // limitations under the License 00406 00407 #ifndef JSONLITE_TOKEN_H 00408 #define JSONLITE_TOKEN_H 00409 00410 #include <stdio.h> 00411 #include <stddef.h> 00412 #include <stdint.h> 00413 00414 #ifdef __cplusplus 00415 extern "C" { 00416 #endif 00417 struct jsonlite_token; 00418 struct jsonlite_parser_struct; 00419 00420 /** @brief Provides the hints for number token parsing. 00421 * 00422 * This values is valid for jsonlite_parser_callbacks::number_found callback only. 00423 */ 00424 typedef enum { 00425 /** @brief Indicates that number token has integer part. 00426 * 00427 * @note 00428 * This flag is always set because of JSON number always has integer part (value .123 is not allowed). 00429 */ 00430 jsonlite_number_int = 0x01, 00431 00432 /** @brief Indicates that number token has fraction part. 00433 * 00434 * This flag will set if token has an fraction part. For example: 123.987; 00435 * in current case fraction part is .987. 00436 */ 00437 jsonlite_number_frac = 0x02, 00438 00439 /** @brief Indicates that number token has exponent part. 00440 * 00441 * This flag will set if token has an exponent part. 00442 * 00443 * For example: 00444 * For integer values: 123e5, 123E5, 123E+5, 123e+5; 00445 * all of this numbers are equal to each other and has exponent part. 00446 * 00447 * An other case 12300000 is also equals to previous numbers but has no exponent part. 00448 * 00449 * For floating point values: 123.01e5, 123.01E5, 123.01E+5, 123.01e+5; 00450 * all of this number are equal to each other and has exponent part. 00451 * An other case 12301000 is also equals to previous numbers but has no exponent part. 00452 */ 00453 jsonlite_number_exp = 0x04, 00454 00455 /** @brief Indicates that number token has negative value. 00456 * 00457 * This flag will set if token starts with '-' character. 00458 */ 00459 jsonlite_number_negative = 0x08, 00460 00461 /** @brief Indicates that number token starts with zero character. 00462 */ 00463 jsonlite_number_zero_leading = 0x10, 00464 \ 00465 /** @brief Indicates that number token starts with digit that is greater 0. 00466 */ 00467 jsonlite_number_digit_leading = 0x20 00468 } jsonlite_number_type; 00469 00470 /** @brief Provides the hints for string token parsing. 00471 * 00472 * This values is valid for jsonlite_parser_callbacks::string_found 00473 * and jsonlite_parser_callbacks::key_found callbacks only. 00474 */ 00475 typedef enum { 00476 /** @brief Indicates that string token contains ASCII characters. 00477 * 00478 * @note 00479 * This flag is always set because of JSON string always has ASCII characters. 00480 */ 00481 jsonlite_string_ascii = 0x01, 00482 00483 /** @brief Indicates that string token has the sequences of UTF-8 characters. 00484 * 00485 * @note 00486 * This flag will set if string token has 2, 3 or 4 subsequently. 00487 */ 00488 jsonlite_string_utf8 = 0x02, 00489 00490 /** @brief Indicates that string token has an escaped character(s). 00491 * 00492 * This flag will be set if string token has one or more following escaped character: 00493 * - \\" 00494 * - \\\\ 00495 * - \\n 00496 * - \\r 00497 * - \\/ 00498 * - \\b 00499 * - \\f 00500 * - \\t 00501 */ 00502 jsonlite_string_escape = 0x04, 00503 00504 /** @brief Indicates that string token has one or more unicode escaped character(s). 00505 * 00506 * This flag will be set if string token has \\uXXXX escape - where (XXXX is an unicode character code) 00507 */ 00508 jsonlite_string_unicode_escape = 0x08, 00509 00510 00511 /** @brief Indicates that string token has one or more unicode noncharacter(s). 00512 * 00513 * This flag will be set if string token has \\uFDD0-\\uFDEF and \\uFFFE-\\uFFFF unicode character 00514 */ 00515 jsonlite_string_unicode_noncharacter = 0x10 00516 } jsonlite_string_type; 00517 00518 /** @brief Contains information about parsed token. 00519 */ 00520 typedef struct jsonlite_token { 00521 /** @brief This variable is reserved for high-level libraries. 00522 */ 00523 void *ext; 00524 00525 /** @brief Contains the start position of token. 00526 */ 00527 const uint8_t *start; 00528 00529 /** @brief Contains the end position of tokens. 00530 * 00531 * End position does not below to token, it should be interpreted as position of zero character. 