Mbed 4dGenie class
Dependents: Genie_Test 039847382-S3_DS1621_and_LCD_V1
mbed_genie.h
00001 00002 00003 // Genie commands & replys: 00004 00005 #define GENIE_ACK 0x06 00006 #define GENIE_NAK 0x15 00007 00008 #define TIMEOUT_PERIOD 500 00009 #define RESYNC_PERIOD 100 00010 00011 #define GENIE_READ_OBJ 0 00012 #define GENIE_WRITE_OBJ 1 00013 #define GENIE_WRITE_STR 2 00014 #define GENIE_WRITE_STRU 3 00015 #define GENIE_WRITE_CONTRAST 4 00016 #define GENIE_REPORT_OBJ 5 00017 #define GENIE_REPORT_EVENT 7 00018 00019 // Objects 00020 // the manual says: 00021 // Note: Object IDs may change with future releases; it is not 00022 // advisable to code their values as constants. 00023 00024 #define GENIE_OBJ_DIPSW 0 00025 #define GENIE_OBJ_KNOB 1 00026 #define GENIE_OBJ_ROCKERSW 2 00027 #define GENIE_OBJ_ROTARYSW 3 00028 #define GENIE_OBJ_SLIDER 4 00029 #define GENIE_OBJ_TRACKBAR 5 00030 #define GENIE_OBJ_WINBUTTON 6 00031 #define GENIE_OBJ_ANGULAR_METER 7 00032 #define GENIE_OBJ_COOL_GAUGE 8 00033 #define GENIE_OBJ_CUSTOM_DIGITS 9 00034 #define GENIE_OBJ_FORM 10 00035 #define GENIE_OBJ_GAUGE 11 00036 #define GENIE_OBJ_IMAGE 12 00037 #define GENIE_OBJ_KEYBOARD 13 00038 #define GENIE_OBJ_LED 14 00039 #define GENIE_OBJ_LED_DIGITS 15 00040 #define GENIE_OBJ_METER 16 00041 #define GENIE_OBJ_STRINGS 17 00042 #define GENIE_OBJ_THERMOMETER 18 00043 #define GENIE_OBJ_USER_LED 19 00044 #define GENIE_OBJ_VIDEO 20 00045 #define GENIE_OBJ_STATIC_TEXT 21 00046 #define GENIE_OBJ_SOUND 22 00047 #define GENIE_OBJ_TIMER 23 00048 #define GENIE_OBJ_SPECTRUM 24 00049 #define GENIE_OBJ_SCOPE 25 00050 #define GENIE_OBJ_TANK 26 00051 #define GENIE_OBJ_USERIMAGES 27 00052 #define GENIE_OBJ_PINOUTPUT 28 00053 #define GENIE_OBJ_PININPUT 29 00054 #define GENIE_OBJ_4DBUTTON 30 00055 #define GENIE_OBJ_ANIBUTTON 31 00056 #define GENIE_OBJ_COLORPICKER 32 00057 #define GENIE_OBJ_USERBUTTON 33 00058 00059 // Structure to store replys returned from a display 00060 00061 #define GENIE_FRAME_SIZE 6 00062 00063 #define ERROR_NONE 0 00064 #define ERROR_TIMEOUT -1 // 255 0xFF 00065 #define ERROR_NOHANDLER -2 // 254 0xFE 00066 #define ERROR_NOCHAR -3 // 253 0xFD 00067 #define ERROR_NAK -4 // 252 0xFC 00068 #define ERROR_REPLY_OVR -5 // 251 0xFB 00069 #define ERROR_RESYNC -6 // 250 0xFA 00070 #define ERROR_NODISPLAY -7 // 249 0xF9 00071 #define ERROR_BAD_CS -8 // 248 0xF8 00072 00073 #define GENIE_LINK_IDLE 0 00074 #define GENIE_LINK_WFAN 1 // waiting for Ack or Nak 00075 #define GENIE_LINK_WF_RXREPORT 2 // waiting for a report frame 00076 #define GENIE_LINK_RXREPORT 3 // receiving a report frame 00077 #define GENIE_LINK_RXEVENT 4 // receiving an event frame 00078 #define GENIE_LINK_SHDN 5 00079 00080 #define GENIE_EVENT_NONE 0 00081 #define GENIE_EVENT_RXCHAR 1 00082 00083 #ifndef TRUE 00084 #define TRUE (1==1) 00085 #define FALSE (!TRUE) 00086 #endif 00087 00088 #define NO_RESPONSE 0 00089 00090 #define GENIE_FRAME_SIZE 6 00091 // Structure to store replys returned from a display 00092 struct genieFrameReportObj { 00093 uint8_t cmd; 00094 uint8_t object; 00095 uint8_t index; 00096 uint8_t data_msb; 00097 uint8_t data_lsb; 00098 }; 00099 ///////////////////////////////////////////////////////////////////// 