Embed:
(wiki syntax)
Show/hide line numbers
USBDevice_Types.h
00001 /* USBDevice_Types.h */ 00002 /* USB Device type definitions, conversions and constants */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef USB_DEVICE_TYPES 00006 #define USB_DEVICE_TYPES 00007 00008 /* Standard requests */ 00009 #define GET_STATUS (0) 00010 #define CLEAR_FEATURE (1) 00011 #define SET_FEATURE (3) 00012 #define SET_ADDRESS (5) 00013 #define GET_DESCRIPTOR (6) 00014 #define SET_DESCRIPTOR (7) 00015 #define GET_CONFIGURATION (8) 00016 #define SET_CONFIGURATION (9) 00017 #define GET_INTERFACE (10) 00018 #define SET_INTERFACE (11) 00019 00020 /* bmRequestType.dataTransferDirection */ 00021 #define HOST_TO_DEVICE (0) 00022 #define DEVICE_TO_HOST (1) 00023 00024 /* bmRequestType.Type*/ 00025 #define STANDARD_TYPE (0) 00026 #define CLASS_TYPE (1) 00027 #define VENDOR_TYPE (2) 00028 #define RESERVED_TYPE (3) 00029 00030 /* bmRequestType.Recipient */ 00031 #define DEVICE_RECIPIENT (0) 00032 #define INTERFACE_RECIPIENT (1) 00033 #define ENDPOINT_RECIPIENT (2) 00034 #define OTHER_RECIPIENT (3) 00035 00036 /* Descriptors */ 00037 #define DESCRIPTOR_TYPE(wValue) (wValue >> 8) 00038 #define DESCRIPTOR_INDEX(wValue) (wValue & 0xf) 00039 00040 typedef struct { 00041 struct { 00042 uint8_t dataTransferDirection; 00043 uint8_t Type; 00044 uint8_t Recipient; 00045 } bmRequestType; 00046 uint8_t bRequest; 00047 uint16_t wValue; 00048 uint16_t wIndex; 00049 uint16_t wLength; 00050 } SETUP_PACKET; 00051 00052 typedef struct { 00053 SETUP_PACKET setup; 00054 uint8_t *ptr; 00055 uint32_t remaining; 00056 uint8_t direction; 00057 bool zlp; 00058 bool notify; 00059 } CONTROL_TRANSFER; 00060 00061 typedef enum {ATTACHED, POWERED, DEFAULT, ADDRESS, CONFIGURED} DEVICE_STATE; 00062 00063 typedef struct { 00064 DEVICE_STATE state; 00065 uint8_t configuration; 00066 bool suspended; 00067 } USB_DEVICE; 00068 00069 #endif
Generated on Fri Jul 15 2022 02:22:27 by
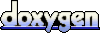