00532 * @note 00533 * To measure token length you can use following expression: token->end - token->start. 00534 */ 00535 const uint8_t *end; 00536 00537 /** @brief Contains the hints for token parsing. 00538 */ 00539 union { 00540 /** @brief Contains the hints for number token parsing. 00541 */ 00542 jsonlite_number_type number; 00543 00544 /** @brief Contains the hints for string token parsing. 00545 */ 00546 jsonlite_string_type string; 00547 } type; 00548 } jsonlite_token; 00549 00550 00551 /** @brief Returns a size of memory that is required for token conversion to UTF-8 string. 00552 * @param ts jsonlite token 00553 * @return 0 if ts is NULL; otherwise required size of for token conversion. 00554 */ 00555 size_t jsonlite_token_size_of_uft8(jsonlite_token *ts); 00556 00557 /** @brief Converts specified token to UTF-8 string. 00558 * 00559 * Function converts specified token to UTF-8 string encoding and copy zero terminated string to buffer. 00560 * @note 00561 * Function will alloc memory by itself if *buffer == NULL. 00562 * In this case you are responsible for memory releasing by using free() function. 00563 * @param ts jsonlite token 00564 * @return length in bytes of converted string. 00565 */ 00566 size_t jsonlite_token_to_uft8(jsonlite_token *ts, uint8_t **buffer); 00567 00568 /** @brief Returns a size of memory that is required for token conversion to UTF-16 string. 00569 * @param ts jsonlite token 00570 * @return 0 if ts is NULL; otherwise required size of for token conversion. 00571 */ 00572 size_t jsonlite_token_size_of_uft16(jsonlite_token *ts); 00573 00574 /** @brief Converts specified token to UTF-16 string. 00575 * 00576 * Function converts specified token to UTF-16 string encoding and copy zero terminated string to buffer. 00577 * @note 00578 * Function will alloc memory by itself if *buffer == NULL. 00579 * In this case you are responsible for memory releasing by using free() function. 00580 * @param ts jsonlite token 00581 * @return length in bytes of converted string. 00582 */ 00583 size_t jsonlite_token_to_uft16(jsonlite_token *ts, uint16_t **buffer); 00584 00585 size_t jsonlite_token_size_of_base64_binary(jsonlite_token *ts); 00586 size_t jsonlite_token_base64_to_binary(jsonlite_token *ts, void **buffer); 00587 00588 long jsonlite_token_to_long(jsonlite_token *token); 00589 long long jsonlite_token_to_long_long(jsonlite_token *token); 00590 00591 #ifdef __cplusplus 00592 } 00593 #endif 00594 00595 #endif 00596 00597 // #include "jsonlite_types.h" 00598 00599 00600 #ifdef __cplusplus 00601 extern "C" { 00602 #endif 00603 00604 struct jsonlite_parser_struct; 00605 typedef struct jsonlite_parser_struct* jsonlite_parser; 00606 00607 /** @brief Contains callback information. 00608 */ 00609 typedef struct { 00610 /** @brief jsonlite parser object that initiate callback. 00611 * @note 00612 * You can use ::jsonlite_parser_suspend to stop tokenization. 00613 */ 00614 jsonlite_parser parser; 00615 00616 /** @brief Reserved for client usage. 00617 */ 00618 void *client_state; 00619 } jsonlite_callback_context; 00620 00621 /** @brief Type of value callback function. 00622 */ 00623 typedef void (*jsonlite_value_callback)(jsonlite_callback_context *, jsonlite_token *); 00624 00625 /** @brief Type of state callback function. 00626 */ 00627 typedef void (*jsonlite_state_callback)(jsonlite_callback_context *); 00628 00629 /** @brief Contains references to client callback functions. 00630 * 00631 * You can use the global jsonlite_default_callbacks constant to initialize default values. 00632 */ 00633 typedef struct { 00634 /** @brief Called when parser finished tokenization. 00635 * You can retrieve result of parsing using jsonlite_parser_get_result. 00636 */ 00637 jsonlite_state_callback parse_finished; 00638 00639 /** @brief Called when parser found object start. 00640 */ 00641 jsonlite_state_callback object_start; 00642 00643 /** @brief Called when parser found object end. 00644 */ 00645 jsonlite_state_callback object_end; 00646 00647 /** @brief Called when parser found array start. 