00100 // The Genie frame definition 00101 // 00102 // The union allows the data to be referenced as an array of uint8_t 00103 // or a structure of type genieFrameReportObj, eg 00104 // 00105 // genieFrame f; 00106 // f.bytes[4]; 00107 // f.reportObject.data_lsb 00108 // 00109 // both methods get the same byte 00110 // 00111 union genieFrame { 00112 uint8_t bytes[GENIE_FRAME_SIZE]; 00113 genieFrameReportObj reportObject; 00114 }; 00115 #define MAX_GENIE_EVENTS 16 // MUST be a power of 2 00116 struct genieEventQueueStruct { 00117 genieFrame frames[MAX_GENIE_EVENTS]; 00118 uint8_t rd_index; 00119 uint8_t wr_index; 00120 uint8_t n_events; 00121 }; 00122 00123 typedef void (*genieUserEventHandlerPtr) (void); 00124 00125 class Mbed4dGenie{ 00126 public: 00127 00128 typedef enum{ 00129 CommIdle = 0, 00130 CommInProgress = 1 00131 }CommState; 00132 00133 /* 00134 Constructor to initialise the pins used to control the lcd screen 00135 Note: When the class is first initialised, the lcd screen will be put 00136 in reset and kept reset until Start() is called 00137 */ 00138 Mbed4dGenie(PinName TxPin,PinName RxPin, PinName resetpin); 00139 Mbed4dGenie(PinName TxPin,PinName RxPin, PinName resetpin,uint32_t baud); 00140 /* 00141 Deassert the reset pin and give some time to the lcd to 00142 initialise itself before sending any commands. 00143 */ 00144 void Start(); 00145 void Reset(void); 00146 /* 00147 Generic command to write data to a Genie object 00148 returns the status code of operation 00149 */ 00150 int8_t genieWriteObject (uint16_t object, uint16_t index, uint16_t data); 00151 int8_t genieWriteStr (uint16_t index, char *string); 00152 int8_t genieReadObj (uint16_t object, uint16_t index); 00153 00154 bool genieDequeueEvent(genieFrame * buff); 00155 void genieAttachEventHandler (genieUserEventHandlerPtr handler); 00156 bool PendingFrames(void); 00157 00158 uint16_t genieGetEventData (genieFrame * e); //added by to read value of object (combine MSB and LSB in a single variable) 00159 00160 private: 00161 void writec(char data); 00162 void RxIrqHandler(void); 00163 void ManageReceiveData(char data); 00164 bool _genieEnqueueEvent (uint8_t * data); 00165 void _genieFlushEventQueue(void); 00166 /* 00167 Wait for the screen to aknowledge the command 00168 returns the status code of operation 00169 */ 00170 int8_t WaitForAnswer(); 00171 bool WaitForIdle(); 00172 int8_t WaitForReadAnswer(); 00173 00174 ////////////////////////////////////////////////////////////// 00175 // A structure to hold up to MAX_GENIE_EVENTS events receive 00176 // from the display 00177 // 00178 genieEventQueueStruct _genieEventQueue; 00179 uint8_t rxframe_count; 00180 uint8_t rx_data[GENIE_FRAME_SIZE]; 00181 uint8_t checksum; 00182 ////////////////////////////////////////////////////////////// 00183 // Pointer to the user's event handler function 00184 // 00185 genieUserEventHandlerPtr _genieUserHandler; 00186 00187 RawSerial _screen; 00188 DigitalOut _reset; 00189 Timer _waitTimer,_receptionTimer; 00190 int state; 00191 int LastResponse; 00192 long RxMaxTimeout; 00193 long RxStateTimeoutErrors; 00194 };
Generated on Sat Jul 16 2022 01:44:22 by
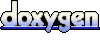