
MBED experiment.
Embed:
(wiki syntax)
Show/hide line numbers
Autoline.h
00001 #include "mbed.h" 00002 00003 // Our libraries 00004 #include "Connectivity.h" 00005 #include "Digitalio.h" 00006 #include <iostream> 00007 #include <sstream> 00008 00009 #include <vector> 00010 using namespace std; 00011 00012 #ifndef AUTOLINE_AUTOLINE_H_ 00013 #define AUTOLINE_AUTOLINE_H_ 00014 00015 #define MAX_SIZE_OF_CMMD 5 00016 00017 00018 // General states 00019 #define MAINTENANCE_MODE 0 00020 #define AUTOMATIC_MODE 1 00021 #define EMERGENCY_MODE 2 00022 #define PAUSED_MODE 3 00023 00024 00025 // Autoline's network protocol 00026 #define CMMD_1 '1' // Read I/Os 0-79 Sensors - {1} 00027 #define CMMD_2 '2' // Activate actuator - {2 XX Y} XX - Actuator ADDR || Y = 1 or 0 (on or off) 00028 //#define CMMD_3 '3' // Start test (CLP never receives it) 00029 #define CMMD_4 '4' // Read I/Os 80-179 Actuators - {4} 00030 #define CMMD_5 '5' // Enter MAINTENANCE MODE - {5} 00031 #define CMMD_6 '6' // Enter AUTOMATIC MODE - {6} 00032 #define CMMD_7 '7' // Test finished - {7 X} X - Station where test has finished (B being the release button on power station) 00033 #define CMMD_S 'S' // Configure SKIP - {S X X X X} X - 1 or 0 (1 to do the test, 0 to skip it) 00034 #define CMMD_P 'P' // Safe pause - {P X} x - 1 or 0 (1 to pause, 0 to leave pause) 00035 #define CMMD_E 'E' // Emergency command - {E} - IHM Asks if its on emergency mode or not 00036 00037 class Autoline { 00038 public: 00039 Autoline(); 00040 virtual ~Autoline(); 00041 00042 00043 void run(); 00044 void parser(char *cmmd, int size); 00045 00046 private: 00047 // Attributes 00048 00049 Connectivity connection; 00050 DigitalIO dio; 00051 Serial pc; 00052 00053 short int state; // It could be a char for less memory usage 00054 00055 00056 vector<char*> queue; 00057 00058 void protocoler(char *receivedFromEth, int size); 00059 void print_queue(); 00060 00061 std::string read_ios_in_range(int start, int end); 00062 00063 // General functions 00064 void set_state(short int newState); 00065 00066 // CMMD's functions 00067 void read_sensors(); 00068 00069 // Stations 00070 void input_elevator(); 00071 void supply_station(); 00072 void hipot_wait_station(); 00073 void hipot_station(); 00074 void pf_station(); 00075 void ate_wait_station(); 00076 void ate1_station(); 00077 void ate2_station(); 00078 void eprom_station(); 00079 void remove_station(); 00080 void output_elevator(); 00081 00082 Mutex _mutex; 00083 }; 00084 00085 00086 #endif
Generated on Mon Jul 25 2022 15:13:16 by
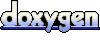