00648 */ 00649 jsonlite_state_callback array_start; 00650 00651 /** @brief Called when parser found array end. 00652 */ 00653 jsonlite_state_callback array_end; 00654 00655 /** @brief Called when parser found \a true token. 00656 */ 00657 jsonlite_state_callback true_found; 00658 00659 /** @brief Called when parser found \a false token. 00660 */ 00661 jsonlite_state_callback false_found; 00662 00663 /** @brief Called when parser found \a null token. 00664 */ 00665 jsonlite_state_callback null_found; 00666 00667 /** @brief Called when parser found key token. 00668 */ 00669 jsonlite_value_callback key_found; 00670 00671 /** @brief Called when parser found string token. 00672 */ 00673 jsonlite_value_callback string_found; 00674 00675 /** @brief Called when parser found number token. 00676 */ 00677 jsonlite_value_callback number_found; 00678 00679 /** @brief Callbacks' context, will be past as first parameter of callback function. 00680 */ 00681 jsonlite_callback_context context; 00682 } jsonlite_parser_callbacks; 00683 00684 /** @brief Estimates memory usage. 00685 * @note 00686 * This value depends on CPU architectures. 00687 * @param depth the parsing depth. 00688 * @return Estimated size in bytes. 00689 */ 00690 size_t jsonlite_parser_estimate_size(size_t depth); 00691 00692 /** @brief Creates and initializes new instance of parser object. 00693 * 00694 * You should release jsonlite_parser object using ::jsonlite_parser_release. 00695 * @see jsonlite_parser 00696 * @see jsonlite_parser_release 00697 * @param depth the parsing depth. 00698 * @return jsonlite_parser object. 00699 */ 00700 jsonlite_parser jsonlite_parser_init(size_t depth); 00701 00702 /** @brief Initializes memory for parser object. 00703 * 00704 * You should release internal resources using ::jsonlite_parser_cleanup 00705 * @see jsonlite_parser 00706 * @see jsonlite_parser_reset 00707 * @param memory the memory for parser. 00708 * @param size the memory size. 00709 * @return jsonlite_parser object. 00710 */ 00711 jsonlite_parser jsonlite_parser_init_memory(void *memory, size_t size); 00712 00713 /** \brief Copies provided callbacks structure to parser object. 00714 * @see jsonlite_parser 00715 * @see jsonlite_parser_callbacks 00716 * @see jsonlite_result 00717 * @param parser the parser object. 00718 * @param parser the callbacks object. 00719 * @return jsonlite_result_invalid_argument when parser or cbs are NULL; otherwise jsonlite_result_ok. 00720 */ 00721 jsonlite_result jsonlite_parser_set_callback(jsonlite_parser parser, const jsonlite_parser_callbacks *cbs); 00722 00723 /** \brief Returns result of last operation. 00724 * @see jsonlite_parser 00725 * @see jsonlite_result 00726 * @param parser the parser object. 00727 * @return jsonlite_result_invalid_argument when parser is NULL; otherwise s result of last operation. 00728 */ 00729 jsonlite_result jsonlite_parser_get_result(jsonlite_parser parser); 00730 00731 /** \brief Performs JSON tokenization. 00732 * 00733 * jsonlite is a chunk parser and you can use this function to parser a fragment of JSON. 00734 * @see jsonlite_parser 00735 * @see jsonlite_result 00736 * @param parser the parser object. 00737 * @param buffer the pointer to JSON payload buffer. 00738 * @param size the JSON payload buffer size. 00739 * @return JSON parsing result or jsonlite_result_invalid_argument when some parameter is invalid. 00740 * 00741 * There is an example of JSON validation 00742 * @code{.c} 00743 * char json[] = "{\"key\" : 12345, \"obj\": {}, \"array\":[null, true, false, \"string\"]}"; 00744 * jsonlite_parser p = jsonlite_parser_init(16); 00745 * jsonlite_result result = jsonlite_parser_tokenize(p, json, sizeof(json)); 00746 * assert(result == jsonlite_result_ok); 00747 * jsonlite_parser_release(p); 00748 * @endcode 00749 * 00750 * There is an another example of JSON chunk parsing. 00751 * @code{.c} 00752 * char chunk1[] = "{\"key\" : 12345, \"obj\": {}, \"arr"; 00753 * char chunk2[] = "ay\":[null, true, false, \"string\"]}"; 00754 * jsonlite_parser p = jsonlite_parser_init(16); 00755 * 00756 * jsonlite_result result = jsonlite_parser_tokenize(p, chunk1, sizeof(chunk1) - 1); 00757 * assert(result == jsonlite_result_end_of_stream); 00758 * // Now you can release or reuse chunk1 buffer. 00759 * 00760 * result = jsonlite_parser_tokenize(p, chunk2, sizeof(chunk2) - 1); 00761 * assert(result == jsonlite_result_ok); 00762 * 00763 * jsonlite_parser_release(p); 00764 * @endcode 00765 */ 00766 jsonlite_result jsonlite_parser_tokenize(jsonlite_parser parser, const void *buffer, size_t size); 00767 00768 /** \brief Resumes JSON tokenization. 00769 * @see jsonlite_parser 00770 * @see jsonlite_result 00771 * @param parser the parser object. 00772 * @return JSON parsing result or jsonlite_result_invalid_argument when parser is NULL. 00773 */ 00774 jsonlite_result jsonlite_parser_resume(jsonlite_parser parser); 00775 00776 /** \brief Suspends JSON tokenization. 00777 * 00778 * You can continue tokenization later by calling ::jsonlite_parser_resume. 00779 * @see jsonlite_parser 00780 * @see jsonlite_result 00781 * @param parser the parser object. 00782 * @return jsonlite_result_invalid_argument when parser is NULL; 00783 * jsonlite_result_not_allowed when operation is not allowed; 00784 * otherwise jsonlite_result_ok. 00785 */ 00786 jsonlite_result jsonlite_parser_suspend(jsonlite_parser parser); 00787 00788 /** \brief Terminate JSON tokenization. 00789 * 00790 * @see jsonlite_parser 00791 * @see jsonlite_result 00792 * @param parser the parser object. 00793 * @return jsonlite_result_invalid_argument when parser is NULL or result is jsonlite_result_unknown; 00794 * otherwise jsonlite_result_ok. 00795 */ 00796 jsonlite_result jsonlite_parser_terminate(jsonlite_parser parser, jsonlite_result result); 00797 00798 /** \brief Releases parser object. 00799 * 00800 * If parser is NULL, jsonlite_parser_release does nothing. 00801 * @see jsonlite_parser 00802 * @param parser the parser object. 00803 */ 00804 void jsonlite_parser_release(jsonlite_parser parser); 00805 00806 /** \brief Releases internal resources and states. 00807 * 00808 * If parser is NULL, jsonlite_parser_reset does nothing. 00809 * @see jsonlite_parser 00810 * @param parser the parser object. 00811 */ 00812 void jsonlite_parser_cleanup(jsonlite_parser parser); 00813 00814 /** \brief jsonlite_parser_callbacks structure initialized with callbacks that do nothing. 00815 */ 00816 extern const jsonlite_parser_callbacks jsonlite_default_callbacks; 00817 00818 #ifdef __cplusplus 00819 } 00820 #endif 00821 00822 #endif 00823 00824 // #include "jsonlite_stream.h" 00825 00826 // #include "jsonlite_token.h" 00827 00828 // #include "jsonlite_token_pool.h" 00829 // 00830 // Copyright 2012-2013, Andrii Mamchur 00831 // 00832 // Licensed under the Apache License, Version 2.0 (the "License"); 00833 // you may not use this file except in compliance with the License. 00834 // You may obtain a copy of the License at 00835 // 00836 // http://www.apache.org/licenses/LICENSE-2.0 00837 // 00838 // Unless required by applicable law or agreed to in writing, software 00839 // distributed under the License is distributed on an "AS IS" BASIS, 00840 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00841 // See the License for the specific language governing permissions and 00842 // limitations under the License 00843 00844 #ifndef JSONLITE_TOKEN_POOL_H 00845 #define JSONLITE_TOKEN_POOL_H 00846 00847 // #include "jsonlite_token.h" 00848 00849 00850 #ifdef __cplusplus 00851 extern "C" { 00852 #endif 00853 00854 typedef void (*jsonlite_token_pool_release_value_fn)(void *); 00855 typedef struct jsonlite_token_pool_struct* jsonlite_token_pool; 00856 00857 typedef struct jsonlite_token_bucket { 00858 const uint8_t *start; 00859 const uint8_t *end; 00860 00861 ptrdiff_t hash; 00862 ptrdiff_t value_hash; 00863 00864 const void *value; 00865 } jsonlite_token_bucket; 00866 00867 jsonlite_token_pool jsonlite_token_pool_create(jsonlite_token_pool_release_value_fn release_fn); 00868 void jsonlite_token_pool_copy_tokens(jsonlite_token_pool pool); 00869 void jsonlite_token_pool_release(jsonlite_token_pool pool); 00870 jsonlite_token_bucket* jsonlite_token_pool_get_bucket(jsonlite_token_pool pool, jsonlite_token *token); 00871 00872 #ifdef __cplusplus 00873 } 00874 #endif 00875 00876 #endif 00877 00878 00879 extern const char *jsonlite_version; 00880 00881 #endif
Generated on Thu Jul 28 2022 08:30:58 by
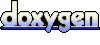